We read every piece of feedback, and take your input very seriously.
To see all available qualifiers, see our documentation.
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
At the beginning of chapter 5 I try to compile previously added code in chapter 4 and receive the following output:
Here's my Greeter.sol code: `pragma solidity ^0.8.0;
import "@openzeppelin/contracts/access/Ownable.sol";
contract Greeter is Ownable { string private _greeting = "Hello, World!"; address private _owner;
constructor() { _owner = msg.sender; } modifier onlyOwner() { require( msg.sender == _owner, "Ownable: caller is not the owner!" ); _; } function greet() external view returns(string memory) { return _greeting; } function setGreeting(string calldata greeting) external { _greeting = greeting; } function owner() public view returns(address) { return _owner; }
} `
The text was updated successfully, but these errors were encountered:
If you import Ownable from Zeppelin, there should be no need for you to write your own onlyOwner() modifier. Here's a working example
Ownable
onlyOwner()
pragma solidity >= 0.4.0; import "@openzeppelin/contracts/access/Ownable.sol"; contract Greeter is Ownable { string private _greeting = "Hello, World!"; function greet() external view returns(string memory) { return _greeting; } function setGreeting(string calldata greeting) external onlyOwner { _greeting = greeting; } }
Sorry, something went wrong.
No branches or pull requests
At the beginning of chapter 5 I try to compile previously added code in chapter 4 and receive the following output:
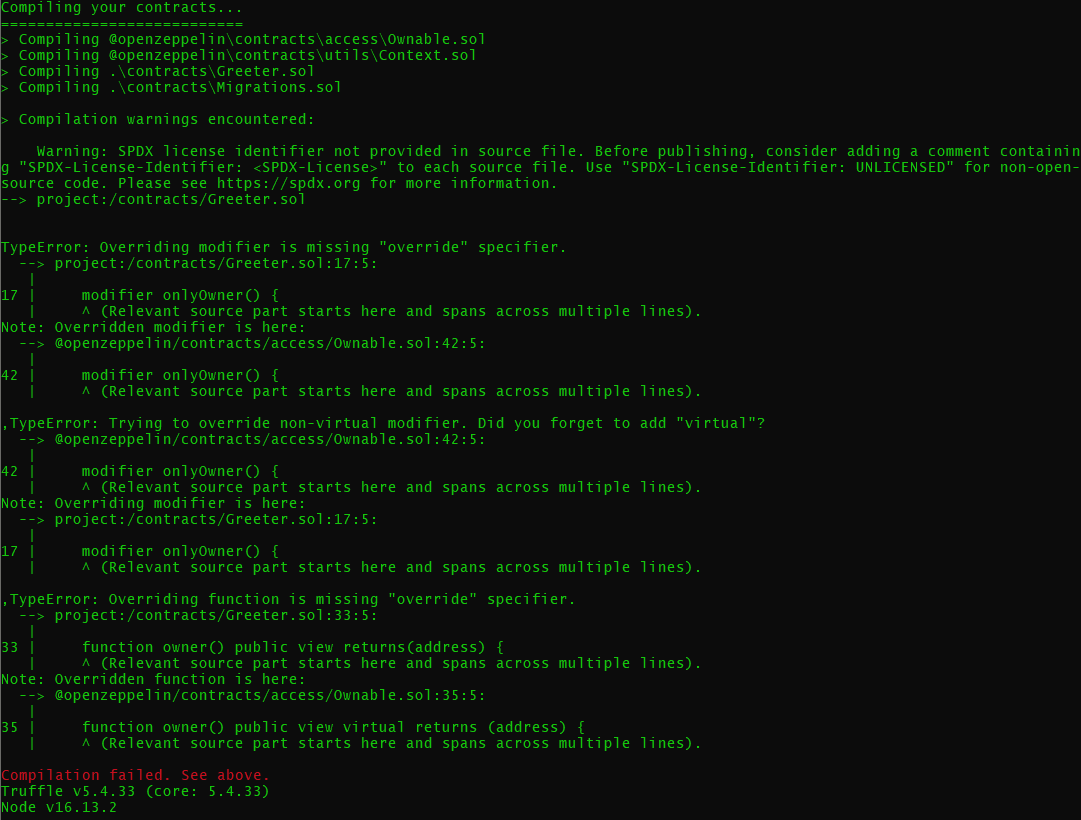
Here's my Greeter.sol code:
`pragma solidity ^0.8.0;
import "@openzeppelin/contracts/access/Ownable.sol";
contract Greeter is Ownable {
string private _greeting = "Hello, World!";
address private _owner;
} `
The text was updated successfully, but these errors were encountered: