diff --git a/.gitignore b/.gitignore
index 6a728c8..232f438 100644
--- a/.gitignore
+++ b/.gitignore
@@ -41,3 +41,4 @@ media/*
.mr.developer.cfg
.project
.pydevproject
+venv
diff --git a/Makefile b/Makefile
new file mode 100644
index 0000000..a65a7bc
--- /dev/null
+++ b/Makefile
@@ -0,0 +1,32 @@
+.PHONY: test pep8 clean install build publish tree reenv
+
+test: pep8
+ py.test --cov=flask_googlemaps -l --tb=short --maxfail=1 tests/
+
+pep8:
+ @flake8 flask_googlemaps --ignore=F403
+
+clean:
+ @find ./ -name '*.pyc' -exec rm -f {} \;
+ @find ./ -name 'Thumbs.db' -exec rm -f {} \;
+ @find ./ -name '*~' -exec rm -f {} \;
+ @rm -rf dist/
+ @rm -rf *.egg
+ @rm -rf *.egg-info
+
+install:
+ @pip install flit pypandoc pygments
+ @flit install -s
+
+build:
+ @flit build
+
+publish:
+ @flit publish
+
+tree:
+ @tree -L 1 -a -I __pycache__ --dirsfirst --noreport
+
+reenv:
+ @rm -rf venv
+ @python3.6 -m venv venv
diff --git a/README.md b/README.md
index 07f4068..369e12f 100644
--- a/README.md
+++ b/README.md
@@ -7,35 +7,38 @@ Easy to use Google Maps in your Flask application
Look the Live DEMO: http://flaskgooglemaps.pythonanywhere.com
-### requires
+## requires
+
- Jinja
- Flask
- A google api key [get here](https://developers.google.com/maps/documentation/javascript/get-api-key)
-### Installation
+## Installation
```pip install flask-googlemaps```
or
```bash
+
git clone https://github.com/rochacbruno/Flask-GoogleMaps
cd Flask-GoogleMaps
python setup.py install
-```
+```
-### How it works
+## How it works
Flask-GoogleMaps includes some global functions and template filters in your Jinja environment, also it allows you to use the Map in views if needed.
-#### registering
+### registering
in your app
```python
+
from flask import Flask
from flask_googlemaps import GoogleMaps
@@ -55,7 +58,9 @@ GoogleMaps(app, key="8JZ7i18MjFuM35dJHq70n3Hx4")
In template
```html
+
{{googlemap("my_awesome_map", lat=0.23234234, lng=-0.234234234, markers=[(0.12, -0.45345), ...])}}
+
```
That's it! now you have some template filters and functions to use, more details in examples and screenshot below.
@@ -71,6 +76,7 @@ That's it! now you have some template filters and functions to use, more details
#### 1. View
```python
+
from flask import Flask, render_template
from flask_googlemaps import GoogleMaps
from flask_googlemaps import Map
@@ -110,9 +116,10 @@ def mapview():
if __name__ == "__main__":
app.run(debug=True)
+
```
-##### `Map()` Parameters:
+##### `Map()` Parameters
- **lat**: The latitude coordinate for centering the map.
- **lng**: The longitutde coordinate for centering the map.
@@ -143,6 +150,7 @@ Also controls True or False:
#### 2. Template
```html
+
@@ -159,7 +167,6 @@ Also controls True or False:
Template filter decoupled with single marker
{{"decoupled-map"|googlemap_html(37.4419, -122.1419)}}
-
Template function with multiple markers
{% with map=googlemap_obj("another-map", 37.4419, -122.1419, markers=[(37.4419, -122.1419), (37.4300, -122.1400)]) %}
{{map.html}}
@@ -180,8 +187,9 @@ Also controls True or False:
### Infobox
-Here's an example snippet of code:
+Here's an example snippet of code:
```python
+
Map(
identifier="catsmap",
lat=37.4419,
@@ -213,11 +221,15 @@ Here's an example snippet of code:
Which results in something like the following map:
+
### Fit all markers within bounds
+
Allow users to easily fit all markers within view on page load
#### Without bounds
+
```python
+
@app.route('/map-unbounded/')
def map_unbounded():
"""Create map with markers out of bounds."""
@@ -228,11 +240,15 @@ def map_unbounded():
markers=[(loc.latitude, loc.longitude) for loc in locations]
)
return render_template('map.html', map=map)
+
```
+
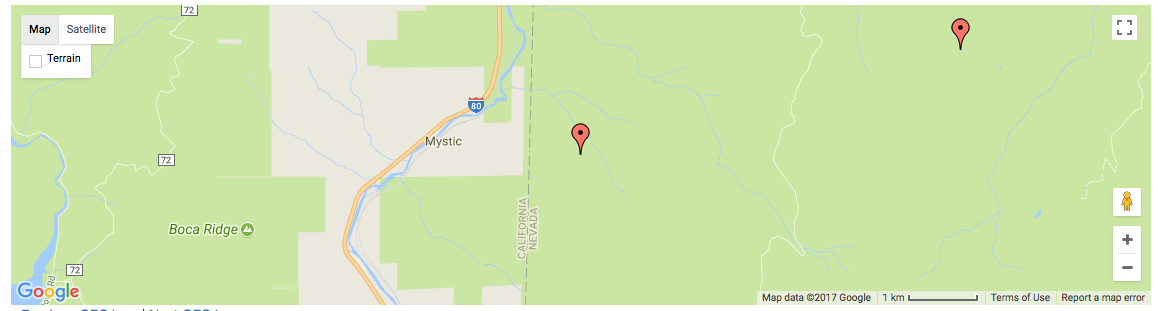
#### With bounds
+
```python
+
@app.route('/map-bounded/')
def map_bounded():
"""Create map with all markers within bounds."""
@@ -244,22 +260,26 @@ def map_bounded():
fit_markers_to_bounds = True
)
return render_template('map.html', map=map)
+
```
+
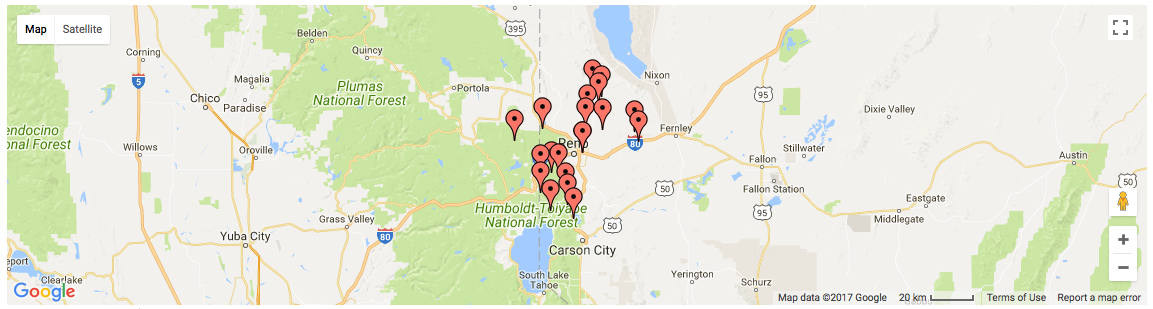
### Run the example app
```bash
+
$ git clone https://github.com/rochacbruno/Flask-GoogleMaps
$ cd Flask-GoogleMaps/examples
$ python setup.py develop
$ python example.py
+running..
```
Access: http://localhost:5000/ and http://localhost:5000/fullmap
-### TODO (open a Pull Request):
+### TODO (open a Pull Request)
Implement other methods from the api, add layers etc...
diff --git a/flask_googlemaps/__init__.py b/flask_googlemaps/__init__.py
index d2f6303..fd64bb4 100644
--- a/flask_googlemaps/__init__.py
+++ b/flask_googlemaps/__init__.py
@@ -1,4 +1,6 @@
-# coding: utf-8
+"""FlaskGoogleMaps - Google Maps Extension for Flask"""
+
+__version__ = '0.2.5'
from flask import render_template, Blueprint, Markup, g
from flask_googlemaps.icons import dots
diff --git a/flit.ini b/flit.ini
new file mode 100644
index 0000000..9ba3822
--- /dev/null
+++ b/flit.ini
@@ -0,0 +1,18 @@
+[metadata]
+module = flask_googlemaps
+dist-name = Flask-GoogleMaps
+author = Bruno Rocha
+author-email = rochacbruno@gmail.com
+maintainer = rochacbruno
+maintainer-email = rochacbruno@gmail.com
+home-page = https://github.com/rochacbruno/Flask-GoogleMaps/
+requires = flask
+description-file = README.md
+classifiers = Programming Language :: Python
+ Programming Language :: Python :: 2.7
+ Programming Language :: Python :: 3.6
+ Intended Audience :: Developers
+ License :: OSI Approved :: MIT License
+ Framework :: Flask
+ Topic :: Internet :: WWW/HTTP :: Dynamic Content
+ Topic :: Software Development :: Libraries :: Python Modules
diff --git a/setup.py b/setup.py
index a9ed971..4835f4e 100644
--- a/setup.py
+++ b/setup.py
@@ -4,7 +4,7 @@
setup(
name='Flask-GoogleMaps',
- version='0.2.4',
+ version='0.2.5',
license='MIT',
description='Small extension for Flask to make using Google Maps easy',
long_description=open('README.md').read(),