diff --git a/README.md b/README.md
index ef9930a..c9edd99 100644
--- a/README.md
+++ b/README.md
@@ -2,769 +2,17 @@
The official Python client for [Prometheus](https://prometheus.io).
-## Three Step Demo
-
-**One**: Install the client:
-```
-pip install prometheus-client
-```
-
-**Two**: Paste the following into a Python interpreter:
-```python
-from prometheus_client import start_http_server, Summary
-import random
-import time
-
-# Create a metric to track time spent and requests made.
-REQUEST_TIME = Summary('request_processing_seconds', 'Time spent processing request')
-
-# Decorate function with metric.
-@REQUEST_TIME.time()
-def process_request(t):
- """A dummy function that takes some time."""
- time.sleep(t)
-
-if __name__ == '__main__':
- # Start up the server to expose the metrics.
- start_http_server(8000)
- # Generate some requests.
- while True:
- process_request(random.random())
-```
-
-**Three**: Visit [http://localhost:8000/](http://localhost:8000/) to view the metrics.
-
-From one easy to use decorator you get:
- * `request_processing_seconds_count`: Number of times this function was called.
- * `request_processing_seconds_sum`: Total amount of time spent in this function.
-
-Prometheus's `rate` function allows calculation of both requests per second,
-and latency over time from this data.
-
-In addition if you're on Linux the `process` metrics expose CPU, memory and
-other information about the process for free!
-
## Installation
```
pip install prometheus-client
```
-This package can be found on
-[PyPI](https://pypi.python.org/pypi/prometheus_client).
-
-## Instrumenting
-
-Four types of metric are offered: Counter, Gauge, Summary and Histogram.
-See the documentation on [metric types](http://prometheus.io/docs/concepts/metric_types/)
-and [instrumentation best practices](https://prometheus.io/docs/practices/instrumentation/#counter-vs-gauge-summary-vs-histogram)
-on how to use them.
-
-### Counter
-
-Counters go up, and reset when the process restarts.
-
-
-```python
-from prometheus_client import Counter
-c = Counter('my_failures', 'Description of counter')
-c.inc() # Increment by 1
-c.inc(1.6) # Increment by given value
-```
-
-If there is a suffix of `_total` on the metric name, it will be removed. When
-exposing the time series for counter, a `_total` suffix will be added. This is
-for compatibility between OpenMetrics and the Prometheus text format, as OpenMetrics
-requires the `_total` suffix.
-
-There are utilities to count exceptions raised:
-
-```python
-@c.count_exceptions()
-def f():
- pass
-
-with c.count_exceptions():
- pass
-
-# Count only one type of exception
-with c.count_exceptions(ValueError):
- pass
-```
-
-### Gauge
-
-Gauges can go up and down.
-
-```python
-from prometheus_client import Gauge
-g = Gauge('my_inprogress_requests', 'Description of gauge')
-g.inc() # Increment by 1
-g.dec(10) # Decrement by given value
-g.set(4.2) # Set to a given value
-```
-
-There are utilities for common use cases:
-
-```python
-g.set_to_current_time() # Set to current unixtime
-
-# Increment when entered, decrement when exited.
-@g.track_inprogress()
-def f():
- pass
-
-with g.track_inprogress():
- pass
-```
-
-A Gauge can also take its value from a callback:
-
-```python
-d = Gauge('data_objects', 'Number of objects')
-my_dict = {}
-d.set_function(lambda: len(my_dict))
-```
-
-### Summary
-
-Summaries track the size and number of events.
-
-```python
-from prometheus_client import Summary
-s = Summary('request_latency_seconds', 'Description of summary')
-s.observe(4.7) # Observe 4.7 (seconds in this case)
-```
-
-There are utilities for timing code:
-
-```python
-@s.time()
-def f():
- pass
-
-with s.time():
- pass
-```
-
-The Python client doesn't store or expose quantile information at this time.
-
-### Histogram
-
-Histograms track the size and number of events in buckets.
-This allows for aggregatable calculation of quantiles.
-
-```python
-from prometheus_client import Histogram
-h = Histogram('request_latency_seconds', 'Description of histogram')
-h.observe(4.7) # Observe 4.7 (seconds in this case)
-```
-
-The default buckets are intended to cover a typical web/rpc request from milliseconds to seconds.
-They can be overridden by passing `buckets` keyword argument to `Histogram`.
-
-There are utilities for timing code:
-
-```python
-@h.time()
-def f():
- pass
-
-with h.time():
- pass
-```
-
-### Info
-
-Info tracks key-value information, usually about a whole target.
-
-```python
-from prometheus_client import Info
-i = Info('my_build_version', 'Description of info')
-i.info({'version': '1.2.3', 'buildhost': 'foo@bar'})
-```
-
-### Enum
-
-Enum tracks which of a set of states something is currently in.
-
-```python
-from prometheus_client import Enum
-e = Enum('my_task_state', 'Description of enum',
- states=['starting', 'running', 'stopped'])
-e.state('running')
-```
-
-### Labels
-
-All metrics can have labels, allowing grouping of related time series.
-
-See the best practices on [naming](http://prometheus.io/docs/practices/naming/)
-and [labels](http://prometheus.io/docs/practices/instrumentation/#use-labels).
-
-Taking a counter as an example:
-
-```python
-from prometheus_client import Counter
-c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
-c.labels('get', '/').inc()
-c.labels('post', '/submit').inc()
-```
-
-Labels can also be passed as keyword-arguments:
-
-```python
-from prometheus_client import Counter
-c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
-c.labels(method='get', endpoint='/').inc()
-c.labels(method='post', endpoint='/submit').inc()
-```
-
-Metrics with labels are not initialized when declared, because the client can't
-know what values the label can have. It is recommended to initialize the label
-values by calling the `.labels()` method alone:
-
-```python
-from prometheus_client import Counter
-c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
-c.labels('get', '/')
-c.labels('post', '/submit')
-```
-
-### Exemplars
-
-Exemplars can be added to counter and histogram metrics. Exemplars can be
-specified by passing a dict of label value pairs to be exposed as the exemplar.
-For example with a counter:
-
-```python
-from prometheus_client import Counter
-c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
-c.labels('get', '/').inc(exemplar={'trace_id': 'abc123'})
-c.labels('post', '/submit').inc(1.0, {'trace_id': 'def456'})
-```
-
-And with a histogram:
-
-```python
-from prometheus_client import Histogram
-h = Histogram('request_latency_seconds', 'Description of histogram')
-h.observe(4.7, {'trace_id': 'abc123'})
-```
-
-Exemplars are only rendered in the OpenMetrics exposition format. If using the
-HTTP server or apps in this library, content negotiation can be used to specify
-OpenMetrics (which is done by default in Prometheus). Otherwise it will be
-necessary to use `generate_latest` from
-`prometheus_client.openmetrics.exposition` to view exemplars.
-
-To view exemplars in Prometheus it is also necessary to enable the the
-exemplar-storage feature flag:
-```
---enable-feature=exemplar-storage
-```
-Additional information is available in [the Prometheus
-documentation](https://prometheus.io/docs/prometheus/latest/feature_flags/#exemplars-storage).
-
-### Disabling `_created` metrics
-
-By default counters, histograms, and summaries export an additional series
-suffixed with `_created` and a value of the unix timestamp for when the metric
-was created. If this information is not helpful, it can be disabled by setting
-the environment variable `PROMETHEUS_DISABLE_CREATED_SERIES=True`.
-
-### Process Collector
-
-The Python client automatically exports metrics about process CPU usage, RAM,
-file descriptors and start time. These all have the prefix `process`, and
-are only currently available on Linux.
-
-The namespace and pid constructor arguments allows for exporting metrics about
-other processes, for example:
-```
-ProcessCollector(namespace='mydaemon', pid=lambda: open('/var/run/daemon.pid').read())
-```
-
-### Platform Collector
-
-The client also automatically exports some metadata about Python. If using Jython,
-metadata about the JVM in use is also included. This information is available as
-labels on the `python_info` metric. The value of the metric is 1, since it is the
-labels that carry information.
-
-### Disabling Default Collector metrics
-
-By default the collected `process`, `gc`, and `platform` collector metrics are exported.
-If this information is not helpful, it can be disabled using the following:
-```python
-import prometheus_client
-
-prometheus_client.REGISTRY.unregister(prometheus_client.GC_COLLECTOR)
-prometheus_client.REGISTRY.unregister(prometheus_client.PLATFORM_COLLECTOR)
-prometheus_client.REGISTRY.unregister(prometheus_client.PROCESS_COLLECTOR)
-```
-
-## Exporting
-
-There are several options for exporting metrics.
-
-### HTTP
-
-Metrics are usually exposed over HTTP, to be read by the Prometheus server.
-
-The easiest way to do this is via `start_http_server`, which will start a HTTP
-server in a daemon thread on the given port:
-
-```python
-from prometheus_client import start_http_server
-
-start_http_server(8000)
-```
-
-Visit [http://localhost:8000/](http://localhost:8000/) to view the metrics.
-
-To add Prometheus exposition to an existing HTTP server, see the `MetricsHandler` class
-which provides a `BaseHTTPRequestHandler`. It also serves as a simple example of how
-to write a custom endpoint.
-
-#### Twisted
-
-To use prometheus with [twisted](https://twistedmatrix.com/), there is `MetricsResource` which exposes metrics as a twisted resource.
-
-```python
-from prometheus_client.twisted import MetricsResource
-from twisted.web.server import Site
-from twisted.web.resource import Resource
-from twisted.internet import reactor
-
-root = Resource()
-root.putChild(b'metrics', MetricsResource())
-
-factory = Site(root)
-reactor.listenTCP(8000, factory)
-reactor.run()
-```
-
-#### WSGI
-
-To use Prometheus with [WSGI](http://wsgi.readthedocs.org/en/latest/), there is
-`make_wsgi_app` which creates a WSGI application.
-
-```python
-from prometheus_client import make_wsgi_app
-from wsgiref.simple_server import make_server
-
-app = make_wsgi_app()
-httpd = make_server('', 8000, app)
-httpd.serve_forever()
-```
-
-Such an application can be useful when integrating Prometheus metrics with WSGI
-apps.
-
-The method `start_wsgi_server` can be used to serve the metrics through the
-WSGI reference implementation in a new thread.
-
-```python
-from prometheus_client import start_wsgi_server
-
-start_wsgi_server(8000)
-```
-
-By default, the WSGI application will respect `Accept-Encoding:gzip` headers used by Prometheus
-and compress the response if such a header is present. This behaviour can be disabled by passing
-`disable_compression=True` when creating the app, like this:
+This package can be found on [PyPI](https://pypi.python.org/pypi/prometheus_client).
-```python
-app = make_wsgi_app(disable_compression=True)
-```
-
-#### ASGI
-
-To use Prometheus with [ASGI](http://asgi.readthedocs.org/en/latest/), there is
-`make_asgi_app` which creates an ASGI application.
-
-```python
-from prometheus_client import make_asgi_app
-
-app = make_asgi_app()
-```
-Such an application can be useful when integrating Prometheus metrics with ASGI
-apps.
-
-By default, the WSGI application will respect `Accept-Encoding:gzip` headers used by Prometheus
-and compress the response if such a header is present. This behaviour can be disabled by passing
-`disable_compression=True` when creating the app, like this:
-
-```python
-app = make_asgi_app(disable_compression=True)
-```
-
-#### Flask
-
-To use Prometheus with [Flask](http://flask.pocoo.org/) we need to serve metrics through a Prometheus WSGI application. This can be achieved using [Flask's application dispatching](http://flask.pocoo.org/docs/latest/patterns/appdispatch/). Below is a working example.
-
-Save the snippet below in a `myapp.py` file
-
-```python
-from flask import Flask
-from werkzeug.middleware.dispatcher import DispatcherMiddleware
-from prometheus_client import make_wsgi_app
-
-# Create my app
-app = Flask(__name__)
-
-# Add prometheus wsgi middleware to route /metrics requests
-app.wsgi_app = DispatcherMiddleware(app.wsgi_app, {
- '/metrics': make_wsgi_app()
-})
-```
-
-Run the example web application like this
-
-```bash
-# Install uwsgi if you do not have it
-pip install uwsgi
-uwsgi --http 127.0.0.1:8000 --wsgi-file myapp.py --callable app
-```
-
-Visit http://localhost:8000/metrics to see the metrics
-
-#### FastAPI + Gunicorn
-
-To use Prometheus with [FastAPI](https://fastapi.tiangolo.com/) and [Gunicorn](https://gunicorn.org/) we need to serve metrics through a Prometheus ASGI application.
-
-Save the snippet below in a `myapp.py` file
-
-```python
-from fastapi import FastAPI
-from prometheus_client import make_asgi_app
-
-# Create app
-app = FastAPI(debug=False)
-
-# Add prometheus asgi middleware to route /metrics requests
-metrics_app = make_asgi_app()
-app.mount("/metrics", metrics_app)
-```
-
-For Multiprocessing support, use this modified code snippet. Full multiprocessing instructions are provided [here](https://github.com/prometheus/client_python#multiprocess-mode-eg-gunicorn).
-
-```python
-from fastapi import FastAPI
-from prometheus_client import make_asgi_app
-
-app = FastAPI(debug=False)
-
-# Using multiprocess collector for registry
-def make_metrics_app():
- registry = CollectorRegistry()
- multiprocess.MultiProcessCollector(registry)
- return make_asgi_app(registry=registry)
-
-
-metrics_app = make_metrics_app()
-app.mount("/metrics", metrics_app)
-```
-
-Run the example web application like this
-
-```bash
-# Install gunicorn if you do not have it
-pip install gunicorn
-# If using multiple workers, add `--workers n` parameter to the line below
-gunicorn -b 127.0.0.1:8000 myapp:app -k uvicorn.workers.UvicornWorker
-```
-
-Visit http://localhost:8000/metrics to see the metrics
-
-
-### Node exporter textfile collector
-
-The [textfile collector](https://github.com/prometheus/node_exporter#textfile-collector)
-allows machine-level statistics to be exported out via the Node exporter.
-
-This is useful for monitoring cronjobs, or for writing cronjobs to expose metrics
-about a machine system that the Node exporter does not support or would not make sense
-to perform at every scrape (for example, anything involving subprocesses).
-
-```python
-from prometheus_client import CollectorRegistry, Gauge, write_to_textfile
-
-registry = CollectorRegistry()
-g = Gauge('raid_status', '1 if raid array is okay', registry=registry)
-g.set(1)
-write_to_textfile('/configured/textfile/path/raid.prom', registry)
-```
-
-A separate registry is used, as the default registry may contain other metrics
-such as those from the Process Collector.
-
-## Exporting to a Pushgateway
-
-The [Pushgateway](https://github.com/prometheus/pushgateway)
-allows ephemeral and batch jobs to expose their metrics to Prometheus.
-
-```python
-from prometheus_client import CollectorRegistry, Gauge, push_to_gateway
-
-registry = CollectorRegistry()
-g = Gauge('job_last_success_unixtime', 'Last time a batch job successfully finished', registry=registry)
-g.set_to_current_time()
-push_to_gateway('localhost:9091', job='batchA', registry=registry)
-```
-
-A separate registry is used, as the default registry may contain other metrics
-such as those from the Process Collector.
-
-Pushgateway functions take a grouping key. `push_to_gateway` replaces metrics
-with the same grouping key, `pushadd_to_gateway` only replaces metrics with the
-same name and grouping key and `delete_from_gateway` deletes metrics with the
-given job and grouping key. See the
-[Pushgateway documentation](https://github.com/prometheus/pushgateway/blob/master/README.md)
-for more information.
-
-`instance_ip_grouping_key` returns a grouping key with the instance label set
-to the host's IP address.
-
-### Handlers for authentication
-
-If the push gateway you are connecting to is protected with HTTP Basic Auth,
-you can use a special handler to set the Authorization header.
-
-```python
-from prometheus_client import CollectorRegistry, Gauge, push_to_gateway
-from prometheus_client.exposition import basic_auth_handler
-
-def my_auth_handler(url, method, timeout, headers, data):
- username = 'foobar'
- password = 'secret123'
- return basic_auth_handler(url, method, timeout, headers, data, username, password)
-registry = CollectorRegistry()
-g = Gauge('job_last_success_unixtime', 'Last time a batch job successfully finished', registry=registry)
-g.set_to_current_time()
-push_to_gateway('localhost:9091', job='batchA', registry=registry, handler=my_auth_handler)
-```
-
-TLS Auth is also supported when using the push gateway with a special handler.
-
-```python
-from prometheus_client import CollectorRegistry, Gauge, push_to_gateway
-from prometheus_client.exposition import tls_auth_handler
-
-
-def my_auth_handler(url, method, timeout, headers, data):
- certfile = 'client-crt.pem'
- keyfile = 'client-key.pem'
- return tls_auth_handler(url, method, timeout, headers, data, certfile, keyfile)
-
-registry = CollectorRegistry()
-g = Gauge('job_last_success_unixtime', 'Last time a batch job successfully finished', registry=registry)
-g.set_to_current_time()
-push_to_gateway('localhost:9091', job='batchA', registry=registry, handler=my_auth_handler)
-```
-
-## Bridges
-
-It is also possible to expose metrics to systems other than Prometheus.
-This allows you to take advantage of Prometheus instrumentation even
-if you are not quite ready to fully transition to Prometheus yet.
-
-### Graphite
-
-Metrics are pushed over TCP in the Graphite plaintext format.
-
-```python
-from prometheus_client.bridge.graphite import GraphiteBridge
-
-gb = GraphiteBridge(('graphite.your.org', 2003))
-# Push once.
-gb.push()
-# Push every 10 seconds in a daemon thread.
-gb.start(10.0)
-```
-
-Graphite [tags](https://grafana.com/blog/2018/01/11/graphite-1.1-teaching-an-old-dog-new-tricks/) are also supported.
-
-```python
-from prometheus_client.bridge.graphite import GraphiteBridge
-
-gb = GraphiteBridge(('graphite.your.org', 2003), tags=True)
-c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
-c.labels('get', '/').inc()
-gb.push()
-```
-
-## Custom Collectors
-
-Sometimes it is not possible to directly instrument code, as it is not
-in your control. This requires you to proxy metrics from other systems.
-
-To do so you need to create a custom collector, for example:
-
-```python
-from prometheus_client.core import GaugeMetricFamily, CounterMetricFamily, REGISTRY
-from prometheus_client.registry import Collector
-
-class CustomCollector(Collector):
- def collect(self):
- yield GaugeMetricFamily('my_gauge', 'Help text', value=7)
- c = CounterMetricFamily('my_counter_total', 'Help text', labels=['foo'])
- c.add_metric(['bar'], 1.7)
- c.add_metric(['baz'], 3.8)
- yield c
-
-REGISTRY.register(CustomCollector())
-```
-
-`SummaryMetricFamily`, `HistogramMetricFamily` and `InfoMetricFamily` work similarly.
-
-A collector may implement a `describe` method which returns metrics in the same
-format as `collect` (though you don't have to include the samples). This is
-used to predetermine the names of time series a `CollectorRegistry` exposes and
-thus to detect collisions and duplicate registrations.
-
-Usually custom collectors do not have to implement `describe`. If `describe` is
-not implemented and the CollectorRegistry was created with `auto_describe=True`
-(which is the case for the default registry) then `collect` will be called at
-registration time instead of `describe`. If this could cause problems, either
-implement a proper `describe`, or if that's not practical have `describe`
-return an empty list.
-
-
-## Multiprocess Mode (E.g. Gunicorn)
-
-Prometheus client libraries presume a threaded model, where metrics are shared
-across workers. This doesn't work so well for languages such as Python where
-it's common to have processes rather than threads to handle large workloads.
-
-To handle this the client library can be put in multiprocess mode.
-This comes with a number of limitations:
-
-- Registries can not be used as normal, all instantiated metrics are exported
- - Registering metrics to a registry later used by a `MultiProcessCollector`
- may cause duplicate metrics to be exported
-- Custom collectors do not work (e.g. cpu and memory metrics)
-- Info and Enum metrics do not work
-- The pushgateway cannot be used
-- Gauges cannot use the `pid` label
-- Exemplars are not supported
-
-There's several steps to getting this working:
-
-**1. Deployment**:
-
-The `PROMETHEUS_MULTIPROC_DIR` environment variable must be set to a directory
-that the client library can use for metrics. This directory must be wiped
-between process/Gunicorn runs (before startup is recommended).
-
-This environment variable should be set from a start-up shell script,
-and not directly from Python (otherwise it may not propagate to child processes).
-
-**2. Metrics collector**:
-
-The application must initialize a new `CollectorRegistry`, and store the
-multi-process collector inside. It is a best practice to create this registry
-inside the context of a request to avoid metrics registering themselves to a
-collector used by a `MultiProcessCollector`. If a registry with metrics
-registered is used by a `MultiProcessCollector` duplicate metrics may be
-exported, one for multiprocess, and one for the process serving the request.
-
-```python
-from prometheus_client import multiprocess
-from prometheus_client import generate_latest, CollectorRegistry, CONTENT_TYPE_LATEST, Counter
-
-MY_COUNTER = Counter('my_counter', 'Description of my counter')
-
-# Expose metrics.
-def app(environ, start_response):
- registry = CollectorRegistry()
- multiprocess.MultiProcessCollector(registry)
- data = generate_latest(registry)
- status = '200 OK'
- response_headers = [
- ('Content-type', CONTENT_TYPE_LATEST),
- ('Content-Length', str(len(data)))
- ]
- start_response(status, response_headers)
- return iter([data])
-```
-
-**3. Gunicorn configuration**:
-
-The `gunicorn` configuration file needs to include the following function:
-
-```python
-from prometheus_client import multiprocess
-
-def child_exit(server, worker):
- multiprocess.mark_process_dead(worker.pid)
-```
-
-**4. Metrics tuning (Gauge)**:
-
-When `Gauge`s are used in multiprocess applications,
-you must decide how to handle the metrics reported by each process.
-Gauges have several modes they can run in, which can be selected with the `multiprocess_mode` parameter.
-
-- 'all': Default. Return a timeseries per process (alive or dead), labelled by the process's `pid` (the label is added internally).
-- 'min': Return a single timeseries that is the minimum of the values of all processes (alive or dead).
-- 'max': Return a single timeseries that is the maximum of the values of all processes (alive or dead).
-- 'sum': Return a single timeseries that is the sum of the values of all processes (alive or dead).
-- 'mostrecent': Return a single timeseries that is the most recent value among all processes (alive or dead).
-
-Prepend 'live' to the beginning of the mode to return the same result but only considering living processes
-(e.g., 'liveall, 'livesum', 'livemax', 'livemin', 'livemostrecent').
-
-```python
-from prometheus_client import Gauge
-
-# Example gauge
-IN_PROGRESS = Gauge("inprogress_requests", "help", multiprocess_mode='livesum')
-```
-
-
-## Parser
-
-The Python client supports parsing the Prometheus text format.
-This is intended for advanced use cases where you have servers
-exposing Prometheus metrics and need to get them into some other
-system.
-
-```python
-from prometheus_client.parser import text_string_to_metric_families
-for family in text_string_to_metric_families(u"my_gauge 1.0\n"):
- for sample in family.samples:
- print("Name: {0} Labels: {1} Value: {2}".format(*sample))
-```
-
-## Restricted registry
-
-Registries support restriction to only return specific metrics.
-If you’re using the built-in HTTP server, you can use the GET parameter "name[]", since it’s an array it can be used multiple times.
-If you’re directly using `generate_latest`, you can use the function `restricted_registry()`.
-
-```python
-curl --get --data-urlencode "name[]=python_gc_objects_collected_total" --data-urlencode "name[]=python_info" http://127.0.0.1:9200/metrics
-```
-
-```python
-from prometheus_client import generate_latest
-
-generate_latest(REGISTRY.restricted_registry(['python_gc_objects_collected_total', 'python_info']))
-```
-
-```python
-# HELP python_info Python platform information
-# TYPE python_info gauge
-python_info{implementation="CPython",major="3",minor="9",patchlevel="3",version="3.9.3"} 1.0
-# HELP python_gc_objects_collected_total Objects collected during gc
-# TYPE python_gc_objects_collected_total counter
-python_gc_objects_collected_total{generation="0"} 73129.0
-python_gc_objects_collected_total{generation="1"} 8594.0
-python_gc_objects_collected_total{generation="2"} 296.0
-```
+## Documentation
+Documentation is available on https://prometheus.github.io/client_python
## Links
diff --git a/docs/.gitignore b/docs/.gitignore
new file mode 100644
index 0000000..2a8645f
--- /dev/null
+++ b/docs/.gitignore
@@ -0,0 +1 @@
+.hugo_build.lock
diff --git a/docs/README.md b/docs/README.md
new file mode 100644
index 0000000..e9248d5
--- /dev/null
+++ b/docs/README.md
@@ -0,0 +1,37 @@
+Docs
+----
+
+This directory contains [hugo](https://gohugo.io) documentation to be published in Github pages.
+
+Run Locally
+-----------
+
+```
+hugo server -D
+```
+
+This will serve the docs on [http://localhost:1313](http://localhost:1313).
+
+Deploy to Github Pages
+----------------------
+
+Changes to the `main` branch will be deployed automatically with Github actions.
+
+Update Geekdocs
+---------------
+
+The docs use the [Geekdocs](https://geekdocs.de/) theme. The theme is checked in to Github in the `./docs/themes/hugo-geekdoc/` folder. To update [Geekdocs](https://geekdocs.de/), remove the current folder and create a new one with the latest [release](https://github.com/thegeeklab/hugo-geekdoc/releases). There are no local modifications in `./docs/themes/hugo-geekdoc/`.
+
+Notes
+-----
+
+Here's how the initial `docs/` folder was set up:
+
+```
+hugo new site docs
+cd docs/
+mkdir -p themes/hugo-geekdoc/
+curl -L https://github.com/thegeeklab/hugo-geekdoc/releases/download/v0.41.1/hugo-geekdoc.tar.gz | tar -xz -C themes/hugo-geekdoc/ --strip-components=1
+```
+
+Create the initial `hugo.toml` file as described in [https://geekdocs.de/usage/getting-started/](https://geekdocs.de/usage/getting-started/).
diff --git a/docs/archetypes/default.md b/docs/archetypes/default.md
new file mode 100644
index 0000000..c6f3fce
--- /dev/null
+++ b/docs/archetypes/default.md
@@ -0,0 +1,5 @@
++++
+title = '{{ replace .File.ContentBaseName "-" " " | title }}'
+date = {{ .Date }}
+draft = true
++++
diff --git a/docs/content/_index.md b/docs/content/_index.md
new file mode 100644
index 0000000..e8c571d
--- /dev/null
+++ b/docs/content/_index.md
@@ -0,0 +1,5 @@
+---
+title: "client_python"
+---
+
+This is the documentation for the [Prometheus Python client library](https://github.com/prometheus/client_python).
diff --git a/docs/content/bridges/_index.md b/docs/content/bridges/_index.md
new file mode 100644
index 0000000..8ebdb0c
--- /dev/null
+++ b/docs/content/bridges/_index.md
@@ -0,0 +1,8 @@
+---
+title: Bridges
+weight: 5
+---
+
+It is also possible to expose metrics to systems other than Prometheus.
+This allows you to take advantage of Prometheus instrumentation even
+if you are not quite ready to fully transition to Prometheus yet.
\ No newline at end of file
diff --git a/docs/content/bridges/graphite.md b/docs/content/bridges/graphite.md
new file mode 100644
index 0000000..fe29905
--- /dev/null
+++ b/docs/content/bridges/graphite.md
@@ -0,0 +1,27 @@
+---
+title: Graphite
+weight: 1
+---
+
+Metrics are pushed over TCP in the Graphite plaintext format.
+
+```python
+from prometheus_client.bridge.graphite import GraphiteBridge
+
+gb = GraphiteBridge(('graphite.your.org', 2003))
+# Push once.
+gb.push()
+# Push every 10 seconds in a daemon thread.
+gb.start(10.0)
+```
+
+Graphite [tags](https://grafana.com/blog/2018/01/11/graphite-1.1-teaching-an-old-dog-new-tricks/) are also supported.
+
+```python
+from prometheus_client.bridge.graphite import GraphiteBridge
+
+gb = GraphiteBridge(('graphite.your.org', 2003), tags=True)
+c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
+c.labels('get', '/').inc()
+gb.push()
+```
\ No newline at end of file
diff --git a/docs/content/collector/_index.md b/docs/content/collector/_index.md
new file mode 100644
index 0000000..957c8ba
--- /dev/null
+++ b/docs/content/collector/_index.md
@@ -0,0 +1,36 @@
+---
+title: Collector
+weight: 3
+---
+
+# Process Collector
+
+The Python client automatically exports metrics about process CPU usage, RAM,
+file descriptors and start time. These all have the prefix `process`, and
+are only currently available on Linux.
+
+The namespace and pid constructor arguments allows for exporting metrics about
+other processes, for example:
+```
+ProcessCollector(namespace='mydaemon', pid=lambda: open('/var/run/daemon.pid').read())
+```
+
+# Platform Collector
+
+The client also automatically exports some metadata about Python. If using Jython,
+metadata about the JVM in use is also included. This information is available as
+labels on the `python_info` metric. The value of the metric is 1, since it is the
+labels that carry information.
+
+# Disabling Default Collector metrics
+
+By default the collected `process`, `gc`, and `platform` collector metrics are exported.
+If this information is not helpful, it can be disabled using the following:
+
+```python
+import prometheus_client
+
+prometheus_client.REGISTRY.unregister(prometheus_client.GC_COLLECTOR)
+prometheus_client.REGISTRY.unregister(prometheus_client.PLATFORM_COLLECTOR)
+prometheus_client.REGISTRY.unregister(prometheus_client.PROCESS_COLLECTOR)
+```
\ No newline at end of file
diff --git a/docs/content/collector/custom.md b/docs/content/collector/custom.md
new file mode 100644
index 0000000..bc6a021
--- /dev/null
+++ b/docs/content/collector/custom.md
@@ -0,0 +1,38 @@
+---
+title: Custom Collectors
+weight: 1
+---
+
+Sometimes it is not possible to directly instrument code, as it is not
+in your control. This requires you to proxy metrics from other systems.
+
+To do so you need to create a custom collector, for example:
+
+```python
+from prometheus_client.core import GaugeMetricFamily, CounterMetricFamily, REGISTRY
+from prometheus_client.registry import Collector
+
+class CustomCollector(Collector):
+ def collect(self):
+ yield GaugeMetricFamily('my_gauge', 'Help text', value=7)
+ c = CounterMetricFamily('my_counter_total', 'Help text', labels=['foo'])
+ c.add_metric(['bar'], 1.7)
+ c.add_metric(['baz'], 3.8)
+ yield c
+
+REGISTRY.register(CustomCollector())
+```
+
+`SummaryMetricFamily`, `HistogramMetricFamily` and `InfoMetricFamily` work similarly.
+
+A collector may implement a `describe` method which returns metrics in the same
+format as `collect` (though you don't have to include the samples). This is
+used to predetermine the names of time series a `CollectorRegistry` exposes and
+thus to detect collisions and duplicate registrations.
+
+Usually custom collectors do not have to implement `describe`. If `describe` is
+not implemented and the CollectorRegistry was created with `auto_describe=True`
+(which is the case for the default registry) then `collect` will be called at
+registration time instead of `describe`. If this could cause problems, either
+implement a proper `describe`, or if that's not practical have `describe`
+return an empty list.
\ No newline at end of file
diff --git a/docs/content/exporting/_index.md b/docs/content/exporting/_index.md
new file mode 100644
index 0000000..1e532f8
--- /dev/null
+++ b/docs/content/exporting/_index.md
@@ -0,0 +1,6 @@
+---
+title: Exporting
+weight: 4
+---
+
+There are several options for exporting metrics.
diff --git a/docs/content/exporting/http/_index.md b/docs/content/exporting/http/_index.md
new file mode 100644
index 0000000..b074a3b
--- /dev/null
+++ b/docs/content/exporting/http/_index.md
@@ -0,0 +1,46 @@
+---
+title: HTTP/HTTPS
+weight: 1
+---
+
+# HTTP
+
+Metrics are usually exposed over HTTP, to be read by the Prometheus server.
+
+The easiest way to do this is via `start_http_server`, which will start a HTTP
+server in a daemon thread on the given port:
+
+```python
+from prometheus_client import start_http_server
+
+start_http_server(8000)
+```
+
+Visit [http://localhost:8000/](http://localhost:8000/) to view the metrics.
+
+To add Prometheus exposition to an existing HTTP server, see the `MetricsHandler` class
+which provides a `BaseHTTPRequestHandler`. It also serves as a simple example of how
+to write a custom endpoint.
+
+# HTTPS
+
+By default, the prometheus client will accept only HTTP requests from Prometheus.
+To enable HTTPS, `certfile` and `keyfile` need to be provided. The certificate is
+presented to Prometheus as a server certificate during the TLS handshake, and
+the private key in the key file must belong to the public key in the certificate.
+
+When HTTPS is enabled, you can enable mutual TLS (mTLS) by setting `client_auth_required=True`
+(i.e. Prometheus is required to present a client certificate during TLS handshake) and the
+client certificate including its hostname is validated against the CA certificate chain.
+
+`client_cafile` can be used to specify a certificate file containing a CA certificate
+chain that is used to validate the client certificate. `client_capath` can be used to
+specify a certificate directory containing a CA certificate chain that is used to
+validate the client certificate. If neither of them is provided, a default CA certificate
+chain is used (see Python [ssl.SSLContext.load_default_certs()](https://docs.python.org/3/library/ssl.html#ssl.SSLContext.load_default_certs))
+
+```python
+from prometheus_client import start_http_server
+
+start_http_server(8000, certfile="server.crt", keyfile="server.key")
+```
\ No newline at end of file
diff --git a/docs/content/exporting/http/asgi.md b/docs/content/exporting/http/asgi.md
new file mode 100644
index 0000000..4ff115e
--- /dev/null
+++ b/docs/content/exporting/http/asgi.md
@@ -0,0 +1,23 @@
+---
+title: ASGI
+weight: 3
+---
+
+To use Prometheus with [ASGI](http://asgi.readthedocs.org/en/latest/), there is
+`make_asgi_app` which creates an ASGI application.
+
+```python
+from prometheus_client import make_asgi_app
+
+app = make_asgi_app()
+```
+Such an application can be useful when integrating Prometheus metrics with ASGI
+apps.
+
+By default, the WSGI application will respect `Accept-Encoding:gzip` headers used by Prometheus
+and compress the response if such a header is present. This behaviour can be disabled by passing
+`disable_compression=True` when creating the app, like this:
+
+```python
+app = make_asgi_app(disable_compression=True)
+```
\ No newline at end of file
diff --git a/docs/content/exporting/http/fastapi-gunicorn.md b/docs/content/exporting/http/fastapi-gunicorn.md
new file mode 100644
index 0000000..9ce1238
--- /dev/null
+++ b/docs/content/exporting/http/fastapi-gunicorn.md
@@ -0,0 +1,50 @@
+---
+title: FastAPI + Gunicorn
+weight: 5
+---
+
+To use Prometheus with [FastAPI](https://fastapi.tiangolo.com/) and [Gunicorn](https://gunicorn.org/) we need to serve metrics through a Prometheus ASGI application.
+
+Save the snippet below in a `myapp.py` file
+
+```python
+from fastapi import FastAPI
+from prometheus_client import make_asgi_app
+
+# Create app
+app = FastAPI(debug=False)
+
+# Add prometheus asgi middleware to route /metrics requests
+metrics_app = make_asgi_app()
+app.mount("/metrics", metrics_app)
+```
+
+For Multiprocessing support, use this modified code snippet. Full multiprocessing instructions are provided [here](https://github.com/prometheus/client_python#multiprocess-mode-eg-gunicorn).
+
+```python
+from fastapi import FastAPI
+from prometheus_client import make_asgi_app
+
+app = FastAPI(debug=False)
+
+# Using multiprocess collector for registry
+def make_metrics_app():
+ registry = CollectorRegistry()
+ multiprocess.MultiProcessCollector(registry)
+ return make_asgi_app(registry=registry)
+
+
+metrics_app = make_metrics_app()
+app.mount("/metrics", metrics_app)
+```
+
+Run the example web application like this
+
+```bash
+# Install gunicorn if you do not have it
+pip install gunicorn
+# If using multiple workers, add `--workers n` parameter to the line below
+gunicorn -b 127.0.0.1:8000 myapp:app -k uvicorn.workers.UvicornWorker
+```
+
+Visit http://localhost:8000/metrics to see the metrics
\ No newline at end of file
diff --git a/docs/content/exporting/http/flask.md b/docs/content/exporting/http/flask.md
new file mode 100644
index 0000000..a666a18
--- /dev/null
+++ b/docs/content/exporting/http/flask.md
@@ -0,0 +1,32 @@
+---
+title: Flask
+weight: 4
+---
+
+To use Prometheus with [Flask](http://flask.pocoo.org/) we need to serve metrics through a Prometheus WSGI application. This can be achieved using [Flask's application dispatching](http://flask.pocoo.org/docs/latest/patterns/appdispatch/). Below is a working example.
+
+Save the snippet below in a `myapp.py` file
+
+```python
+from flask import Flask
+from werkzeug.middleware.dispatcher import DispatcherMiddleware
+from prometheus_client import make_wsgi_app
+
+# Create my app
+app = Flask(__name__)
+
+# Add prometheus wsgi middleware to route /metrics requests
+app.wsgi_app = DispatcherMiddleware(app.wsgi_app, {
+ '/metrics': make_wsgi_app()
+})
+```
+
+Run the example web application like this
+
+```bash
+# Install uwsgi if you do not have it
+pip install uwsgi
+uwsgi --http 127.0.0.1:8000 --wsgi-file myapp.py --callable app
+```
+
+Visit http://localhost:8000/metrics to see the metrics
\ No newline at end of file
diff --git a/docs/content/exporting/http/twisted.md b/docs/content/exporting/http/twisted.md
new file mode 100644
index 0000000..a45fe91
--- /dev/null
+++ b/docs/content/exporting/http/twisted.md
@@ -0,0 +1,20 @@
+---
+title: Twisted
+weight: 1
+---
+
+To use prometheus with [twisted](https://twistedmatrix.com/), there is `MetricsResource` which exposes metrics as a twisted resource.
+
+```python
+from prometheus_client.twisted import MetricsResource
+from twisted.web.server import Site
+from twisted.web.resource import Resource
+from twisted.internet import reactor
+
+root = Resource()
+root.putChild(b'metrics', MetricsResource())
+
+factory = Site(root)
+reactor.listenTCP(8000, factory)
+reactor.run()
+```
\ No newline at end of file
diff --git a/docs/content/exporting/http/wsgi.md b/docs/content/exporting/http/wsgi.md
new file mode 100644
index 0000000..3c3bc3e
--- /dev/null
+++ b/docs/content/exporting/http/wsgi.md
@@ -0,0 +1,36 @@
+---
+title: WSGI
+weight: 2
+---
+
+To use Prometheus with [WSGI](http://wsgi.readthedocs.org/en/latest/), there is
+`make_wsgi_app` which creates a WSGI application.
+
+```python
+from prometheus_client import make_wsgi_app
+from wsgiref.simple_server import make_server
+
+app = make_wsgi_app()
+httpd = make_server('', 8000, app)
+httpd.serve_forever()
+```
+
+Such an application can be useful when integrating Prometheus metrics with WSGI
+apps.
+
+The method `start_wsgi_server` can be used to serve the metrics through the
+WSGI reference implementation in a new thread.
+
+```python
+from prometheus_client import start_wsgi_server
+
+start_wsgi_server(8000)
+```
+
+By default, the WSGI application will respect `Accept-Encoding:gzip` headers used by Prometheus
+and compress the response if such a header is present. This behaviour can be disabled by passing
+`disable_compression=True` when creating the app, like this:
+
+```python
+app = make_wsgi_app(disable_compression=True)
+```
\ No newline at end of file
diff --git a/docs/content/exporting/pushgateway.md b/docs/content/exporting/pushgateway.md
new file mode 100644
index 0000000..f1ea5e4
--- /dev/null
+++ b/docs/content/exporting/pushgateway.md
@@ -0,0 +1,66 @@
+---
+title: Pushgateway
+weight: 3
+---
+
+The [Pushgateway](https://github.com/prometheus/pushgateway)
+allows ephemeral and batch jobs to expose their metrics to Prometheus.
+
+```python
+from prometheus_client import CollectorRegistry, Gauge, push_to_gateway
+
+registry = CollectorRegistry()
+g = Gauge('job_last_success_unixtime', 'Last time a batch job successfully finished', registry=registry)
+g.set_to_current_time()
+push_to_gateway('localhost:9091', job='batchA', registry=registry)
+```
+
+A separate registry is used, as the default registry may contain other metrics
+such as those from the Process Collector.
+
+Pushgateway functions take a grouping key. `push_to_gateway` replaces metrics
+with the same grouping key, `pushadd_to_gateway` only replaces metrics with the
+same name and grouping key and `delete_from_gateway` deletes metrics with the
+given job and grouping key. See the
+[Pushgateway documentation](https://github.com/prometheus/pushgateway/blob/master/README.md)
+for more information.
+
+`instance_ip_grouping_key` returns a grouping key with the instance label set
+to the host's IP address.
+
+# Handlers for authentication
+
+If the push gateway you are connecting to is protected with HTTP Basic Auth,
+you can use a special handler to set the Authorization header.
+
+```python
+from prometheus_client import CollectorRegistry, Gauge, push_to_gateway
+from prometheus_client.exposition import basic_auth_handler
+
+def my_auth_handler(url, method, timeout, headers, data):
+ username = 'foobar'
+ password = 'secret123'
+ return basic_auth_handler(url, method, timeout, headers, data, username, password)
+registry = CollectorRegistry()
+g = Gauge('job_last_success_unixtime', 'Last time a batch job successfully finished', registry=registry)
+g.set_to_current_time()
+push_to_gateway('localhost:9091', job='batchA', registry=registry, handler=my_auth_handler)
+```
+
+TLS Auth is also supported when using the push gateway with a special handler.
+
+```python
+from prometheus_client import CollectorRegistry, Gauge, push_to_gateway
+from prometheus_client.exposition import tls_auth_handler
+
+
+def my_auth_handler(url, method, timeout, headers, data):
+ certfile = 'client-crt.pem'
+ keyfile = 'client-key.pem'
+ return tls_auth_handler(url, method, timeout, headers, data, certfile, keyfile)
+
+registry = CollectorRegistry()
+g = Gauge('job_last_success_unixtime', 'Last time a batch job successfully finished', registry=registry)
+g.set_to_current_time()
+push_to_gateway('localhost:9091', job='batchA', registry=registry, handler=my_auth_handler)
+```
diff --git a/docs/content/exporting/textfile.md b/docs/content/exporting/textfile.md
new file mode 100644
index 0000000..80360e4
--- /dev/null
+++ b/docs/content/exporting/textfile.md
@@ -0,0 +1,23 @@
+---
+title: Node exporter textfile collector
+weight: 2
+---
+
+The [textfile collector](https://github.com/prometheus/node_exporter#textfile-collector)
+allows machine-level statistics to be exported out via the Node exporter.
+
+This is useful for monitoring cronjobs, or for writing cronjobs to expose metrics
+about a machine system that the Node exporter does not support or would not make sense
+to perform at every scrape (for example, anything involving subprocesses).
+
+```python
+from prometheus_client import CollectorRegistry, Gauge, write_to_textfile
+
+registry = CollectorRegistry()
+g = Gauge('raid_status', '1 if raid array is okay', registry=registry)
+g.set(1)
+write_to_textfile('/configured/textfile/path/raid.prom', registry)
+```
+
+A separate registry is used, as the default registry may contain other metrics
+such as those from the Process Collector.
\ No newline at end of file
diff --git a/docs/content/getting-started/_index.md b/docs/content/getting-started/_index.md
new file mode 100644
index 0000000..4272691
--- /dev/null
+++ b/docs/content/getting-started/_index.md
@@ -0,0 +1,4 @@
+---
+title: Getting Started
+weight: 1
+---
diff --git a/docs/content/getting-started/three-step-demo.md b/docs/content/getting-started/three-step-demo.md
new file mode 100644
index 0000000..bf06e39
--- /dev/null
+++ b/docs/content/getting-started/three-step-demo.md
@@ -0,0 +1,48 @@
+---
+title: Three Step Demo
+weight: 1
+---
+
+This tutorial shows the quickest way to get started with the Prometheus Python library.
+
+# Three Step Demo
+
+**One**: Install the client:
+```
+pip install prometheus-client
+```
+
+**Two**: Paste the following into a Python interpreter:
+```python
+from prometheus_client import start_http_server, Summary
+import random
+import time
+
+# Create a metric to track time spent and requests made.
+REQUEST_TIME = Summary('request_processing_seconds', 'Time spent processing request')
+
+# Decorate function with metric.
+@REQUEST_TIME.time()
+def process_request(t):
+ """A dummy function that takes some time."""
+ time.sleep(t)
+
+if __name__ == '__main__':
+ # Start up the server to expose the metrics.
+ start_http_server(8000)
+ # Generate some requests.
+ while True:
+ process_request(random.random())
+```
+
+**Three**: Visit [http://localhost:8000/](http://localhost:8000/) to view the metrics.
+
+From one easy to use decorator you get:
+ * `request_processing_seconds_count`: Number of times this function was called.
+ * `request_processing_seconds_sum`: Total amount of time spent in this function.
+
+Prometheus's `rate` function allows calculation of both requests per second,
+and latency over time from this data.
+
+In addition if you're on Linux the `process` metrics expose CPU, memory and
+other information about the process for free!
diff --git a/docs/content/instrumenting/_index.md b/docs/content/instrumenting/_index.md
new file mode 100644
index 0000000..7f28273
--- /dev/null
+++ b/docs/content/instrumenting/_index.md
@@ -0,0 +1,16 @@
+---
+title: Instrumenting
+weight: 2
+---
+
+Four types of metric are offered: Counter, Gauge, Summary and Histogram.
+See the documentation on [metric types](http://prometheus.io/docs/concepts/metric_types/)
+and [instrumentation best practices](https://prometheus.io/docs/practices/instrumentation/#counter-vs-gauge-summary-vs-histogram)
+on how to use them.
+
+## Disabling `_created` metrics
+
+By default counters, histograms, and summaries export an additional series
+suffixed with `_created` and a value of the unix timestamp for when the metric
+was created. If this information is not helpful, it can be disabled by setting
+the environment variable `PROMETHEUS_DISABLE_CREATED_SERIES=True`.
\ No newline at end of file
diff --git a/docs/content/instrumenting/counter.md b/docs/content/instrumenting/counter.md
new file mode 100644
index 0000000..9461802
--- /dev/null
+++ b/docs/content/instrumenting/counter.md
@@ -0,0 +1,34 @@
+---
+title: Counter
+weight: 1
+---
+
+Counters go up, and reset when the process restarts.
+
+
+```python
+from prometheus_client import Counter
+c = Counter('my_failures', 'Description of counter')
+c.inc() # Increment by 1
+c.inc(1.6) # Increment by given value
+```
+
+If there is a suffix of `_total` on the metric name, it will be removed. When
+exposing the time series for counter, a `_total` suffix will be added. This is
+for compatibility between OpenMetrics and the Prometheus text format, as OpenMetrics
+requires the `_total` suffix.
+
+There are utilities to count exceptions raised:
+
+```python
+@c.count_exceptions()
+def f():
+ pass
+
+with c.count_exceptions():
+ pass
+
+# Count only one type of exception
+with c.count_exceptions(ValueError):
+ pass
+```
\ No newline at end of file
diff --git a/docs/content/instrumenting/enum.md b/docs/content/instrumenting/enum.md
new file mode 100644
index 0000000..102091a
--- /dev/null
+++ b/docs/content/instrumenting/enum.md
@@ -0,0 +1,13 @@
+---
+title: Enum
+weight: 6
+---
+
+Enum tracks which of a set of states something is currently in.
+
+```python
+from prometheus_client import Enum
+e = Enum('my_task_state', 'Description of enum',
+ states=['starting', 'running', 'stopped'])
+e.state('running')
+```
\ No newline at end of file
diff --git a/docs/content/instrumenting/exemplars.md b/docs/content/instrumenting/exemplars.md
new file mode 100644
index 0000000..d472aad
--- /dev/null
+++ b/docs/content/instrumenting/exemplars.md
@@ -0,0 +1,37 @@
+---
+title: Exemplars
+weight: 8
+---
+
+Exemplars can be added to counter and histogram metrics. Exemplars can be
+specified by passing a dict of label value pairs to be exposed as the exemplar.
+For example with a counter:
+
+```python
+from prometheus_client import Counter
+c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
+c.labels('get', '/').inc(exemplar={'trace_id': 'abc123'})
+c.labels('post', '/submit').inc(1.0, {'trace_id': 'def456'})
+```
+
+And with a histogram:
+
+```python
+from prometheus_client import Histogram
+h = Histogram('request_latency_seconds', 'Description of histogram')
+h.observe(4.7, {'trace_id': 'abc123'})
+```
+
+Exemplars are only rendered in the OpenMetrics exposition format. If using the
+HTTP server or apps in this library, content negotiation can be used to specify
+OpenMetrics (which is done by default in Prometheus). Otherwise it will be
+necessary to use `generate_latest` from
+`prometheus_client.openmetrics.exposition` to view exemplars.
+
+To view exemplars in Prometheus it is also necessary to enable the the
+exemplar-storage feature flag:
+```
+--enable-feature=exemplar-storage
+```
+Additional information is available in [the Prometheus
+documentation](https://prometheus.io/docs/prometheus/latest/feature_flags/#exemplars-storage).
diff --git a/docs/content/instrumenting/gauge.md b/docs/content/instrumenting/gauge.md
new file mode 100644
index 0000000..0b1529e
--- /dev/null
+++ b/docs/content/instrumenting/gauge.md
@@ -0,0 +1,36 @@
+---
+title: Gauge
+weight: 2
+---
+
+Gauges can go up and down.
+
+```python
+from prometheus_client import Gauge
+g = Gauge('my_inprogress_requests', 'Description of gauge')
+g.inc() # Increment by 1
+g.dec(10) # Decrement by given value
+g.set(4.2) # Set to a given value
+```
+
+There are utilities for common use cases:
+
+```python
+g.set_to_current_time() # Set to current unixtime
+
+# Increment when entered, decrement when exited.
+@g.track_inprogress()
+def f():
+ pass
+
+with g.track_inprogress():
+ pass
+```
+
+A Gauge can also take its value from a callback:
+
+```python
+d = Gauge('data_objects', 'Number of objects')
+my_dict = {}
+d.set_function(lambda: len(my_dict))
+```
\ No newline at end of file
diff --git a/docs/content/instrumenting/histogram.md b/docs/content/instrumenting/histogram.md
new file mode 100644
index 0000000..cb85f18
--- /dev/null
+++ b/docs/content/instrumenting/histogram.md
@@ -0,0 +1,27 @@
+---
+title: Histogram
+weight: 4
+---
+
+Histograms track the size and number of events in buckets.
+This allows for aggregatable calculation of quantiles.
+
+```python
+from prometheus_client import Histogram
+h = Histogram('request_latency_seconds', 'Description of histogram')
+h.observe(4.7) # Observe 4.7 (seconds in this case)
+```
+
+The default buckets are intended to cover a typical web/rpc request from milliseconds to seconds.
+They can be overridden by passing `buckets` keyword argument to `Histogram`.
+
+There are utilities for timing code:
+
+```python
+@h.time()
+def f():
+ pass
+
+with h.time():
+ pass
+```
\ No newline at end of file
diff --git a/docs/content/instrumenting/info.md b/docs/content/instrumenting/info.md
new file mode 100644
index 0000000..6334d92
--- /dev/null
+++ b/docs/content/instrumenting/info.md
@@ -0,0 +1,12 @@
+---
+title: Info
+weight: 5
+---
+
+Info tracks key-value information, usually about a whole target.
+
+```python
+from prometheus_client import Info
+i = Info('my_build_version', 'Description of info')
+i.info({'version': '1.2.3', 'buildhost': 'foo@bar'})
+```
diff --git a/docs/content/instrumenting/labels.md b/docs/content/instrumenting/labels.md
new file mode 100644
index 0000000..ebf80b5
--- /dev/null
+++ b/docs/content/instrumenting/labels.md
@@ -0,0 +1,38 @@
+---
+title: Labels
+weight: 7
+---
+
+All metrics can have labels, allowing grouping of related time series.
+
+See the best practices on [naming](http://prometheus.io/docs/practices/naming/)
+and [labels](http://prometheus.io/docs/practices/instrumentation/#use-labels).
+
+Taking a counter as an example:
+
+```python
+from prometheus_client import Counter
+c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
+c.labels('get', '/').inc()
+c.labels('post', '/submit').inc()
+```
+
+Labels can also be passed as keyword-arguments:
+
+```python
+from prometheus_client import Counter
+c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
+c.labels(method='get', endpoint='/').inc()
+c.labels(method='post', endpoint='/submit').inc()
+```
+
+Metrics with labels are not initialized when declared, because the client can't
+know what values the label can have. It is recommended to initialize the label
+values by calling the `.labels()` method alone:
+
+```python
+from prometheus_client import Counter
+c = Counter('my_requests_total', 'HTTP Failures', ['method', 'endpoint'])
+c.labels('get', '/')
+c.labels('post', '/submit')
+```
\ No newline at end of file
diff --git a/docs/content/instrumenting/summary.md b/docs/content/instrumenting/summary.md
new file mode 100644
index 0000000..fa40749
--- /dev/null
+++ b/docs/content/instrumenting/summary.md
@@ -0,0 +1,25 @@
+---
+title: Summary
+weight: 3
+---
+
+Summaries track the size and number of events.
+
+```python
+from prometheus_client import Summary
+s = Summary('request_latency_seconds', 'Description of summary')
+s.observe(4.7) # Observe 4.7 (seconds in this case)
+```
+
+There are utilities for timing code:
+
+```python
+@s.time()
+def f():
+ pass
+
+with s.time():
+ pass
+```
+
+The Python client doesn't store or expose quantile information at this time.
\ No newline at end of file
diff --git a/docs/content/multiprocess/_index.md b/docs/content/multiprocess/_index.md
new file mode 100644
index 0000000..c69cad8
--- /dev/null
+++ b/docs/content/multiprocess/_index.md
@@ -0,0 +1,93 @@
+---
+title: Multiprocess Mode
+weight: 5
+---
+
+Prometheus client libraries presume a threaded model, where metrics are shared
+across workers. This doesn't work so well for languages such as Python where
+it's common to have processes rather than threads to handle large workloads.
+
+To handle this the client library can be put in multiprocess mode.
+This comes with a number of limitations:
+
+- Registries can not be used as normal, all instantiated metrics are exported
+ - Registering metrics to a registry later used by a `MultiProcessCollector`
+ may cause duplicate metrics to be exported
+- Custom collectors do not work (e.g. cpu and memory metrics)
+- Info and Enum metrics do not work
+- The pushgateway cannot be used
+- Gauges cannot use the `pid` label
+- Exemplars are not supported
+
+There's several steps to getting this working:
+
+**1. Deployment**:
+
+The `PROMETHEUS_MULTIPROC_DIR` environment variable must be set to a directory
+that the client library can use for metrics. This directory must be wiped
+between process/Gunicorn runs (before startup is recommended).
+
+This environment variable should be set from a start-up shell script,
+and not directly from Python (otherwise it may not propagate to child processes).
+
+**2. Metrics collector**:
+
+The application must initialize a new `CollectorRegistry`, and store the
+multi-process collector inside. It is a best practice to create this registry
+inside the context of a request to avoid metrics registering themselves to a
+collector used by a `MultiProcessCollector`. If a registry with metrics
+registered is used by a `MultiProcessCollector` duplicate metrics may be
+exported, one for multiprocess, and one for the process serving the request.
+
+```python
+from prometheus_client import multiprocess
+from prometheus_client import generate_latest, CollectorRegistry, CONTENT_TYPE_LATEST, Counter
+
+MY_COUNTER = Counter('my_counter', 'Description of my counter')
+
+# Expose metrics.
+def app(environ, start_response):
+ registry = CollectorRegistry()
+ multiprocess.MultiProcessCollector(registry)
+ data = generate_latest(registry)
+ status = '200 OK'
+ response_headers = [
+ ('Content-type', CONTENT_TYPE_LATEST),
+ ('Content-Length', str(len(data)))
+ ]
+ start_response(status, response_headers)
+ return iter([data])
+```
+
+**3. Gunicorn configuration**:
+
+The `gunicorn` configuration file needs to include the following function:
+
+```python
+from prometheus_client import multiprocess
+
+def child_exit(server, worker):
+ multiprocess.mark_process_dead(worker.pid)
+```
+
+**4. Metrics tuning (Gauge)**:
+
+When `Gauge`s are used in multiprocess applications,
+you must decide how to handle the metrics reported by each process.
+Gauges have several modes they can run in, which can be selected with the `multiprocess_mode` parameter.
+
+- 'all': Default. Return a timeseries per process (alive or dead), labelled by the process's `pid` (the label is added internally).
+- 'min': Return a single timeseries that is the minimum of the values of all processes (alive or dead).
+- 'max': Return a single timeseries that is the maximum of the values of all processes (alive or dead).
+- 'sum': Return a single timeseries that is the sum of the values of all processes (alive or dead).
+- 'mostrecent': Return a single timeseries that is the most recent value among all processes (alive or dead).
+
+Prepend 'live' to the beginning of the mode to return the same result but only considering living processes
+(e.g., 'liveall, 'livesum', 'livemax', 'livemin', 'livemostrecent').
+
+```python
+from prometheus_client import Gauge
+
+# Example gauge
+IN_PROGRESS = Gauge("inprogress_requests", "help", multiprocess_mode='livesum')
+```
diff --git a/docs/content/parser/_index.md b/docs/content/parser/_index.md
new file mode 100644
index 0000000..19f3b0e
--- /dev/null
+++ b/docs/content/parser/_index.md
@@ -0,0 +1,16 @@
+---
+title: Parser
+weight: 6
+---
+
+The Python client supports parsing the Prometheus text format.
+This is intended for advanced use cases where you have servers
+exposing Prometheus metrics and need to get them into some other
+system.
+
+```python
+from prometheus_client.parser import text_string_to_metric_families
+for family in text_string_to_metric_families(u"my_gauge 1.0\n"):
+ for sample in family.samples:
+ print("Name: {0} Labels: {1} Value: {2}".format(*sample))
+```
\ No newline at end of file
diff --git a/docs/content/restricted-registry/_index.md b/docs/content/restricted-registry/_index.md
new file mode 100644
index 0000000..49b36e1
--- /dev/null
+++ b/docs/content/restricted-registry/_index.md
@@ -0,0 +1,29 @@
+---
+title: Restricted registry
+weight: 7
+---
+
+Registries support restriction to only return specific metrics.
+If you’re using the built-in HTTP server, you can use the GET parameter "name[]", since it’s an array it can be used multiple times.
+If you’re directly using `generate_latest`, you can use the function `restricted_registry()`.
+
+```python
+curl --get --data-urlencode "name[]=python_gc_objects_collected_total" --data-urlencode "name[]=python_info" http://127.0.0.1:9200/metrics
+```
+
+```python
+from prometheus_client import generate_latest
+
+generate_latest(REGISTRY.restricted_registry(['python_gc_objects_collected_total', 'python_info']))
+```
+
+```python
+# HELP python_info Python platform information
+# TYPE python_info gauge
+python_info{implementation="CPython",major="3",minor="9",patchlevel="3",version="3.9.3"} 1.0
+# HELP python_gc_objects_collected_total Objects collected during gc
+# TYPE python_gc_objects_collected_total counter
+python_gc_objects_collected_total{generation="0"} 73129.0
+python_gc_objects_collected_total{generation="1"} 8594.0
+python_gc_objects_collected_total{generation="2"} 296.0
+```
\ No newline at end of file
diff --git a/docs/data/menu/extra.yaml b/docs/data/menu/extra.yaml
new file mode 100644
index 0000000..7ee8af7
--- /dev/null
+++ b/docs/data/menu/extra.yaml
@@ -0,0 +1,6 @@
+---
+header:
+ - name: GitHub
+ ref: https://github.com/prometheus/client_python
+ icon: gdoc_github
+ external: true
diff --git a/docs/data/menu/more.yaml b/docs/data/menu/more.yaml
new file mode 100644
index 0000000..ea3b861
--- /dev/null
+++ b/docs/data/menu/more.yaml
@@ -0,0 +1,14 @@
+---
+more:
+ - name: Releases
+ ref: "https://github.com/prometheus/client_python/releases"
+ external: true
+ icon: "gdoc_download"
+ - name: GitHub
+ ref: "https://github.com/prometheus/client_python"
+ external: true
+ icon: "gdoc_github"
+ - name: PyPi
+ ref: "https://pypi.python.org/pypi/prometheus_client"
+ icon: "gdoc_link"
+ external: true
diff --git a/docs/hugo.toml b/docs/hugo.toml
new file mode 100644
index 0000000..0888549
--- /dev/null
+++ b/docs/hugo.toml
@@ -0,0 +1,30 @@
+baseURL = "http://localhost"
+languageCode = 'en-us'
+title = "client_python"
+theme = "hugo-geekdoc"
+
+pluralizeListTitles = false
+
+# Geekdoc required configuration
+#pygmentsUseClasses = true
+pygmentsUseClasses = false
+pygmentsCodeFences = true
+disablePathToLower = true
+# geekdocFileTreeSortBy = "linkTitle"
+
+# Required if you want to render robots.txt template
+enableRobotsTXT = true
+
+# Needed for mermaid shortcodes
+[markup]
+ [markup.goldmark.renderer]
+ # Needed for mermaid shortcode
+ unsafe = true
+ [markup.tableOfContents]
+ startLevel = 1
+ endLevel = 9
+ [markup.highlight]
+ style = 'solarized-dark'
+
+[taxonomies]
+ tag = "tags"
diff --git a/docs/static/.gitignore b/docs/static/.gitignore
new file mode 100644
index 0000000..eedd89b
--- /dev/null
+++ b/docs/static/.gitignore
@@ -0,0 +1 @@
+api
diff --git a/docs/static/brand.svg b/docs/static/brand.svg
new file mode 100644
index 0000000..5c51f66
--- /dev/null
+++ b/docs/static/brand.svg
@@ -0,0 +1,50 @@
+
+
+
+
\ No newline at end of file
diff --git a/docs/static/custom.css b/docs/static/custom.css
new file mode 100644
index 0000000..41ab7c5
--- /dev/null
+++ b/docs/static/custom.css
@@ -0,0 +1,39 @@
+/*
+ * Didn't find much time to create a theme yet,
+ * so there are just a few non-default settings for now.
+ */
+:root,
+:root[color-theme="light"] {
+ --header-background: #222222;
+ --footer-background: #e6522c;
+ --footer-link-color: #ffffff;
+ --footer-link-color-visited: #ffffff;
+}
+
+@media (prefers-color-scheme: light) {
+ :root {
+ --header-background: #222222;
+ --footer-background: #e6522c;
+ --footer-link-color: #ffffff;
+ --footer-link-color-visited: #ffffff;
+ }
+}
+
+:root[color-theme="dark"]
+{
+ --header-background: #111c24;
+ --body-background: #1f1f21;
+ --footer-background: #e6522c;
+ --footer-link-color: #ffffff;
+ --footer-link-color-visited: #ffffff;
+}
+
+@media (prefers-color-scheme: dark) {
+ :root {
+ --header-background: #111c24;
+ --body-background: #1f1f21;
+ --footer-background: #e6522c;
+ --footer-link-color: #ffffff;
+ --footer-link-color-visited: #ffffff;
+ }
+}
diff --git a/docs/static/favicon/favicon-16x16.png b/docs/static/favicon/favicon-16x16.png
new file mode 100644
index 0000000..7a8cc58
Binary files /dev/null and b/docs/static/favicon/favicon-16x16.png differ
diff --git a/docs/static/favicon/favicon-32x32.png b/docs/static/favicon/favicon-32x32.png
new file mode 100644
index 0000000..7d5a3ae
Binary files /dev/null and b/docs/static/favicon/favicon-32x32.png differ
diff --git a/docs/static/favicon/favicon.ico b/docs/static/favicon/favicon.ico
new file mode 100644
index 0000000..34bd1fb
Binary files /dev/null and b/docs/static/favicon/favicon.ico differ
diff --git a/docs/static/favicon/favicon.svg b/docs/static/favicon/favicon.svg
new file mode 100644
index 0000000..5c51f66
--- /dev/null
+++ b/docs/static/favicon/favicon.svg
@@ -0,0 +1,50 @@
+
+
+
+
\ No newline at end of file
diff --git a/docs/themes/hugo-geekdoc/LICENSE b/docs/themes/hugo-geekdoc/LICENSE
new file mode 100644
index 0000000..3812eb4
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/LICENSE
@@ -0,0 +1,21 @@
+MIT License
+
+Copyright (c) 2022 Robert Kaussow
+
+Permission is hereby granted, free of charge, to any person obtaining a copy
+of this software and associated documentation files (the "Software"), to deal
+in the Software without restriction, including without limitation the rights
+to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
+copies of the Software, and to permit persons to whom the Software is furnished
+to do so, subject to the following conditions:
+
+The above copyright notice and this permission notice (including the next
+paragraph) shall be included in all copies or substantial portions of the
+Software.
+
+THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
+IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS
+FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS
+OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
+WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF
+OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
diff --git a/docs/themes/hugo-geekdoc/README.md b/docs/themes/hugo-geekdoc/README.md
new file mode 100644
index 0000000..99358d8
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/README.md
@@ -0,0 +1,46 @@
+# Geekdoc
+
+[](https://ci.thegeeklab.de/repos/thegeeklab/hugo-geekdoc)
+[](https://gohugo.io)
+[](https://github.com/thegeeklab/hugo-geekdoc/releases/latest)
+[](https://github.com/thegeeklab/hugo-geekdoc/graphs/contributors)
+[](https://github.com/thegeeklab/hugo-geekdoc/blob/main/LICENSE)
+
+Geekdoc is a simple Hugo theme for documentations. It is intentionally designed as a fast and lean theme and may not fit the requirements of complex projects. If a more feature-complete theme is required there are a lot of good alternatives out there. You can find a demo and the full documentation at [https://geekdocs.de](https://geekdocs.de).
+
+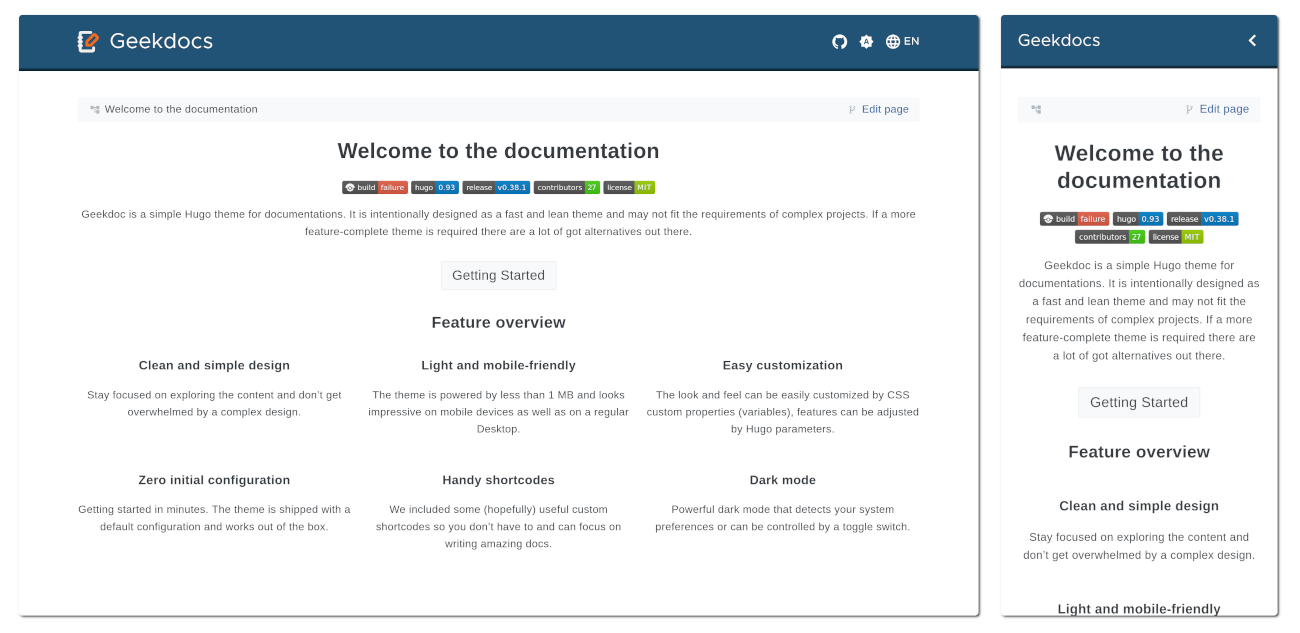
+
+## Build and release process
+
+This theme is subject to a CI driven build and release process common for software development. During the release build, all necessary assets are automatically built by [webpack](https://webpack.js.org/) and bundled in a release tarball. You can download the latest release from the GitHub [release page](https://github.com/thegeeklab/hugo-geekdoc/releases).
+
+Due to the fact that `webpack` and `npm scripts` are used as pre-processors, the theme cannot be used from the main branch by default. If you want to use the theme from a cloned branch instead of a release tarball you'll need to install `webpack` locally and run the build script once to create all required assets.
+
+```shell
+# install required packages from package.json
+npm install
+
+# run the build script to build required assets
+npm run build
+
+# build release tarball
+npm run pack
+```
+
+See the [Getting Started Guide](https://geekdocs.de/usage/getting-started/) for details about the different setup options.
+
+## Contributors
+
+Special thanks to all [contributors](https://github.com/thegeeklab/hugo-geekdoc/graphs/contributors). If you would like to contribute, please see the [instructions](https://github.com/thegeeklab/hugo-geekdoc/blob/main/CONTRIBUTING.md).
+
+Geekdoc is inspired and partially based on the [hugo-book](https://github.com/alex-shpak/hugo-book) theme, thanks [Alex Shpak](https://github.com/alex-shpak/) for your work.
+
+## License
+
+This project is licensed under the MIT License - see the [LICENSE](https://github.com/thegeeklab/hugo-geekdoc/blob/main/LICENSE) file for details.
+
+The used SVG icons and generated icon fonts are licensed under the license of the respective icon pack:
+
+- Font Awesome: [CC BY 4.0 License](https://github.com/FortAwesome/Font-Awesome#license)
+- IcoMoon Free Pack: [GPL/CC BY 4.0](https://icomoon.io/#icons-icomoon)
+- Material Icons: [Apache License 2.0](https://github.com/google/material-design-icons/blob/main/LICENSE)
diff --git a/docs/themes/hugo-geekdoc/VERSION b/docs/themes/hugo-geekdoc/VERSION
new file mode 100644
index 0000000..d0cca40
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/VERSION
@@ -0,0 +1 @@
+v0.41.1
diff --git a/docs/themes/hugo-geekdoc/archetypes/docs.md b/docs/themes/hugo-geekdoc/archetypes/docs.md
new file mode 100644
index 0000000..aa0d88f
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/archetypes/docs.md
@@ -0,0 +1,7 @@
+---
+title: "{{ .Name | humanize | title }}"
+weight: 1
+# geekdocFlatSection: false
+# geekdocToc: 6
+# geekdocHidden: false
+---
diff --git a/docs/themes/hugo-geekdoc/archetypes/posts.md b/docs/themes/hugo-geekdoc/archetypes/posts.md
new file mode 100644
index 0000000..fdccff8
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/archetypes/posts.md
@@ -0,0 +1,4 @@
+---
+title: "{{ replace .Name "-" " " | title }}"
+date: {{ .Date }}
+---
diff --git a/docs/themes/hugo-geekdoc/assets/search/config.json b/docs/themes/hugo-geekdoc/assets/search/config.json
new file mode 100644
index 0000000..1a5582a
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/assets/search/config.json
@@ -0,0 +1,8 @@
+{{- $searchDataFile := printf "search/%s.data.json" .Language.Lang -}}
+{{- $searchData := resources.Get "search/data.json" | resources.ExecuteAsTemplate $searchDataFile . | resources.Minify -}}
+{
+ "dataFile": {{ $searchData.RelPermalink | jsonify }},
+ "indexConfig": {{ .Site.Params.geekdocSearchConfig | jsonify }},
+ "showParent": {{ if .Site.Params.geekdocSearchShowParent }}true{{ else }}false{{ end }},
+ "showDescription": {{ if .Site.Params.geekdocSearchshowDescription }}true{{ else }}false{{ end }}
+}
diff --git a/docs/themes/hugo-geekdoc/assets/search/data.json b/docs/themes/hugo-geekdoc/assets/search/data.json
new file mode 100644
index 0000000..f1c0e80
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/assets/search/data.json
@@ -0,0 +1,13 @@
+[
+ {{ range $index, $page := (where .Site.Pages "Params.geekdocProtected" "ne" true) }}
+ {{ if ne $index 0 }},{{ end }}
+ {
+ "id": {{ $index }},
+ "href": "{{ $page.RelPermalink }}",
+ "title": {{ (partial "utils/title" $page) | jsonify }},
+ "parent": {{ with $page.Parent }}{{ (partial "utils/title" .) | jsonify }}{{ else }}""{{ end }},
+ "content": {{ $page.Plain | jsonify }},
+ "description": {{ $page.Summary | plainify | jsonify }}
+ }
+ {{ end }}
+]
diff --git a/docs/themes/hugo-geekdoc/assets/sprites/geekdoc.svg b/docs/themes/hugo-geekdoc/assets/sprites/geekdoc.svg
new file mode 100644
index 0000000..4f3cfd2
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/assets/sprites/geekdoc.svg
@@ -0,0 +1 @@
+
\ No newline at end of file
diff --git a/docs/themes/hugo-geekdoc/data/assets.json b/docs/themes/hugo-geekdoc/data/assets.json
new file mode 100644
index 0000000..9a34ed5
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/data/assets.json
@@ -0,0 +1,158 @@
+{
+ "main.js": {
+ "src": "js/main-924a1933.bundle.min.js",
+ "integrity": "sha512-0QF6awwW0WbBo491yytmULiHrc9gx94bloJ9MSXIvdJh3YHWw7CWyeX2YXu0rzOQefJp4jW/I6ZjUDYpNVFhdA=="
+ },
+ "colortheme.js": {
+ "src": "js/colortheme-d3e4d351.bundle.min.js",
+ "integrity": "sha512-HpQogL/VeKqG/v1qYOfJOgFUzBnQvW4yO4tAJO+54IiwbLbB9feROdeaYf7dpO6o5tSHsSZhaYLhtLMRlEgpJQ=="
+ },
+ "mermaid.js": {
+ "src": "js/mermaid-d305d450.bundle.min.js",
+ "integrity": "sha512-TASG03QptoVv1mkfOL47vm5A5kvmyOrnsi8PXhc82j1+FuHZuMOHXc2x5/jGEkOxbKi7mum0h/W7qYhrV29raw=="
+ },
+ "katex.js": {
+ "src": "js/katex-d4d5881d.bundle.min.js",
+ "integrity": "sha512-M8CLtMTu/HVXo11Et+lv3OqPanLf5Bl+GljNAn2yQuLclg/ZpZK1KUpHDRsZJhmkhCcCH90+bVj5CW3lLlmBgg=="
+ },
+ "search.js": {
+ "src": "js/search-9719be99.bundle.min.js",
+ "integrity": "sha512-/7NZxFUEbalC/8RKDgfAsHFDI42/Ydp33uJmCLckZgnO+kuz9LrTfmPFfVJxPJ31StMxa3MTQ5Jq049CmNK4pw=="
+ },
+ "js/637-86fbbecd.chunk.min.js": {
+ "src": "js/637-86fbbecd.chunk.min.js",
+ "integrity": "sha512-vD1y0C4MPPV/JhEKmNVAye9SQg7mB5v87nLf63keSALdnM7P+L0ybjEn2MzYzVTzs6JnOCryM7A6/t0TkYucDA=="
+ },
+ "js/116-341f79d9.chunk.min.js": {
+ "src": "js/116-341f79d9.chunk.min.js",
+ "integrity": "sha512-F7tq1KsF5mnJl0AAA6x2jZcx8x69kEZrUIZJJM4RZ1KlEO79yrrFHf4CZRvhNrYOxmkBpkQ84U9J0vFtRPKjTw=="
+ },
+ "js/545-8e970b03.chunk.min.js": {
+ "src": "js/545-8e970b03.chunk.min.js",
+ "integrity": "sha512-vDOXX1FstnT8UMkRIAMn6z4ucL8LVqI5kZw+T7LrD8pGC9xtKwwhWcmNeqnngc7FHc5Ogt7ppXBNp+uFPUgrJg=="
+ },
+ "js/728-5df4a5e5.chunk.min.js": {
+ "src": "js/728-5df4a5e5.chunk.min.js",
+ "integrity": "sha512-vX2dPV1VjOgv8DP4XbZ9xk9ZzHS9hPAUwPfHM8UG42efxXxH/qCqTpyavqob98onxR2FuiF+j1Vn56d3wqsgaw=="
+ },
+ "js/81-4e653aac.chunk.min.js": {
+ "src": "js/81-4e653aac.chunk.min.js",
+ "integrity": "sha512-a80h3DpDlMG6HhYXv9n9Q7r1M+rQX5kfJ7sFhfmPHlDRVimult9nn7vvTHFzTzmMFM+tLcfg4pZGd+AkxPcjEw=="
+ },
+ "js/430-cc171d93.chunk.min.js": {
+ "src": "js/430-cc171d93.chunk.min.js",
+ "integrity": "sha512-cqQyiIE22ZPo2PIPR4eb0DPSu1TWjxgJUKrIIyfVF48gc2vdLKnbHzWBZg6MpiOWYmUvJb5Ki+n5U6qEvNp2KQ=="
+ },
+ "js/729-32b017b3.chunk.min.js": {
+ "src": "js/729-32b017b3.chunk.min.js",
+ "integrity": "sha512-KAW7lnN0NHmIzfD6aIwVaU3TcpUkO8ladLrbOE83zq80NOJH/MGS4Ep+2rIfQZTvZP+a7nqZLHkmezfs27c2pw=="
+ },
+ "js/773-8f0c4fb8.chunk.min.js": {
+ "src": "js/773-8f0c4fb8.chunk.min.js",
+ "integrity": "sha512-HxtbZvs0J28pB9fImN8n82aprG/GW0QenIBzC7BHWhEUX6IhmxTeBqG4IZFbbAURG17VegOr2UlJg6w0qaX9gw=="
+ },
+ "js/433-f2655a46.chunk.min.js": {
+ "src": "js/433-f2655a46.chunk.min.js",
+ "integrity": "sha512-/yMUz6rxhVpvCPpQG+f28jFgdJK+X/5/3XWVsrAE2FHC57jxnHaL7SxZluZ4klUl0YsRCrhxAQj8maNspwwH1Q=="
+ },
+ "js/546-560b35c2.chunk.min.js": {
+ "src": "js/546-560b35c2.chunk.min.js",
+ "integrity": "sha512-am1/hYno7/cFQ8reHZqnbsth2KcphvKqLfkueVIm8I/i/6f9u+bbc0Z6zpYTLysl3oWddYXqyeO58zXoJoDVIA=="
+ },
+ "js/118-f1de6a20.chunk.min.js": {
+ "src": "js/118-f1de6a20.chunk.min.js",
+ "integrity": "sha512-tikydCOmBT1keN0AlCqvkpvbV1gB9U8lVXX8wmrS6fQ2faNc8DnH1QV9dzAlLtGeA1p8HAXnh+AevnVKxhXVbg=="
+ },
+ "js/19-86f47ecd.chunk.min.js": {
+ "src": "js/19-86f47ecd.chunk.min.js",
+ "integrity": "sha512-qRG0UrV25Kr/36tJTPZ49QobR6a/zv2BRAMDzSZwjlPgqSwse1HtgP9EEZtn59b1Vq7ayB1LoWfB9MZ9Gcm7Gw=="
+ },
+ "js/361-f7cd601a.chunk.min.js": {
+ "src": "js/361-f7cd601a.chunk.min.js",
+ "integrity": "sha512-7kwaFQhXUyiM/v2v0n6vI9wz6nSAu7sz2236r+MbwT0r4aBxYqeOxij+PkGnTUqR2n1UExnbWKjuruDi9V/H5g=="
+ },
+ "js/519-8d0cec7f.chunk.min.js": {
+ "src": "js/519-8d0cec7f.chunk.min.js",
+ "integrity": "sha512-tFsZN3iyUMIMeB/b4E1PZNOFDKqMM4Fes63RGNkHNhtRTL/AIUpqPcTKZ+Fi2ZTdyYvPSTtjc5urnzLUi196Wg=="
+ },
+ "js/747-b55f0f97.chunk.min.js": {
+ "src": "js/747-b55f0f97.chunk.min.js",
+ "integrity": "sha512-hoyvC5SSJcX9NGij9J9l4Ov1JAFNBX0UxlFXyiB5TC7TGW3lgIvm41zyfKhLyJudVGftY/qKxIO2EYtYD2pqOQ=="
+ },
+ "js/642-12e7dea2.chunk.min.js": {
+ "src": "js/642-12e7dea2.chunk.min.js",
+ "integrity": "sha512-ZVUj7NYSa8mMHdWaztAf3DCg7qznXTbMWWwqJaS2nfaqh0lVDOf5kVExPy6SGkXCeNu/B9gGbWLtDUa7kHFF6A=="
+ },
+ "js/626-1706197a.chunk.min.js": {
+ "src": "js/626-1706197a.chunk.min.js",
+ "integrity": "sha512-OlpbPXiGmQJR/ISfBSsHU2UGATggZDuHbopvAhhfVpw7ObMZgc/UvE6pK1FmGCjgI9iS+qmPhQyvf9SIbLFyxQ=="
+ },
+ "js/438-760c9ed3.chunk.min.js": {
+ "src": "js/438-760c9ed3.chunk.min.js",
+ "integrity": "sha512-Wo2DxS59Y8DVBTWNWDUmg6V+UCyLoiDd4sPs2xc7TkflQy7reGWPt/oHZCANXeGjZPpqcR3qfKYidNofUyIWEA=="
+ },
+ "js/639-88c6538a.chunk.min.js": {
+ "src": "js/639-88c6538a.chunk.min.js",
+ "integrity": "sha512-KbTKHxx+/Xwv+GH8jQsIJ9X1CFaGSsqgeSXnR8pW27YZpFz4ly8R6K7h+yq6P2b2AzQdW7krradZzyNo7Vz26w=="
+ },
+ "js/940-25dfc794.chunk.min.js": {
+ "src": "js/940-25dfc794.chunk.min.js",
+ "integrity": "sha512-qst5aejItmhzMvZ3CsAXyJe2F3FtLkyZwBqj422/8ViyQptcQFgP3x8bPsLwJEfiWFJVrLJkk4VhwflQuIyDtw=="
+ },
+ "js/662-17acb8f4.chunk.min.js": {
+ "src": "js/662-17acb8f4.chunk.min.js",
+ "integrity": "sha512-S/UlqDqwt++RzVZMVqjsdCNyhe1xNQ9/Qm38yIphmXfn9VBHzGqobIQTuhloYZVfTE4/GezrH+1T7mdrUqpAKQ=="
+ },
+ "js/579-9222afff.chunk.min.js": {
+ "src": "js/579-9222afff.chunk.min.js",
+ "integrity": "sha512-rl3bxxl/uhUFYlIuoHfVQE+VkmxfJr7TAuC/fxOBJXBCCMpdxP0XCPzms1zjEjOVjIs4bi4SUwn8r4STSl09Lg=="
+ },
+ "js/771-942a62df.chunk.min.js": {
+ "src": "js/771-942a62df.chunk.min.js",
+ "integrity": "sha512-8WfA8U1Udlfa6uWAYbdNKJzjlJ91qZ0ZhC+ldKdhghUgilxqA6UmZxHFKGRDQydjOFDk828O28XVmZU2IEvckA=="
+ },
+ "js/506-6950d52c.chunk.min.js": {
+ "src": "js/506-6950d52c.chunk.min.js",
+ "integrity": "sha512-h2po0SsM4N3IXiBbNWlIbasxX7zSm5XVDpgYfmsEmcfQkMcFwJtTJGppek085Mxi1XZmrhjfxq2AUtnUs03LJg=="
+ },
+ "js/76-732e78f1.chunk.min.js": {
+ "src": "js/76-732e78f1.chunk.min.js",
+ "integrity": "sha512-ZjF2oB76jiCtdQNJZ9v1MUJSPaBcZCXmTA2T3qDBuU260uVA99wGeprrNQ3WdHQeK+VYXCq26dOE9w+I3b6Q4w=="
+ },
+ "js/476-86e5cf96.chunk.min.js": {
+ "src": "js/476-86e5cf96.chunk.min.js",
+ "integrity": "sha512-siq24cNKFc1tXGACAQlpbXOb2gRKDnncf39INGAPlnJSiAsYewhNusq1UxhMDFA836eucVq7NzE1TqEYskI0ug=="
+ },
+ "js/813-0d3c16f5.chunk.min.js": {
+ "src": "js/813-0d3c16f5.chunk.min.js",
+ "integrity": "sha512-gDVyQtM781xlTfyZzuEJ1tnQWyasbFKLRPwgGUF5lpdS3QpW6KTIwCnMuVn2b5XF2qKSxpei9YNIushpBI4ILA=="
+ },
+ "js/423-897d7f17.chunk.min.js": {
+ "src": "js/423-897d7f17.chunk.min.js",
+ "integrity": "sha512-ERAmXYsLT59PDGFPLTHNgaNw5CsaTOK88orlaXr+7SOxf+Yjf5fvDmpXCNJe1odvU6OF4cajtlVM1qO9hzOqWw=="
+ },
+ "js/535-dcead599.chunk.min.js": {
+ "src": "js/535-dcead599.chunk.min.js",
+ "integrity": "sha512-3gB2l6iJbt94EMd1Xh6udlMXjdHlAbuRKkyl87X/LSuG1fGbfTe11O5ma+un13BBX1wZ1xnHtUv6Fyc3pgbgDg=="
+ },
+ "main.scss": {
+ "src": "main-252d384c.min.css",
+ "integrity": "sha512-WiV7BVk76Yp0EACJrwdWDk7+WNa+Jyiupi9aCKFrzZyiKkXk7BH+PL2IJcuDQpCMtMBFJEgen2fpKu9ExjjrUQ=="
+ },
+ "katex.css": {
+ "src": "katex-66092164.min.css",
+ "integrity": "sha512-ng+uY3bZP0IENn+fO0T+jdk1v1r7HQJjsVRJgzU+UiJJadAevmo0gVNrpVxrBFGpRQqSz42q20uTre1C1Qrnow=="
+ },
+ "mobile.scss": {
+ "src": "mobile-79ddc617.min.css",
+ "integrity": "sha512-dzw2wMOouDwhSgstQKLbXD/vIqS48Ttc2IV6DeG7yam9yvKUuChJVaworzL8s2UoGMX4x2jEm50PjFJE4R4QWw=="
+ },
+ "print.scss": {
+ "src": "print-735ccc12.min.css",
+ "integrity": "sha512-c28KLNtBnKDW1+/bNWFhwuGBLw9octTXA2wnuaS2qlvpNFL0DytCapui9VM4YYkZg6e9TVp5LyuRQc2lTougDw=="
+ },
+ "custom.css": {
+ "src": "custom.css",
+ "integrity": "sha512-1kALo+zc1L2u1rvyxPIew+ZDPWhnIA1Ei2rib3eHHbskQW+EMxfI9Ayyva4aV+YRrHvH0zFxvPSFIuZ3mfsbRA=="
+ }
+}
\ No newline at end of file
diff --git a/docs/themes/hugo-geekdoc/i18n/cs.yaml b/docs/themes/hugo-geekdoc/i18n/cs.yaml
new file mode 100644
index 0000000..71dd8ed
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/cs.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: Upravit stránku
+
+nav_navigation: Navigace
+nav_tags: Tagy
+nav_more: Více
+nav_top: Zpět nahoru
+
+form_placeholder_search: Vyhledat
+
+error_page_title: Ztracen? Nic se neděje
+error_message_title: Ztracen?
+error_message_code: Error 404
+error_message_text: >
+ Vypadá to že stránka, kterou hledáte, neexistuje. Nemějte obavy, můžete
+ se vrátit zpět na domovskou stránku.
+
+button_toggle_dark: Přepnout tmavý/světlý/automatický režim
+button_nav_open: Otevřít navigaci
+button_nav_close: Zavřít navigaci
+button_menu_open: Otevřít lištu nabídky
+button_menu_close: Zavřít lištu nabídky
+button_homepage: Zpět na domovskou stránku
+
+title_anchor_prefix: "Odkaz na:"
+
+posts_read_more: Přečíst celý příspěvek
+posts_read_time:
+ one: "Doba čtení: 1 minuta"
+ other: "Doba čtení: {{ . }} minut(y)"
+posts_update_prefix: Naposledy upraveno
+posts_count:
+ one: "Jeden příspěvek"
+ other: "Příspěvků: {{ . }}"
+posts_tagged_with: Všechny příspěvky označeny '{{ . }}'
+
+footer_build_with: >
+ Vytvořeno za pomocí Hugo a
+
+footer_legal_notice: Právní upozornění
+footer_privacy_policy: Zásady ochrany soukromí
+footer_content_license_prefix: >
+ Obsah licencovaný pod
+
+language_switch_no_tranlation_prefix: "Stránka není přeložena:"
+
+propertylist_required: povinné
+propertylist_optional: volitené
+propertylist_default: výchozí
+
+pagination_page_prev: předchozí
+pagination_page_next: další
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/i18n/de.yaml b/docs/themes/hugo-geekdoc/i18n/de.yaml
new file mode 100644
index 0000000..ae3dc99
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/de.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: Seite bearbeiten
+
+nav_navigation: Navigation
+nav_tags: Tags
+nav_more: Weitere
+nav_top: Nach oben
+
+form_placeholder_search: Suchen
+
+error_page_title: Verlaufen? Keine Sorge
+error_message_title: Verlaufen?
+error_message_code: Fehler 404
+error_message_text: >
+ Wir können die Seite nach der Du gesucht hast leider nicht finden. Keine Sorge,
+ wir bringen Dich zurück zur Startseite.
+
+button_toggle_dark: Wechsel zwischen Dunkel/Hell/Auto Modus
+button_nav_open: Navigation öffnen
+button_nav_close: Navigation schließen
+button_menu_open: Menüband öffnen
+button_menu_close: Menüband schließen
+button_homepage: Zurück zur Startseite
+
+title_anchor_prefix: "Link zu:"
+
+posts_read_more: Ganzen Artikel lesen
+posts_read_time:
+ one: "Eine Minute Lesedauer"
+ other: "{{ . }} Minuten Lesedauer"
+posts_update_prefix: Aktualisiert am
+posts_count:
+ one: "Ein Artikel"
+ other: "{{ . }} Artikel"
+posts_tagged_with: Alle Artikel mit dem Tag '{{ . }}'
+
+footer_build_with: >
+ Entwickelt mit Hugo und
+
+footer_legal_notice: Impressum
+footer_privacy_policy: Datenschutzerklärung
+footer_content_license_prefix: >
+ Inhalt lizensiert unter
+
+language_switch_no_tranlation_prefix: "Seite nicht übersetzt:"
+
+propertylist_required: erforderlich
+propertylist_optional: optional
+propertylist_default: Standardwert
+
+pagination_page_prev: vorher
+pagination_page_next: weiter
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/i18n/en.yaml b/docs/themes/hugo-geekdoc/i18n/en.yaml
new file mode 100644
index 0000000..ff19ea4
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/en.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: Edit page
+
+nav_navigation: Navigation
+nav_tags: Tags
+nav_more: More
+nav_top: Back to top
+
+form_placeholder_search: Search
+
+error_page_title: Lost? Don't worry
+error_message_title: Lost?
+error_message_code: Error 404
+error_message_text: >
+ Seems like what you are looking for can't be found. Don't worry, we can
+ bring you back to the homepage.
+
+button_toggle_dark: Toggle Dark/Light/Auto mode
+button_nav_open: Open Navigation
+button_nav_close: Close Navigation
+button_menu_open: Open Menu Bar
+button_menu_close: Close Menu Bar
+button_homepage: Back to homepage
+
+title_anchor_prefix: "Anchor to:"
+
+posts_read_more: Read full post
+posts_read_time:
+ one: "One minute to read"
+ other: "{{ . }} minutes to read"
+posts_update_prefix: Updated on
+posts_count:
+ one: "One post"
+ other: "{{ . }} posts"
+posts_tagged_with: All posts tagged with '{{ . }}'
+
+footer_build_with: >
+ Built with Hugo and
+
+footer_legal_notice: Legal Notice
+footer_privacy_policy: Privacy Policy
+footer_content_license_prefix: >
+ Content licensed under
+
+language_switch_no_tranlation_prefix: "Page not translated:"
+
+propertylist_required: required
+propertylist_optional: optional
+propertylist_default: default
+
+pagination_page_prev: prev
+pagination_page_next: next
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/i18n/es.yaml b/docs/themes/hugo-geekdoc/i18n/es.yaml
new file mode 100644
index 0000000..8e65cec
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/es.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: Editar página
+
+nav_navigation: Navegación
+nav_tags: Etiquetas
+nav_more: Más
+nav_top: Inicio de la página
+
+form_placeholder_search: Buscar
+
+error_page_title: Perdido? No te preocupes
+error_message_title: Perdido?
+error_message_code: Error 404
+error_message_text: >
+ Al parecer, lo que estás buscando no pudo ser encontrado. No te preocupes, podemos
+ llevarte de vuelta al inicio.
+
+button_toggle_dark: Cambiar el modo Oscuro/Claro/Auto
+button_nav_open: Abrir la Navegación
+button_nav_close: Cerrar la Navegación
+button_menu_open: Abrir el Menú Bar
+button_menu_close: Cerrar el Menú Bar
+button_homepage: Volver al Inicio
+
+title_anchor_prefix: "Anclado a:"
+
+posts_read_more: Lee la publicación completa
+posts_read_time:
+ one: "Un minuto para leer"
+ other: "{{ . }} minutos para leer"
+posts_update_prefix: Actualizado en
+posts_count:
+ one: "Una publicación"
+ other: "{{ . }} publicaciones"
+posts_tagged_with: Todas las publicaciones etiquetadas con '{{ . }}'
+
+footer_build_with: >
+ Creado con Hugo y
+
+footer_legal_notice: Aviso Legal
+footer_privacy_policy: Política de Privacidad
+footer_content_license_prefix: >
+ Contenido licenciado con
+
+language_switch_no_tranlation_prefix: "Página no traducida:"
+
+propertylist_required: requerido
+propertylist_optional: opcional
+propertylist_default: estándar
+
+pagination_page_prev: previo
+pagination_page_next: siguiente
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/i18n/it.yaml b/docs/themes/hugo-geekdoc/i18n/it.yaml
new file mode 100644
index 0000000..ce7c40b
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/it.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: Modifica la pagina
+
+nav_navigation: Navigazione
+nav_tags: Etichette
+nav_more: Altro
+nav_top: Torna su
+
+form_placeholder_search: Cerca
+
+error_page_title: Perso? Non ti preoccupare
+error_message_title: Perso?
+error_message_code: Errore 404
+error_message_text: >
+ Sembra che non sia possibile trovare quello che stavi cercando. Non ti preoccupare,
+ possiamo riportarti alla pagina iniziale.
+
+button_toggle_dark: Seleziona il tema Chiaro/Scuro/Automatico
+button_nav_open: Apri la Navigazione
+button_nav_close: Chiudi la Navigazione
+button_menu_open: Apri la Barra del Menu
+button_menu_close: Chiudi la Barra del Menu
+button_homepage: Torna alla pagina iniziale
+
+title_anchor_prefix: "Ancora a:"
+
+posts_read_more: Leggi tutto il post
+posts_read_time:
+ one: "Tempo di lettura: un minuto"
+ other: "Tempo di lettura: {{ . }} minuti"
+posts_update_prefix: Aggiornato il
+posts_count:
+ one: "Un post"
+ other: "{{ . }} post"
+posts_tagged_with: Tutti i post etichettati con '{{ . }}'
+
+footer_build_with: >
+ Realizzato con Hugo e
+
+footer_legal_notice: Avviso Legale
+footer_privacy_policy: Politica sulla Privacy
+footer_content_license_prefix: >
+ Contenuto sotto licenza
+
+language_switch_no_tranlation_prefix: "Pagina non tradotta:"
+
+propertylist_required: richiesto
+propertylist_optional: opzionale
+propertylist_default: valore predefinito
+
+pagination_page_prev: precedente
+pagination_page_next: prossimo
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/i18n/ja.yaml b/docs/themes/hugo-geekdoc/i18n/ja.yaml
new file mode 100644
index 0000000..506e7b4
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/ja.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: ページの編集
+
+nav_navigation: ナビゲーション
+nav_tags: タグ
+nav_more: さらに
+nav_top: トップへ戻る
+
+form_placeholder_search: 検索
+
+error_page_title: お困りですか?ご心配なく
+error_message_title: お困りですか?
+error_message_code: 404 エラー
+error_message_text: >
+ お探しのものが見つからないようです。トップページ
+ へ戻ることができるので、ご安心ください。
+
+button_toggle_dark: モードの切替 ダーク/ライト/自動
+button_nav_open: ナビゲーションを開く
+button_nav_close: ナビゲーションを閉じる
+button_menu_open: メニューバーを開く
+button_menu_close: メニューバーを閉じる
+button_homepage: トップページへ戻る
+
+title_anchor_prefix: "アンカー先:"
+
+posts_read_more: 全投稿を閲覧
+posts_read_time:
+ one: "読むのに 1 分かかります"
+ other: "読むのに要する時間 {{ . }} (分)"
+posts_update_prefix: 更新時刻
+posts_count:
+ one: "一件の投稿"
+ other: "{{ . }} 件の投稿"
+posts_tagged_with: "'{{ . }}'のタグが付いた記事全部"
+
+footer_build_with: >
+ Hugo でビルドしています。
+
+footer_legal_notice: 法的な告知事項
+footer_privacy_policy: プライバシーポリシー
+footer_content_license_prefix: >
+ 提供するコンテンツのライセンス
+
+language_switch_no_tranlation_prefix: "未翻訳のページ:"
+
+propertylist_required: 必須
+propertylist_optional: 任意
+propertylist_default: 既定値
+
+pagination_page_prev: 前
+pagination_page_next: 次
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/i18n/nl.yaml b/docs/themes/hugo-geekdoc/i18n/nl.yaml
new file mode 100644
index 0000000..8e24d62
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/nl.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: Wijzig pagina
+
+nav_navigation: Navigatie
+nav_tags: Markering
+nav_more: Meer
+nav_top: Terug naar boven
+
+form_placeholder_search: Zoek
+
+error_page_title: Verdwaald? Geen probleem
+error_message_title: Verdwaald?
+error_message_code: Error 404
+error_message_text: >
+ Het lijkt er op dat wat je zoekt niet gevonden kan worden. Geen probleem,
+ we kunnen je terug naar de startpagina brengen.
+
+button_toggle_dark: Wijzig Donker/Licht/Auto weergave
+button_nav_open: Open navigatie
+button_nav_close: Sluit navigatie
+button_menu_open: Open menubalk
+button_menu_close: Sluit menubalk
+button_homepage: Terug naar startpagina
+
+title_anchor_prefix: "Link naar:"
+
+posts_read_more: Lees volledige bericht
+posts_read_time:
+ one: "Een minuut leestijd"
+ other: "{{ . }} minuten leestijd"
+posts_update_prefix: Bijgewerkt op
+posts_count:
+ one: "Een bericht"
+ other: "{{ . }} berichten"
+posts_tagged_with: Alle berichten gemarkeerd met '{{ . }}'
+
+footer_build_with: >
+ Gebouwd met Hugo en
+
+footer_legal_notice: Juridische mededeling
+footer_privacy_policy: Privacybeleid
+footer_content_license_prefix: >
+ Inhoud gelicenseerd onder
+
+language_switch_no_tranlation_prefix: "Pagina niet vertaald:"
+
+propertylist_required: verplicht
+propertylist_optional: optioneel
+propertylist_default: standaard
+
+pagination_page_prev: vorige
+pagination_page_next: volgende
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/i18n/zh-cn.yaml b/docs/themes/hugo-geekdoc/i18n/zh-cn.yaml
new file mode 100644
index 0000000..e6403ac
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/i18n/zh-cn.yaml
@@ -0,0 +1,53 @@
+---
+edit_page: 编辑页面
+
+nav_navigation: 导航
+nav_tags: 标签
+nav_more: 更多
+nav_top: 回到顶部
+
+form_placeholder_search: 搜索
+
+error_page_title: 迷路了? 不用担心
+error_message_title: 迷路了?
+error_message_code: 错误 404
+error_message_text: >
+ 好像找不到你要找的东西。 别担心,我们可以
+ 带您回到主页。
+
+button_toggle_dark: 切换暗/亮/自动模式
+button_nav_open: 打开导航
+button_nav_close: 关闭导航
+button_menu_open: 打开菜单栏
+button_menu_close: 关闭菜单栏
+button_homepage: 返回首页
+
+title_anchor_prefix: "锚定到:"
+
+posts_read_more: 阅读全文
+posts_read_time:
+ one: "一分钟阅读时间"
+ other: "{{ . }} 分钟阅读时间"
+posts_update_prefix: 更新时间
+posts_count:
+ one: 一篇文章
+ other: "{{ . }} 个帖子"
+posts_tagged_with: 所有带有“{{ . }}”标签的帖子。
+
+footer_build_with: >
+ 基于 Hugo
+ 制作
+footer_legal_notice: "法律声明"
+footer_privacy_policy: "隐私政策"
+footer_content_license_prefix: >
+ 内容许可证
+
+language_switch_no_tranlation_prefix: "页面未翻译:"
+
+propertylist_required: 需要
+propertylist_optional: 可选
+propertylist_default: 默认值
+
+pagination_page_prev: 以前
+pagination_page_next: 下一个
+pagination_page_state: "{{ .PageNumber }}/{{ .TotalPages }}"
diff --git a/docs/themes/hugo-geekdoc/images/readme.png b/docs/themes/hugo-geekdoc/images/readme.png
new file mode 100644
index 0000000..10c8ff1
Binary files /dev/null and b/docs/themes/hugo-geekdoc/images/readme.png differ
diff --git a/docs/themes/hugo-geekdoc/images/screenshot.png b/docs/themes/hugo-geekdoc/images/screenshot.png
new file mode 100644
index 0000000..af24360
Binary files /dev/null and b/docs/themes/hugo-geekdoc/images/screenshot.png differ
diff --git a/docs/themes/hugo-geekdoc/images/tn.png b/docs/themes/hugo-geekdoc/images/tn.png
new file mode 100644
index 0000000..ee6e42e
Binary files /dev/null and b/docs/themes/hugo-geekdoc/images/tn.png differ
diff --git a/docs/themes/hugo-geekdoc/layouts/404.html b/docs/themes/hugo-geekdoc/layouts/404.html
new file mode 100644
index 0000000..f8a61bb
--- /dev/null
+++ b/docs/themes/hugo-geekdoc/layouts/404.html
@@ -0,0 +1,40 @@
+
+
+
+ {{ partial "head/meta" . }}
+ {{ i18n "error_page_title" }}
+
+ {{ partial "head/favicons" . }}
+ {{ partial "head/others" . }}
+
+
+
+ {{ partial "svg-icon-symbols" . }}
+
+
+