|
| 1 | +# Node.js PostgreSQL CRUD example with Express Rest APIs |
| 2 | + |
| 3 | +Full Article with implementation: |
| 4 | +> [Node.js PostgreSQL CRUD example with Express Rest APIs](https://bezkoder.com/node-express-sequelize-postgresql/) |
| 5 | +
|
| 6 | +We will build Rest Apis that can create, retrieve, update, delete and find Tutorials by title. |
| 7 | + |
| 8 | +The following table shows overview of the Rest APIs that will be exported: |
| 9 | + |
| 10 | +- GET `api/tutorials` get all Tutorials |
| 11 | +- GET `api/tutorials/:id` get Tutorial by id |
| 12 | +- POST `api/tutorials` add new Tutorial |
| 13 | +- PUT `api/tutorials/:id` update Tutorial by id |
| 14 | +- DELETE `api/tutorials/:id` remove Tutorial by id |
| 15 | +- DELETE `api/tutorials` remove all Tutorials |
| 16 | +- GET `api/tutorials/published` find all published Tutorials |
| 17 | +- GET `api/tutorials?title=[kw]` find all Tutorials which title contains 'kw' |
| 18 | + |
| 19 | +## Demo Video |
| 20 | +This is our Node.js PostgreSQL CRUD example using Express & Sequelize application demo, test Rest Apis with Postman. |
| 21 | + |
| 22 | +[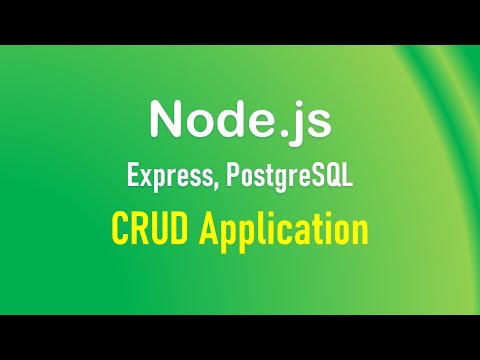](http://www.youtube.com/watch?v=x1pZHN_sjGk "Node.js PostgreSQL CRUD example Github") |
| 23 | + |
| 24 | +### Test the APIs |
| 25 | +Run our Node.js application with command: `node server.js`. |
| 26 | + |
| 27 | +Using Postman, we're gonna test all the Apis above. |
| 28 | + |
| 29 | +- Create a new Tutorial using `POST /tutorials` Api |
| 30 | + |
| 31 | +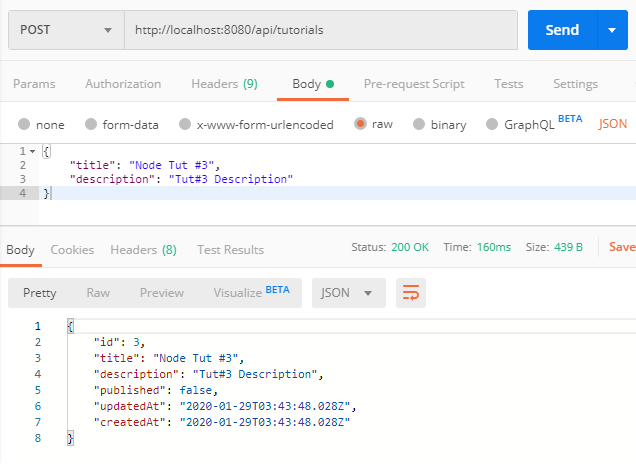 |
| 32 | + |
| 33 | +After creating some new Tutorials, you can check PostgreSQL table: |
| 34 | +```testdb=# select * from tutorials; |
| 35 | + id | title | description | published | createdAt | updatedAt |
| 36 | +----+-------------+-------------------+-----------+----------------------------+---------------------------- |
| 37 | + 1 | Node Tut #1 | Tut#1 Description | f | 2020-01-29 10:42:57.121+07 | 2020-01-29 10:42:57.121+07 |
| 38 | + 2 | Node Tut #2 | Tut#2 Description | f | 2020-01-29 10:43:05.131+07 | 2020-01-29 10:43:05.131+07 |
| 39 | + 3 | Node Tut #3 | Tut#3 Description | f | 2020-01-29 10:43:48.028+07 | 2020-01-29 10:43:48.028+07 |
| 40 | + 4 | Js Tut #4 | Tut#4 Desc | f | 2020-01-29 10:45:40.016+07 | 2020-01-29 10:45:40.016+07 |
| 41 | + 5 | Js Tut #5 | Tut#5 Desc | f | 2020-01-29 10:45:44.289+07 | 2020-01-29 10:45:44.289+07 |
| 42 | +``` |
| 43 | + |
| 44 | +- Retrieve all Tutorials using `GET /tutorials` Api |
| 45 | + |
| 46 | +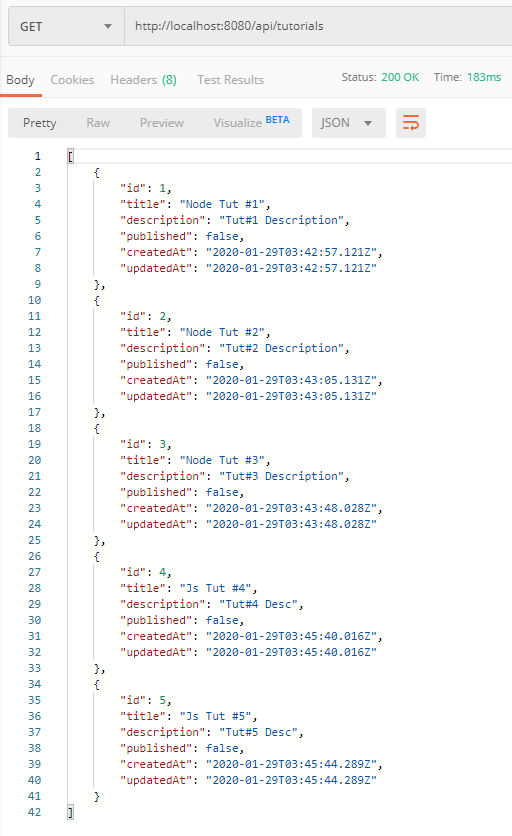 |
| 47 | + |
| 48 | +- Retrieve a single Tutorial by id using `GET /tutorials/:id` Api |
| 49 | + |
| 50 | +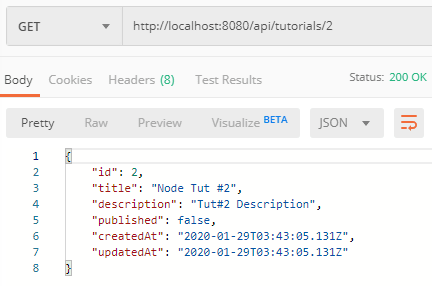 |
| 51 | + |
| 52 | +- Update a Tutorial using `PUT /tutorials/:id` Api |
| 53 | + |
| 54 | +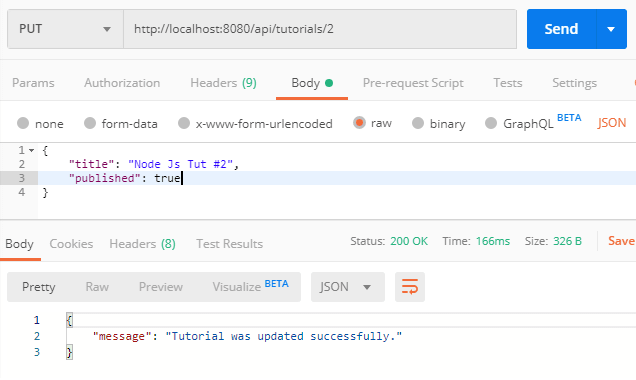 |
| 55 | + |
| 56 | +Check `tutorials` table after some rows were updated: |
| 57 | +```testdb=# select * from tutorials; |
| 58 | + id | title | description | published | createdAt | updatedAt |
| 59 | +----+----------------+-------------------+-----------+----------------------------+---------------------------- |
| 60 | + 1 | Node Tut #1 | Tut#1 Description | f | 2020-01-29 10:42:57.121+07 | 2020-01-29 10:42:57.121+07 |
| 61 | + 3 | Node Tut #3 | Tut#3 Description | f | 2020-01-29 10:43:48.028+07 | 2020-01-29 10:43:48.028+07 |
| 62 | + 2 | Node Js Tut #2 | Tut#2 Description | t | 2020-01-29 10:43:05.131+07 | 2020-01-29 10:51:55.235+07 |
| 63 | + 4 | Js Tut #4 | Tut#4 Desc | t | 2020-01-29 10:45:40.016+07 | 2020-01-29 10:54:17.468+07 |
| 64 | + 5 | Js Tut #5 | Tut#5 Desc | t | 2020-01-29 10:45:44.289+07 | 2020-01-29 10:54:20.544+07 |
| 65 | +``` |
| 66 | + |
| 67 | +- Find all Tutorials which title contains 'js': `GET /tutorials?title=js` |
| 68 | + |
| 69 | +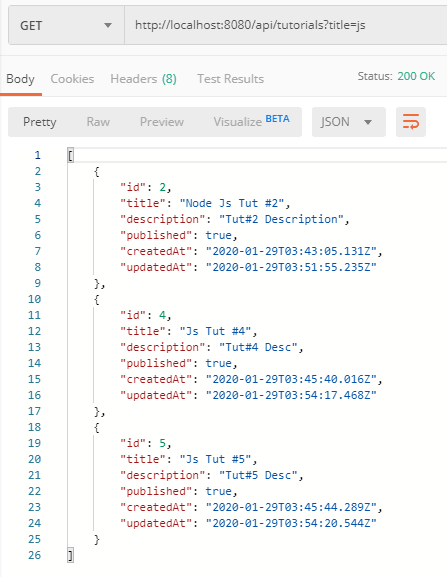 |
| 70 | + |
| 71 | +- Find all published Tutorials using `GET /tutorials/published` Api |
| 72 | + |
| 73 | +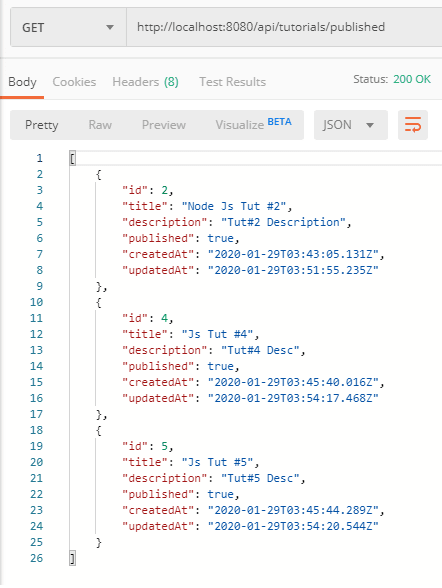 |
| 74 | + |
| 75 | +- Delete a Tutorial using `DELETE /tutorials/:id` Api |
| 76 | + |
| 77 | +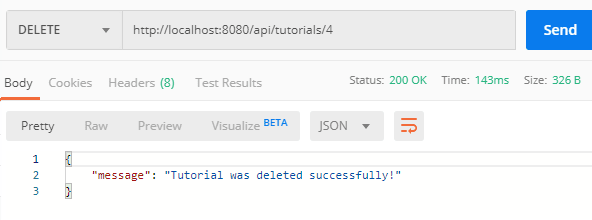 |
| 78 | + |
| 79 | +Tutorial with id=4 was removed from `tutorials` table: |
| 80 | +```testdb=# select * from tutorials; |
| 81 | + id | title | description | published | createdAt | updatedAt |
| 82 | +----+----------------+-------------------+-----------+----------------------------+---------------------------- |
| 83 | + 1 | Node Tut #1 | Tut#1 Description | f | 2020-01-29 10:42:57.121+07 | 2020-01-29 10:42:57.121+07 |
| 84 | + 3 | Node Tut #3 | Tut#3 Description | f | 2020-01-29 10:43:48.028+07 | 2020-01-29 10:43:48.028+07 |
| 85 | + 2 | Node Js Tut #2 | Tut#2 Description | t | 2020-01-29 10:43:05.131+07 | 2020-01-29 10:51:55.235+07 |
| 86 | + 5 | Js Tut #5 | Tut#5 Desc | t | 2020-01-29 10:45:44.289+07 | 2020-01-29 10:54:20.544+07 |
| 87 | +``` |
| 88 | + |
| 89 | +- Delete all Tutorials using `DELETE /tutorials` Api |
| 90 | + |
| 91 | +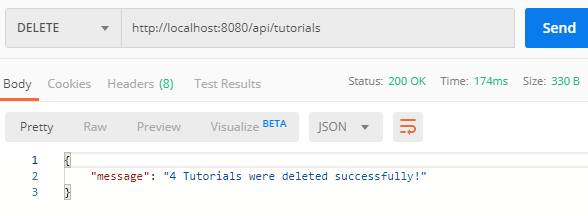 |
| 92 | + |
| 93 | +Now there are no rows in `tutorials` table: |
| 94 | +```testdb=# select * from tutorials; |
| 95 | + id | title | description | published | createdAt | updatedAt |
| 96 | +----+-------+-------------+-----------+-----------+----------- |
| 97 | +``` |
| 98 | + |
| 99 | +For more detail, please visit: |
| 100 | +> [Node.js PostgreSQL CRUD example with Express Rest APIs](https://bezkoder.com/node-express-sequelize-postgresql/) |
| 101 | +
|
| 102 | +> [Node.js Express Pagination with PostgreSQL example](https://bezkoder.com/node-js-pagination-postgresql/) |
| 103 | +
|
| 104 | +Security: |
| 105 | +> [Node.js JWT Authentication & Authorization with PostgreSQL example](https://bezkoder.com/node-js-jwt-authentication-postgresql/) |
| 106 | +
|
| 107 | +Associations: |
| 108 | +> [Sequelize Associations: One-to-Many Relationship example](https://bezkoder.com/sequelize-associate-one-to-many/) |
| 109 | +
|
| 110 | +> [Sequelize Associations: Many-to-Many Relationship example](https://bezkoder.com/sequelize-associate-many-to-many/) |
| 111 | +
|
| 112 | +Fullstack: |
| 113 | +> [Vue + Node.js + Express + PostgreSQL example](https://bezkoder.com/vue-node-express-postgresql/) |
| 114 | +
|
| 115 | +> [React + Node.js + Express + PostgreSQL example](https://bezkoder.com/react-node-express-postgresql/) |
| 116 | +
|
| 117 | +> [ANgular 8 + Node.js + Express + PostgreSQL example](https://bezkoder.com/angular-node-express-postgresql/) |
| 118 | +
|
| 119 | +> [ANgular 10 + Node.js + Express + PostgreSQL example](https://bezkoder.com/angular-10-node-express-postgresql/) |
| 120 | +
|
| 121 | +> [ANgular 11 + Node.js + Express + PostgreSQL example](https://bezkoder.com/angular-11-node-js-express-postgresql/) |
| 122 | +
|
| 123 | +## Project setup |
| 124 | +``` |
| 125 | +npm install |
| 126 | +``` |
| 127 | + |
| 128 | +### Run |
| 129 | +``` |
| 130 | +node server.js |
| 131 | +``` |
0 commit comments