diff --git a/jsk_fetch_robot/README.md b/jsk_fetch_robot/README.md
index 9aab1b786d..9a838c5d6f 100644
--- a/jsk_fetch_robot/README.md
+++ b/jsk_fetch_robot/README.md
@@ -33,22 +33,21 @@
## How to Run
+### Setup Environment (For Remote PC)
-### Setup Environment
-
-First, you need to install ros. For ros indigo, please refer to install guide like [here](http://wiki.ros.org/indigo/Installation/Ubuntu)
+First, you need to install ROS. For ROS melodic, please refer to install guide like [here](http://wiki.ros.org/melodic/Installation/Ubuntu).
+Please make sure your ROS Distribution is indigo, kinetic or melodic.
```bash
mkdir -p catkin_ws/src
cd catkin_ws/src
wstool init .
wstool set --git jsk-ros-pkg/jsk_robot https://github.com/jsk-ros-pkg/jsk_robot.git -y
-if [[ $ROS_DISTRO =~ ^(indigo|kinetic|melodic)$ ]]; then
- wstool merge -t . https://raw.githubusercontent.com/jsk-ros-pkg/jsk_robot/master/jsk_fetch_robot/jsk_fetch_user.rosinstall.$ROS_DISTRO
-else
- echo "Your ROS distribution $ROS_DISTRO is not supported."
-fi
+wstool merge -t . https://raw.githubusercontent.com/jsk-ros-pkg/jsk_robot/master/jsk_fetch_robot/jsk_fetch_user.rosinstall.$ROS_DISTRO
wstool update -t .
+# To use eus10, furuschev script is required.
+wget https://raw.githubusercontent.com/jsk-ros-pkg/jsk_roseus/master/setup_upstream.sh -O /tmp/setup_upstream.sh
+bash /tmp/setup_upstream.sh -w ../ -p jsk-ros-pkg/geneus -p euslisp/jskeus
source /opt/ros/$ROS_DISTRO/setup.bash
rosdep install -y -r --from-paths . --ignore-src
cd ../
@@ -56,6 +55,23 @@ catkin build fetcheus jsk_fetch_startup
source devel/setup.bash
```
+#### Setup Environment (For Robot Internal PC, only for advanced developer)
+
+```bash
+mkdir -p catkin_ws/src
+cd catkin_ws/src
+wstool init .
+wstool set --git jsk-ros-pkg/jsk_robot https://github.com/knorth55/jsk_robot.git -v fetch15 -y
+wstool update -t .
+wstool merge -t . jsk-ros-pkg/jsk_robot/jsk_fetch_robot/jsk_fetch.rosinstall.$ROS_DISTRO
+wstool update -t .
+source /opt/ros/$ROS_DISTRO/setup.bash
+rosdep install -y -r --from-paths . --ignore-src
+cd ../
+catkin build
+source devel/setup.bash
+```
+
### Connecting to Fetch
You need to install `ros-indigo-jsk-tools` to use `rosset*` tools, otherwise use setenv command
diff --git a/jsk_fetch_robot/fetcheus/fetch-interface.l b/jsk_fetch_robot/fetcheus/fetch-interface.l
index f90a4fd1be..57d64fb419 100644
--- a/jsk_fetch_robot/fetcheus/fetch-interface.l
+++ b/jsk_fetch_robot/fetcheus/fetch-interface.l
@@ -1,6 +1,7 @@
(require "package://fetcheus/fetch-utils.l")
(require "package://pr2eus/robot-interface.l")
(require "package://pr2eus_moveit/euslisp/robot-moveit.l")
+(require "package://roseus_resume/euslisp/interruption-handler.l")
(ros::load-ros-package "fetcheus")
(ros::load-ros-package "fetch_driver_msgs")
@@ -8,11 +9,11 @@
(defclass fetch-interface
:super robot-move-base-interface
- :slots (gripper-action moveit-robot fetch-controller-action)
+ :slots (gripper-action moveit-robot fetch-controller-action point-head-action)
)
(defmethod fetch-interface
- (:init (&key (default-collision-object t) &rest args)
+ (:init (&rest args &key (default-collision-object t) &allow-other-keys)
(prog1 (send-super* :init :robot fetch-robot :base-frame-id "base_link" :odom-topic "/odom_combined" :base-controller-action-name nil args)
(send self :add-controller :arm-controller)
(send self :add-controller :torso-controller)
@@ -29,17 +30,24 @@
(setq moveit-robot (instance fetch-robot :init))
(send self :set-moveit-environment (instance fetch-moveit-environment :init :robot moveit-robot))
(when (and (boundp '*co*) default-collision-object)
- (send self :delete-headbox-collision-object)
(send self :delete-keepout-collision-object)
(send self :delete-ground-collision-object)
- (send self :add-headbox-collision-object)
(send self :add-keepout-collision-object)
(send self :add-ground-collision-object))
(setq fetch-controller-action
(instance ros::simple-action-client :init
"/query_controller_states"
robot_controllers_msgs::QueryControllerStatesAction))
- ))
+ (setq point-head-action
+ (instance ros::simple-action-client :init
+ "/head_controller/point_head"
+ control_msgs::PointHeadAction))
+
+ (roseus_resume:install-interruption-handler self
+ gripper-action
+ move-base-action
+ move-base-trajectory-action)
+ (roseus_resume:install-default-intervention self)))
(:state (&rest args)
"We do not have :wait-until-update option for :state :worldcoords.
In other cases, :state calls with :wait-until-update by default, since Fetch publishes /joint_states from body and gripper at almost same frequency.
@@ -117,8 +125,8 @@ Example usage:
(subseq args (+ (position :use-torso args) 2))))))
(return-from :angle-vector (send* self :angle-vector-raw av tm ctype start-time args)))
;;
- (when (not (numberp tm))
- (ros::warn ":angle-vector tm is not a number, use :angle-vector av tm args"))
+ (when (and (not (numberp tm)) (not (eql tm :fast)))
+ (ros::warn ":angle-vector tm is not a number, use :angle-vector av tm args~%"))
(send* self :angle-vector-motion-plan av :ctype ctype :move-arm :rarm :total-time tm
:start-offset-time (if start-offset-time start-offset-time start-time)
:clear-velocities clear-velocities :use-torso use-torso args)))
@@ -191,6 +199,17 @@ Example usage:
(:stop-grasp
(&rest args &key &allow-other-keys)
(send* self :go-grasp :pos 0.1 args))
+ (:point-head (pos &key (frame-id "base_link") (wait t))
+ (let ((goal (instance control_msgs::PointHeadGoal :init)))
+ (send goal :target :header :stamp (ros::time-now))
+ (send goal :target :header :frame_id frame-id)
+ (send goal :target :point (ros::pos->tf-point pos))
+ (if wait
+ (send point-head-action :send-goal goal)
+ (send point-head-action :send-goal-and-wait goal)
+ )
+ )
+ )
(:go-grasp
(&key (pos 0) (effort 50) (wait t))
(when (send self :simulation-modep)
@@ -243,18 +262,6 @@ Example: (send self :gripper :position) => 0.00"
(:delete-workspace ()
(send *co* :delete-attached-object-by-id "workspace")
(send *co* :delete-object-by-id "workspace"))
- (:add-headbox-collision-object ()
- (let ()
- ;; fetch must be :reset-pose when we run this method
- (setq *fetch-headbox* (make-cube 100 201 120))
- (send *fetch-headbox* :move-coords (send robot :head_pan_link_lk :worldcoords)
- (send robot :base_link_lk :worldcoords))
- (send *fetch-headbox* :translate #f(33.75 0 150) (send robot :head_pan_link_lk :worldcoords))
- (send *co* :add-attached-object *fetch-headbox* "head_pan_link"
- :frame_id "head_pan_link"
- :object_id "fetchheadbox")))
- (:delete-headbox-collision-object ()
- (send *co* :delete-attached-object-by-id "fetchheadbox"))
(:add-keepout-collision-object ()
(let ((cube (make-cube 200 350 10))
(keepout (make-cylinder 300 10)))
diff --git a/jsk_fetch_robot/fetcheus/fetch-utils.l b/jsk_fetch_robot/fetcheus/fetch-utils.l
index ea4a1d27e2..67932a47fe 100644
--- a/jsk_fetch_robot/fetcheus/fetch-utils.l
+++ b/jsk_fetch_robot/fetcheus/fetch-utils.l
@@ -5,16 +5,40 @@
(defmethod fetch-robot
(:inverse-kinematics
- (target-coords &rest args &key link-list move-arm (use-torso t) move-target &allow-other-keys)
+ (target-coords &rest args &key link-list move-arm (use-torso t)
+ use-base (start-coords (send self :copy-worldcoords))
+ (base-range (list :min #f(-30 -30 -30)
+ :max #f( 30 30 30)))
+ move-target &allow-other-keys)
(unless move-arm (setq move-arm :rarm))
(unless move-target (setq move-target (send self :rarm :end-coords)))
(unless link-list
(setq link-list (send self :link-list (send move-target :parent)
(unless use-torso (car (send self :rarm))))))
- (send-super* :inverse-kinematics target-coords
- :move-target move-target
- :link-list link-list
- args))
+ (cond
+ (use-base
+ (let ((diff-pos-rot
+ (concatenate float-vector
+ (send start-coords :difference-position self)
+ (send start-coords :difference-rotation self))))
+ (send self :move-to start-coords :world)
+ (with-append-root-joint
+ (ll self link-list
+ :joint-class omniwheel-joint
+ :joint-args base-range)
+ (send (caar ll) :joint :joint-angle
+ (float-vector (elt diff-pos-rot 0)
+ (elt diff-pos-rot 1)
+ (rad2deg (elt diff-pos-rot 5))))
+ (send-super* :inverse-kinematics target-coords
+ :move-target move-target
+ :link-list ll ;; link-list
+ args))))
+ (t
+ (send-super* :inverse-kinematics target-coords
+ :move-target move-target
+ :link-list link-list
+ args))))
(:go-grasp
(&key (pos 0)) ;; pos is between 0.0 and 0.1
(send self :l_gripper_finger_joint :joint-angle (/ (* pos 1000) 2)) ;; m -> mm
diff --git a/jsk_fetch_robot/jsk_fetch.rosinstall.indigo b/jsk_fetch_robot/jsk_fetch.rosinstall.indigo
index bae0cdd09b..189d65d616 100644
--- a/jsk_fetch_robot/jsk_fetch.rosinstall.indigo
+++ b/jsk_fetch_robot/jsk_fetch.rosinstall.indigo
@@ -4,29 +4,32 @@
- git:
local-name: PR2/app_manager
uri: https://github.com/PR2/app_manager.git
- version: 1.1.0
-# For fetch to use twitter
+ version: kinetic-devel
- git:
- local-name: furushchev/image_pipeline
- uri: https://github.com/furushchev/image_pipeline.git
- version: develop
-# To send lifelog data to musca
+ local-name: RobotWebTools/rosbridge_suite
+ uri: https://github.com/RobotWebTools/rosbridge_suite.git
+ version: 0.11.9
- git:
- local-name: strands-project/mongodb_store
- uri: https://github.com/strands-project/mongodb_store.git
- version: 0.4.4
-# to install jsk_robot_startup/lifelog/common_logger.launch
-# remove after current master is released
+ local-name: RoboticMaterials/FA-I-sensor
+ uri: https://github.com/RoboticMaterials/FA-I-sensor.git
+- git:
+ local-name: fetchrobotics/fetch_ros
+ uri: https://github.com/fetchrobotics/fetch_ros.git
+ version: indigo-devel
- git:
- local-name: jsk-ros-pkg/jsk_robot
- uri: https://github.com/jsk-ros-pkg/jsk_robot.git
+ local-name: fetchrobotics/robot_controllers
+ uri: https://github.com/fetchrobotics/robot_controllers.git
+ version: indigo-devel
+- git:
+ local-name: jsk-ros-pkg/jsk_3rdparty
+ uri: https://github.com/jsk-ros-pkg/jsk_3rdparty.git
version: master
# to pass build of jsk_robot
-# remove after 2.2.10 is released
+# remove after 2.2.11 is released
- git:
local-name: jsk-ros-pkg/jsk_common
uri: https://github.com/jsk-ros-pkg/jsk_common.git
- version: 2.2.10
+ version: 799fd309c1519801fcb3a37c9094814004d78594
# to avoid volume 0 problem
# remove after 0.3.14 (https://github.com/jsk-ros-pkg/jsk_pr2eus/commit/41183fe3401d742bbec0edd13b67cb909a6968bd) is released
- git:
@@ -39,7 +42,12 @@
- git:
local-name: jsk-ros-pkg/jsk_demos
uri: https://github.com/jsk-ros-pkg/jsk_demos.git
- version: 810acc7
+ version: 7c429715d0adf12c5dd34459ceb4b0a5b11dec6c
+# need to build from source because of nodelet version
+- git:
+ local-name: jsk-ros-pkg/jsk_recognition
+ uri: https://github.com/jsk-ros-pkg/jsk_recognition.git
+ version: acb7e7dc5b549f8663dec403abdb80cbac36edf1
# jsk_topic_tools requires nodelet gte 1.9.11
# remove after 1.9.11 is released by apt
- git:
@@ -51,20 +59,20 @@
- git:
local-name: ros-drivers/audio_common
uri: https://github.com/ros-drivers/audio_common.git
- version: 0.3.3
+ version: master
# to install nodelet_plugins.xml
# remove after 1.3.10 is released by apt
- git:
local-name: ros-perception/slam_gmapping
uri: https://github.com/ros-perception/slam_gmapping.git
version: 1.3.10
-# indigo is already EOL and 2.1.13 is never released.
+# indigo is already EOL and 2.1.15 is never released.
# set the same version as https://github.com/jsk-ros-pkg/jsk_robot/blob/master/.travis.rosinstall.indigo#L7-L11
-# change to 2.1.14 when it is released.
+# change to 2.1.15 when it is released.
- git:
local-name: jsk-ros-pkg/jsk_3rdparty
uri: https://github.com/jsk-ros-pkg/jsk_3rdparty.git
- version: 82e897dcbdcd6aa0cbd126fa122d4dbdc9df67c9
+ version: ffbd7b0592d7dd2f830f185636a1dd1695d8cb44
# Use joy/joy_remap.py
- git:
local-name: ros-drivers/joystick_drivers
@@ -103,3 +111,12 @@
local-name: fetchrobotics/fetch_open_auto_dock
uri: https://github.com/fetchrobotics/fetch_open_auto_dock.git
version: 0.1.2
+# indigo is already EOL and visualization_msgs is never released
+- tar:
+ local-name: ros/common_msgs/visualization_msgs
+ uri: https://github.com/ros-gbp/common_msgs-release/archive/release/kinetic/visualization_msgs/1.12.7-0.tar.gz
+ version: common_msgs-release-release-kinetic-visualization_msgs-1.12.7-0
+- git:
+ local-name: locusrobotics/catkin_virtualenv
+ uri: https://github.com/locusrobotics/catkin_virtualenv.git
+ version: 0.5.0
diff --git a/jsk_fetch_robot/jsk_fetch.rosinstall.melodic b/jsk_fetch_robot/jsk_fetch.rosinstall.melodic
index 22b0bb9cb6..11cf98a74e 100644
--- a/jsk_fetch_robot/jsk_fetch.rosinstall.melodic
+++ b/jsk_fetch_robot/jsk_fetch.rosinstall.melodic
@@ -1,10 +1,9 @@
# This is rosinstall file for melodic PC inside fetch.
# $ ln -s $(rospack find jsk_fetch_startup)/../jsk_fetch.rosinstall.$ROS_DISTRO $HOME/ros/$ROS_DISTRO/src/.rosinstall
-
- git:
local-name: PR2/app_manager
- uri: https://github.com/PR2/app_manager.git
- version: kinetic-devel
+ uri: https://github.com/knorth55/app_manager.git
+ version: fetch15
- git:
local-name: RobotWebTools/rosbridge_suite
uri: https://github.com/RobotWebTools/rosbridge_suite.git
@@ -26,8 +25,14 @@
local-name: jsk-ros-pkg/jsk_3rdparty
uri: https://github.com/knorth55/jsk_3rdparty.git
version: fetch15
-# we need to use the development branch (fetch15 branch in knorth55's fork)
-# until it is merged to master
+# we need to use the development branch until PR below are merged.
+# - https://github.com/jsk-ros-pkg/jsk_common/pull/1719
+# - https://github.com/jsk-ros-pkg/jsk_common/pull/1746
+# Waiting 2.2.12 release
+# - git:
+# local-name: jsk-ros-pkg/jsk_common
+# uri: https://github.com/jsk-ros-pkg/jsk_common.git
+# version: master
- git:
local-name: jsk-ros-pkg/jsk_common
uri: https://github.com/knorth55/jsk_common.git
@@ -42,16 +47,17 @@
local-name: jsk-ros-pkg/jsk_pr2eus
uri: https://github.com/jsk-ros-pkg/jsk_pr2eus.git
version: master
-# we need to use the development branch (throttle-insta360 branch in 708yamaguchi's fork)
-# Restore jsk-ros-pkg/jsk_recognition master branch if https://github.com/jsk-ros-pkg/jsk_recognition/pull/2596 is merged.
+# we need to use the development branch until PR below are merged.
+# - https://github.com/jsk-ros-pkg/jsk_recognition/pull/2642
+# Restore jsk-ros-pkg/jsk_recognition master branch when the PRs are merged.
# - git:
# local-name: jsk-ros-pkg/jsk_recognition
# uri: https://github.com/jsk-ros-pkg/jsk_recognition.git
# version: master
- git:
local-name: jsk-ros-pkg/jsk_recognition
- uri: https://github.com/708yamaguchi/jsk_recognition.git
- version: throttle-insta360
+ uri: https://github.com/sktometometo/jsk_recognition.git
+ version: develop/fetch
# we need to use the development branch (fetch15 branch in knorth55's fork)
# until it is merged to master
- git:
@@ -62,10 +68,6 @@
local-name: knorth55/app_manager_utils
uri: https://github.com/knorth55/app_manager_utils
version: master
-- git:
- local-name: locusrobotics/catkin_virtualenv
- uri: https://github.com/locusrobotics/catkin_virtualenv.git
- version: 0.5.0
- git:
local-name: mikeferguson/robot_calibration
uri: https://github.com/mikeferguson/robot_calibration.git
@@ -99,16 +101,23 @@
local-name: strands-project/mongodb_store
uri: https://github.com/708yamaguchi/mongodb_store.git
version: fetch15
-# we need to use the development branch (fetch15 branch in knorth55's fork)
-# until it is merged to master
+# we need to use the develop branch for basic authentication
- git:
local-name: tork-a/roswww
- uri: https://github.com/knorth55/roswww.git
- version: fetch15
+ uri: https://github.com/tork-a/roswww.git
+ version: develop
+# we need to use the development branch until PR below are merged.
+# - https://github.com/tork-a/visualization_rwt/pull/117
+# - https://github.com/tork-a/visualization_rwt/pull/124
+# Restore original repository when the PRs are merged.
+# - git:
+# local-name: tork-a/visualization_rwt
+# uri: https://github.com/tork-a/visualization_rwt.git
+# version: kinetic-devel
- git:
local-name: tork-a/visualization_rwt
- uri: https://github.com/tork-a/visualization_rwt.git
- version: kinetic-devel
+ uri: https://github.com/knorth55/visualization_rwt.git
+ version: fetch15
# robot_pose_publisher is not released on melodic
- git:
local-name: GT-RAIL/robot_pose_publisher
@@ -119,16 +128,57 @@
# If you upgrade realsense-ros version, please upgrade librealsense packages.
# Currently, realsense-ros 2.3.0 (source) and librealsense 2.45.0 (apt) works
# https://github.com/IntelRealSense/librealsense/issues/10304#issuecomment-1067354378
+# realsense_camera has a problem in src/base_realsense_node.cpp which prevents it from build (find_if -> std::find_if)
+# https://github.com/IntelRealSense/realsense-ros/issues/910
+# Using Affonso-Gui/realsense e155795440b29d4edb78827ac0d1f50e4aad8750 for now
- git:
local-name: IntelRealSense/realsense-ros
- uri: https://github.com/IntelRealSense/realsense-ros.git
- version: 2.3.0
+ uri: https://github.com/Affonso-Gui/realsense.git
+ version: fetch15
+# - git:
+# local-name: IntelRealSense/realsense-ros
+# uri: https://github.com/IntelRealSense/realsense-ros.git
+# version: 2.3.0
+# Until PRs below merged, we need to use develop branch
+# - https://github.com/fetchrobotics/fetch_open_auto_dock/pull/9
+# - https://github.com/fetchrobotics/fetch_open_auto_dock/pull/8
- git:
local-name: fetchrobotics/fetch_open_auto_dock
- uri: https://github.com/fetchrobotics/fetch_open_auto_dock.git
- version: melodic-devel
+ uri: https://github.com/sktometometo/fetch_open_auto_dock.git
+ version: fetchdev
# Use https://github.com/ros-planning/navigation/pull/839 based on 7f22997e6804d9d7249b8a1d789bf27343b26f75
- git:
local-name: ros-planning/navigation
uri: https://github.com/708yamaguchi/navigation.git
version: fetch15
+# Use coral_usb_ros + cv_bridge_python3
+- git:
+ local-name: jsk-ros-pkg/coral_usb_ros
+ uri: https://github.com/jsk-ros-pkg/coral_usb_ros.git
+ version: master
+# eus10 and roseus_resume
+- git:
+ local-name: euslisp/Euslisp
+ uri: https://github.com/Affonso-Gui/EusLisp.git
+ version: eus10
+- git:
+ local-name: jsk-ros-pkg/jsk_roseus
+ uri: https://github.com/knorth55/jsk_roseus.git
+ version: fetch15
+- git:
+ local-name: euslisp/jskeus
+ uri: https://github.com/euslisp/jskeus.git
+ version: master
+- git:
+ local-name: jsk-ros-pkg/geneus
+ uri: https://github.com/jsk-ros-pkg/geneus.git
+ version: master
+- git:
+ local-name: roseus_resume
+ uri: https://github.com/Affonso-Gui/roseus_resume.git
+ version: eus10
+# roseus_bt
+- git:
+ local-name: BehaviorTree.ROS
+ uri: https://github.com/BehaviorTree/BehaviorTree.ROS
+ version: master
diff --git a/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount.step b/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount.step
new file mode 100755
index 0000000000..534ba78990
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount.step
@@ -0,0 +1,3571 @@
+ISO-10303-21;
+HEADER;
+FILE_DESCRIPTION (( 'STEP AP214' ),
+ '1' );
+FILE_NAME ('jsk_fetch_l515headmount.STEP',
+ '2021-07-29T05:55:12',
+ ( '' ),
+ ( '' ),
+ 'SwSTEP 2.0',
+ 'SolidWorks 2019',
+ '' );
+FILE_SCHEMA (( 'AUTOMOTIVE_DESIGN' ));
+ENDSEC;
+
+DATA;
+#1 = ORIENTED_EDGE ( 'NONE', *, *, #2348, .T. ) ;
+#2 = CARTESIAN_POINT ( 'NONE', ( -42.23330461419529058, 8.916679505932764371, -9.689801797596356181 ) ) ;
+#3 = CARTESIAN_POINT ( 'NONE', ( -42.92726601009340470, 6.534591258039998962, 13.39603710458470509 ) ) ;
+#4 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#5 = CARTESIAN_POINT ( 'NONE', ( 36.80118201742403272, 30.26574514009470107, 6.931169661506055846 ) ) ;
+#6 = DIRECTION ( 'NONE', ( -0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#7 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#8 = ORIENTED_EDGE ( 'NONE', *, *, #564, .T. ) ;
+#9 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -13.98362640057776396 ) ) ;
+#10 = LINE ( 'NONE', #1151, #2476 ) ;
+#11 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981658586, -13.75537618510425908 ) ) ;
+#12 = ORIENTED_EDGE ( 'NONE', *, *, #142, .T. ) ;
+#13 = CARTESIAN_POINT ( 'NONE', ( -39.54706189466428867, 22.29032810037977441, -7.246389550346690633 ) ) ;
+#14 = CARTESIAN_POINT ( 'NONE', ( -42.98895923545502029, 6.322823416230354709, 12.85975734684950211 ) ) ;
+#15 = ORIENTED_EDGE ( 'NONE', *, *, #2391, .F. ) ;
+#16 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, 20.50000000000000000 ) ) ;
+#17 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -12.50000000000000000 ) ) ;
+#18 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, 17.99999999999999645 ) ) ;
+#19 = ADVANCED_FACE ( 'NONE', ( #52, #1774 ), #1120, .F. ) ;
+#20 = LINE ( 'NONE', #646, #2637 ) ;
+#21 = ORIENTED_EDGE ( 'NONE', *, *, #1711, .F. ) ;
+#22 = AXIS2_PLACEMENT_3D ( 'NONE', #2158, #1471, #25 ) ;
+#23 = EDGE_CURVE ( 'NONE', #1380, #2155, #589, .T. ) ;
+#24 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#25 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#26 = CARTESIAN_POINT ( 'NONE', ( -33.77993903277662469, 33.99780946660671077, -16.55285594409495431 ) ) ;
+#27 = ORIENTED_EDGE ( 'NONE', *, *, #1107, .T. ) ;
+#28 = CARTESIAN_POINT ( 'NONE', ( -41.50565207264286727, 16.60159655432851622, -8.399460044431059202 ) ) ;
+#29 = CARTESIAN_POINT ( 'NONE', ( -38.21994831615298693, 22.69290528549759500, 18.00000000000000000 ) ) ;
+#30 = VERTEX_POINT ( 'NONE', #336 ) ;
+#31 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 10.00000000000000000, -0.5000000000000004441 ) ) ;
+#32 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#33 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#34 = CARTESIAN_POINT ( 'NONE', ( -33.03257378525533738, 31.99619020741757680, -20.50000000000000000 ) ) ;
+#35 = ORIENTED_EDGE ( 'NONE', *, *, #2010, .T. ) ;
+#36 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -13.98362640057776396 ) ) ;
+#37 = CARTESIAN_POINT ( 'NONE', ( -33.59905643722286328, 39.56632930884226340, 8.586160965561267844 ) ) ;
+#38 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#39 = CARTESIAN_POINT ( 'NONE', ( 39.33771734142730736, 22.89837003650006153, 17.86806588320381906 ) ) ;
+#40 = VERTEX_POINT ( 'NONE', #765 ) ;
+#41 = CIRCLE ( 'NONE', #1564, 4.000000000000000000 ) ;
+#42 = CARTESIAN_POINT ( 'NONE', ( -42.54375550167739561, 7.851027417807084241, -10.29502450199527885 ) ) ;
+#43 = AXIS2_PLACEMENT_3D ( 'NONE', #2395, #472, #1329 ) ;
+#44 = CARTESIAN_POINT ( 'NONE', ( -44.36934426592454628, 8.283993259259583297, 15.18396257553578543 ) ) ;
+#45 = MECHANICAL_DESIGN_GEOMETRIC_PRESENTATION_REPRESENTATION ( '', ( #1028 ), #1164 ) ;
+#46 = ORIENTED_EDGE ( 'NONE', *, *, #2669, .T. ) ;
+#47 = CARTESIAN_POINT ( 'NONE', ( -31.80596576484949978, 35.85259229793309999, -12.25433914335906138 ) ) ;
+#48 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#49 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#50 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #2409, #1139, #72 ),
+ ( #929, #2006, #967 ),
+ ( #1982, #2033, #518 ),
+ ( #1594, #2670, #494 ),
+ ( #2621, #931, #322 ),
+ ( #270, #918, #296 ),
+ ( #87, #715, #700 ),
+ ( #2647, #1166, #1818 ),
+ ( #737, #1375, #2636 ),
+ ( #955, #477, #1768 ),
+ ( #2206, #2190, #1794 ),
+ ( #1348, #2227, #1128 ),
+ ( #2451, #726, #285 ),
+ ( #2659, #2412, #75 ),
+ ( #2021, #1573, #1556 ),
+ ( #2429, #1334, #111 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 0.000000000000000000, 0.0007846569004674718581, 0.001176985350701207841, 0.001569313800934943716, 0.001961642251168679591, 0.002353970701402415683, 0.002746299151636151341, 0.003138627601869887433 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.2168728904164866356, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2100147327725616075, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2040713226379783085, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1976904878458617398, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1959997285647044618, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1937506485301896675, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1931712647203893130, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1931624902695895929, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1937461617684644033, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1960171411409001030, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1976866490321846082, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2019151207544363225, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2044880519031182509, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2102903139294395318, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2134843460331373177, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2168728904164864135, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#51 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732930828, -12.50000000000000000 ) ) ;
+#52 = FACE_BOUND ( 'NONE', #149, .T. ) ;
+#53 = VECTOR ( 'NONE', #2401, 1000.000000000000000 ) ;
+#54 = CARTESIAN_POINT ( 'NONE', ( -33.27426852239292288, 34.99052006355213251, -17.37092549432447797 ) ) ;
+#55 = DIRECTION ( 'NONE', ( -0.3223706153912012651, -0.9363265015356202481, -0.1391756762310300544 ) ) ;
+#56 = CYLINDRICAL_SURFACE ( 'NONE', #2012, 10.00000000000000178 ) ;
+#57 = CARTESIAN_POINT ( 'NONE', ( 38.21994831614073718, 22.69290528549494113, 18.00000000000000000 ) ) ;
+#58 = CARTESIAN_POINT ( 'NONE', ( -34.58241321390985945, 33.72141263154953350, -17.16371861517608721 ) ) ;
+#59 = CARTESIAN_POINT ( 'NONE', ( -43.57608948207057153, 10.58800436055562955, 12.97832405504144404 ) ) ;
+#60 = EDGE_CURVE ( 'NONE', #776, #1800, #1194, .T. ) ;
+#61 = ORIENTED_EDGE ( 'NONE', *, *, #228, .F. ) ;
+#62 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#63 = CARTESIAN_POINT ( 'NONE', ( -30.33154712770451766, 33.30668923918088353, -10.77472311316831899 ) ) ;
+#64 = CARTESIAN_POINT ( 'NONE', ( 28.36585991138574769, 54.76616564920129804, -135.3085362679971126 ) ) ;
+#65 = CARTESIAN_POINT ( 'NONE', ( 32.33154110754892940, 43.24783170891807060, 14.42725225151875534 ) ) ;
+#66 = ORIENTED_EDGE ( 'NONE', *, *, #196, .F. ) ;
+#67 = CARTESIAN_POINT ( 'NONE', ( -31.69971801055696758, 34.68748463690393891, -16.18572902251193923 ) ) ;
+#68 = CARTESIAN_POINT ( 'NONE', ( -31.98742823517704892, 44.24730866661698769, 11.64916250785373286 ) ) ;
+#69 = DIRECTION ( 'NONE', ( -0.3255388549733763681, 0.9455286637128580418, 0.000000000000000000 ) ) ;
+#70 = CARTESIAN_POINT ( 'NONE', ( 43.34254294013591391, 11.26634105166344746, 16.79537387900334622 ) ) ;
+#71 = VECTOR ( 'NONE', #1551, 1000.000000000000000 ) ;
+#72 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -13.98362640057776396 ) ) ;
+#73 = CARTESIAN_POINT ( 'NONE', ( -38.93961336429644859, 20.22258681569368122, 17.85684972154301775 ) ) ;
+#74 = EDGE_CURVE ( 'NONE', #1838, #2746, #886, .T. ) ;
+#75 = CARTESIAN_POINT ( 'NONE', ( -30.19304736377159770, 32.97365565854406100, -11.24041560048074473 ) ) ;
+#76 = CARTESIAN_POINT ( 'NONE', ( -33.96276985076541166, 34.85354108118977479, -7.259012294323295755 ) ) ;
+#77 = CARTESIAN_POINT ( 'NONE', ( -9.209006928406447301, 19.08775981524247101, 4.500000000000000888 ) ) ;
+#78 = CARTESIAN_POINT ( 'NONE', ( -4.000000000000001776, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#79 = CARTESIAN_POINT ( 'NONE', ( 32.80462327031811753, 41.87376302754749702, 15.75747938236830414 ) ) ;
+#80 = EDGE_CURVE ( 'NONE', #2740, #839, #865, .T. ) ;
+#81 = ORIENTED_EDGE ( 'NONE', *, *, #686, .F. ) ;
+#82 =( LENGTH_UNIT ( ) NAMED_UNIT ( * ) SI_UNIT ( .MILLI., .METRE. ) );
+#83 = LINE ( 'NONE', #2003, #469 ) ;
+#84 = FACE_OUTER_BOUND ( 'NONE', #2066, .T. ) ;
+#85 = CARTESIAN_POINT ( 'NONE', ( -34.11999303975385089, 36.76639026393176835, 16.89816763280588674 ) ) ;
+#86 = CIRCLE ( 'NONE', #987, 27.50000000000000000 ) ;
+#87 = CARTESIAN_POINT ( 'NONE', ( -32.00002563971278846, 32.30164887348127678, -12.61180519082622808 ) ) ;
+#88 =( GEOMETRIC_REPRESENTATION_CONTEXT ( 3 ) GLOBAL_UNCERTAINTY_ASSIGNED_CONTEXT ( ( #1813 ) ) GLOBAL_UNIT_ASSIGNED_CONTEXT ( ( #1396, #1419, #2275 ) ) REPRESENTATION_CONTEXT ( 'NONE', 'WORKASPACE' ) );
+#89 = CARTESIAN_POINT ( 'NONE', ( -34.52677352094674745, 33.75846259183247611, -7.871530568415401774 ) ) ;
+#90 = DIRECTION ( 'NONE', ( -1.224646799147352961E-16, -3.556990613648029896E-16, 1.000000000000000000 ) ) ;
+#91 = VECTOR ( 'NONE', #1006, 1000.000000000000000 ) ;
+#92 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#93 = CARTESIAN_POINT ( 'NONE', ( 32.28090642499899587, 43.07922933215750305, 14.27218612157843225 ) ) ;
+#94 = EDGE_CURVE ( 'NONE', #1630, #1626, #419, .T. ) ;
+#95 = ORIENTED_EDGE ( 'NONE', *, *, #2149, .T. ) ;
+#96 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#97 = VECTOR ( 'NONE', #1799, 1000.000000000000000 ) ;
+#98 = CARTESIAN_POINT ( 'NONE', ( -33.89385567669372534, 38.71008395840141247, 4.349116141318785544 ) ) ;
+#99 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, -15.25000000000000355 ) ) ;
+#100 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639387876, 32.97280677233779045, -18.46760616633292429 ) ) ;
+#101 = CARTESIAN_POINT ( 'NONE', ( -33.67197404367669833, 35.02009655344337347, -8.538045649365114897 ) ) ;
+#102 = CARTESIAN_POINT ( 'NONE', ( -10.88337182448036522, 14.37644341801383874, -0.5000000000000004441 ) ) ;
+#103 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#104 = CARTESIAN_POINT ( 'NONE', ( -32.07817912651937320, 34.84403317655319654, -8.472129640656142158 ) ) ;
+#105 = ORIENTED_EDGE ( 'NONE', *, *, #2656, .T. ) ;
+#106 = EDGE_CURVE ( 'NONE', #1614, #2559, #2478, .T. ) ;
+#107 = CARTESIAN_POINT ( 'NONE', ( -43.67796458834995832, 10.29210778282046235, 12.80896965692393508 ) ) ;
+#108 = PLANE ( 'NONE', #2081 ) ;
+#109 = CARTESIAN_POINT ( 'NONE', ( -31.98742823517723011, 44.24730866661645479, 13.35083749214627780 ) ) ;
+#110 = AXIS2_PLACEMENT_3D ( 'NONE', #2154, #1238, #2537 ) ;
+#111 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -11.01637359942223959 ) ) ;
+#112 = PRESENTATION_STYLE_ASSIGNMENT (( #1819 ) ) ;
+#113 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#114 = CARTESIAN_POINT ( 'NONE', ( -42.99999999999999289, 6.284924945759550852, -9.278174593052025187 ) ) ;
+#115 = CARTESIAN_POINT ( 'NONE', ( 42.81541933757661411, 6.918515559511263291, 13.92432882938398286 ) ) ;
+#116 = VERTEX_POINT ( 'NONE', #2027 ) ;
+#117 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #2356, #1055, #1692, #1083 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 4.712388980384689674, 6.283185307179586232 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#118 = CARTESIAN_POINT ( 'NONE', ( -42.96469508733384401, 6.406112403811265565, 11.87134553244891144 ) ) ;
+#119 = VERTEX_POINT ( 'NONE', #959 ) ;
+#120 = CARTESIAN_POINT ( 'NONE', ( -34.07699738444878790, 38.17814827220534823, 4.194022380370180159 ) ) ;
+#121 = CARTESIAN_POINT ( 'NONE', ( -32.04197879035282170, 44.08886640158232950, 13.28475262339844143 ) ) ;
+#122 = CARTESIAN_POINT ( 'NONE', ( -43.08759061399219092, 12.00685091267271609, -13.40234256660552958 ) ) ;
+#123 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688397407, 32.64726791736432432, -7.450487090025933234 ) ) ;
+#124 = CARTESIAN_POINT ( 'NONE', ( -35.84294207509136498, 33.06965825867597175, -17.78067036384265620 ) ) ;
+#125 = CIRCLE ( 'NONE', #490, 1.750000000000000888 ) ;
+#126 = CARTESIAN_POINT ( 'NONE', ( 42.95367559951917968, 6.443937839889442465, 11.78200188557572403 ) ) ;
+#127 = CARTESIAN_POINT ( 'NONE', ( -31.56936906224671446, 34.61866784516882234, -8.943873010434925774 ) ) ;
+#128 = CARTESIAN_POINT ( 'NONE', ( 43.96825760039320130, 9.448950784602986985, 15.93434294849813426 ) ) ;
+#129 = ORIENTED_EDGE ( 'NONE', *, *, #2281, .T. ) ;
+#130 = CIRCLE ( 'NONE', #1385, 4.000000000000001776 ) ;
+#131 = PRESENTATION_STYLE_ASSIGNMENT (( #1802 ) ) ;
+#132 = CARTESIAN_POINT ( 'NONE', ( -43.64736563081370235, 10.38098255456961638, 13.50780290470233425 ) ) ;
+#133 = EDGE_LOOP ( 'NONE', ( #2282, #2759 ) ) ;
+#134 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#135 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.21079393477482000, 12.49999999999999822 ) ) ;
+#136 = CIRCLE ( 'NONE', #1486, 5.499999999999996447 ) ;
+#137 = EDGE_LOOP ( 'NONE', ( #66, #1600, #1112, #403 ) ) ;
+#138 = EDGE_CURVE ( 'NONE', #620, #1225, #394, .T. ) ;
+#139 = CARTESIAN_POINT ( 'NONE', ( -44.71499975903603996, 7.280035754606394960, -10.77904823010123891 ) ) ;
+#140 = CARTESIAN_POINT ( 'NONE', ( -35.28033923126954363, 32.78339202938938257, -7.467607744548145021 ) ) ;
+#141 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#142 = EDGE_CURVE ( 'NONE', #2735, #2480, #2054, .T. ) ;
+#143 = CARTESIAN_POINT ( 'NONE', ( 43.05645483273637097, 12.09728489066162815, 13.23782609026664758 ) ) ;
+#144 = CARTESIAN_POINT ( 'NONE', ( -35.74840755681335480, 33.32353198708886310, -6.567148390511783340 ) ) ;
+#145 = CARTESIAN_POINT ( 'NONE', ( -41.08453677334735943, 17.82472731161873725, -18.46428714509136881 ) ) ;
+#146 = ORIENTED_EDGE ( 'NONE', *, *, #1593, .F. ) ;
+#147 = CARTESIAN_POINT ( 'NONE', ( -43.08988301295357104, 12.00019263241778589, 10.51163916011575772 ) ) ;
+#148 = ORIENTED_EDGE ( 'NONE', *, *, #1927, .T. ) ;
+#149 = EDGE_LOOP ( 'NONE', ( #2303, #2492 ) ) ;
+#150 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#151 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#152 = CARTESIAN_POINT ( 'NONE', ( -34.39450537660771090, 33.83467480295717422, -7.959224172008664944 ) ) ;
+#153 = ORIENTED_EDGE ( 'NONE', *, *, #2129, .F. ) ;
+#154 = CARTESIAN_POINT ( 'NONE', ( -43.46764395096884215, 10.90298475856552329, -13.90979738967100587 ) ) ;
+#155 = CARTESIAN_POINT ( 'NONE', ( -31.86183217147021907, 34.77050092954914362, -16.34513348874330063 ) ) ;
+#156 = ADVANCED_FACE ( 'NONE', ( #989 ), #108, .T. ) ;
+#157 = VECTOR ( 'NONE', #608, 1000.000000000000000 ) ;
+#158 = CARTESIAN_POINT ( 'NONE', ( 42.92726601009340470, 6.534591258040051365, 11.60396289541529669 ) ) ;
+#159 = CARTESIAN_POINT ( 'NONE', ( 32.72692620558899534, 41.54822417257172873, 9.743671291842574078 ) ) ;
+#160 = ADVANCED_FACE ( 'NONE', ( #1372 ), #759, .F. ) ;
+#161 = CARTESIAN_POINT ( 'NONE', ( -43.47883299615393327, 10.87048614040918082, -13.40461196430244328 ) ) ;
+#162 = ORIENTED_EDGE ( 'NONE', *, *, #1171, .F. ) ;
+#163 = CARTESIAN_POINT ( 'NONE', ( -43.67474508509558007, 10.30145884049983174, 13.29169076162558127 ) ) ;
+#164 = ORIENTED_EDGE ( 'NONE', *, *, #828, .F. ) ;
+#165 = ORIENTED_EDGE ( 'NONE', *, *, #106, .F. ) ;
+#166 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 9.749999999999998224 ) ) ;
+#167 = CARTESIAN_POINT ( 'NONE', ( -30.09358264888986056, 32.72699386974174729, -13.01985449771353309 ) ) ;
+#168 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, -15.25000000000000355 ) ) ;
+#169 = ORIENTED_EDGE ( 'NONE', *, *, #1270, .T. ) ;
+#170 = CARTESIAN_POINT ( 'NONE', ( -43.01892871247722638, 12.20627962907616926, -12.98569229327060270 ) ) ;
+#171 = CARTESIAN_POINT ( 'NONE', ( -34.33743115981847893, 33.86227435829512444, -17.00121632836259877 ) ) ;
+#172 = CARTESIAN_POINT ( 'NONE', ( 44.90380467601645620, 6.731651258784860481, 11.75704472016694524 ) ) ;
+#173 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#174 = CARTESIAN_POINT ( 'NONE', ( -43.59320368275488988, 10.53829610896492497, -11.29710649816777490 ) ) ;
+#175 = VERTEX_POINT ( 'NONE', #1604 ) ;
+#176 = VECTOR ( 'NONE', #103, 1000.000000000000000 ) ;
+#177 = ADVANCED_FACE ( 'NONE', ( #84 ), #1792, .F. ) ;
+#178 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#179 = AXIS2_PLACEMENT_3D ( 'NONE', #2294, #1157, #1377 ) ;
+#180 = ORIENTED_EDGE ( 'NONE', *, *, #1994, .T. ) ;
+#181 = FACE_BOUND ( 'NONE', #2318, .T. ) ;
+#182 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 16.99999999999999645 ) ) ;
+#183 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.8591838389225134875, 0.5116670117707144971 ) ) ;
+#184 = EDGE_LOOP ( 'NONE', ( #1421, #1851, #1633, #818 ) ) ;
+#185 = CARTESIAN_POINT ( 'NONE', ( -44.59669480201716141, 7.623652887282672097, -10.39498267265966192 ) ) ;
+#186 = CARTESIAN_POINT ( 'NONE', ( 15.54977303496059449, 10.28868360277136418, -0.5000000000000004441 ) ) ;
+#187 = CIRCLE ( 'NONE', #949, 2.999999999999999112 ) ;
+#188 = CARTESIAN_POINT ( 'NONE', ( 42.91187591077668628, 6.587419232237252231, 11.51526756757565906 ) ) ;
+#189 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000147793, 44.21079393474678199, 12.10821283959560901 ) ) ;
+#190 = CARTESIAN_POINT ( 'NONE', ( -41.24826085141513943, 12.29793555972578289, -16.55044276841356066 ) ) ;
+#191 = ORIENTED_EDGE ( 'NONE', *, *, #1462, .T. ) ;
+#192 = B_SPLINE_CURVE_WITH_KNOTS ( 'NONE', 3,
+ ( #9, #866, #1903, #2569, #167, #2541, #1661, #414, #2322, #1873, #621, #1268, #1021, #2724 ),
+ .UNSPECIFIED., .F., .F.,
+ ( 4, 2, 2, 2, 2, 2, 4 ),
+ ( 0.000000000000000000, 0.0007814279436001421528, 0.001172141915400213555, 0.001562855887200285173, 0.001953569859000356575, 0.002344283830800427976, 0.003125711774400576418 ),
+ .UNSPECIFIED. ) ;
+#193 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 0.000000000000000000, -20.50000000000000000 ) ) ;
+#194 = ORIENTED_EDGE ( 'NONE', *, *, #2091, .T. ) ;
+#195 = FACE_BOUND ( 'NONE', #424, .T. ) ;
+#196 = EDGE_CURVE ( 'NONE', #2749, #1788, #2461, .T. ) ;
+#197 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#198 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 17.99999999999999645 ) ) ;
+#199 = EDGE_CURVE ( 'NONE', #2197, #2706, #2305, .T. ) ;
+#200 = ORIENTED_EDGE ( 'NONE', *, *, #1548, .T. ) ;
+#201 = CARTESIAN_POINT ( 'NONE', ( -43.66413554073179171, 10.33227429663104502, -13.34465359738889134 ) ) ;
+#202 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 5.000000000000000000, -20.50000000000000000 ) ) ;
+#203 = CARTESIAN_POINT ( 'NONE', ( 43.20911154149872857, 11.65389298324826051, 11.25261746734080681 ) ) ;
+#204 = CARTESIAN_POINT ( 'NONE', ( 33.27732909717393994, 40.50078741505782887, 8.535066353114178384 ) ) ;
+#205 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#206 = ORIENTED_EDGE ( 'NONE', *, *, #1159, .F. ) ;
+#207 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371264344, 6.610463800733539230, 12.39364399429241104 ) ) ;
+#208 = ORIENTED_EDGE ( 'NONE', *, *, #1942, .F. ) ;
+#209 = FACE_OUTER_BOUND ( 'NONE', #225, .T. ) ;
+#210 = ORIENTED_EDGE ( 'NONE', *, *, #862, .F. ) ;
+#211 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#212 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 15.25000000000000355 ) ) ;
+#213 = EDGE_CURVE ( 'NONE', #1589, #2058, #1862, .T. ) ;
+#214 = CARTESIAN_POINT ( 'NONE', ( 43.01186382226980953, 12.22679962567527312, 12.11340478805033705 ) ) ;
+#215 = CARTESIAN_POINT ( 'NONE', ( 43.29723134156520814, 11.39794873718754076, 11.00854648015568849 ) ) ;
+#216 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371259370, 6.610463800733696438, -12.63395078727329413 ) ) ;
+#217 = ORIENTED_EDGE ( 'NONE', *, *, #736, .F. ) ;
+#218 = FACE_OUTER_BOUND ( 'NONE', #802, .T. ) ;
+#219 = VERTEX_POINT ( 'NONE', #1804 ) ;
+#220 = CIRCLE ( 'NONE', #569, 30.00000000000000711 ) ;
+#221 = STYLED_ITEM ( 'NONE', ( #131 ), #781 ) ;
+#222 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371286370, 44.36900610829383140, 12.50000000000000000 ) ) ;
+#223 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, -12.50000000000000000 ) ) ;
+#224 = CARTESIAN_POINT ( 'NONE', ( -31.67121089991161043, 36.36515437509692106, -11.90483886919879097 ) ) ;
+#225 = EDGE_LOOP ( 'NONE', ( #344, #951, #2028, #668, #1184, #180, #2142, #393 ) ) ;
+#226 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688395986, 32.64726791736432432, -7.450487090025976755 ) ) ;
+#227 = FACE_OUTER_BOUND ( 'NONE', #588, .T. ) ;
+#228 = EDGE_CURVE ( 'NONE', #2469, #2690, #249, .T. ) ;
+#229 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#230 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#231 = CARTESIAN_POINT ( 'NONE', ( -44.00000000000000000, 6.284924945759554404, -12.50000000000000000 ) ) ;
+#232 = CARTESIAN_POINT ( 'NONE', ( -34.27817459305202163, 36.22341709370545004, 18.00000000000000000 ) ) ;
+#233 = EDGE_CURVE ( 'NONE', #1869, #2551, #1617, .T. ) ;
+#234 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#235 = ORIENTED_EDGE ( 'NONE', *, *, #842, .T. ) ;
+#236 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#237 = CARTESIAN_POINT ( 'NONE', ( -32.53238346409453641, 34.95761181683994323, -16.88420805175061901 ) ) ;
+#238 = EDGE_LOOP ( 'NONE', ( #381, #2510, #2013, #146, #590 ) ) ;
+#239 = CARTESIAN_POINT ( 'NONE', ( -43.47883299615207875, 10.87048614041807326, -11.59538803570062981 ) ) ;
+#240 = CARTESIAN_POINT ( 'NONE', ( -39.93733163486376014, 16.79782431294328049, 17.44358128244661188 ) ) ;
+#241 = ORIENTED_EDGE ( 'NONE', *, *, #1424, .F. ) ;
+#242 = CARTESIAN_POINT ( 'NONE', ( 42.98233690606030422, 6.345555189378215388, 12.94953407607404650 ) ) ;
+#243 = EDGE_CURVE ( 'NONE', #2587, #1870, #1409, .T. ) ;
+#244 = CARTESIAN_POINT ( 'NONE', ( -4.000000000000001776, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#245 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, -12.50000000000000000 ) ) ;
+#246 = CARTESIAN_POINT ( 'NONE', ( -42.98248968273709636, 6.345030768958843659, -12.95293874365824927 ) ) ;
+#247 = ORIENTED_EDGE ( 'NONE', *, *, #898, .F. ) ;
+#248 = CIRCLE ( 'NONE', #1676, 1.000000000000000888 ) ;
+#249 = CIRCLE ( 'NONE', #1722, 10.00000000000000178 ) ;
+#250 = CARTESIAN_POINT ( 'NONE', ( -32.36046589817100028, 34.93525169092457361, -16.76273340782259424 ) ) ;
+#251 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371286370, 44.36900610829383851, 12.50000000000000000 ) ) ;
+#252 = CARTESIAN_POINT ( 'NONE', ( -34.91452504304472626, 34.37640409097092231, -17.37547336651662633 ) ) ;
+#253 = CARTESIAN_POINT ( 'NONE', ( 35.24008933272911293, 34.79994392320067220, 17.60429368842698494 ) ) ;
+#254 = VERTEX_POINT ( 'NONE', #320 ) ;
+#255 = CARTESIAN_POINT ( 'NONE', ( -44.40633062024019040, 8.176566272780355860, -9.894119865994008833 ) ) ;
+#256 = CARTESIAN_POINT ( 'NONE', ( -44.72738788084880923, 7.244054414484955906, 14.18329770745371476 ) ) ;
+#257 = B_SPLINE_CURVE_WITH_KNOTS ( 'NONE', 3,
+ ( #1759, #486, #277, #1135, #1988, #1529, #1311, #1150, #2198, #925, #1973, #2629, #54, #2582, #707, #250, #2157, #67, #1958, #910, #1356, #663, #2393, #2002, #680, #36 ),
+ .UNSPECIFIED., .F., .F.,
+ ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 0.000000000000000000, 0.0005491052105900589902, 0.001098210421180117980, 0.001647315631770177079, 0.002196420842360235961, 0.003294631263540333775, 0.003843736474130380731, 0.004392841684720428554, 0.005491052105900521597, 0.006040157316490555976, 0.006589262527080589488, 0.007687472948260670388, 0.008785683369440753024 ),
+ .UNSPECIFIED. ) ;
+#258 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371285660, 6.610463800732986783, 12.49999999999998934 ) ) ;
+#259 = LINE ( 'NONE', #2564, #1527 ) ;
+#260 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#261 = FACE_OUTER_BOUND ( 'NONE', #2124, .T. ) ;
+#262 = ORIENTED_EDGE ( 'NONE', *, *, #1623, .T. ) ;
+#263 = EDGE_CURVE ( 'NONE', #1281, #2512, #1481, .T. ) ;
+#264 = CARTESIAN_POINT ( 'NONE', ( -32.55641871737685022, 42.13350728234175335, 10.06266836556246069 ) ) ;
+#265 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, 12.50000000000000000 ) ) ;
+#266 = CARTESIAN_POINT ( 'NONE', ( 33.54851438728953639, 39.71312885736416121, 16.79537387900164802 ) ) ;
+#267 = CARTESIAN_POINT ( 'NONE', ( -31.17158371284658358, 32.72879920981659296, -13.98362640057776396 ) ) ;
+#268 = CARTESIAN_POINT ( 'NONE', ( -44.60389381109560247, 7.602743341985673808, 14.59040309685429726 ) ) ;
+#269 = EDGE_LOOP ( 'NONE', ( #1081, #854, #262, #1023, #95 ) ) ;
+#270 = CARTESIAN_POINT ( 'NONE', ( -32.00337995441419281, 32.31131413025062216, -12.72169276642418367 ) ) ;
+#271 = CARTESIAN_POINT ( 'NONE', ( -30.93353752410647317, 34.14291697002418857, -9.698515052243562806 ) ) ;
+#272 = CARTESIAN_POINT ( 'NONE', ( -33.45489901264950561, 39.98503501767059021, 4.500000000000003553 ) ) ;
+#273 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, -0.000000000000000000 ) ) ;
+#274 = CARTESIAN_POINT ( 'NONE', ( 32.27750898416621794, 43.40476818713108287, 14.59440178004758515 ) ) ;
+#275 = EDGE_LOOP ( 'NONE', ( #1843, #2291 ) ) ;
+#276 = AXIS2_PLACEMENT_3D ( 'NONE', #388, #2517, #2283 ) ;
+#277 = CARTESIAN_POINT ( 'NONE', ( -35.73397950841482640, 33.31345185808230269, -18.42824659332720927 ) ) ;
+#278 = CARTESIAN_POINT ( 'NONE', ( -36.06038663570740965, 30.10580538338960821, 7.143150278455386193 ) ) ;
+#279 = EDGE_CURVE ( 'NONE', #2740, #1823, #1177, .T. ) ;
+#280 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#281 = CARTESIAN_POINT ( 'NONE', ( 33.32773819346316913, 40.35437403084119268, 16.12052427299085622 ) ) ;
+#282 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688394565, 32.64726791736439537, -17.54951290997401614 ) ) ;
+#283 = CARTESIAN_POINT ( 'NONE', ( -42.17307833103708958, 9.123411929629689610, 15.40598249488322935 ) ) ;
+#284 = EDGE_CURVE ( 'NONE', #1700, #40, #1885, .T. ) ;
+#285 = CARTESIAN_POINT ( 'NONE', ( -30.15188376376476498, 32.87082142819874520, -11.47847238159971361 ) ) ;
+#286 = CARTESIAN_POINT ( 'NONE', ( -33.96223294681012561, 33.97850134521514320, -8.287068595243203717 ) ) ;
+#287 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#288 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#289 = LINE ( 'NONE', #498, #2242 ) ;
+#290 = VERTEX_POINT ( 'NONE', #1888 ) ;
+#291 = CARTESIAN_POINT ( 'NONE', ( -32.72692620558866139, 41.54822417257454958, 9.743671291843066129 ) ) ;
+#292 = CARTESIAN_POINT ( 'NONE', ( 34.73009560827771480, 34.67215538705879396, 17.30582513607999573 ) ) ;
+#293 = CIRCLE ( 'NONE', #1852, 5.499999999999996447 ) ;
+#294 = CARTESIAN_POINT ( 'NONE', ( -36.78005168384700596, 27.63548691358242237, 18.00000000000000000 ) ) ;
+#295 = ADVANCED_FACE ( 'NONE', ( #2070 ), #1871, .T. ) ;
+#296 = CARTESIAN_POINT ( 'NONE', ( -30.07850211878325553, 32.69008087267586404, -12.76200054212559287 ) ) ;
+#297 = CARTESIAN_POINT ( 'NONE', ( -33.03506008595107346, 35.15678958969931500, -9.262171264487561118 ) ) ;
+#298 = CARTESIAN_POINT ( 'NONE', ( 42.99999999999999289, 6.284924945759606807, 9.278174593052025187 ) ) ;
+#299 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#300 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#301 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #2116, #636, #2293, #222 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 0.000000000000000000, 3.141592653589793116 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.3333333333333333703, 0.3333333333333333703, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#302 = ORIENTED_EDGE ( 'NONE', *, *, #632, .T. ) ;
+#303 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#304 = CARTESIAN_POINT ( 'NONE', ( -33.54851438729056667, 39.71312885736113429, 8.204626120997511762 ) ) ;
+#305 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#306 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -12.50000000000000000 ) ) ;
+#307 = AXIS2_PLACEMENT_3D ( 'NONE', #231, #288, #1547 ) ;
+#308 = CARTESIAN_POINT ( 'NONE', ( -35.73620674677023601, 31.21858356992108696, -17.72920197293977296 ) ) ;
+#309 = AXIS2_PLACEMENT_3D ( 'NONE', #1842, #2032, #540 ) ;
+#310 = CARTESIAN_POINT ( 'NONE', ( -35.16385991789602627, 34.13327680790062146, -7.501224860967944608 ) ) ;
+#311 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, 4.500000000000000888 ) ) ;
+#312 = VECTOR ( 'NONE', #791, 1000.000000000000000 ) ;
+#313 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, 79.70475956827036157 ) ) ;
+#314 = EDGE_CURVE ( 'NONE', #484, #550, #1440, .T. ) ;
+#315 = CARTESIAN_POINT ( 'NONE', ( -36.74760041787510545, 27.74687895898129142, -18.00000000000000000 ) ) ;
+#316 = ADVANCED_FACE ( 'NONE', ( #181, #2308, #1687, #2723, #2707 ), #1902, .F. ) ;
+#317 = EDGE_LOOP ( 'NONE', ( #476, #15, #1395, #820 ) ) ;
+#318 = CARTESIAN_POINT ( 'NONE', ( -38.93961336429978815, 20.22258681569468663, 7.143150278458371361 ) ) ;
+#319 = AXIS2_PLACEMENT_3D ( 'NONE', #2181, #907, #1103 ) ;
+#320 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, 20.50000000000000000 ) ) ;
+#321 = CARTESIAN_POINT ( 'NONE', ( -33.32773819346034827, 40.35437403084400643, 16.12052427299086332 ) ) ;
+#322 = CARTESIAN_POINT ( 'NONE', ( -30.09360412887403413, 32.72704643675674419, -13.02023507940720393 ) ) ;
+#323 = SURFACE_STYLE_FILL_AREA ( #751 ) ;
+#324 = CARTESIAN_POINT ( 'NONE', ( -35.77035682042875209, 33.24402226456976450, -17.75534695141841013 ) ) ;
+#325 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -11.24462381489573914 ) ) ;
+#326 = ORIENTED_EDGE ( 'NONE', *, *, #485, .T. ) ;
+#327 = ADVANCED_FACE ( 'NONE', ( #400, #195 ), #578, .F. ) ;
+#328 = CARTESIAN_POINT ( 'NONE', ( -42.17307833103743775, 9.123411929629707373, 9.594017505117285793 ) ) ;
+#329 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #251, #681, #1803 ),
+ ( #1747, #525, #1974 ),
+ ( #68, #732, #2394 ),
+ ( #487, #2654, #1959 ),
+ ( #513, #2447, #2644 ),
+ ( #471, #1343, #264 ),
+ ( #2170, #2436, #291 ),
+ ( #1328, #722, #1109 ),
+ ( #304, #37, #897 ),
+ ( #2185, #911, #1760 ),
+ ( #2630, #708, #2616 ),
+ ( #694, #2199, #278 ),
+ ( #1567, #2422, #502 ),
+ ( #1357, #2213, #756 ),
+ ( #1628, #786, #318 ),
+ ( #537, #2481, #1176 ),
+ ( #986, #2067, #1224 ),
+ ( #2082, #1602, #2250 ),
+ ( #1001, #803, #1197 ),
+ ( #1407, #147, #2691 ),
+ ( #2289, #2666, #770 ),
+ ( #1853, #2704, #328 ),
+ ( #1868, #1640, #2494 ),
+ ( #575, #560, #1397 ),
+ ( #973, #107, #1437 ),
+ ( #1420, #163, #2276 ),
+ ( #2052, #132, #367 ),
+ ( #353, #1211, #1017 ),
+ ( #1615, #339, #2720 ),
+ ( #1382, #1658, #2511 ),
+ ( #1185, #2460, #2239 ),
+ ( #549, #2029, #2040 ),
+ ( #961, #1814, #118 ),
+ ( #1825, #2677, #744 ),
+ ( #2470, #2261, #1839 ),
+ ( #2524, #849, #382 ),
+ ( #207, #1280, #439 ),
+ ( #1295, #819, #1467 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 3.141592653589793116, 3.337942194439155408, 3.534291735288517255, 3.730641276137879103, 3.926990816987241395, 4.319689898685965090, 4.712388980384689674, 5.105088062083414258, 5.301437602932775661, 5.497787143782137953, 5.694136684631500245, 5.792311455056180947, 5.890486225480861648, 5.988660995905542350, 6.086835766330223940, 6.135923151542565179, 6.185010536754905530, 6.234097921967245881, 6.283185307179586232 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.9862881586313551052, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9862886620239492697, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9847468226703547378, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9785975666658145444, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9739896480940227752, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9617554693256528120, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9541292101921223789, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9359386994017488970, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9253744439781098619, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8894434017616509314, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8598216432907053175, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7905347754438739605, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7508658766753982805, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6633476856976968650, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6154803776380897151, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5395685366565845520, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5135864445227050767, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4607773684079000676, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4339434541726965300, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3801944580785211469, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3532598395136424818, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3138283910423176337, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3008525186326038714, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2755469356016050364, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2632145179655118739, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2396680134331112821, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2284507283938273792, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2078644858772128179, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1984960600877155867, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1866179000439710245, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1830235777788575269, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1767131491669811094, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1739978385178161113, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1695842193437420986, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1678868551765243244, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1656105720155000849, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650312009201779229, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650323245415012685, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#330 = CARTESIAN_POINT ( 'NONE', ( -32.00347813279103093, 32.31159637716974231, -12.27482124481528380 ) ) ;
+#331 = CYLINDRICAL_SURFACE ( 'NONE', #309, 5.499999999999996447 ) ;
+#332 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759554404, -12.50000000000000000 ) ) ;
+#333 = CARTESIAN_POINT ( 'NONE', ( -34.88087167186577631, 33.45756437772778469, -7.662864697124865287 ) ) ;
+#334 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, 4.500000000000003553 ) ) ;
+#335 = CARTESIAN_POINT ( 'NONE', ( 42.51245935571762402, 7.958454404286210426, 10.22895474377726543 ) ) ;
+#336 = CARTESIAN_POINT ( 'NONE', ( -25.58861349562032572, 34.92662621701362013, 4.500000000000000888 ) ) ;
+#337 = CARTESIAN_POINT ( 'NONE', ( 33.12690805908246716, 40.17524856008240874, 15.85494385505738535 ) ) ;
+#338 = ORIENTED_EDGE ( 'NONE', *, *, #2467, .F. ) ;
+#339 = CARTESIAN_POINT ( 'NONE', ( -43.47300727646225482, 10.88740696221511328, 13.92728719611389110 ) ) ;
+#340 = EDGE_LOOP ( 'NONE', ( #2362, #2069, #2458, #634, #2753 ) ) ;
+#341 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, 0.000000000000000000 ) ) ;
+#342 = EDGE_CURVE ( 'NONE', #2735, #594, #2292, .T. ) ;
+#343 = CARTESIAN_POINT ( 'NONE', ( -32.65564714380938938, 42.30646467182851467, 15.14364952153608535 ) ) ;
+#344 = ORIENTED_EDGE ( 'NONE', *, *, #1581, .F. ) ;
+#345 = CARTESIAN_POINT ( 'NONE', ( -44.87938373321212282, 6.802581966636197741, -11.56541359197904306 ) ) ;
+#346 = CARTESIAN_POINT ( 'NONE', ( -35.89971384576186608, 32.88406237842249169, -17.79979218800362517 ) ) ;
+#347 = VECTOR ( 'NONE', #729, 1000.000000000000000 ) ;
+#348 = AXIS2_PLACEMENT_3D ( 'NONE', #2615, #2379, #2405 ) ;
+#349 = CARTESIAN_POINT ( 'NONE', ( 43.96825760039332209, 9.448950784602622832, 9.065657051501897712 ) ) ;
+#350 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#351 = CARTESIAN_POINT ( 'NONE', ( -38.47676016858855519, 25.39902294696122809, -19.02197129286439292 ) ) ;
+#352 = ORIENTED_EDGE ( 'NONE', *, *, #595, .F. ) ;
+#353 = CARTESIAN_POINT ( 'NONE', ( -44.72738788084878792, 7.244054414485028737, 10.81670229254635629 ) ) ;
+#354 = ORIENTED_EDGE ( 'NONE', *, *, #1052, .F. ) ;
+#355 = EDGE_LOOP ( 'NONE', ( #1430, #2262, #1987, #1318 ) ) ;
+#356 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#357 = CYLINDRICAL_SURFACE ( 'NONE', #395, 4.000000000000001776 ) ;
+#358 = EDGE_CURVE ( 'NONE', #1823, #1614, #1583, .T. ) ;
+#359 = CARTESIAN_POINT ( 'NONE', ( -34.92487634117518525, 33.40639490680176493, -7.639419080906765025 ) ) ;
+#360 = ORIENTED_EDGE ( 'NONE', *, *, #2264, .F. ) ;
+#361 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, 12.50000000000000000 ) ) ;
+#362 = CARTESIAN_POINT ( 'NONE', ( -34.62647062134031017, 34.56406577910099287, -18.04333830505539993 ) ) ;
+#363 = CARTESIAN_POINT ( 'NONE', ( 44.53214698414789297, 7.811132234350230519, 10.20907795478962043 ) ) ;
+#364 = CARTESIAN_POINT ( 'NONE', ( 32.85740444909577462, 41.72045992200443010, 9.514080636734924212 ) ) ;
+#365 = CARTESIAN_POINT ( 'NONE', ( -40.14276169517929560, 16.09266616533567529, -17.34472462097040690 ) ) ;
+#366 = ORIENTED_EDGE ( 'NONE', *, *, #2216, .T. ) ;
+#367 = CARTESIAN_POINT ( 'NONE', ( -42.71092435547765831, 7.277204487012230771, 10.73119737958485054 ) ) ;
+#368 = ORIENTED_EDGE ( 'NONE', *, *, #2403, .F. ) ;
+#369 = ORIENTED_EDGE ( 'NONE', *, *, #2754, .T. ) ;
+#370 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#371 = FACE_BOUND ( 'NONE', #2277, .T. ) ;
+#372 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, -17.99999999999999645 ) ) ;
+#373 = VERTEX_POINT ( 'NONE', #2513 ) ;
+#374 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#375 = CARTESIAN_POINT ( 'NONE', ( -30.33149636771388558, 33.30663077529035832, -14.22519690359264644 ) ) ;
+#376 = EDGE_CURVE ( 'NONE', #1002, #1098, #1741, .T. ) ;
+#377 = AXIS2_PLACEMENT_3D ( 'NONE', #1199, #539, #2224 ) ;
+#378 = CARTESIAN_POINT ( 'NONE', ( 44.89078164496277168, 6.769476694862829547, 11.65145677386211709 ) ) ;
+#379 = CARTESIAN_POINT ( 'NONE', ( 32.65564714380716538, 42.30646467183074577, 9.856350478463367537 ) ) ;
+#380 = CARTESIAN_POINT ( 'NONE', ( -41.70775951432999307, 10.72066285937099650, -16.04910501072829376 ) ) ;
+#381 = ORIENTED_EDGE ( 'NONE', *, *, #691, .F. ) ;
+#382 = CARTESIAN_POINT ( 'NONE', ( -42.99779078280187150, 6.292508293126855001, 12.32001292924796587 ) ) ;
+#383 = VERTEX_POINT ( 'NONE', #2085 ) ;
+#384 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 8.000000000000003553 ) ) ;
+#385 = CARTESIAN_POINT ( 'NONE', ( -4.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#386 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371286370, 44.36900610829383140, 12.50000000000000000 ) ) ;
+#387 = VERTEX_POINT ( 'NONE', #1035 ) ;
+#388 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#389 = CARTESIAN_POINT ( 'NONE', ( -44.94291777066050031, 6.618047148100451693, 12.71271199270700336 ) ) ;
+#390 = EDGE_CURVE ( 'NONE', #2559, #2325, #796, .T. ) ;
+#391 = CARTESIAN_POINT ( 'NONE', ( 41.26442097287823429, 17.30225306865500556, 8.207638216924999952 ) ) ;
+#392 = CARTESIAN_POINT ( 'NONE', ( -41.50565207264016721, 16.60159655433335857, -16.60053995556925699 ) ) ;
+#393 = ORIENTED_EDGE ( 'NONE', *, *, #517, .F. ) ;
+#394 = B_SPLINE_CURVE_WITH_KNOTS ( 'NONE', 3,
+ ( #325, #1182, #941, #1580, #962, #330, #2667, #1384, #514, #1815, #1152, #1423, #2438, #2004 ),
+ .UNSPECIFIED., .F., .F.,
+ ( 4, 2, 2, 2, 2, 2, 4 ),
+ ( 0.000000000000000000, 0.0006820525966107297269, 0.001023078894916093669, 0.001364105193221457502, 0.001705131491526821336, 0.002046157789832185603, 0.002728210386442917607 ),
+ .UNSPECIFIED. ) ;
+#395 = AXIS2_PLACEMENT_3D ( 'NONE', #1215, #579, #2019 ) ;
+#396 = ADVANCED_FACE ( 'NONE', ( #606 ), #2590, .F. ) ;
+#397 = FACE_BOUND ( 'NONE', #1887, .T. ) ;
+#398 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #2613, #1710, #2565, #645 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 4.712388980384689674, 6.283185307179586232 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#399 = ORIENTED_EDGE ( 'NONE', *, *, #2701, .T. ) ;
+#400 = FACE_OUTER_BOUND ( 'NONE', #1015, .T. ) ;
+#401 = DIRECTION ( 'NONE', ( -0.3348729792147798201, -0.9422632794457278527, 0.000000000000000000 ) ) ;
+#402 = FACE_OUTER_BOUND ( 'NONE', #1019, .T. ) ;
+#403 = ORIENTED_EDGE ( 'NONE', *, *, #1921, .T. ) ;
+#404 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -6.532393833667063276 ) ) ;
+#405 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, -0.000000000000000000 ) ) ;
+#406 = AXIS2_PLACEMENT_3D ( 'NONE', #2355, #432, #1082 ) ;
+#407 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, -9.749999999999998224 ) ) ;
+#408 = CARTESIAN_POINT ( 'NONE', ( 33.54851438729055246, 39.71312885736116982, 8.204626120998362637 ) ) ;
+#409 = CARTESIAN_POINT ( 'NONE', ( -44.40633062024040356, 8.176566272779744793, -15.10588013400562168 ) ) ;
+#410 = VERTEX_POINT ( 'NONE', #2269 ) ;
+#411 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, 9.749999999999998224 ) ) ;
+#412 = ORIENTED_EDGE ( 'NONE', *, *, #1753, .T. ) ;
+#413 = EDGE_CURVE ( 'NONE', #1838, #119, #2246, .T. ) ;
+#414 = CARTESIAN_POINT ( 'NONE', ( -30.07457024476144269, 32.68049414076453729, -12.37283457400364739 ) ) ;
+#415 = CARTESIAN_POINT ( 'NONE', ( -32.80386453857610718, 33.68702801529958180, -9.627364753122026997 ) ) ;
+#416 = VERTEX_POINT ( 'NONE', #2061 ) ;
+#417 = CARTESIAN_POINT ( 'NONE', ( -36.91745922585356965, 29.92801760975707026, -6.957240472960338096 ) ) ;
+#418 = CARTESIAN_POINT ( 'NONE', ( -44.90380467601645620, 6.731651258784808967, 13.24295527983305654 ) ) ;
+#419 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1416, #2234, #315, #535 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 4.304835433566710279, 4.712388980384689674 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.9862061738295022639, 0.9862061738295022639, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#420 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#421 = CARTESIAN_POINT ( 'NONE', ( 40.14688991242650218, 20.54812567066736406, 6.169177601812957157 ) ) ;
+#422 = TOROIDAL_SURFACE ( 'NONE', #22, 4.499999999999996447, 1.000000000000000000 ) ;
+#423 = CARTESIAN_POINT ( 'NONE', ( 42.99999999999999289, 6.284924945759606807, 15.72182540694797659 ) ) ;
+#424 = EDGE_LOOP ( 'NONE', ( #2594, #2695 ) ) ;
+#425 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000000000, 12.50000000000000000 ) ) ;
+#426 = ORIENTED_EDGE ( 'NONE', *, *, #1714, .F. ) ;
+#427 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371286370, 44.36900610829383140, 12.50000000000000000 ) ) ;
+#428 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#429 = ADVANCED_FACE ( 'NONE', ( #2255 ), #2444, .F. ) ;
+#430 = CARTESIAN_POINT ( 'NONE', ( -32.16910211311316914, 32.80059666762780779, -13.85738956026218460 ) ) ;
+#431 = ORIENTED_EDGE ( 'NONE', *, *, #1942, .T. ) ;
+#432 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#433 = CARTESIAN_POINT ( 'NONE', ( -42.96469508733385823, 6.406112403811332179, 13.12865446755100507 ) ) ;
+#434 = EDGE_CURVE ( 'NONE', #1369, #119, #2100, .T. ) ;
+#435 = CARTESIAN_POINT ( 'NONE', ( 44.85957031200506862, 6.860130113013480191, 13.55895294178186106 ) ) ;
+#436 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#437 = FACE_OUTER_BOUND ( 'NONE', #1237, .T. ) ;
+#438 = DIRECTION ( 'NONE', ( 1.000000000000000000, -4.625929269271486900E-15, 0.000000000000000000 ) ) ;
+#439 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945760107740, 12.41000645670897207 ) ) ;
+#440 = VERTEX_POINT ( 'NONE', #1846 ) ;
+#441 = AXIS2_PLACEMENT_3D ( 'NONE', #1402, #2433, #1576 ) ;
+#442 = AXIS2_PLACEMENT_3D ( 'NONE', #2665, #303, #731 ) ;
+#443 = AXIS2_PLACEMENT_3D ( 'NONE', #480, #32, #230 ) ;
+#444 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, -12.50000000000000000 ) ) ;
+#445 = CARTESIAN_POINT ( 'NONE', ( -33.43771148342548827, 33.97047127824377100, -16.20971450554219828 ) ) ;
+#446 = ORIENTED_EDGE ( 'NONE', *, *, #979, .T. ) ;
+#447 = EDGE_CURVE ( 'NONE', #594, #851, #969, .T. ) ;
+#448 = CARTESIAN_POINT ( 'NONE', ( -43.68618486730048289, 10.26823195585920345, -12.92259534589628700 ) ) ;
+#449 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, 20.50000000000000000 ) ) ;
+#450 = ORIENTED_EDGE ( 'NONE', *, *, #138, .T. ) ;
+#451 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, -12.50000000000000000 ) ) ;
+#452 = CIRCLE ( 'NONE', #2730, 2.750000000000002665 ) ;
+#453 = LINE ( 'NONE', #64, #2418 ) ;
+#454 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371286370, 44.36900610829383140, 12.50000000000000000 ) ) ;
+#455 = CARTESIAN_POINT ( 'NONE', ( -43.13581435095201755, 11.86678491173436534, -11.42552890006263411 ) ) ;
+#456 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 4.500000000000000888 ) ) ;
+#457 = FACE_BOUND ( 'NONE', #2495, .T. ) ;
+#458 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#459 = CARTESIAN_POINT ( 'NONE', ( -32.29055821324739384, 33.06974159880335407, -14.26733026968242868 ) ) ;
+#460 = CARTESIAN_POINT ( 'NONE', ( 34.50846982257009188, 36.92493517148989923, 17.21047068398527102 ) ) ;
+#461 = ORIENTED_EDGE ( 'NONE', *, *, #1610, .T. ) ;
+#462 = CARTESIAN_POINT ( 'NONE', ( -36.17477690138717605, 29.71314995735622944, -7.142824459707864726 ) ) ;
+#463 = CARTESIAN_POINT ( 'NONE', ( -43.08988301295879353, 12.00019263240737644, 14.48836083989945855 ) ) ;
+#464 = CARTESIAN_POINT ( 'NONE', ( 44.89078164496277878, 6.769476694862786914, 13.34854322613787581 ) ) ;
+#465 = LINE ( 'NONE', #77, #2196 ) ;
+#466 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#467 = ADVANCED_FACE ( 'NONE', ( #510 ), #947, .F. ) ;
+#468 = EDGE_LOOP ( 'NONE', ( #1, #835, #755, #1641 ) ) ;
+#469 = VECTOR ( 'NONE', #1579, 1000.000000000000000 ) ;
+#470 =( NAMED_UNIT ( * ) SI_UNIT ( $, .STERADIAN. ) SOLID_ANGLE_UNIT ( ) );
+#471 = CARTESIAN_POINT ( 'NONE', ( -32.60311442061208709, 42.45904613731469368, 9.619517159301437914 ) ) ;
+#472 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#473 = CARTESIAN_POINT ( 'NONE', ( 43.67474508509675246, 10.30145884050452310, 11.70830923837393200 ) ) ;
+#474 = CARTESIAN_POINT ( 'NONE', ( -34.67425274517010081, 33.65279005882512564, -17.21963221568602975 ) ) ;
+#475 = CARTESIAN_POINT ( 'NONE', ( -34.73009560828618447, 34.67215538705777078, 17.30582513608350581 ) ) ;
+#476 = ORIENTED_EDGE ( 'NONE', *, *, #935, .F. ) ;
+#477 = CARTESIAN_POINT ( 'NONE', ( -31.94357522606386013, 37.26341713563340363, -13.02528983019722730 ) ) ;
+#478 = APPLICATION_CONTEXT ( 'automotive_design' ) ;
+#479 = CARTESIAN_POINT ( 'NONE', ( -32.53579838877607955, 33.42734138321499415, -10.12951273671701813 ) ) ;
+#480 = CARTESIAN_POINT ( 'NONE', ( -33.03257378525533738, 31.99619020741757680, 4.500000000000000888 ) ) ;
+#481 = FACE_OUTER_BOUND ( 'NONE', #2087, .T. ) ;
+#482 = VECTOR ( 'NONE', #1660, 1000.000000000000000 ) ;
+#483 = CARTESIAN_POINT ( 'NONE', ( -43.00000000007026557, 12.26125813586037872, -12.25525971688855975 ) ) ;
+#484 = VERTEX_POINT ( 'NONE', #2672 ) ;
+#485 = EDGE_CURVE ( 'NONE', #1459, #2587, #1765, .T. ) ;
+#486 = CARTESIAN_POINT ( 'NONE', ( -35.80901780882527419, 33.14748931286850109, -18.45029627805127959 ) ) ;
+#487 = CARTESIAN_POINT ( 'NONE', ( -32.15342044700667401, 43.76518374714815707, 10.81466330593661240 ) ) ;
+#488 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732933493, -7.324346477178758974 ) ) ;
+#489 = CARTESIAN_POINT ( 'NONE', ( 44.07774513821160411, 9.130943874690144568, 15.75167402737496403 ) ) ;
+#490 = AXIS2_PLACEMENT_3D ( 'NONE', #456, #660, #1528 ) ;
+#491 = CARTESIAN_POINT ( 'NONE', ( -32.25272515909306748, 33.00465678385931056, -14.16221685464599034 ) ) ;
+#492 = CARTESIAN_POINT ( 'NONE', ( -36.06038663569781022, 30.10580538338211909, 17.85684972154064454 ) ) ;
+#493 = AXIS2_PLACEMENT_3D ( 'NONE', #858, #2357, #1288 ) ;
+#494 = CARTESIAN_POINT ( 'NONE', ( -30.10500962285524906, 32.75500483102697302, -13.14968065242663897 ) ) ;
+#495 = CARTESIAN_POINT ( 'NONE', ( -32.16915092262876641, 32.80074319802087501, -11.14240224248994338 ) ) ;
+#496 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#497 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#498 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -20.50000000000000000 ) ) ;
+#499 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 15.00000000000000000, -0.5000000000000004441 ) ) ;
+#500 = AXIS2_PLACEMENT_3D ( 'NONE', #267, #713, #1126 ) ;
+#501 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#502 = CARTESIAN_POINT ( 'NONE', ( -36.78005168385927703, 27.63548691358504072, 7.000000000000001776 ) ) ;
+#503 = CARTESIAN_POINT ( 'NONE', ( 43.29723134155775455, 11.39794873719436552, 13.99145351984449270 ) ) ;
+#504 = EDGE_CURVE ( 'NONE', #1677, #1315, #2220, .T. ) ;
+#505 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -13.75537618510426086 ) ) ;
+#506 = CARTESIAN_POINT ( 'NONE', ( -44.53214698414789297, 7.811132234350179893, 14.79092204521037957 ) ) ;
+#507 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#508 = CARTESIAN_POINT ( 'NONE', ( -34.62068687147460366, 33.69417232600314094, -7.812778574186847180 ) ) ;
+#509 = CARTESIAN_POINT ( 'NONE', ( 40.72182540694797126, 14.10497510537461352, 7.000000000000000888 ) ) ;
+#510 = FACE_OUTER_BOUND ( 'NONE', #340, .T. ) ;
+#511 = ORIENTED_EDGE ( 'NONE', *, *, #1105, .F. ) ;
+#512 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #100, #1363, #2441 ),
+ ( #1155, #944, #308 ),
+ ( #1806, #1387, #529 ),
+ ( #2243, #2389, #2272 ),
+ ( #351, #2302, #1435 ),
+ ( #2509, #1683, #2123 ),
+ ( #2287, #1882, #2732 ),
+ ( #145, #2535, #1046 ),
+ ( #2479, #392, #365 ),
+ ( #615, #1236, #999 ),
+ ( #1031, #1223, #190 ),
+ ( #1465, #984, #380 ),
+ ( #832, #587, #2715 ),
+ ( #1669, #161, #2093 ),
+ ( #2078, #1898, #801 ),
+ ( #817, #783, #1014 ),
+ ( #409, #2491, #1479 ),
+ ( #2521, #2317, #1209 ),
+ ( #2331, #2745, #767 ),
+ ( #1655, #174, #1449 ),
+ ( #2065, #602, #1249 ),
+ ( #1837, #2108, #1263 ),
+ ( #1850, #2689, #1625 ),
+ ( #558, #2702, #1417 ),
+ ( #1638, #573, #1865 ),
+ ( #659, #455, #1494 ),
+ ( #2402, #690, #246 ),
+ ( #1727, #2550, #2360 ),
+ ( #216, #483, #1059 ),
+ ( #51, #1525, #1549 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 4.304835433566708502, 4.552129167768318219, 4.799422901969927935, 5.046716636171537651, 5.294010370373147367, 5.541304104574757083, 5.664950971675562386, 5.788597838776366800, 5.912244705877171214, 5.974068139427574309, 6.035891572977976516, 6.097715006528378723, 6.159538440078781818, 6.221361873629184025, 6.283185307179586232 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.8290880361187457082, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8075124374855047193, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7839358397903772868, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7332210067122884034, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7060804060492770207, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6487707199110942513, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6185992827454469545, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5559131927632718906, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5233918139855477936, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4569611680055477576, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4230301067180500252, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3722889375816920698, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3554073528898792911, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3220670159444026237, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3056035870504272278, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2736703402485362036, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2581920883499866926, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2364545543615540202, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2294594316545695289, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2161570347704336315, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2098496721007437893, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1981596860163650220, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1927776193291231566, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1832210536906958565, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1790482828115478875, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1722066456629600117, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1695406270279364858, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1659522367849540214, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650295672437424033, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650323245415012685, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#513 = CARTESIAN_POINT ( 'NONE', ( -32.27750898416690006, 43.40476818712910756, 10.40559821995241663 ) ) ;
+#514 = CARTESIAN_POINT ( 'NONE', ( -32.00351250389886104, 32.31169563575704018, -12.72592212208823526 ) ) ;
+#515 = CIRCLE ( 'NONE', #1368, 2.750000000000002665 ) ;
+#516 = CARTESIAN_POINT ( 'NONE', ( -43.51720173353851351, 10.75904401780500486, 13.24358781161986798 ) ) ;
+#517 = EDGE_CURVE ( 'NONE', #383, #416, #1603, .T. ) ;
+#518 = CARTESIAN_POINT ( 'NONE', ( -30.14881835579600633, 32.86308127774037757, -13.52570216091755029 ) ) ;
+#519 = EDGE_CURVE ( 'NONE', #652, #1700, #958, .T. ) ;
+#520 = CARTESIAN_POINT ( 'NONE', ( -33.51941604685471532, 33.99137022734336000, -8.696963810950791185 ) ) ;
+#521 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, -0.5000000000000004441 ) ) ;
+#522 = FACE_OUTER_BOUND ( 'NONE', #804, .T. ) ;
+#523 = ORIENTED_EDGE ( 'NONE', *, *, #1994, .F. ) ;
+#524 = ORIENTED_EDGE ( 'NONE', *, *, #2043, .T. ) ;
+#525 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000147793, 44.21079393475268660, 12.10821283959214867 ) ) ;
+#526 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#527 = CARTESIAN_POINT ( 'NONE', ( -33.12690805908247427, 40.17524856008518697, 15.85494385505739245 ) ) ;
+#528 = ADVANCED_FACE ( 'NONE', ( #522 ), #1587, .F. ) ;
+#529 = CARTESIAN_POINT ( 'NONE', ( -36.17477690138199620, 29.71314995735627562, -17.85717554029130127 ) ) ;
+#530 = CARTESIAN_POINT ( 'NONE', ( -31.95322838119606956, 35.56626065605323106, -11.60005878369882204 ) ) ;
+#531 = CARTESIAN_POINT ( 'NONE', ( -35.24939283215580588, 32.88961845997135214, -7.480968100045668479 ) ) ;
+#532 = AXIS2_PLACEMENT_3D ( 'NONE', #933, #90, #2639 ) ;
+#533 = CARTESIAN_POINT ( 'NONE', ( -35.49711784373716483, 33.72827315773044887, -17.64949409743674735 ) ) ;
+#534 = ADVANCED_FACE ( 'NONE', ( #1378 ), #2601, .T. ) ;
+#535 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, -17.99999999999999645 ) ) ;
+#536 = ORIENTED_EDGE ( 'NONE', *, *, #1390, .T. ) ;
+#537 = CARTESIAN_POINT ( 'NONE', ( -41.32601150491552033, 17.12336316791691360, 6.657585757108619440 ) ) ;
+#538 = CIRCLE ( 'NONE', #2110, 1.750000000000000888 ) ;
+#539 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#540 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#541 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#542 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#543 = CARTESIAN_POINT ( 'NONE', ( 43.29946974291465267, 11.39144729317238713, 13.87817639135612779 ) ) ;
+#544 = EDGE_CURVE ( 'NONE', #839, #1281, #706, .T. ) ;
+#545 = CARTESIAN_POINT ( 'NONE', ( -31.94490745452773695, 34.79553597278510324, -8.587371876224860756 ) ) ;
+#546 = CARTESIAN_POINT ( 'NONE', ( 40.85494385521288052, 13.64803341803775005, 16.87309194081363017 ) ) ;
+#547 = ORIENTED_EDGE ( 'NONE', *, *, #632, .F. ) ;
+#548 = SHAPE_DEFINITION_REPRESENTATION ( #2627, #781 ) ;
+#549 = CARTESIAN_POINT ( 'NONE', ( -44.89078164496267220, 6.769476694863037380, 11.65145677386212597 ) ) ;
+#550 = VERTEX_POINT ( 'NONE', #2617 ) ;
+#551 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#552 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#553 = LINE ( 'NONE', #1665, #2156 ) ;
+#554 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000000000, 12.50000000000000000 ) ) ;
+#555 = CARTESIAN_POINT ( 'NONE', ( -35.49391820253964624, 33.72397523779684292, -18.35380280381703955 ) ) ;
+#556 = CARTESIAN_POINT ( 'NONE', ( 43.65830555055399032, 10.34920752207618122, 12.54874507021277807 ) ) ;
+#557 = CARTESIAN_POINT ( 'NONE', ( -34.83188052505340693, 34.42191729793909616, -6.876150094621122477 ) ) ;
+#558 = CARTESIAN_POINT ( 'NONE', ( -44.85875296978372972, 6.862504086155303717, -13.56700365951409815 ) ) ;
+#559 = ORIENTED_EDGE ( 'NONE', *, *, #1267, .T. ) ;
+#560 = CARTESIAN_POINT ( 'NONE', ( -43.65830555055534745, 10.34920752207009009, 12.54874507020919161 ) ) ;
+#561 = EDGE_LOOP ( 'NONE', ( #2182, #2288, #2538, #1965 ) ) ;
+#562 = ORIENTED_EDGE ( 'NONE', *, *, #544, .T. ) ;
+#563 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 17.99999999999999645 ) ) ;
+#564 = EDGE_CURVE ( 'NONE', #1962, #887, #912, .T. ) ;
+#565 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#566 = ORIENTED_EDGE ( 'NONE', *, *, #1427, .F. ) ;
+#567 = CARTESIAN_POINT ( 'NONE', ( -44.80934957045266742, 7.005996420292145643, -11.16968940606473737 ) ) ;
+#568 = CARTESIAN_POINT ( 'NONE', ( -32.51580311285691494, 35.24081814342464014, -14.90728475592333346 ) ) ;
+#569 = AXIS2_PLACEMENT_3D ( 'NONE', #1087, #2180, #1524 ) ;
+#570 = CIRCLE ( 'NONE', #493, 1.750000000000000888 ) ;
+#571 = CARTESIAN_POINT ( 'NONE', ( 43.51720173353851351, 10.75904401780432806, 11.75641218838013202 ) ) ;
+#572 = CARTESIAN_POINT ( 'NONE', ( 33.12690805908301428, 40.17524856008519407, 9.145056144941799303 ) ) ;
+#573 = CARTESIAN_POINT ( 'NONE', ( -43.24436591354658077, 11.55149654489924238, -11.18404672621899110 ) ) ;
+#574 = ORIENTED_EDGE ( 'NONE', *, *, #651, .T. ) ;
+#575 = CARTESIAN_POINT ( 'NONE', ( -44.27828862574268243, 8.548464662501777056, 9.623516448636951992 ) ) ;
+#576 = EDGE_LOOP ( 'NONE', ( #360, #1550 ) ) ;
+#577 = ORIENTED_EDGE ( 'NONE', *, *, #1892, .F. ) ;
+#578 = PLANE ( 'NONE', #1040 ) ;
+#579 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#580 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 10.00000000000000000, 4.500000000000000888 ) ) ;
+#581 = EDGE_CURVE ( 'NONE', #254, #1520, #709, .T. ) ;
+#582 = EDGE_LOOP ( 'NONE', ( #577, #164 ) ) ;
+#583 = CARTESIAN_POINT ( 'NONE', ( -42.92657441282921127, 6.536965231181891767, -11.59715074964191750 ) ) ;
+#584 = CARTESIAN_POINT ( 'NONE', ( -30.62935254936612139, 33.79555764238799043, -14.82952189107471597 ) ) ;
+#585 = CARTESIAN_POINT ( 'NONE', ( 43.08855886698587057, 12.00403861870643496, 13.39448169393815036 ) ) ;
+#586 = CARTESIAN_POINT ( 'NONE', ( 40.25632870800386343, 15.70283663275118258, 7.726926205486392973 ) ) ;
+#587 = CARTESIAN_POINT ( 'NONE', ( -43.26390430068227033, 11.49474723588365599, -14.04725606519312997 ) ) ;
+#588 = EDGE_LOOP ( 'NONE', ( #1018, #1468, #2109, #616, #338 ) ) ;
+#589 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1849, #114, #970, #983 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 0.000000000000000000, 1.570796326794896780 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#590 = ORIENTED_EDGE ( 'NONE', *, *, #23, .F. ) ;
+#591 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#592 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#593 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371285660, 6.610463800732987671, 12.50000000000000000 ) ) ;
+#594 = VERTEX_POINT ( 'NONE', #1568 ) ;
+#595 = EDGE_CURVE ( 'NONE', #290, #373, #2051, .T. ) ;
+#596 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945760252513, -12.38665702615338127 ) ) ;
+#597 = CARTESIAN_POINT ( 'NONE', ( -43.00000000005574918, 12.26125813590357794, 12.30585011635637116 ) ) ;
+#598 = EDGE_CURVE ( 'NONE', #2761, #2543, #894, .T. ) ;
+#599 = VECTOR ( 'NONE', #2655, 1000.000000000000000 ) ;
+#600 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371287791, 6.610463800732935269, 12.39364399429249808 ) ) ;
+#601 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759544634, -12.50000000000000000 ) ) ;
+#602 = CARTESIAN_POINT ( 'NONE', ( -43.55646050725455609, 10.64501678178302768, -11.20697888771249318 ) ) ;
+#603 = VERTEX_POINT ( 'NONE', #2603 ) ;
+#604 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#605 = ORIENTED_EDGE ( 'NONE', *, *, #1406, .F. ) ;
+#606 = FACE_OUTER_BOUND ( 'NONE', #468, .T. ) ;
+#607 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, 4.500000000000000888 ) ) ;
+#608 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#609 = EDGE_LOOP ( 'NONE', ( #1502, #1578, #2130, #2084 ) ) ;
+#610 = CARTESIAN_POINT ( 'NONE', ( -41.85058052292311004, 10.23041621433557680, -9.127488232614899388 ) ) ;
+#611 = CARTESIAN_POINT ( 'NONE', ( -44.89078164496277168, 6.769476694862777144, 13.34854322613788291 ) ) ;
+#612 = CARTESIAN_POINT ( 'NONE', ( 42.96469508733384401, 6.406112403811317968, 13.12865446755109033 ) ) ;
+#613 = CARTESIAN_POINT ( 'NONE', ( 32.55641871737685733, 42.13350728233953646, 10.06266836556244826 ) ) ;
+#614 = ADVANCED_FACE ( 'NONE', ( #1124 ), #1523, .F. ) ;
+#615 = CARTESIAN_POINT ( 'NONE', ( -42.46419766629936277, 13.81749775803288749, -17.63686523080132673 ) ) ;
+#616 = ORIENTED_EDGE ( 'NONE', *, *, #952, .T. ) ;
+#617 = AXIS2_PLACEMENT_3D ( 'NONE', #1037, #197, #2139 ) ;
+#618 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#619 = VECTOR ( 'NONE', #496, 1000.000000000000000 ) ;
+#620 = VERTEX_POINT ( 'NONE', #2631 ) ;
+#621 = CARTESIAN_POINT ( 'NONE', ( -30.10461952914602080, 32.75404730845718149, -11.85447970338051071 ) ) ;
+#622 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#623 = ORIENTED_EDGE ( 'NONE', *, *, #1801, .F. ) ;
+#624 = CARTESIAN_POINT ( 'NONE', ( -43.26390430068434512, 11.49474723587444558, -10.95274393480526243 ) ) ;
+#625 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 12.26125813606935644, 12.49999999999993783 ) ) ;
+#626 = CIRCLE ( 'NONE', #1611, 2.750000000000002665 ) ;
+#627 = FACE_OUTER_BOUND ( 'NONE', #2486, .T. ) ;
+#628 = CARTESIAN_POINT ( 'NONE', ( 32.27750898416623215, 43.40476818713106866, 10.40559821995239886 ) ) ;
+#629 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, 8.000000000000003553 ) ) ;
+#630 = VERTEX_POINT ( 'NONE', #1748 ) ;
+#631 = VECTOR ( 'NONE', #350, 1000.000000000000000 ) ;
+#632 = EDGE_CURVE ( 'NONE', #2068, #2620, #2635, .T. ) ;
+#633 = CARTESIAN_POINT ( 'NONE', ( -10.88337182448036522, 14.37644341801383874, 79.70475956827036157 ) ) ;
+#634 = ORIENTED_EDGE ( 'NONE', *, *, #1189, .F. ) ;
+#635 = ADVANCED_FACE ( 'NONE', ( #1976 ), #56, .F. ) ;
+#636 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, -0.4999999999999935052 ) ) ;
+#637 = CARTESIAN_POINT ( 'NONE', ( -33.72269196666496782, 34.00889032105771292, -8.494423585908723595 ) ) ;
+#638 = CARTESIAN_POINT ( 'NONE', ( -31.20145246549144957, 34.38444387679342640, -15.65651356072780764 ) ) ;
+#639 = ORIENTED_EDGE ( 'NONE', *, *, #2247, .T. ) ;
+#640 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, -0.000000000000000000 ) ) ;
+#641 = ORIENTED_EDGE ( 'NONE', *, *, #963, .F. ) ;
+#642 = CARTESIAN_POINT ( 'NONE', ( 42.91187591077665786, 6.587419232237142985, 13.48473243242447417 ) ) ;
+#643 = LINE ( 'NONE', #1508, #1246 ) ;
+#644 = CIRCLE ( 'NONE', #2747, 0.9999999999999987788 ) ;
+#645 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759544634, 12.50000000000000000 ) ) ;
+#646 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 7.000000000000004441 ) ) ;
+#647 = ORIENTED_EDGE ( 'NONE', *, *, #1938, .F. ) ;
+#648 = ADVANCED_FACE ( 'NONE', ( #481 ), #1784, .F. ) ;
+#649 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#650 = CARTESIAN_POINT ( 'NONE', ( -34.53780195468802816, 34.63303889978092087, -17.14300095962763848 ) ) ;
+#651 = EDGE_CURVE ( 'NONE', #1698, #116, #1073, .T. ) ;
+#652 = VERTEX_POINT ( 'NONE', #2359 ) ;
+#653 = FACE_BOUND ( 'NONE', #655, .T. ) ;
+#654 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#655 = EDGE_LOOP ( 'NONE', ( #2445, #547 ) ) ;
+#656 = CARTESIAN_POINT ( 'NONE', ( 43.29946974291509321, 11.39144729316962135, 11.12182360864258435 ) ) ;
+#657 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -20.50000000000000000 ) ) ;
+#658 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#659 = CARTESIAN_POINT ( 'NONE', ( -44.91242457837373792, 6.706614724335260291, -13.16873016854403744 ) ) ;
+#660 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#661 = AXIS2_PLACEMENT_3D ( 'NONE', #202, #1518, #405 ) ;
+#662 = AXIS2_PLACEMENT_3D ( 'NONE', #1728, #1900, #1511 ) ;
+#663 = CARTESIAN_POINT ( 'NONE', ( -30.93292422953481591, 34.15495047542153628, -15.31221607047747568 ) ) ;
+#664 = CIRCLE ( 'NONE', #532, 1.000000000000000888 ) ;
+#665 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, -12.50000000000000000 ) ) ;
+#666 = CARTESIAN_POINT ( 'NONE', ( -32.00804062969424280, 35.49900831481810570, -13.60042106215898627 ) ) ;
+#667 = CARTESIAN_POINT ( 'NONE', ( 44.27828862574268243, 8.548464662501828570, 15.37648355136304801 ) ) ;
+#668 = ORIENTED_EDGE ( 'NONE', *, *, #1482, .T. ) ;
+#669 = CARTESIAN_POINT ( 'NONE', ( -38.49829738906406362, 25.33646801997567977, -6.984734518072002629 ) ) ;
+#670 = CARTESIAN_POINT ( 'NONE', ( -43.64736563080817433, 10.38098255457813046, 11.49219709530480849 ) ) ;
+#671 = ADVANCED_FACE ( 'NONE', ( #2194 ), #1756, .F. ) ;
+#672 = EDGE_LOOP ( 'NONE', ( #169, #1160, #2383, #805 ) ) ;
+#673 = CARTESIAN_POINT ( 'NONE', ( 43.08855886698517423, 12.00403861870570665, 11.60551830606362600 ) ) ;
+#674 = CIRCLE ( 'NONE', #406, 5.499999999999994671 ) ;
+#675 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759544634, 12.50000000000000000 ) ) ;
+#676 = LINE ( 'NONE', #2624, #53 ) ;
+#677 = DIRECTION ( 'NONE', ( 0.9422632794457278527, -0.3348729792147797646, 0.000000000000000000 ) ) ;
+#678 = AXIS2_PLACEMENT_3D ( 'NONE', #821, #789, #2498 ) ;
+#679 = ORIENTED_EDGE ( 'NONE', *, *, #1889, .T. ) ;
+#680 = CARTESIAN_POINT ( 'NONE', ( -30.33456232408972042, 33.33254759904097142, -14.24943462682488615 ) ) ;
+#681 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.21079393477482000, 12.49999999999999822 ) ) ;
+#682 = CARTESIAN_POINT ( 'NONE', ( -31.62984376346780735, 36.62285101022552425, -11.55718020732114226 ) ) ;
+#683 = CARTESIAN_POINT ( 'NONE', ( 43.08988301295705980, 12.00019263241546774, 14.48836083988715195 ) ) ;
+#684 = CARTESIAN_POINT ( 'NONE', ( -33.72389765545759133, 34.00869459316927390, -16.50659491195139950 ) ) ;
+#685 = CARTESIAN_POINT ( 'NONE', ( -37.59468065372130496, 27.96102576855722432, 19.00000000000000000 ) ) ;
+#686 = EDGE_CURVE ( 'NONE', #1264, #387, #2762, .T. ) ;
+#687 = CARTESIAN_POINT ( 'NONE', ( -30.69783405021556177, 33.88365275426978940, -10.05477273901099089 ) ) ;
+#688 = LINE ( 'NONE', #1289, #619 ) ;
+#689 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#690 = CARTESIAN_POINT ( 'NONE', ( -43.08759061399217671, 12.00685091267252069, -11.59765743339665711 ) ) ;
+#691 = EDGE_CURVE ( 'NONE', #1281, #1380, #2274, .T. ) ;
+#692 = ORIENTED_EDGE ( 'NONE', *, *, #2043, .F. ) ;
+#693 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#694 = CARTESIAN_POINT ( 'NONE', ( -36.74416741499855021, 30.43134423836137259, 6.169177601812672940 ) ) ;
+#695 = CARTESIAN_POINT ( 'NONE', ( -40.62089237294635069, 19.17138398148065903, -4.480948801707161699 ) ) ;
+#696 = CARTESIAN_POINT ( 'NONE', ( 33.35637253533320745, 39.38759000239073060, 16.13454712838523264 ) ) ;
+#697 = CARTESIAN_POINT ( 'NONE', ( -33.54139374628734060, 33.99473049945354575, -16.32622377373466449 ) ) ;
+#698 = CARTESIAN_POINT ( 'NONE', ( -33.59905643722286328, 39.56632930884550348, 16.41383903443793457 ) ) ;
+#699 = VERTEX_POINT ( 'NONE', #78 ) ;
+#700 = CARTESIAN_POINT ( 'NONE', ( -30.07465626233681633, 32.68070413098902094, -12.63213340734007595 ) ) ;
+#701 = CARTESIAN_POINT ( 'NONE', ( -32.11434084504631414, 34.86669446324285104, -8.434407110591786605 ) ) ;
+#702 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688396697, 32.64726791736432432, -7.450487090025976755 ) ) ;
+#703 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#704 = CARTESIAN_POINT ( 'NONE', ( 32.17590843201791984, 43.43964489217512437, 13.92605412574600798 ) ) ;
+#705 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#706 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1552, #695, #488, #2407 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 4.304835433566713832, 6.283185307179586232 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.6995862986750142065, 0.6995862986750142065, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#707 = CARTESIAN_POINT ( 'NONE', ( -32.80647276483281871, 34.99140825999616311, -17.07639056697697555 ) ) ;
+#708 = CARTESIAN_POINT ( 'NONE', ( -35.24008933272887134, 34.79994392319321861, 7.395706311578155834 ) ) ;
+#709 = LINE ( 'NONE', #1344, #1918 ) ;
+#710 = AXIS2_PLACEMENT_3D ( 'NONE', #334, #542, #1193 ) ;
+#711 = CARTESIAN_POINT ( 'NONE', ( 44.36934426592475234, 8.283993259259004205, 15.18396257553581563 ) ) ;
+#712 = AXIS2_PLACEMENT_3D ( 'NONE', #1069, #654, #2146 ) ;
+#713 = DIRECTION ( 'NONE', ( 0.1974601447941596311, -0.5015927567843166957, 0.8422672957911752079 ) ) ;
+#714 = CARTESIAN_POINT ( 'NONE', ( -42.74090254271485634, 13.01380754396807227, 17.39701427642247822 ) ) ;
+#715 = CARTESIAN_POINT ( 'NONE', ( -31.99991003093284192, 37.38075727824945460, -12.35973869815139103 ) ) ;
+#716 = CARTESIAN_POINT ( 'NONE', ( -34.46299344196333436, 34.63959879037075495, -7.029990670834037836 ) ) ;
+#717 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#718 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, -20.50000000000000355 ) ) ;
+#719 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, 12.50000000000000000 ) ) ;
+#720 = ORIENTED_EDGE ( 'NONE', *, *, #1663, .T. ) ;
+#721 = EDGE_CURVE ( 'NONE', #1520, #2102, #220, .T. ) ;
+#722 = CARTESIAN_POINT ( 'NONE', ( -33.32773819346601130, 40.35437403084119268, 8.879475727009140229 ) ) ;
+#723 = CARTESIAN_POINT ( 'NONE', ( -33.70831313507061822, 39.24899287438046969, 4.460636945253258823 ) ) ;
+#724 = ADVANCED_FACE ( 'NONE', ( #2640 ), #1337, .F. ) ;
+#725 = CARTESIAN_POINT ( 'NONE', ( -44.77913493337637618, 7.093754932011590242, 13.97671189358419142 ) ) ;
+#726 = CARTESIAN_POINT ( 'NONE', ( -31.79596971711020004, 36.95827019245823664, -13.36200435939616504 ) ) ;
+#727 = CARTESIAN_POINT ( 'NONE', ( -30.43859957767574187, 33.50756392938859562, -10.53374068455000589 ) ) ;
+#728 = FACE_OUTER_BOUND ( 'NONE', #1106, .T. ) ;
+#729 = DIRECTION ( 'NONE', ( -1.000000000000000000, -0.000000000000000000, -0.000000000000000000 ) ) ;
+#730 = ORIENTED_EDGE ( 'NONE', *, *, #80, .T. ) ;
+#731 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#732 = CARTESIAN_POINT ( 'NONE', ( -32.04197879035449859, 44.08886640157222558, 11.71524737660623927 ) ) ;
+#733 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#734 = AXIS2_PLACEMENT_3D ( 'NONE', #1123, #96, #2223 ) ;
+#735 = CARTESIAN_POINT ( 'NONE', ( 1.750000000000000888, 24.99999999999999645, 4.500000000000000888 ) ) ;
+#736 = EDGE_CURVE ( 'NONE', #603, #1459, #2233, .T. ) ;
+#737 = CARTESIAN_POINT ( 'NONE', ( -32.00335348115621770, 32.31123735782023942, -12.27853751794684634 ) ) ;
+#738 = CARTESIAN_POINT ( 'NONE', ( -33.44831640414376039, 35.07421879785913177, -8.767637688663361573 ) ) ;
+#739 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, -9.749999999999998224 ) ) ;
+#740 = CARTESIAN_POINT ( 'NONE', ( -35.10832082258161080, 33.14804689480647681, -17.45321775919601848 ) ) ;
+#741 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#742 = ORIENTED_EDGE ( 'NONE', *, *, #279, .F. ) ;
+#743 = PRODUCT_DEFINITION_CONTEXT ( 'detailed design', #1056, 'design' ) ;
+#744 = CARTESIAN_POINT ( 'NONE', ( -42.98233690606030422, 6.345555189378163874, 12.05046592392595528 ) ) ;
+#745 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#746 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, 4.500000000000000888 ) ) ;
+#747 = AXIS2_PLACEMENT_3D ( 'NONE', #1666, #1260, #1919 ) ;
+#748 = AXIS2_PLACEMENT_3D ( 'NONE', #384, #1227, #2098 ) ;
+#749 = ADVANCED_FACE ( 'NONE', ( #1985, #1509, #437 ), #1291, .T. ) ;
+#750 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000000000, -0.5000000000000004441 ) ) ;
+#751 = FILL_AREA_STYLE ('',( #778 ) ) ;
+#752 = VECTOR ( 'NONE', #2661, 1000.000000000000114 ) ;
+#753 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -15.25000000000000355 ) ) ;
+#754 = CARTESIAN_POINT ( 'NONE', ( 40.14688991242804406, 20.54812567066288764, 18.83082239818627812 ) ) ;
+#755 = ORIENTED_EDGE ( 'NONE', *, *, #1267, .F. ) ;
+#756 = CARTESIAN_POINT ( 'NONE', ( -38.21994831614073718, 22.69290528549491270, 7.000000000000003553 ) ) ;
+#757 = VECTOR ( 'NONE', #1894, 1000.000000000000000 ) ;
+#758 = VERTEX_POINT ( 'NONE', #454 ) ;
+#759 = CYLINDRICAL_SURFACE ( 'NONE', #377, 2.750000000000002665 ) ;
+#760 = CARTESIAN_POINT ( 'NONE', ( -32.33154110754893651, 43.24783170891804929, 14.42725225151876955 ) ) ;
+#761 = CARTESIAN_POINT ( 'NONE', ( -44.91242457837384450, 6.706614724334936106, -11.83126983145600342 ) ) ;
+#762 = CARTESIAN_POINT ( 'NONE', ( -35.22949827446942805, 32.91062772082233323, -7.490079767141366140 ) ) ;
+#763 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639386455, 32.97280677233783308, -18.46760616633292429 ) ) ;
+#764 = CARTESIAN_POINT ( 'NONE', ( 42.85920530509989845, 6.768216077038142764, 11.25047455158256859 ) ) ;
+#765 = CARTESIAN_POINT ( 'NONE', ( 15.54977303496059449, 10.28868360277136063, 4.500000000000000888 ) ) ;
+#766 = EDGE_CURVE ( 'NONE', #1947, #30, #86, .T. ) ;
+#767 = CARTESIAN_POINT ( 'NONE', ( -42.70483288625755591, 7.298114032308856913, -14.28116850774944702 ) ) ;
+#768 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #1029, #2106, #2687 ),
+ ( #600, #815, #2489 ),
+ ( #1463, #2465, #1848 ),
+ ( #1433, #143, #2048 ),
+ ( #2076, #585, #1247 ),
+ ( #172, #799, #997 ),
+ ( #378, #2673, #126 ),
+ ( #1622, #543, #158 ),
+ ( #2300, #1667, #188 ),
+ ( #1011, #982, #764 ),
+ ( #1044, #2713, #2519 ),
+ ( #1447, #2700, #2477 ),
+ ( #363, #780, #2315 ),
+ ( #2090, #2256, #335 ),
+ ( #1863, #556, #1415 ),
+ ( #1234, #2270, #1653 ),
+ ( #349, #571, #2285 ),
+ ( #2507, #215, #2520 ),
+ ( #1045, #2534, #2316 ),
+ ( #2077, #1909, #1897 ),
+ ( #2343, #1248, #1464 ),
+ ( #1682, #1434, #586 ),
+ ( #860, #391, #2107 ),
+ ( #421, #1222, #1276 ),
+ ( #2134, #844, #1924 ),
+ ( #1448, #1072, #2549 ),
+ ( #816, #5, #2286 ),
+ ( #1696, #2490, #1262 ),
+ ( #2714, #2731, #2301 ),
+ ( #408, #2122, #2757 ),
+ ( #204, #1058, #572 ),
+ ( #2744, #364, #159 ),
+ ( #1478, #379, #613 ),
+ ( #628, #1493, #831 ),
+ ( #1013, #1864, #1654 ),
+ ( #800, #2508, #1668 ),
+ ( #1235, #189, #1881 ),
+ ( #2330, #2092, #173 ) ),
+ .UNSPECIFIED., .F., .F., .F. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( -0.000000000000000000, 0.04908738521234051744, 0.09817477042468103487, 0.1472621556370215523, 0.1963495408493620697, 0.2945243112740431046, 0.3926990816987241395, 0.4908738521234051744, 0.5890486225480862092, 0.7853981633974482790, 0.9817477042468103487, 1.178097245096172418, 1.570796326794896558, 1.963495408493620697, 2.356194490192344837, 2.552544031041707129, 2.748893571891068976, 2.945243112740430824, 3.141592653589793116 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.1650323245415012685, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650312009201844454, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1656105720154912864, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1678868551765326789, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1695842193436412626, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1739978385179177800, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1767131491670237697, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1830235777788156992, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1866179000439102120, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1984960600878372672, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2078644858773363580, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2284507283937017852, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2396680134330792800, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2632145179655434597, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2755469356012947846, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3008525186329124579, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3138283910420504030, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3532598395141761660, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3801944580803622298, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4339434541708537818, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4607773684084630617, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5135864445221429708, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5395685366562826824, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6154803776386914560, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6633476856979385605, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7508658766751572511, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7905347754414473460, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8598216432931320430, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8894434017616511534, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9253744439781100839, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9359386994017486749, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9541292101921221569, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9617554693256525900, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9739896480940225532, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9785975666657328320, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9847468226704357841, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9862886620238880964, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9862881586313551052, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#769 = ORIENTED_EDGE ( 'NONE', *, *, #2202, .T. ) ;
+#770 = CARTESIAN_POINT ( 'NONE', ( -41.87309194091710651, 10.15314363899526739, 9.145056144941985821 ) ) ;
+#771 = ORIENTED_EDGE ( 'NONE', *, *, #1445, .T. ) ;
+#772 = ORIENTED_EDGE ( 'NONE', *, *, #1753, .F. ) ;
+#773 = CARTESIAN_POINT ( 'NONE', ( -33.95521765768089750, 38.53185788492605468, 4.303130558510118497 ) ) ;
+#774 = CARTESIAN_POINT ( 'NONE', ( 44.00000000000000000, 6.284924945759610360, 12.50000000000000000 ) ) ;
+#775 = AXIS2_PLACEMENT_3D ( 'NONE', #1483, #234, #1912 ) ;
+#776 = VERTEX_POINT ( 'NONE', #1970 ) ;
+#777 = FACE_OUTER_BOUND ( 'NONE', #2497, .T. ) ;
+#778 = FILL_AREA_STYLE_COLOUR ( '', #1828 ) ;
+#779 = CARTESIAN_POINT ( 'NONE', ( -32.85141556144905195, 35.18208530508464094, -15.47331317117085625 ) ) ;
+#780 = CARTESIAN_POINT ( 'NONE', ( 43.67474508509557296, 10.30145884050165250, 13.29169076162657248 ) ) ;
+#781 = ADVANCED_BREP_SHAPE_REPRESENTATION ( 'jsk_fetch_l515headmount', ( #1362, #442 ), #2540 ) ;
+#782 = CARTESIAN_POINT ( 'NONE', ( -31.33893166490059912, 34.47609613105670689, -9.190905035202046491 ) ) ;
+#783 = CARTESIAN_POINT ( 'NONE', ( -43.66728881046966393, 10.32311561441583336, -12.40537740076413442 ) ) ;
+#784 = EDGE_LOOP ( 'NONE', ( #446, #1217, #1690, #1049 ) ) ;
+#785 = EDGE_CURVE ( 'NONE', #1271, #219, #676, .T. ) ;
+#786 = CARTESIAN_POINT ( 'NONE', ( -40.17685806913497970, 20.46108306200392590, 7.477634347489793498 ) ) ;
+#787 = ORIENTED_EDGE ( 'NONE', *, *, #2553, .T. ) ;
+#788 = ORIENTED_EDGE ( 'NONE', *, *, #106, .T. ) ;
+#789 = DIRECTION ( 'NONE', ( 0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#790 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#791 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#792 = EDGE_LOOP ( 'NONE', ( #2094, #937, #2249, #2160, #1258 ) ) ;
+#793 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #872, #1116, #1376 ),
+ ( #495, #1335, #1585 ),
+ ( #2399, #1167, #63 ),
+ ( #2431, #47, #727 ),
+ ( #2452, #1574, #1364 ),
+ ( #2192, #530, #1596 ),
+ ( #2023, #2638, #687 ),
+ ( #2207, #1129, #1388 ),
+ ( #479, #956, #271 ),
+ ( #2244, #919, #1983 ),
+ ( #1967, #1769, #2610 ),
+ ( #2218, #1807, #2622 ),
+ ( #1557, #297, #701 ),
+ ( #520, #738, #1546 ),
+ ( #2414, #101, #1349 ),
+ ( #286, #932, #2660 ),
+ ( #1143, #2228, #1997 ),
+ ( #903, #1156, #76 ),
+ ( #2007, #1782, #2648 ),
+ ( #89, #1754, #716 ),
+ ( #508, #945, #1796 ),
+ ( #2442, #310, #2683 ),
+ ( #333, #1205, #2046 ),
+ ( #2474, #1608, #1401 ),
+ ( #2697, #2463, #994 ),
+ ( #762, #1413, #968 ),
+ ( #140, #2060, #1180 ),
+ ( #123, #980, #2254 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 0.009244993961633881860, 0.009677031696171775610, 0.01010906943070966763, 0.01054110716524755964, 0.01097314489978545166, 0.01183722036886123569, 0.01270129583793701972, 0.01356537130701280375, 0.01399740904155069576, 0.01442944677608858778, 0.01486148451062647979, 0.01529352224516437181, 0.01572555997970226382, 0.01615759771424015584 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.2168728904164866356, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2374361215246205692, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2575450339862633276, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2971226315443064592, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3165897502856311330, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3549966455741205285, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3739345513055724046, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4113252261681221711, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4297773352029153338, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4844208125641403795, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5199734647860911885, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5869155412020345963, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6185741957824533177, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6777655986561057500, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7044060086928554121, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7393498023838022126, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7502271050966030641, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7696309372632342827, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7782359754378944672, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7933528242876843883, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7998620367471896975, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8104716960234968903, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8146694414438047094, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8213301770714689365, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8237619531728652689, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8272414356351114328, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8283393696832602737, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8290880361187438208, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#794 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725332046475, 9.278174593052025187 ) ) ;
+#795 = CARTESIAN_POINT ( 'NONE', ( -31.95476396459450186, 35.56406957751627118, -13.40589334827961743 ) ) ;
+#796 = CIRCLE ( 'NONE', #1383, 10.00000000000000000 ) ;
+#797 = CIRCLE ( 'NONE', #1872, 1.750000000000000888 ) ;
+#798 = VECTOR ( 'NONE', #1100, 1000.000000000000000 ) ;
+#799 = CARTESIAN_POINT ( 'NONE', ( 43.16529390050403237, 11.78116146411267273, 13.64926868013135675 ) ) ;
+#800 = CARTESIAN_POINT ( 'NONE', ( 31.98742823517670431, 44.24730866661797535, 11.64916250785372398 ) ) ;
+#801 = CARTESIAN_POINT ( 'NONE', ( -42.23330461419605797, 8.916679505932222582, -15.31019820240221385 ) ) ;
+#802 = EDGE_LOOP ( 'NONE', ( #772, #1629, #2097, #1649 ) ) ;
+#803 = CARTESIAN_POINT ( 'NONE', ( -42.57530924775475967, 13.49477380810001215, 9.621967686061859482 ) ) ;
+#804 = EDGE_LOOP ( 'NONE', ( #1840, #639, #1278, #908, #1707 ) ) ;
+#805 = ORIENTED_EDGE ( 'NONE', *, *, #1735, .F. ) ;
+#806 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, 12.50000000000000000 ) ) ;
+#807 = ADVANCED_FACE ( 'NONE', ( #1145 ), #857, .F. ) ;
+#808 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371286370, 44.36900610829383140, 12.50000000000000000 ) ) ;
+#809 = AXIS2_PLACEMENT_3D ( 'NONE', #2434, #2651, #922 ) ;
+#810 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 6.284924945759599701, 12.50000000000000000 ) ) ;
+#811 = VECTOR ( 'NONE', #591, 1000.000000000000000 ) ;
+#812 = EDGE_LOOP ( 'NONE', ( #153, #788, #1034, #200 ) ) ;
+#813 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732930828, -12.50000000000000000 ) ) ;
+#814 = CARTESIAN_POINT ( 'NONE', ( -32.25687261167739450, 33.00434678836186464, -14.16464040109613975 ) ) ;
+#815 = CARTESIAN_POINT ( 'NONE', ( 43.00000000005574918, 12.26125813590289404, 12.69414988364373009 ) ) ;
+#816 = CARTESIAN_POINT ( 'NONE', ( 36.74416741499790362, 30.43134423836325553, 6.169177601812959821 ) ) ;
+#817 = CARTESIAN_POINT ( 'NONE', ( -44.29376301731546306, 8.503519241818372265, -15.34954841740972142 ) ) ;
+#818 = ORIENTED_EDGE ( 'NONE', *, *, #233, .F. ) ;
+#819 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 12.26125813606935644, 12.49999999999998046 ) ) ;
+#820 = ORIENTED_EDGE ( 'NONE', *, *, #595, .T. ) ;
+#821 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#822 = PLANE ( 'NONE', #1475 ) ;
+#823 = EDGE_CURVE ( 'NONE', #2551, #219, #626, .T. ) ;
+#824 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, 7.000000000000004441 ) ) ;
+#825 = ORIENTED_EDGE ( 'NONE', *, *, #1801, .T. ) ;
+#826 = LINE ( 'NONE', #1042, #1170 ) ;
+#827 = CARTESIAN_POINT ( 'NONE', ( -43.16529390050403237, 11.78116146411261767, 11.35073131986864325 ) ) ;
+#828 = EDGE_CURVE ( 'NONE', #2749, #776, #2062, .T. ) ;
+#829 = CIRCLE ( 'NONE', #2658, 1.750000000000000888 ) ;
+#830 = DIRECTION ( 'NONE', ( 0.000000000000000000, 1.000000000000000000, 0.000000000000000000 ) ) ;
+#831 = CARTESIAN_POINT ( 'NONE', ( 32.28090642499900298, 43.07922933215748884, 10.72781387842155354 ) ) ;
+#832 = CARTESIAN_POINT ( 'NONE', ( -43.58712382716773703, 10.55595506930863969, -16.48569572509164161 ) ) ;
+#833 = ORIENTED_EDGE ( 'NONE', *, *, #358, .T. ) ;
+#834 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725332046475, 15.72182540694797659 ) ) ;
+#835 = ORIENTED_EDGE ( 'NONE', *, *, #284, .F. ) ;
+#836 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#837 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, 12.50000000000000000 ) ) ;
+#838 = DIRECTION ( 'NONE', ( -0.1974601447941585486, 0.5015927567843139201, 0.8422672957911769842 ) ) ;
+#839 = VERTEX_POINT ( 'NONE', #1322 ) ;
+#840 = CARTESIAN_POINT ( 'NONE', ( -39.54431025431560442, 22.29832024870238527, -6.071177093122991586 ) ) ;
+#841 = DIRECTION ( 'NONE', ( -0.9422632794457279637, 0.3348729792147796536, 1.161824037028343345E-16 ) ) ;
+#842 = EDGE_CURVE ( 'NONE', #1516, #1956, #1572, .T. ) ;
+#843 = CARTESIAN_POINT ( 'NONE', ( 43.05645483273530516, 12.09728489066297463, 11.76217390973267563 ) ) ;
+#844 = CARTESIAN_POINT ( 'NONE', ( 39.33771734144060161, 22.89837003650888292, 7.131934116793090972 ) ) ;
+#845 = AXIS2_PLACEMENT_3D ( 'NONE', #265, #1990, #927 ) ;
+#846 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, 12.50000000000000000 ) ) ;
+#847 = ORIENTED_EDGE ( 'NONE', *, *, #243, .T. ) ;
+#848 = AXIS2_PLACEMENT_3D ( 'NONE', #2694, #1178, #966 ) ;
+#849 = CARTESIAN_POINT ( 'NONE', ( -43.01186382226980953, 12.22679962567521983, 12.88659521194966473 ) ) ;
+#850 = VECTOR ( 'NONE', #1984, 1000.000000000000000 ) ;
+#851 = VERTEX_POINT ( 'NONE', #1009 ) ;
+#852 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#853 = CARTESIAN_POINT ( 'NONE', ( -33.21412629083663859, 33.91520775805124543, -9.044031610458405979 ) ) ;
+#854 = ORIENTED_EDGE ( 'NONE', *, *, #1141, .T. ) ;
+#855 = CARTESIAN_POINT ( 'NONE', ( -40.14276169517789583, 16.09266616533440342, -7.655275379028670280 ) ) ;
+#856 = CARTESIAN_POINT ( 'NONE', ( -44.84138201281258063, 6.912958087210509639, 13.66377469286515556 ) ) ;
+#857 = TOROIDAL_SURFACE ( 'NONE', #1657, 8.000000000000000000, 5.000000000000000000 ) ;
+#858 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 4.500000000000000888 ) ) ;
+#859 = ADVANCED_FACE ( 'NONE', ( #1634 ), #1412, .F. ) ;
+#860 = CARTESIAN_POINT ( 'NONE', ( 41.32601150491565534, 17.12336316791656543, 6.657585757108477331 ) ) ;
+#861 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#862 = EDGE_CURVE ( 'NONE', #2543, #2197, #826, .T. ) ;
+#863 = DIRECTION ( 'NONE', ( 0.3223706153912043182, 0.9363265015356214693, -0.1391756762310144557 ) ) ;
+#864 = ORIENTED_EDGE ( 'NONE', *, *, #74, .F. ) ;
+#865 = B_SPLINE_CURVE_WITH_KNOTS ( 'NONE', 3,
+ ( #1836, #1208, #2248, #2688, #2271, #1821, #782, #127, #2064, #545, #104, #2674, #1612, #2457, #998, #1624, #1403, #557, #2257, #1637, #144, #1599 ),
+ .UNSPECIFIED., .F., .F.,
+ ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 0.000000000000000000, 0.001108078520948957554, 0.002216157041897915108, 0.002770196302372403968, 0.003324235562846892394, 0.003878274823321381255, 0.004432314083795870115, 0.005540392604744849570, 0.006648471125693828157, 0.007756549646642807612, 0.008864628167591787067 ),
+ .UNSPECIFIED. ) ;
+#866 = CARTESIAN_POINT ( 'NONE', ( -30.19021555168547977, 32.96587464041111559, -13.75994990573270904 ) ) ;
+#867 = CARTESIAN_POINT ( 'NONE', ( -32.25228189023766845, 33.00361171489560519, -10.83935851711716225 ) ) ;
+#868 = CARTESIAN_POINT ( 'NONE', ( -31.40252164865539442, 34.52806025565234904, -15.88782948103038173 ) ) ;
+#869 = EDGE_LOOP ( 'NONE', ( #1198, #2536, #825, #450, #2001, #1115, #1541, #574 ) ) ;
+#870 = CARTESIAN_POINT ( 'NONE', ( -36.87935591080537989, 30.03868881232924437, -6.168792543291592878 ) ) ;
+#871 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371264344, 6.610463800733539230, 12.60635600570750370 ) ) ;
+#872 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981658586, -11.24462381489573914 ) ) ;
+#873 = CYLINDRICAL_SURFACE ( 'NONE', #1342, 1.750000000000000888 ) ;
+#874 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#875 = AXIS2_PLACEMENT_3D ( 'NONE', #2380, #1513, #1312 ) ;
+#876 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, 0.000000000000000000 ) ) ;
+#877 = FACE_OUTER_BOUND ( 'NONE', #2628, .T. ) ;
+#878 = ORIENTED_EDGE ( 'NONE', *, *, #2226, .F. ) ;
+#879 = ADVANCED_FACE ( 'NONE', ( #777 ), #2681, .F. ) ;
+#880 = FILL_AREA_STYLE_COLOUR ( '', #1428 ) ;
+#881 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 4.500000000000000888 ) ) ;
+#882 = CARTESIAN_POINT ( 'NONE', ( -33.03631303454734081, 35.15647620981305010, -15.73909685935100988 ) ) ;
+#883 = ORIENTED_EDGE ( 'NONE', *, *, #228, .T. ) ;
+#884 = CARTESIAN_POINT ( 'NONE', ( -41.70775951432997886, 10.72066285937119190, -8.950894989271690250 ) ) ;
+#885 = CARTESIAN_POINT ( 'NONE', ( -36.80118201742375561, 30.26574514008666839, 18.06883033849386067 ) ) ;
+#886 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1811, #2466, #2663, #741 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 1.570796326794896780, 3.141592653589793116 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243650485, 0.8047378541243650485, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#887 = VERTEX_POINT ( 'NONE', #1831 ) ;
+#888 = PLANE ( 'NONE', #2103 ) ;
+#889 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#890 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -12.50000000000000000 ) ) ;
+#891 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#892 = ORIENTED_EDGE ( 'NONE', *, *, #785, .F. ) ;
+#893 = PRESENTATION_LAYER_ASSIGNMENT ( '', '', ( #221 ) ) ;
+#894 = CIRCLE ( 'NONE', #845, 2.750000000000002665 ) ;
+#895 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#896 = AXIS2_PLACEMENT_3D ( 'NONE', #1875, #2649, #717 ) ;
+#897 = CARTESIAN_POINT ( 'NONE', ( -33.35637253533406010, 39.38759000238735553, 8.865452871613927144 ) ) ;
+#898 = EDGE_CURVE ( 'NONE', #1729, #2555, #2727, .T. ) ;
+#899 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#900 = CARTESIAN_POINT ( 'NONE', ( 41.70300804589836474, 16.02837548772427567, 18.14090539351653319 ) ) ;
+#901 = CARTESIAN_POINT ( 'NONE', ( -34.92456918379070885, 33.40671433021112335, -17.36041369813036894 ) ) ;
+#902 = CARTESIAN_POINT ( 'NONE', ( -44.27828862574277480, 8.548464662501514155, 15.37648355136317413 ) ) ;
+#903 = CARTESIAN_POINT ( 'NONE', ( -34.24459611162955497, 33.90109078729228997, -8.065318095925460895 ) ) ;
+#904 = VECTOR ( 'NONE', #1275, 1000.000000000000000 ) ;
+#905 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#906 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#907 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#908 = ORIENTED_EDGE ( 'NONE', *, *, #434, .T. ) ;
+#909 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1679, #1043, #2546, #2368 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 0.000000000000000000, 1.570796326794897002 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#910 = CARTESIAN_POINT ( 'NONE', ( -31.34597063188975596, 34.48088066318003087, -15.81700846586263687 ) ) ;
+#911 = CARTESIAN_POINT ( 'NONE', ( -34.50846982257024109, 36.92493517149638649, 7.789529316019790706 ) ) ;
+#912 = LINE ( 'NONE', #2186, #1122 ) ;
+#913 = CARTESIAN_POINT ( 'NONE', ( 43.64736563081370235, 10.38098255457012797, 11.49219709529766931 ) ) ;
+#914 = PRODUCT_RELATED_PRODUCT_CATEGORY ( 'part', '', ( #1775 ) ) ;
+#915 = CARTESIAN_POINT ( 'NONE', ( -34.10232452170161110, 33.94812970446760403, -16.82672874840155686 ) ) ;
+#916 = CARTESIAN_POINT ( 'NONE', ( -41.13454712838691307, 12.68826868899491522, 16.64362746466510856 ) ) ;
+#917 = EDGE_CURVE ( 'NONE', #1642, #550, #452, .T. ) ;
+#918 = CARTESIAN_POINT ( 'NONE', ( -31.98801666359724649, 37.35592637510115566, -12.22287686505414506 ) ) ;
+#919 = CARTESIAN_POINT ( 'NONE', ( -32.37004627410350110, 35.27477152405436556, -10.39643848907219770 ) ) ;
+#920 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#921 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#922 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#923 = AXIS2_PLACEMENT_3D ( 'NONE', #2554, #1928, #618 ) ;
+#924 = ORIENTED_EDGE ( 'NONE', *, *, #1581, .T. ) ;
+#925 = CARTESIAN_POINT ( 'NONE', ( -34.23018503598186157, 34.76832575166653783, -17.87064825719161121 ) ) ;
+#926 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#927 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#928 = CARTESIAN_POINT ( 'NONE', ( 42.17307833103743775, 9.123411929629760664, 15.40598249488271598 ) ) ;
+#929 = CARTESIAN_POINT ( 'NONE', ( -32.10100602077799437, 32.59616665187411400, -13.56692531693406956 ) ) ;
+#930 = CARTESIAN_POINT ( 'NONE', ( -43.34545552388527057, 11.25788144268259039, 11.08747574047080420 ) ) ;
+#931 = CARTESIAN_POINT ( 'NONE', ( -31.94391093219630307, 37.26411557281593900, -11.97632202764112819 ) ) ;
+#932 = CARTESIAN_POINT ( 'NONE', ( -34.03702749253581317, 34.88494135999115997, -8.223235512571532624 ) ) ;
+#933 = CARTESIAN_POINT ( 'NONE', ( -31.00000000000000355, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#934 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#935 = EDGE_CURVE ( 'NONE', #1250, #373, #553, .T. ) ;
+#936 = CARTESIAN_POINT ( 'NONE', ( 32.15342044700667401, 43.76518374714815707, 14.18533669406338760 ) ) ;
+#937 = ORIENTED_EDGE ( 'NONE', *, *, #691, .T. ) ;
+#938 = ADVANCED_FACE ( 'NONE', ( #2473 ), #2031, .T. ) ;
+#939 = LINE ( 'NONE', #953, #71 ) ;
+#940 = AXIS2_PLACEMENT_3D ( 'NONE', #890, #1771, #49 ) ;
+#941 = CARTESIAN_POINT ( 'NONE', ( -32.06546185742052302, 32.49184667508445301, -11.62773158287541087 ) ) ;
+#942 = AXIS2_PLACEMENT_3D ( 'NONE', #2252, #1816, #1829 ) ;
+#943 = CARTESIAN_POINT ( 'NONE', ( -43.34254294013596365, 11.26634105166322719, 16.79537387900263568 ) ) ;
+#944 = CARTESIAN_POINT ( 'NONE', ( -36.40037353087242167, 31.42989469372711397, -17.95773641296150558 ) ) ;
+#945 = CARTESIAN_POINT ( 'NONE', ( -34.92177046683571717, 34.35795269972074806, -7.627011478124432919 ) ) ;
+#946 = LINE ( 'NONE', #2725, #2459 ) ;
+#947 = PLANE ( 'NONE', #441 ) ;
+#948 = FACE_OUTER_BOUND ( 'NONE', #2435, .T. ) ;
+#949 = AXIS2_PLACEMENT_3D ( 'NONE', #34, #6, #438 ) ;
+#950 = ADVANCED_FACE ( 'NONE', ( #2119, #653 ), #2131, .F. ) ;
+#951 = ORIENTED_EDGE ( 'NONE', *, *, #519, .T. ) ;
+#952 = EDGE_CURVE ( 'NONE', #116, #1698, #674, .T. ) ;
+#953 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, -20.50000000000000000 ) ) ;
+#954 = CARTESIAN_POINT ( 'NONE', ( -34.43307409746501691, 37.14392230042371068, 3.778127667654248789 ) ) ;
+#955 = CARTESIAN_POINT ( 'NONE', ( -32.01665394174994361, 32.34971285684899556, -12.05831618826564444 ) ) ;
+#956 = CARTESIAN_POINT ( 'NONE', ( -32.18121478833108995, 35.36173675890672286, -10.87020591212793086 ) ) ;
+#957 = CARTESIAN_POINT ( 'NONE', ( 1.750000000000000888, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#958 = LINE ( 'NONE', #1809, #347 ) ;
+#959 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000000000, 7.000000000000004441 ) ) ;
+#960 = ORIENTED_EDGE ( 'NONE', *, *, #1680, .F. ) ;
+#961 = CARTESIAN_POINT ( 'NONE', ( -44.90380467601652015, 6.731651258784625114, 11.75704472016699143 ) ) ;
+#962 = CARTESIAN_POINT ( 'NONE', ( -32.01713932801011708, 32.35111862366360214, -12.05200785716763079 ) ) ;
+#963 = EDGE_CURVE ( 'NONE', #1225, #1630, #1565, .T. ) ;
+#964 = CIRCLE ( 'NONE', #875, 5.000000000000001776 ) ;
+#965 = VECTOR ( 'NONE', #2042, 1000.000000000000000 ) ;
+#966 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#967 = CARTESIAN_POINT ( 'NONE', ( -30.19042886650187896, 32.96641650756577491, -13.76088780830553127 ) ) ;
+#968 = CARTESIAN_POINT ( 'NONE', ( -35.70873277584415462, 33.36784647862091902, -6.579185176894301712 ) ) ;
+#969 = LINE ( 'NONE', #718, #2367 ) ;
+#970 = CARTESIAN_POINT ( 'NONE', ( -40.72182540694797126, 14.10497510537457089, -7.000000000000002665 ) ) ;
+#971 = CARTESIAN_POINT ( 'NONE', ( 36.80118201742516959, 30.26574514009140060, 18.06883033848986742 ) ) ;
+#972 = ORIENTED_EDGE ( 'NONE', *, *, #1730, .T. ) ;
+#973 = CARTESIAN_POINT ( 'NONE', ( -44.36934426592458891, 8.283993259259442965, 9.816037424464186145 ) ) ;
+#974 = ORIENTED_EDGE ( 'NONE', *, *, #342, .F. ) ;
+#975 = CARTESIAN_POINT ( 'NONE', ( -33.58269050972878489, 39.61386419851559992, 4.499999999999999112 ) ) ;
+#976 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371482125, 44.36900610828815417, 12.92542883016145439 ) ) ;
+#977 = CIRCLE ( 'NONE', #2413, 1.000000000000000888 ) ;
+#978 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#979 = EDGE_CURVE ( 'NONE', #2692, #1225, #2599, .T. ) ;
+#980 = CARTESIAN_POINT ( 'NONE', ( -35.89971384576190871, 32.88406237842237090, -7.200207811996323315 ) ) ;
+#981 = CARTESIAN_POINT ( 'NONE', ( -34.80337923684058410, 33.55602045099649899, -17.29676711764147612 ) ) ;
+#982 = CARTESIAN_POINT ( 'NONE', ( 43.47300727646558016, 10.88740696221084470, 13.92728719611736743 ) ) ;
+#983 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, -7.000000000000004441 ) ) ;
+#984 = CARTESIAN_POINT ( 'NONE', ( -43.13506908963908160, 11.86894952563805461, -14.35735567708467109 ) ) ;
+#985 = ORIENTED_EDGE ( 'NONE', *, *, #1012, .T. ) ;
+#986 = CARTESIAN_POINT ( 'NONE', ( -41.70300804589836474, 16.02837548772422949, 6.859094606482983636 ) ) ;
+#987 = AXIS2_PLACEMENT_3D ( 'NONE', #1742, #1101, #1940 ) ;
+#988 = VERTEX_POINT ( 'NONE', #1570 ) ;
+#989 = FACE_OUTER_BOUND ( 'NONE', #2525, .T. ) ;
+#990 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 0.000000000000000000, 20.50000000000000000 ) ) ;
+#991 = ORIENTED_EDGE ( 'NONE', *, *, #2754, .F. ) ;
+#992 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1743, #2135, #1119, #846 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 3.141592653589793116, 6.283185307179586232 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.3333333333333333703, 0.3333333333333333703, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#993 = CARTESIAN_POINT ( 'NONE', ( -44.67819521171908548, 7.386934682066240043, -10.65018635782133316 ) ) ;
+#994 = CARTESIAN_POINT ( 'NONE', ( -35.49382296729514508, 33.72405603934700480, -6.646229837023073017 ) ) ;
+#995 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 7.000000000000004441 ) ) ;
+#996 = ADVANCED_FACE ( 'NONE', ( #1763 ), #50, .T. ) ;
+#997 = CARTESIAN_POINT ( 'NONE', ( 42.96469508733385823, 6.406112403811383693, 11.87134553244899671 ) ) ;
+#998 = CARTESIAN_POINT ( 'NONE', ( -33.26615356463607043, 35.00497175787329951, -7.628078471032430308 ) ) ;
+#999 = CARTESIAN_POINT ( 'NONE', ( -40.90041223295836659, 13.49195890305930057, -16.84657827221492354 ) ) ;
+#1000 = VERTEX_POINT ( 'NONE', #1142 ) ;
+#1001 = CARTESIAN_POINT ( 'NONE', ( -42.74090254271514766, 13.01380754396722672, 7.602985723577359245 ) ) ;
+#1002 = VERTEX_POINT ( 'NONE', #1781 ) ;
+#1003 = ORIENTED_EDGE ( 'NONE', *, *, #1251, .T. ) ;
+#1004 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1005 = CARTESIAN_POINT ( 'NONE', ( -32.27750898416555003, 43.40476818713303686, 14.59440178004760291 ) ) ;
+#1006 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#1007 = AXIS2_PLACEMENT_3D ( 'NONE', #1945, #1500, #2338 ) ;
+#1008 = ORIENTED_EDGE ( 'NONE', *, *, #2057, .F. ) ;
+#1009 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233770518, -20.50000000000000355 ) ) ;
+#1010 = CARTESIAN_POINT ( 'NONE', ( -32.19618542919716475, 32.86981725267617804, -13.95978199525807639 ) ) ;
+#1011 = CARTESIAN_POINT ( 'NONE', ( 44.77913493337637618, 7.093754932011645309, 11.02328810641580858 ) ) ;
+#1012 = EDGE_CURVE ( 'NONE', #1729, #1962, #1834, .T. ) ;
+#1013 = CARTESIAN_POINT ( 'NONE', ( 32.15342044700702218, 43.76518374714715520, 10.81466330593660530 ) ) ;
+#1014 = CARTESIAN_POINT ( 'NONE', ( -42.44850599150973380, 8.177980386844691196, -14.91115635319375698 ) ) ;
+#1015 = EDGE_LOOP ( 'NONE', ( #972, #1212 ) ) ;
+#1016 = EDGE_CURVE ( 'NONE', #2325, #1677, #1179, .T. ) ;
+#1017 = CARTESIAN_POINT ( 'NONE', ( -42.81541933757661411, 6.918515559511693169, 11.07567117061601891 ) ) ;
+#1018 = ORIENTED_EDGE ( 'NONE', *, *, #2386, .T. ) ;
+#1019 = EDGE_LOOP ( 'NONE', ( #354, #1333, #1405, #1723 ) ) ;
+#1020 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, 4.500000000000000888 ) ) ;
+#1021 = CARTESIAN_POINT ( 'NONE', ( -30.19016499665614717, 32.96574621937423188, -11.24027237217475239 ) ) ;
+#1022 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -20.50000000000000000 ) ) ;
+#1023 = ORIENTED_EDGE ( 'NONE', *, *, #413, .T. ) ;
+#1024 = CARTESIAN_POINT ( 'NONE', ( -42.80493708065814928, 6.954496899633142881, -11.04381004085507811 ) ) ;
+#1025 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1026 = CARTESIAN_POINT ( 'NONE', ( -33.45555914273361253, 35.07030372904624471, -16.23168523132997976 ) ) ;
+#1027 = VECTOR ( 'NONE', #1490, 1000.000000000000000 ) ;
+#1028 = STYLED_ITEM ( 'NONE', ( #112 ), #1362 ) ;
+#1029 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371285660, 6.610463800732986783, 12.50000000000000000 ) ) ;
+#1030 = LINE ( 'NONE', #2577, #2593 ) ;
+#1031 = CARTESIAN_POINT ( 'NONE', ( -42.87529148811312751, 12.62347441469967535, -17.28688690812499829 ) ) ;
+#1032 = ORIENTED_EDGE ( 'NONE', *, *, #1105, .T. ) ;
+#1033 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1034 = ORIENTED_EDGE ( 'NONE', *, *, #1514, .T. ) ;
+#1035 = CARTESIAN_POINT ( 'NONE', ( 4.000000000000000000, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#1036 = ADVANCED_FACE ( 'NONE', ( #1584 ), #512, .T. ) ;
+#1037 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#1038 = AXIS2_PLACEMENT_3D ( 'NONE', #2487, #978, #374 ) ;
+#1039 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1040 = AXIS2_PLACEMENT_3D ( 'NONE', #806, #2321, #1240 ) ;
+#1041 = VERTEX_POINT ( 'NONE', #1910 ) ;
+#1042 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 9.749999999999998224 ) ) ;
+#1043 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000001421, 5.000000000000000000, 2.428932188134526271 ) ) ;
+#1044 = CARTESIAN_POINT ( 'NONE', ( 44.72738788084891581, 7.244054414484709881, 10.81670229254628701 ) ) ;
+#1045 = CARTESIAN_POINT ( 'NONE', ( 43.34254294013596365, 11.26634105166327693, 8.204626120997366101 ) ) ;
+#1046 = CARTESIAN_POINT ( 'NONE', ( -39.73300686199627307, 17.49918845664421951, -17.54670450738533916 ) ) ;
+#1047 = ORIENTED_EDGE ( 'NONE', *, *, #233, .T. ) ;
+#1048 = DIRECTION ( 'NONE', ( -0.9455286637128577087, 0.3255388549733774228, 0.000000000000000000 ) ) ;
+#1049 = ORIENTED_EDGE ( 'NONE', *, *, #2656, .F. ) ;
+#1050 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1051 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 25.16419609954003533, 7.000000000000004441 ) ) ;
+#1052 = EDGE_CURVE ( 'NONE', #1870, #1002, #136, .T. ) ;
+#1053 = ORIENTED_EDGE ( 'NONE', *, *, #1482, .F. ) ;
+#1054 = CARTESIAN_POINT ( 'NONE', ( -41.24826085141516074, 12.29793555972540808, -8.449557231586465988 ) ) ;
+#1055 = CARTESIAN_POINT ( 'NONE', ( -40.72182540694797126, 14.10497510537457089, -18.00000000000000000 ) ) ;
+#1056 = APPLICATION_CONTEXT ( 'automotive_design' ) ;
+#1057 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1058 = CARTESIAN_POINT ( 'NONE', ( 33.32773819346318334, 40.35437403084399932, 8.879475727009843666 ) ) ;
+#1059 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945760252513, -12.61334297384667380 ) ) ;
+#1060 = VERTEX_POINT ( 'NONE', #1338 ) ;
+#1061 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1062 = ORIENTED_EDGE ( 'NONE', *, *, #1218, .F. ) ;
+#1063 = CARTESIAN_POINT ( 'NONE', ( 13.87540813888669788, 5.577367205542728357, -0.5000000000000004441 ) ) ;
+#1064 = CYLINDRICAL_SURFACE ( 'NONE', #1102, 2.750000000000002665 ) ;
+#1065 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #810, #298, #509, #2101 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 0.000000000000000000, 1.570796326794896780 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1066 = ORIENTED_EDGE ( 'NONE', *, *, #1406, .T. ) ;
+#1067 = ADVANCED_FACE ( 'NONE', ( #1308, #1925 ), #2168, .F. ) ;
+#1068 = CARTESIAN_POINT ( 'NONE', ( -42.44850599151052251, 8.177980386844092564, -10.08884364680770673 ) ) ;
+#1069 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 4.500000000000000888 ) ) ;
+#1070 = EDGE_CURVE ( 'NONE', #1002, #1870, #2203, .T. ) ;
+#1071 = CARTESIAN_POINT ( 'NONE', ( 42.92726601009341891, 6.534591258040115314, 13.39603710458456831 ) ) ;
+#1072 = CARTESIAN_POINT ( 'NONE', ( 37.65733024777571814, 27.77905981872056529, 6.883443643188544847 ) ) ;
+#1073 = CIRCLE ( 'NONE', #1969, 5.499999999999994671 ) ;
+#1074 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 25.16419609954003533, 17.99999999999999645 ) ) ;
+#1075 = ORIENTED_EDGE ( 'NONE', *, *, #376, .T. ) ;
+#1076 = CYLINDRICAL_SURFACE ( 'NONE', #2349, 2.750000000000002665 ) ;
+#1077 = ORIENTED_EDGE ( 'NONE', *, *, #651, .F. ) ;
+#1078 = PLANE ( 'NONE', #1381 ) ;
+#1079 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1080 = CARTESIAN_POINT ( 'NONE', ( -32.40418784956184339, 33.25646888864360307, -14.57236020808944943 ) ) ;
+#1081 = ORIENTED_EDGE ( 'NONE', *, *, #1957, .F. ) ;
+#1082 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1083 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759544634, -12.50000000000000000 ) ) ;
+#1084 = ORIENTED_EDGE ( 'NONE', *, *, #2202, .F. ) ;
+#1085 = FACE_OUTER_BOUND ( 'NONE', #1495, .T. ) ;
+#1086 = CARTESIAN_POINT ( 'NONE', ( 15.50000000000002132, 5.000000000000000000, -20.50000000000000000 ) ) ;
+#1087 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, 20.50000000000000000 ) ) ;
+#1088 = EDGE_CURVE ( 'NONE', #851, #2404, #187, .T. ) ;
+#1089 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 6.284924945759599701, 12.50000000000000000 ) ) ;
+#1090 = CARTESIAN_POINT ( 'NONE', ( 1.750000000000000888, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#1091 = ORIENTED_EDGE ( 'NONE', *, *, #2553, .F. ) ;
+#1092 = APPLICATION_PROTOCOL_DEFINITION ( 'draft international standard', 'automotive_design', 1998, #478 ) ;
+#1093 = CARTESIAN_POINT ( 'NONE', ( -34.10555997850872245, 33.94718821219309035, -8.170759618827506898 ) ) ;
+#1094 = CARTESIAN_POINT ( 'NONE', ( -30.93595433358951752, 34.14523117820939291, -15.30481300744316187 ) ) ;
+#1095 = EDGE_LOOP ( 'NONE', ( #2516, #1736, #1616, #1032 ) ) ;
+#1096 = CARTESIAN_POINT ( 'NONE', ( -43.13506908964116349, 11.86894952563452499, -10.64264432290875817 ) ) ;
+#1097 = CARTESIAN_POINT ( 'NONE', ( -39.29637667370440823, 23.01844414046954412, 19.00000000000000000 ) ) ;
+#1098 = VERTEX_POINT ( 'NONE', #629 ) ;
+#1099 = CYLINDRICAL_SURFACE ( 'NONE', #2148, 30.00000000000000711 ) ;
+#1100 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1101 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1102 = AXIS2_PLACEMENT_3D ( 'NONE', #223, #2557, #649 ) ;
+#1103 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1104 = AXIS2_PLACEMENT_3D ( 'NONE', #2645, #2693, #1187 ) ;
+#1105 = EDGE_CURVE ( 'NONE', #1315, #2690, #1292, .T. ) ;
+#1106 = EDGE_LOOP ( 'NONE', ( #1285, #524, #2733, #210 ) ) ;
+#1107 = EDGE_CURVE ( 'NONE', #758, #603, #992, .T. ) ;
+#1108 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -13.98362640057776396 ) ) ;
+#1109 = CARTESIAN_POINT ( 'NONE', ( -33.12690805908333402, 40.17524856008240874, 9.145056144941321463 ) ) ;
+#1110 = CARTESIAN_POINT ( 'NONE', ( -33.79662054001477145, 34.97389226050179900, -16.56937704322189120 ) ) ;
+#1111 = CARTESIAN_POINT ( 'NONE', ( 33.59905643722281354, 39.56632930884568822, 16.41383903443620795 ) ) ;
+#1112 = ORIENTED_EDGE ( 'NONE', *, *, #60, .T. ) ;
+#1113 = CARTESIAN_POINT ( 'NONE', ( -42.46419766629922066, 13.81749775803332092, -7.363134769199811913 ) ) ;
+#1114 = CARTESIAN_POINT ( 'NONE', ( -42.81541933757666385, 6.918515559511272173, 13.92432882938381944 ) ) ;
+#1115 = ORIENTED_EDGE ( 'NONE', *, *, #94, .T. ) ;
+#1116 = CARTESIAN_POINT ( 'NONE', ( -31.62984376346780380, 36.62285101022547451, -13.44281979267887017 ) ) ;
+#1117 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1118 = CARTESIAN_POINT ( 'NONE', ( -44.00000000000000000, 6.284924945759554404, 12.50000000000000000 ) ) ;
+#1119 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, 25.49999999999999645 ) ) ;
+#1120 = PLANE ( 'NONE', #1491 ) ;
+#1121 = AXIS2_PLACEMENT_3D ( 'NONE', #2337, #1282, #2309 ) ;
+#1122 = VECTOR ( 'NONE', #1761, 1000.000000000000000 ) ;
+#1123 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#1124 = FACE_OUTER_BOUND ( 'NONE', #2339, .T. ) ;
+#1125 = CARTESIAN_POINT ( 'NONE', ( 38.93961336430090370, 20.22258681569049799, 17.85684972154116323 ) ) ;
+#1126 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.8591838389225119332, -0.5116670117707171617 ) ) ;
+#1127 = CARTESIAN_POINT ( 'NONE', ( -35.24008933273383093, 34.79994392319499497, 17.60429368842434172 ) ) ;
+#1128 = CARTESIAN_POINT ( 'NONE', ( -30.13401351642946935, 32.82655668348647993, -11.60121286152213926 ) ) ;
+#1129 = CARTESIAN_POINT ( 'NONE', ( -32.12053186653007941, 35.40005520948766105, -11.04217675959103317 ) ) ;
+#1130 = VECTOR ( 'NONE', #1607, 1000.000000000000000 ) ;
+#1131 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, -7.000000000000004441 ) ) ;
+#1132 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1133 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725331898682, 12.85997824090589248 ) ) ;
+#1134 = ORIENTED_EDGE ( 'NONE', *, *, #2369, .T. ) ;
+#1135 = CARTESIAN_POINT ( 'NONE', ( -35.55598056228235038, 33.62873098028818220, -18.37343026423007331 ) ) ;
+#1136 = FACE_OUTER_BOUND ( 'NONE', #2053, .T. ) ;
+#1137 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1138 = CARTESIAN_POINT ( 'NONE', ( 41.87309194091710651, 10.15314363899532069, 15.85494385505801773 ) ) ;
+#1139 = CARTESIAN_POINT ( 'NONE', ( -31.62984376346780380, 36.62285101022548162, -11.55718020732113160 ) ) ;
+#1140 = CARTESIAN_POINT ( 'NONE', ( -43.67796458835100282, 10.29210778281889027, 12.19103034307719824 ) ) ;
+#1141 = EDGE_CURVE ( 'NONE', #1000, #1174, #1173, .T. ) ;
+#1142 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 25.16419609954003533, 17.99999999999999645 ) ) ;
+#1143 = CARTESIAN_POINT ( 'NONE', ( -34.05650722053313473, 33.95958742075126224, -8.209730131506509210 ) ) ;
+#1144 = CYLINDRICAL_SURFACE ( 'NONE', #443, 3.000000000000002665 ) ;
+#1145 = FACE_OUTER_BOUND ( 'NONE', #2113, .T. ) ;
+#1146 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -13.75537618510426086 ) ) ;
+#1147 = CIRCLE ( 'NONE', #1256, 4.000000000000001776 ) ;
+#1148 = ORIENTED_EDGE ( 'NONE', *, *, #1921, .F. ) ;
+#1149 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #1878, #625, #2341 ),
+ ( #871, #597, #1461 ),
+ ( #389, #1935, #1245 ),
+ ( #2742, #1720, #14 ),
+ ( #1907, #2105, #1895 ),
+ ( #418, #827, #433 ),
+ ( #611, #1273, #1474 ),
+ ( #1287, #2132, #3 ),
+ ( #856, #930, #1793 ),
+ ( #725, #2398, #1780 ),
+ ( #256, #1993, #1114 ),
+ ( #268, #670, #1964 ),
+ ( #506, #2428, #2175 ),
+ ( #44, #1140, #2005 ),
+ ( #902, #1582, #1332 ),
+ ( #1980, #59, #2634 ),
+ ( #2439, #516, #283 ),
+ ( #2205, #1361, #2215 ),
+ ( #943, #463, #1752 ),
+ ( #714, #1373, #916 ),
+ ( #2646, #1347, #1317 ),
+ ( #1153, #1536, #1555 ),
+ ( #1764, #2619, #240 ),
+ ( #1949, #1545, #73 ),
+ ( #1097, #2607, #29 ),
+ ( #685, #1738, #294 ),
+ ( #2410, #885, #492 ),
+ ( #2591, #1127, #475 ),
+ ( #1571, #2385, #85 ),
+ ( #2189, #698, #1618 ),
+ ( #1165, #321, #527 ),
+ ( #2044, #1399, #2056 ),
+ ( #1606, #343, #1645 ),
+ ( #1005, #760, #2265 ),
+ ( #1592, #2668, #2071 ),
+ ( #109, #121, #2708 ),
+ ( #976, #2462, #2449 ),
+ ( #2472, #135, #790 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 0.000000000000000000, 0.04908738521234051744, 0.09817477042468103487, 0.1472621556370215523, 0.1963495408493620697, 0.2945243112740431046, 0.3926990816987241395, 0.4908738521234051744, 0.5890486225480862092, 0.7853981633974482790, 0.9817477042468103487, 1.178097245096172418, 1.570796326794896558, 1.963495408493620697, 2.356194490192344837, 2.552544031041707129, 2.748893571891068976, 2.945243112740430824, 3.141592653589793116 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.1650323245415012685, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650312009201844454, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1656105720154912864, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1678868551765326789, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1695842193436412626, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1739978385179177800, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1767131491670237697, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1830235777788156992, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1866179000439102120, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1984960600878372672, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2078644858773363580, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2284507283937017852, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2396680134330874401, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2632145179655339673, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2755469356012855697, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3008525186329233381, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3138283910420501810, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3532598395141759440, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3801944580800665219, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4339434541711514881, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4607773684084325860, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5135864445221741681, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5395685366565537988, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6154803776381509994, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6633476856979385605, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7508658766751572511, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7905347754417177963, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8598216432928615927, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8894434017614355481, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9253744439782182196, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9359386994017488970, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9541292101921223789, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9617554693256525900, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9739896480940225532, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9785975666657328320, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9847468226704357841, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9862886620238880964, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9862881586313551052, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#1150 = CARTESIAN_POINT ( 'NONE', ( -34.82445533374842483, 34.40873363110451777, -18.11687117007166137 ) ) ;
+#1151 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1152 = CARTESIAN_POINT ( 'NONE', ( -32.02749458758146517, 32.38112555743335008, -13.05953186127941557 ) ) ;
+#1153 = CARTESIAN_POINT ( 'NONE', ( -41.70300804589821553, 16.02837548772466292, 18.14090539351757059 ) ) ;
+#1154 = ADVANCED_FACE ( 'NONE', ( #261 ), #2344, .F. ) ;
+#1155 = CARTESIAN_POINT ( 'NONE', ( -36.36104731350427954, 31.54411781998817688, -18.67996665249634347 ) ) ;
+#1156 = CARTESIAN_POINT ( 'NONE', ( -34.41296052421962059, 34.70515261446727351, -7.940740440419745916 ) ) ;
+#1157 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1158 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371300226, 44.36900610829343350, 12.92542883016144728 ) ) ;
+#1159 = EDGE_CURVE ( 'NONE', #2587, #1516, #1458, .T. ) ;
+#1160 = ORIENTED_EDGE ( 'NONE', *, *, #1427, .T. ) ;
+#1161 = AXIS2_PLACEMENT_3D ( 'NONE', #1169, #299, #2047 ) ;
+#1162 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, 12.50000000000000000 ) ) ;
+#1163 = AXIS2_PLACEMENT_3D ( 'NONE', #306, #2241, #1190 ) ;
+#1164 =( GEOMETRIC_REPRESENTATION_CONTEXT ( 3 ) GLOBAL_UNCERTAINTY_ASSIGNED_CONTEXT ( ( #2237 ) ) GLOBAL_UNIT_ASSIGNED_CONTEXT ( ( #1835, #1207, #1880 ) ) REPRESENTATION_CONTEXT ( 'NONE', 'WORKASPACE' ) );
+#1165 = CARTESIAN_POINT ( 'NONE', ( -33.27732909717364151, 40.50078741505868862, 16.46493364688652150 ) ) ;
+#1166 = CARTESIAN_POINT ( 'NONE', ( -32.00008894653174707, 37.38113046985179011, -12.63708486437931988 ) ) ;
+#1167 = CARTESIAN_POINT ( 'NONE', ( -31.71517016143365453, 36.15757294142542833, -12.78387847678332179 ) ) ;
+#1168 = CARTESIAN_POINT ( 'NONE', ( -28.36585991138575125, 54.76616564920128383, -135.3085362679971126 ) ) ;
+#1169 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#1170 = VECTOR ( 'NONE', #541, 1000.000000000000000 ) ;
+#1171 = EDGE_CURVE ( 'NONE', #1956, #1459, #398, .T. ) ;
+#1172 = CARTESIAN_POINT ( 'NONE', ( 15.50000000000002132, 5.000000000000000000, -0.5000000000000004441 ) ) ;
+#1173 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1074, #1697, #423, #1277 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 4.712388980384689674, 6.283185307179586232 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1174 = VERTEX_POINT ( 'NONE', #1089 ) ;
+#1175 = UNCERTAINTY_MEASURE_WITH_UNIT (LENGTH_MEASURE( 1.000000000000000082E-05 ), #82, 'distance_accuracy_value', 'NONE');
+#1176 = CARTESIAN_POINT ( 'NONE', ( -39.93733163486207616, 16.79782431294281864, 7.556418717552698006 ) ) ;
+#1177 = LINE ( 'NONE', #1020, #2506 ) ;
+#1178 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#1179 = LINE ( 'NONE', #750, #1130 ) ;
+#1180 = CARTESIAN_POINT ( 'NONE', ( -35.79885818798025099, 33.17699796467663731, -6.552627828686637734 ) ) ;
+#1181 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688393855, 32.64726791736441669, -17.54951290997401259 ) ) ;
+#1182 = CARTESIAN_POINT ( 'NONE', ( -32.10226390623606818, 32.59994293174793256, -11.42770916596734487 ) ) ;
+#1183 = CARTESIAN_POINT ( 'NONE', ( 41.26442097287530686, 17.30225306865727930, 16.79236178307509775 ) ) ;
+#1184 = ORIENTED_EDGE ( 'NONE', *, *, #766, .T. ) ;
+#1185 = CARTESIAN_POINT ( 'NONE', ( -44.85957031200517520, 6.860130113013125808, 11.44104705821813894 ) ) ;
+#1186 = ORIENTED_EDGE ( 'NONE', *, *, #2403, .T. ) ;
+#1187 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1188 = AXIS2_PLACEMENT_3D ( 'NONE', #1283, #2140, #1079 ) ;
+#1189 = EDGE_CURVE ( 'NONE', #254, #1677, #1757, .T. ) ;
+#1190 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1191 = CARTESIAN_POINT ( 'NONE', ( -42.99649525893036639, 6.296955301573155417, -12.27331410414104873 ) ) ;
+#1192 = PLANE ( 'NONE', #2055 ) ;
+#1193 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1194 = LINE ( 'NONE', #753, #631 ) ;
+#1195 = CARTESIAN_POINT ( 'NONE', ( 42.74090254271488476, 13.01380754396802608, 17.39701427642179610 ) ) ;
+#1196 = ORIENTED_EDGE ( 'NONE', *, *, #1070, .T. ) ;
+#1197 = CARTESIAN_POINT ( 'NONE', ( -41.13454712838774441, 12.68826868899362204, 8.356372535335728102 ) ) ;
+#1198 = ORIENTED_EDGE ( 'NONE', *, *, #2014, .F. ) ;
+#1199 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#1200 = CARTESIAN_POINT ( 'NONE', ( 13.87540813888669611, 5.577367205542723916, -20.50000000000000000 ) ) ;
+#1201 = EDGE_CURVE ( 'NONE', #2058, #839, #289, .T. ) ;
+#1202 = CIRCLE ( 'NONE', #2147, 4.000000000000001776 ) ;
+#1203 = ORIENTED_EDGE ( 'NONE', *, *, #213, .T. ) ;
+#1204 = CARTESIAN_POINT ( 'NONE', ( -42.94403121265326462, 6.477043111662819541, -11.70919611629001444 ) ) ;
+#1205 = CARTESIAN_POINT ( 'NONE', ( -35.27997614909728696, 34.00828475802443762, -7.446164546780327242 ) ) ;
+#1206 = CARTESIAN_POINT ( 'NONE', ( -42.99999999999999289, 6.284924945759550852, 15.72182540694797659 ) ) ;
+#1207 =( NAMED_UNIT ( * ) PLANE_ANGLE_UNIT ( ) SI_UNIT ( $, .RADIAN. ) );
+#1208 = CARTESIAN_POINT ( 'NONE', ( -30.33478770275461756, 33.33312011105832795, -10.74992447058079925 ) ) ;
+#1209 = CARTESIAN_POINT ( 'NONE', ( -42.66701332672615621, 7.427933254001917618, -14.38807306752410220 ) ) ;
+#1210 = ORIENTED_EDGE ( 'NONE', *, *, #1981, .F. ) ;
+#1211 = CARTESIAN_POINT ( 'NONE', ( -43.54841234348915435, 10.66839269963314685, 13.83319670399202650 ) ) ;
+#1212 = ORIENTED_EDGE ( 'NONE', *, *, #2717, .T. ) ;
+#1213 = VERTEX_POINT ( 'NONE', #1772 ) ;
+#1214 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1215 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1216 = CARTESIAN_POINT ( 'NONE', ( -34.67603907761178306, 33.65127714537908332, -7.779315588964926143 ) ) ;
+#1217 = ORIENTED_EDGE ( 'NONE', *, *, #138, .F. ) ;
+#1218 = EDGE_CURVE ( 'NONE', #2543, #2761, #1350, .T. ) ;
+#1219 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1220 = CARTESIAN_POINT ( 'NONE', ( -30.49879357623249021, 33.60651729438624358, -14.58866304604573827 ) ) ;
+#1221 = EDGE_CURVE ( 'NONE', #484, #1656, #2020, .T. ) ;
+#1222 = CARTESIAN_POINT ( 'NONE', ( 40.17685806913076618, 20.46108306200142124, 7.477634347492172040 ) ) ;
+#1223 = CARTESIAN_POINT ( 'NONE', ( -42.70553305319337056, 13.11653834139425712, -15.19717562793444898 ) ) ;
+#1224 = CARTESIAN_POINT ( 'NONE', ( -40.25632870800318130, 15.70283663275019670, 7.726926205485937338 ) ) ;
+#1225 = VERTEX_POINT ( 'NONE', #1146 ) ;
+#1226 = EDGE_LOOP ( 'NONE', ( #1422, #2710, #235, #1926, #1196 ) ) ;
+#1227 = DIRECTION ( 'NONE', ( -1.000000000000000000, -0.000000000000000000, -0.000000000000000000 ) ) ;
+#1228 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1229 = LINE ( 'NONE', #1797, #1605 ) ;
+#1230 = EDGE_LOOP ( 'NONE', ( #1084, #1686, #883, #511, #2705, #191, #2392, #2722, #2266 ) ) ;
+#1231 = CARTESIAN_POINT ( 'NONE', ( -42.88477153647212958, 6.680457565318799418, -11.37435257436250780 ) ) ;
+#1232 = CARTESIAN_POINT ( 'NONE', ( -32.11570477478471730, 34.86676235285923298, -16.56647899812988811 ) ) ;
+#1233 = ADVANCED_FACE ( 'NONE', ( #948 ), #1279, .T. ) ;
+#1234 = CARTESIAN_POINT ( 'NONE', ( 44.07774513821172491, 9.130943874689785744, 9.248325972625204727 ) ) ;
+#1235 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371300226, 44.36900610829343350, 12.07457116983854739 ) ) ;
+#1236 = CARTESIAN_POINT ( 'NONE', ( -42.34167783728248224, 14.17335700014439759, -15.72498084163509091 ) ) ;
+#1237 = EDGE_LOOP ( 'NONE', ( #2295, #1826, #1951, #2332, #1134, #12 ) ) ;
+#1238 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#1239 = ORIENTED_EDGE ( 'NONE', *, *, #1644, .F. ) ;
+#1240 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1241 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#1242 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1243 = ORIENTED_EDGE ( 'NONE', *, *, #1052, .T. ) ;
+#1244 = CARTESIAN_POINT ( 'NONE', ( -43.59320368275608359, 10.53829610896616664, -13.70289350183125343 ) ) ;
+#1245 = CARTESIAN_POINT ( 'NONE', ( -42.99779078280186440, 6.292508293127065500, 12.67998707075209630 ) ) ;
+#1246 = VECTOR ( 'NONE', #436, 1000.000000000000000 ) ;
+#1247 = CARTESIAN_POINT ( 'NONE', ( 42.98233690606029000, 6.345555189378156768, 12.05046592392583804 ) ) ;
+#1248 = CARTESIAN_POINT ( 'NONE', ( 42.27964029246205513, 14.35354525096334477, 9.216698446040350490 ) ) ;
+#1249 = CARTESIAN_POINT ( 'NONE', ( -42.80493708065813507, 6.954496899632762741, -13.95618995914495208 ) ) ;
+#1250 = VERTEX_POINT ( 'NONE', #1896 ) ;
+#1251 = EDGE_CURVE ( 'NONE', #2664, #2746, #1391, .T. ) ;
+#1252 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1253 = ORIENTED_EDGE ( 'NONE', *, *, #2532, .T. ) ;
+#1254 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, -0.4999999999999995559 ) ) ;
+#1255 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371285660, 6.610463800732987671, 12.50000000000000000 ) ) ;
+#1256 = AXIS2_PLACEMENT_3D ( 'NONE', #2734, #2335, #1712 ) ;
+#1257 = CARTESIAN_POINT ( 'NONE', ( -1.750000000000000888, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#1258 = ORIENTED_EDGE ( 'NONE', *, *, #2057, .T. ) ;
+#1259 = CARTESIAN_POINT ( 'NONE', ( -35.73620674676906361, 31.21858356992176908, -7.270798027060732416 ) ) ;
+#1260 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1261 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#1262 = CARTESIAN_POINT ( 'NONE', ( 34.73009560828655395, 34.67215538704933664, 7.694174863916341423 ) ) ;
+#1263 = CARTESIAN_POINT ( 'NONE', ( -42.86042546973750689, 6.764027746302711641, -13.73640018207928115 ) ) ;
+#1264 = VERTEX_POINT ( 'NONE', #2329 ) ;
+#1265 = CARTESIAN_POINT ( 'NONE', ( 34.27817459305202874, 36.22341709370546425, 18.00000000000000000 ) ) ;
+#1266 = ORIENTED_EDGE ( 'NONE', *, *, #862, .T. ) ;
+#1267 = EDGE_CURVE ( 'NONE', #2325, #1700, #1884, .T. ) ;
+#1268 = CARTESIAN_POINT ( 'NONE', ( -30.14789704064898501, 32.86079898432327440, -11.48062837958230809 ) ) ;
+#1269 = CYLINDRICAL_SURFACE ( 'NONE', #1007, 1.750000000000000888 ) ;
+#1270 = EDGE_CURVE ( 'NONE', #2620, #2068, #538, .T. ) ;
+#1271 = VERTEX_POINT ( 'NONE', #407 ) ;
+#1272 = CARTESIAN_POINT ( 'NONE', ( -40.07226420255292965, 20.76487628785908512, -6.184082296412528379 ) ) ;
+#1273 = CARTESIAN_POINT ( 'NONE', ( -43.20911154149919042, 11.65389298324523715, 11.25261746733953316 ) ) ;
+#1274 = CIRCLE ( 'NONE', #1588, 2.750000000000002665 ) ;
+#1275 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1276 = CARTESIAN_POINT ( 'NONE', ( 38.93961336429532594, 20.22258681569371674, 7.143150278456521285 ) ) ;
+#1277 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 6.284924945759599701, 12.50000000000000000 ) ) ;
+#1278 = ORIENTED_EDGE ( 'NONE', *, *, #1957, .T. ) ;
+#1279 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #2626, #1367, #2195 ),
+ ( #1158, #1560, #1133 ),
+ ( #1986, #2221, #2011 ),
+ ( #936, #2641, #704 ),
+ ( #274, #65, #93 ),
+ ( #2417, #2000, #1353 ),
+ ( #79, #1785, #2586 ),
+ ( #1613, #281, #337 ),
+ ( #266, #1111, #696 ),
+ ( #1961, #460, #2468 ),
+ ( #2258, #253, #292 ),
+ ( #1553, #971, #2408 ),
+ ( #1404, #2172, #1822 ),
+ ( #2675, #39, #57 ),
+ ( #754, #2201, #1125 ),
+ ( #1330, #1183, #1543 ),
+ ( #900, #1991, #1532 ),
+ ( #1345, #2424, #546 ),
+ ( #1195, #1749, #2604 ),
+ ( #70, #683, #2049 ),
+ ( #1762, #503, #1138 ),
+ ( #128, #2396, #928 ),
+ ( #489, #1778, #1734 ),
+ ( #667, #1977, #2632 ),
+ ( #711, #1314, #2382 ),
+ ( #2187, #473, #2037 ),
+ ( #1569, #913, #1359 ),
+ ( #2618, #2214, #115 ),
+ ( #2576, #1477, #1305 ),
+ ( #1319, #1739, #642 ),
+ ( #435, #656, #1071 ),
+ ( #464, #203, #2563 ),
+ ( #2387, #2595, #612 ),
+ ( #1937, #673, #242 ),
+ ( #1908, #843, #1539 ),
+ ( #1708, #214, #1521 ),
+ ( #2756, #2342, #2178 ),
+ ( #258, #2121, #2133 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 3.141592653589793116, 3.337942194439155408, 3.534291735288517255, 3.730641276137879103, 3.926990816987241395, 4.319689898685965090, 4.712388980384689674, 5.105088062083414258, 5.301437602932775661, 5.497787143782137953, 5.694136684631500245, 5.792311455056180947, 5.890486225480861648, 5.988660995905542350, 6.086835766330223940, 6.135923151542565179, 6.185010536754905530, 6.234097921967245881, 6.283185307179586232 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.9862881586313551052, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9862886620239492697, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9847468226703547378, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9785975666658145444, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9739896480940227752, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9617554693256528120, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9541292101921223789, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9359386994017488970, 1.000000000000000000),
+ ( 1.000000000000000000, 0.9253744439780019482, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8894434017618667587, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8598216432909758788, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7905347754436035101, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7508658766753982805, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6633476856976968650, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6154803776383602765, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5395685366564493268, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5135864445223659036, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4607773684082399623, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4339434541725344374, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3801944580786827954, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3532598395135532754, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3138283910423629308, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3008525186323626199, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2755469356018441784, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2632145179657154888, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2396680134329088885, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2284507283938273792, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2078644858772128179, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1984960600879293602, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1866179000438641378, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1830235777788575269, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1767131491669811094, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1739978385178775067, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1695842193436807033, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1678868551765243244, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1656105720155000849, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650312009201779229, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650323245415012685, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#1280 = CARTESIAN_POINT ( 'NONE', ( -43.00000000005575629, 12.26125813590357261, 12.69414988364419727 ) ) ;
+#1281 = VERTEX_POINT ( 'NONE', #451 ) ;
+#1282 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1283 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#1284 = CARTESIAN_POINT ( 'NONE', ( -33.95686232909699953, 34.85544970394357733, -17.73809573338819590 ) ) ;
+#1285 = ORIENTED_EDGE ( 'NONE', *, *, #1218, .T. ) ;
+#1286 = CARTESIAN_POINT ( 'NONE', ( -38.47676016858825676, 25.39902294696209140, -5.978028707135908171 ) ) ;
+#1287 = CARTESIAN_POINT ( 'NONE', ( -44.85957031200508993, 6.860130113013363840, 13.55895294178182198 ) ) ;
+#1288 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1289 = CARTESIAN_POINT ( 'NONE', ( 1.750000000000000888, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1290 = AXIS2_PLACEMENT_3D ( 'NONE', #1621, #2009, #2035 ) ;
+#1291 = PLANE ( 'NONE', #2493 ) ;
+#1292 = LINE ( 'NONE', #1955, #2552 ) ;
+#1293 = VECTOR ( 'NONE', #1050, 1000.000000000000000 ) ;
+#1294 = VECTOR ( 'NONE', #1558, 1000.000000000000114 ) ;
+#1295 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732930828, 12.50000000000001243 ) ) ;
+#1296 = ORIENTED_EDGE ( 'NONE', *, *, #2299, .T. ) ;
+#1297 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#1298 = CARTESIAN_POINT ( 'NONE', ( -32.68692989464853582, 33.58835931915862716, -15.17089609013444473 ) ) ;
+#1299 = ORIENTED_EDGE ( 'NONE', *, *, #358, .F. ) ;
+#1300 = AXIS2_PLACEMENT_3D ( 'NONE', #1510, #677, #2390 ) ;
+#1301 = CARTESIAN_POINT ( 'NONE', ( -44.55199895893480289, 7.753472108974767352, -10.26864092019867236 ) ) ;
+#1302 = FACE_OUTER_BOUND ( 'NONE', #1911, .T. ) ;
+#1303 = ORIENTED_EDGE ( 'NONE', *, *, #823, .T. ) ;
+#1304 = EDGE_CURVE ( 'NONE', #175, #2480, #2743, .T. ) ;
+#1305 = CARTESIAN_POINT ( 'NONE', ( 42.85920530509994819, 6.768216077038371914, 13.74952544841718982 ) ) ;
+#1306 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1307 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1308 = FACE_OUTER_BOUND ( 'NONE', #2652, .T. ) ;
+#1309 = ADVANCED_FACE ( 'NONE', ( #2150 ), #1099, .T. ) ;
+#1310 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 25.16419609954003533, 17.99999999999999645 ) ) ;
+#1311 = CARTESIAN_POINT ( 'NONE', ( -35.09520810662709067, 34.18176677009029873, -18.21823116254233454 ) ) ;
+#1312 = DIRECTION ( 'NONE', ( -0.3348729792147802642, -0.9422632794457275196, 0.000000000000000000 ) ) ;
+#1313 = CARTESIAN_POINT ( 'NONE', ( -33.45976740520268322, 34.96997971622484158, -17.47753426087511386 ) ) ;
+#1314 = CARTESIAN_POINT ( 'NONE', ( 43.67796458834889961, 10.29210778281630034, 12.19103034307616262 ) ) ;
+#1315 = VERTEX_POINT ( 'NONE', #2533 ) ;
+#1316 = CARTESIAN_POINT ( 'NONE', ( -36.36104731350425112, 31.54411781998823372, -6.320033347511607502 ) ) ;
+#1317 = CARTESIAN_POINT ( 'NONE', ( -40.85494385521263183, 13.64803341803676062, 16.87309194081379005 ) ) ;
+#1318 = ORIENTED_EDGE ( 'NONE', *, *, #60, .F. ) ;
+#1319 = CARTESIAN_POINT ( 'NONE', ( 44.84138201281256642, 6.912958087210610003, 13.66377469286522839 ) ) ;
+#1320 = ADVANCED_FACE ( 'NONE', ( #627, #1507 ), #888, .F. ) ;
+#1321 = DIRECTION ( 'NONE', ( -0.3663045011995059719, 0.9304950362043751255, 1.291321266866894766E-15 ) ) ;
+#1322 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -6.532393833667063276 ) ) ;
+#1323 = CIRCLE ( 'NONE', #2217, 0.9999999999999987788 ) ;
+#1324 = CARTESIAN_POINT ( 'NONE', ( -31.99999999999999289, 44.04346725332046475, 15.72182540694797659 ) ) ;
+#1325 = EDGE_CURVE ( 'NONE', #1933, #988, #570, .T. ) ;
+#1326 = EDGE_LOOP ( 'NONE', ( #208, #2136 ) ) ;
+#1327 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, 15.25000000000000355 ) ) ;
+#1328 = CARTESIAN_POINT ( 'NONE', ( -33.27732909717479259, 40.50078741505534197, 8.535066353113485604 ) ) ;
+#1329 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1330 = CARTESIAN_POINT ( 'NONE', ( 41.32601150491486663, 17.12336316791884272, 18.34241424289190903 ) ) ;
+#1331 = CARTESIAN_POINT ( 'NONE', ( -34.29682942451555050, 33.88018324366031919, -16.97263664770851932 ) ) ;
+#1332 = CARTESIAN_POINT ( 'NONE', ( -42.43541227556343642, 8.222925807528207898, 14.93394762038457912 ) ) ;
+#1333 = ORIENTED_EDGE ( 'NONE', *, *, #2671, .T. ) ;
+#1334 = CARTESIAN_POINT ( 'NONE', ( -31.62984376346780024, 36.62285101022548872, -13.44281979267885951 ) ) ;
+#1335 = CARTESIAN_POINT ( 'NONE', ( -31.67128112610105717, 36.36471689561647480, -13.09457092943240930 ) ) ;
+#1336 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1337 = CYLINDRICAL_SURFACE ( 'NONE', #940, 5.499999999999994671 ) ;
+#1338 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 0.000000000000000000, -20.50000000000000000 ) ) ;
+#1339 = EDGE_LOOP ( 'NONE', ( #1148, #2575 ) ) ;
+#1340 = ADVANCED_FACE ( 'NONE', ( #1085 ), #793, .T. ) ;
+#1341 = FACE_OUTER_BOUND ( 'NONE', #2138, .T. ) ;
+#1342 = AXIS2_PLACEMENT_3D ( 'NONE', #1306, #1709, #1336 ) ;
+#1343 = CARTESIAN_POINT ( 'NONE', ( -32.65564714380936806, 42.30646467183075288, 9.856350478464486642 ) ) ;
+#1344 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, 20.50000000000000000 ) ) ;
+#1345 = CARTESIAN_POINT ( 'NONE', ( 42.41046231078216522, 13.97357227301046834, 17.66819956641655054 ) ) ;
+#1346 = CARTESIAN_POINT ( 'NONE', ( -33.06795571089505614, 33.85032747006412279, -15.76553517146966499 ) ) ;
+#1347 = CARTESIAN_POINT ( 'NONE', ( -42.27964029246207645, 14.35354525096323464, 15.78330155395758894 ) ) ;
+#1348 = CARTESIAN_POINT ( 'NONE', ( -32.05179640478741021, 32.45198919690344042, -11.73948780598515818 ) ) ;
+#1349 = CARTESIAN_POINT ( 'NONE', ( -32.97952337561983427, 35.01307201903078692, -7.803581502939895387 ) ) ;
+#1350 = CIRCLE ( 'NONE', #1701, 2.750000000000002665 ) ;
+#1351 = DIRECTION ( 'NONE', ( -0.9304950362043749035, -0.3663045011995061939, 0.000000000000000000 ) ) ;
+#1352 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1353 = CARTESIAN_POINT ( 'NONE', ( 32.55641871737685022, 42.13350728233955067, 14.93733163443754108 ) ) ;
+#1354 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #386, #2515, #2432, #593 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 3.141592653589793116, 6.283185307179586232 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.3333333333333333703, 0.3333333333333333703, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1355 = EDGE_LOOP ( 'NONE', ( #1472, #1066 ) ) ;
+#1356 = CARTESIAN_POINT ( 'NONE', ( -31.23772755445721216, 34.40362048588406907, -15.69215289719728545 ) ) ;
+#1357 = CARTESIAN_POINT ( 'NONE', ( -39.29637667370386112, 23.01844414047110021, 6.000000000000002665 ) ) ;
+#1358 = ADVANCED_FACE ( 'NONE', ( #1620, #1845 ), #1192, .F. ) ;
+#1359 = CARTESIAN_POINT ( 'NONE', ( 42.71092435547765831, 7.277204487012764567, 14.26880262041515124 ) ) ;
+#1360 = CARTESIAN_POINT ( 'NONE', ( -32.68724500943380207, 33.58878436520782884, -15.17162741788891012 ) ) ;
+#1361 = CARTESIAN_POINT ( 'NONE', ( -43.29723134156521525, 11.39794873718619073, 13.99145351984430974 ) ) ;
+#1362 = MANIFOLD_SOLID_BREP ( '̨گ5', #2686 ) ;
+#1363 = CARTESIAN_POINT ( 'NONE', ( -35.89971384576185898, 32.88406237842245616, -17.79979218800362872 ) ) ;
+#1364 = CARTESIAN_POINT ( 'NONE', ( -30.49767916172051940, 33.60471447115438082, -10.41357431976148007 ) ) ;
+#1365 = LINE ( 'NONE', #2008, #312 ) ;
+#1366 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1367 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.21079393477482000, 12.49999999999999822 ) ) ;
+#1368 = AXIS2_PLACEMENT_3D ( 'NONE', #2363, #236, #1039 ) ;
+#1369 = VERTEX_POINT ( 'NONE', #2063 ) ;
+#1370 = VECTOR ( 'NONE', #305, 1000.000000000000000 ) ;
+#1371 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1372 = FACE_OUTER_BOUND ( 'NONE', #1443, .T. ) ;
+#1373 = CARTESIAN_POINT ( 'NONE', ( -42.57530924774837899, 13.49477380811439708, 15.37803231392795844 ) ) ;
+#1374 = ADVANCED_FACE ( 'NONE', ( #1636 ), #768, .T. ) ;
+#1375 = CARTESIAN_POINT ( 'NONE', ( -31.98810384804529861, 37.35610748556290162, -12.77690211619579941 ) ) ;
+#1376 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101608683, -11.01637359942223782 ) ) ;
+#1377 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1378 = FACE_OUTER_BOUND ( 'NONE', #2083, .T. ) ;
+#1379 = ORIENTED_EDGE ( 'NONE', *, *, #279, .T. ) ;
+#1380 = VERTEX_POINT ( 'NONE', #601 ) ;
+#1381 = AXIS2_PLACEMENT_3D ( 'NONE', #607, #1228, #2556 ) ;
+#1382 = CARTESIAN_POINT ( 'NONE', ( -44.84138201281255220, 6.912958087210613556, 11.33622530713477339 ) ) ;
+#1383 = AXIS2_PLACEMENT_3D ( 'NONE', #2736, #1673, #1469 ) ;
+#1384 = CARTESIAN_POINT ( 'NONE', ( -32.00003383277233837, 32.30167251210357193, -12.61409455811673119 ) ) ;
+#1385 = AXIS2_PLACEMENT_3D ( 'NONE', #1968, #2415, #1939 ) ;
+#1386 = CYLINDRICAL_SURFACE ( 'NONE', #1601, 32.50000000000000000 ) ;
+#1387 = CARTESIAN_POINT ( 'NONE', ( -36.91745922585884188, 29.92801760975215331, -18.04275952704307073 ) ) ;
+#1388 = CARTESIAN_POINT ( 'NONE', ( -30.85095568375334452, 34.05872916592048938, -9.816984474666185179 ) ) ;
+#1389 = CARTESIAN_POINT ( 'NONE', ( -35.36679846747600209, 33.89153525923114074, -18.31250398666472989 ) ) ;
+#1390 = EDGE_CURVE ( 'NONE', #594, #1060, #1030, .T. ) ;
+#1391 = CIRCLE ( 'NONE', #2378, 1.000000000000000888 ) ;
+#1392 = VECTOR ( 'NONE', #733, 1000.000000000000000 ) ;
+#1393 = DIRECTION ( 'NONE', ( 0.9422632794457277416, -0.3348729792147797646, 0.000000000000000000 ) ) ;
+#1394 = ADVANCED_FACE ( 'NONE', ( #1998 ), #1144, .T. ) ;
+#1395 = ORIENTED_EDGE ( 'NONE', *, *, #1595, .T. ) ;
+#1396 =( LENGTH_UNIT ( ) NAMED_UNIT ( * ) SI_UNIT ( .MILLI., .METRE. ) );
+#1397 = CARTESIAN_POINT ( 'NONE', ( -42.43541227556363538, 8.222925807528154607, 10.06605237961579391 ) ) ;
+#1398 = CARTESIAN_POINT ( 'NONE', ( -34.64959837723355918, 36.51502682517340759, 3.381018314199685015 ) ) ;
+#1399 = CARTESIAN_POINT ( 'NONE', ( -32.85740444909854574, 41.72045992200442299, 15.48591936326438301 ) ) ;
+#1400 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 12.26125813606935644, -12.49999999999993783 ) ) ;
+#1401 = CARTESIAN_POINT ( 'NONE', ( -35.36682429458187471, 33.89153810191015026, -6.687484199460998369 ) ) ;
+#1402 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, 0.000000000000000000 ) ) ;
+#1403 = CARTESIAN_POINT ( 'NONE', ( -34.22735686600162097, 34.77002330469203173, -7.130526569817990712 ) ) ;
+#1404 = CARTESIAN_POINT ( 'NONE', ( 37.59468065372185919, 27.96102576855563626, 19.00000000000000000 ) ) ;
+#1405 = ORIENTED_EDGE ( 'NONE', *, *, #1730, .F. ) ;
+#1406 = EDGE_CURVE ( 'NONE', #1250, #699, #130, .T. ) ;
+#1407 = CARTESIAN_POINT ( 'NONE', ( -43.34254294013565101, 11.26634105166415267, 8.204626120997501104 ) ) ;
+#1408 = ORIENTED_EDGE ( 'NONE', *, *, #581, .F. ) ;
+#1409 = LINE ( 'NONE', #563, #1392 ) ;
+#1410 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1411 = ORIENTED_EDGE ( 'NONE', *, *, #1595, .F. ) ;
+#1412 = CYLINDRICAL_SURFACE ( 'NONE', #276, 5.499999999999996447 ) ;
+#1413 = CARTESIAN_POINT ( 'NONE', ( -35.76995884981808160, 33.24484818809215625, -7.244799766026903676 ) ) ;
+#1414 = CARTESIAN_POINT ( 'NONE', ( -34.95333993191496091, 34.33593556534344771, -18.16890658897331789 ) ) ;
+#1415 = CARTESIAN_POINT ( 'NONE', ( 42.43541227556343642, 8.222925807528882913, 10.06605237961542265 ) ) ;
+#1416 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688394565, 32.64726791736439537, -17.54951290997401614 ) ) ;
+#1417 = CARTESIAN_POINT ( 'NONE', ( -42.92657441282920416, 6.536965231182059632, -13.40284925035812158 ) ) ;
+#1418 = ORIENTED_EDGE ( 'NONE', *, *, #842, .F. ) ;
+#1419 =( NAMED_UNIT ( * ) PLANE_ANGLE_UNIT ( ) SI_UNIT ( $, .RADIAN. ) );
+#1420 = CARTESIAN_POINT ( 'NONE', ( -44.53214698414783612, 7.811132234350331771, 10.20907795478963287 ) ) ;
+#1421 = ORIENTED_EDGE ( 'NONE', *, *, #2425, .T. ) ;
+#1422 = ORIENTED_EDGE ( 'NONE', *, *, #243, .F. ) ;
+#1423 = CARTESIAN_POINT ( 'NONE', ( -32.06623647702694768, 32.49411535304783172, -13.37737081519266802 ) ) ;
+#1424 = EDGE_CURVE ( 'NONE', #2480, #1060, #259, .T. ) ;
+#1425 = FACE_OUTER_BOUND ( 'NONE', #317, .T. ) ;
+#1426 = AXIS2_PLACEMENT_3D ( 'NONE', #2209, #874, #260 ) ;
+#1427 = EDGE_CURVE ( 'NONE', #2068, #1933, #688, .T. ) ;
+#1428 = COLOUR_RGB ( '',0.7921568627450980005, 0.8196078431372548767, 0.9333333333333333481 ) ;
+#1429 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1255, #2527, #2086, #427 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 0.000000000000000000, 3.141592653589793116 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.3333333333333333703, 0.3333333333333333703, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1430 = ORIENTED_EDGE ( 'NONE', *, *, #1892, .T. ) ;
+#1431 = CARTESIAN_POINT ( 'NONE', ( -43.30319550006319673, 11.38062581941293594, -13.88728319995371230 ) ) ;
+#1432 = CARTESIAN_POINT ( 'NONE', ( -31.85365882742952692, 35.73721022458634167, -12.98227696002299503 ) ) ;
+#1433 = CARTESIAN_POINT ( 'NONE', ( 44.93248048743244283, 6.648362271203773766, 12.07483222645056919 ) ) ;
+#1434 = CARTESIAN_POINT ( 'NONE', ( 41.62144033390043774, 16.26528917268629115, 8.515399109952957346 ) ) ;
+#1435 = CARTESIAN_POINT ( 'NONE', ( -37.52642665797561961, 25.07348409198810657, -18.01859109396136205 ) ) ;
+#1436 = EDGE_LOOP ( 'NONE', ( #742, #730, #2354, #2336 ) ) ;
+#1437 = CARTESIAN_POINT ( 'NONE', ( -42.51245935571760981, 7.958454404286082529, 10.22895474377723701 ) ) ;
+#1438 = VERTEX_POINT ( 'NONE', #2388 ) ;
+#1439 = ORIENTED_EDGE ( 'NONE', *, *, #1107, .F. ) ;
+#1440 = LINE ( 'NONE', #166, #811 ) ;
+#1441 = FACE_OUTER_BOUND ( 'NONE', #184, .T. ) ;
+#1442 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, 15.25000000000000355 ) ) ;
+#1443 = EDGE_LOOP ( 'NONE', ( #368, #46, #1855, #431 ) ) ;
+#1444 = CARTESIAN_POINT ( 'NONE', ( -42.98248968273709636, 6.345030768959085243, -12.04706125634183600 ) ) ;
+#1445 = EDGE_CURVE ( 'NONE', #383, #1520, #453, .T. ) ;
+#1446 = CARTESIAN_POINT ( 'NONE', ( -31.71510282745170173, 36.15765530396839722, -12.21586280284262394 ) ) ;
+#1447 = CARTESIAN_POINT ( 'NONE', ( 44.60389381109550300, 7.602743341986022862, 10.40959690314570452 ) ) ;
+#1448 = CARTESIAN_POINT ( 'NONE', ( 37.59468065372130496, 27.96102576855725275, 6.000000000000002665 ) ) ;
+#1449 = CARTESIAN_POINT ( 'NONE', ( -42.77379477138979524, 7.061395827093332578, -14.06522692799743446 ) ) ;
+#1450 = ORIENTED_EDGE ( 'NONE', *, *, #766, .F. ) ;
+#1451 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#1452 = ORIENTED_EDGE ( 'NONE', *, *, #2717, .F. ) ;
+#1453 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -18.46760616633223506 ) ) ;
+#1454 = CYLINDRICAL_SURFACE ( 'NONE', #1121, 2.750000000000002665 ) ;
+#1455 = LINE ( 'NONE', #1022, #1744 ) ;
+#1456 = AXIS2_PLACEMENT_3D ( 'NONE', #665, #622, #458 ) ;
+#1457 = AXIS2_PLACEMENT_3D ( 'NONE', #1162, #134, #2448 ) ;
+#1458 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #18, #232, #1324, #861 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 1.570796326794896780, 3.141592653589793116 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243650485, 0.8047378541243650485, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1459 = VERTEX_POINT ( 'NONE', #675 ) ;
+#1460 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371259370, 6.610463800733696438, -12.36604921272668456 ) ) ;
+#1461 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945760107740, 12.58999354329094089 ) ) ;
+#1462 = EDGE_CURVE ( 'NONE', #2211, #1060, #2179, .T. ) ;
+#1463 = CARTESIAN_POINT ( 'NONE', ( 44.94291777066050031, 6.618047148100503208, 12.28728800729299842 ) ) ;
+#1464 = CARTESIAN_POINT ( 'NONE', ( 40.85494385521174365, 13.64803341803679970, 8.126908059185613098 ) ) ;
+#1465 = CARTESIAN_POINT ( 'NONE', ( -43.41833536246619474, 11.04620171434411446, -16.69439683086076798 ) ) ;
+#1466 = ORIENTED_EDGE ( 'NONE', *, *, #1519, .T. ) ;
+#1467 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759554404, 12.50000000000001066 ) ) ;
+#1468 = ORIENTED_EDGE ( 'NONE', *, *, #23, .T. ) ;
+#1469 = DIRECTION ( 'NONE', ( -1.000000000000000000, -8.673617379884035472E-16, 0.000000000000000000 ) ) ;
+#1470 = CYLINDRICAL_SURFACE ( 'NONE', #617, 1.750000000000000888 ) ;
+#1471 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1472 = ORIENTED_EDGE ( 'NONE', *, *, #2391, .T. ) ;
+#1473 = CARTESIAN_POINT ( 'NONE', ( -43.41833536246595315, 11.04620171434483034, -8.305603169138516151 ) ) ;
+#1474 = CARTESIAN_POINT ( 'NONE', ( -42.95367559951917968, 6.443937839889390062, 13.21799811442427774 ) ) ;
+#1475 = AXIS2_PLACEMENT_3D ( 'NONE', #1674, #401, #2751 ) ;
+#1476 = PRESENTATION_LAYER_ASSIGNMENT ( '', '', ( #1028 ) ) ;
+#1477 = CARTESIAN_POINT ( 'NONE', ( 43.47300727646540963, 10.88740696220810023, 11.07271280387980994 ) ) ;
+#1478 = CARTESIAN_POINT ( 'NONE', ( 32.60311442061276921, 42.45904613731270416, 9.619517159301420151 ) ) ;
+#1479 = CARTESIAN_POINT ( 'NONE', ( -42.54375550167716824, 7.851027417805985564, -14.70497549800531978 ) ) ;
+#1480 = ORIENTED_EDGE ( 'NONE', *, *, #1462, .F. ) ;
+#1481 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #2030, #2263, #2750, #1453 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 0.000000000000000000, 1.978349873612916143 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.6995862986750021051, 0.6995862986750021051, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1482 = EDGE_CURVE ( 'NONE', #40, #1947, #465, .T. ) ;
+#1483 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#1484 = ORIENTED_EDGE ( 'NONE', *, *, #917, .T. ) ;
+#1485 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 5.000000000000000000, -20.50000000000000000 ) ) ;
+#1486 = AXIS2_PLACEMENT_3D ( 'NONE', #2579, #1941, #2376 ) ;
+#1487 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1488 = ORIENTED_EDGE ( 'NONE', *, *, #1889, .F. ) ;
+#1489 = CARTESIAN_POINT ( 'NONE', ( -38.87646853286444326, 20.43933743288632954, -7.155761943118373658 ) ) ;
+#1490 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1491 = AXIS2_PLACEMENT_3D ( 'NONE', #1745, #1972, #705 ) ;
+#1492 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, -12.50000000000000000 ) ) ;
+#1493 = CARTESIAN_POINT ( 'NONE', ( 32.33154110755113209, 43.24783170891586082, 10.57274774848178112 ) ) ;
+#1494 = CARTESIAN_POINT ( 'NONE', ( -42.97198885086697118, 6.381075869361912289, -13.06584860415254923 ) ) ;
+#1495 = EDGE_LOOP ( 'NONE', ( #1639, #2118, #148, #623 ) ) ;
+#1496 = FACE_OUTER_BOUND ( 'NONE', #2365, .T. ) ;
+#1497 = ORIENTED_EDGE ( 'NONE', *, *, #1767, .T. ) ;
+#1498 = ADVANCED_FACE ( 'NONE', ( #2208 ), #873, .F. ) ;
+#1499 = CARTESIAN_POINT ( 'NONE', ( -1.750000000000000888, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#1500 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1501 = CARTESIAN_POINT ( 'NONE', ( -32.18303208580277897, 35.36076419269976867, -14.13461323153536142 ) ) ;
+#1502 = ORIENTED_EDGE ( 'NONE', *, *, #1445, .F. ) ;
+#1503 = CARTESIAN_POINT ( 'NONE', ( -42.70483288625752749, 7.298114032309239718, -10.71883149225044818 ) ) ;
+#1504 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1505 = VECTOR ( 'NONE', #1791, 1000.000000000000000 ) ;
+#1506 = EDGE_LOOP ( 'NONE', ( #2259, #1480, #1210, #2503 ) ) ;
+#1507 = FACE_BOUND ( 'NONE', #582, .T. ) ;
+#1508 = CARTESIAN_POINT ( 'NONE', ( 13.87540813888669788, 5.577367205542728357, -20.50000000000000355 ) ) ;
+#1509 = FACE_BOUND ( 'NONE', #1893, .T. ) ;
+#1510 = CARTESIAN_POINT ( 'NONE', ( 17.17436489607392147, 9.711316397228637598, -0.5000000000000004441 ) ) ;
+#1511 = DIRECTION ( 'NONE', ( -0.3255388549733763126, 0.9455286637128580418, 0.000000000000000000 ) ) ;
+#1512 = ORIENTED_EDGE ( 'NONE', *, *, #142, .F. ) ;
+#1513 = DIRECTION ( 'NONE', ( -0.9422632794457277416, 0.3348729792147803752, 0.000000000000000000 ) ) ;
+#1514 = EDGE_CURVE ( 'NONE', #2559, #2469, #643, .T. ) ;
+#1515 = CARTESIAN_POINT ( 'NONE', ( -34.24126366280394507, 33.90236320094390265, -16.93223485108799053 ) ) ;
+#1516 = VERTEX_POINT ( 'NONE', #889 ) ;
+#1517 = CARTESIAN_POINT ( 'NONE', ( -36.40037353085005378, 31.42989469371310918, -7.042263587034935490 ) ) ;
+#1518 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#1519 = EDGE_CURVE ( 'NONE', #1823, #30, #2238, .T. ) ;
+#1520 = VERTEX_POINT ( 'NONE', #2193 ) ;
+#1521 = CARTESIAN_POINT ( 'NONE', ( 42.99779078280187150, 6.292508293126906516, 12.67998707075203413 ) ) ;
+#1522 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1523 = TOROIDAL_SURFACE ( 'NONE', #2456, 27.50000000000000000, 5.000000000000000000 ) ;
+#1524 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1525 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 12.26125813606935644, -12.49999999999993783 ) ) ;
+#1526 = CIRCLE ( 'NONE', #942, 2.750000000000002665 ) ;
+#1527 = VECTOR ( 'NONE', #1117, 1000.000000000000000 ) ;
+#1528 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1529 = CARTESIAN_POINT ( 'NONE', ( -35.22101607802353129, 34.05608898746584146, -18.26251427131116145 ) ) ;
+#1530 = CARTESIAN_POINT ( 'NONE', ( -32.19256952747925027, 32.87104776304519049, -11.04251005918754203 ) ) ;
+#1531 = CARTESIAN_POINT ( 'NONE', ( -30.70022793175422038, 33.88660822880560630, -14.94915297329862547 ) ) ;
+#1532 = CARTESIAN_POINT ( 'NONE', ( 40.25632870800274077, 15.70283663275023933, 17.27307379451436020 ) ) ;
+#1533 = ORIENTED_EDGE ( 'NONE', *, *, #213, .F. ) ;
+#1534 = AXIS2_PLACEMENT_3D ( 'NONE', #891, #205, #2758 ) ;
+#1535 = CARTESIAN_POINT ( 'NONE', ( -37.52642665797190347, 25.07348409198746353, -6.981408906038309325 ) ) ;
+#1536 = CARTESIAN_POINT ( 'NONE', ( -41.62144033390046616, 16.26528917268619523, 16.48460089004885276 ) ) ;
+#1537 = EDGE_LOOP ( 'NONE', ( #1062, #2126 ) ) ;
+#1538 = ADVANCED_FACE ( 'NONE', ( #2358 ), #2374, .F. ) ;
+#1539 = CARTESIAN_POINT ( 'NONE', ( 42.98895923545501319, 6.322823416230617610, 12.85975734684958560 ) ) ;
+#1540 = CARTESIAN_POINT ( 'NONE', ( -25.58861349562033283, 34.92662621701362724, -0.4999999999999995559 ) ) ;
+#1541 = ORIENTED_EDGE ( 'NONE', *, *, #2467, .T. ) ;
+#1542 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -0.5000000000000004441 ) ) ;
+#1543 = CARTESIAN_POINT ( 'NONE', ( 39.93733163486151483, 16.79782431294496803, 17.44358128244753203 ) ) ;
+#1544 = CARTESIAN_POINT ( 'NONE', ( -32.39959609828572695, 33.25666577701638715, -14.56947775229932240 ) ) ;
+#1545 = CARTESIAN_POINT ( 'NONE', ( -40.17685806913015512, 20.46108306200315141, 17.52236565250630562 ) ) ;
+#1546 = CARTESIAN_POINT ( 'NONE', ( -32.67722337217189477, 34.98896024521154402, -8.005502686623907849 ) ) ;
+#1547 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1548 = EDGE_CURVE ( 'NONE', #2469, #410, #2400, .T. ) ;
+#1549 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759554404, -12.50000000000000000 ) ) ;
+#1550 = ORIENTED_EDGE ( 'NONE', *, *, #1012, .F. ) ;
+#1551 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1552 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -6.532393833667063276 ) ) ;
+#1553 = CARTESIAN_POINT ( 'NONE', ( 36.74416741500115080, 30.43134423835383728, 18.83082239818627102 ) ) ;
+#1554 = CARTESIAN_POINT ( 'NONE', ( -32.19252059299407165, 32.87090085773285608, -13.95728121050578530 ) ) ;
+#1555 = CARTESIAN_POINT ( 'NONE', ( -40.25632870800297525, 15.70283663275114172, 17.27307379451420033 ) ) ;
+#1556 = CARTESIAN_POINT ( 'NONE', ( -30.21608528462837029, 33.03158952519265057, -11.12630729510718375 ) ) ;
+#1557 = CARTESIAN_POINT ( 'NONE', ( -33.20189256992523497, 33.90985971657129028, -9.059882940539411678 ) ) ;
+#1558 = DIRECTION ( 'NONE', ( -0.3255388549733763126, 0.9455286637128580418, 0.000000000000000000 ) ) ;
+#1559 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, -12.50000000000000000 ) ) ;
+#1560 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000147793, 44.21079393474678909, 12.89178716040784067 ) ) ;
+#1561 = EDGE_CURVE ( 'NONE', #254, #175, #1755, .T. ) ;
+#1562 = AXIS2_PLACEMENT_3D ( 'NONE', #17, #1726, #934 ) ;
+#1563 = ORIENTED_EDGE ( 'NONE', *, *, #447, .F. ) ;
+#1564 = AXIS2_PLACEMENT_3D ( 'NONE', #693, #2184, #2600 ) ;
+#1565 = B_SPLINE_CURVE_WITH_KNOTS ( 'NONE', 3,
+ ( #505, #1554, #491, #1544, #2397, #1360, #2427, #1346, #1779, #697, #684, #1979, #915, #1331, #2204, #58, #474, #1992, #901, #2188, #2633, #1751, #2606, #282 ),
+ .UNSPECIFIED., .F., .F.,
+ ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 0.000000000000000000, 0.0007532897161176469293, 0.001506579432235293859, 0.002259869148352940896, 0.003013158864470592054, 0.003766448580588242778, 0.004143093438647067056, 0.004519738296705892201, 0.004896383154764717346, 0.005273028012823542492, 0.005649672870882366769, 0.006026317728941191915 ),
+ .UNSPECIFIED. ) ;
+#1566 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, 12.50000000000000000 ) ) ;
+#1567 = CARTESIAN_POINT ( 'NONE', ( -37.59468065372185919, 27.96102576855560784, 6.000000000000002665 ) ) ;
+#1568 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, -20.50000000000000355 ) ) ;
+#1569 = CARTESIAN_POINT ( 'NONE', ( 44.60389381109546747, 7.602743341986131220, 14.59040309685441983 ) ) ;
+#1570 = CARTESIAN_POINT ( 'NONE', ( -1.750000000000000888, 65.00000000000000000, 4.500000000000000888 ) ) ;
+#1571 = CARTESIAN_POINT ( 'NONE', ( -34.45097498342182973, 37.09192911890504263, 17.69783447513438190 ) ) ;
+#1572 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #836, #794, #2045, #2741 ),
+ .UNSPECIFIED., .F., .T. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 3.141592653589793116, 4.712388980384689674 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1573 = CARTESIAN_POINT ( 'NONE', ( -31.66928218820409668, 36.70098412075729044, -13.44889173107035418 ) ) ;
+#1574 = CARTESIAN_POINT ( 'NONE', ( -31.85281068133371818, 35.73916661103654491, -12.02183021318687572 ) ) ;
+#1575 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1576 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#1577 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1578 = ORIENTED_EDGE ( 'NONE', *, *, #517, .T. ) ;
+#1579 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1580 = CARTESIAN_POINT ( 'NONE', ( -32.02716457785286508, 32.38016798610966873, -11.94381954748692465 ) ) ;
+#1581 = EDGE_CURVE ( 'NONE', #652, #383, #2611, .T. ) ;
+#1582 = CARTESIAN_POINT ( 'NONE', ( -43.65830555055399742, 10.34920752207545291, 12.45125492978656112 ) ) ;
+#1583 = CIRCLE ( 'NONE', #2323, 32.50000000000000000 ) ;
+#1584 = FACE_OUTER_BOUND ( 'NONE', #792, .T. ) ;
+#1585 = CARTESIAN_POINT ( 'NONE', ( -30.28356930380876122, 33.20301396760286394, -10.89557269667217376 ) ) ;
+#1586 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1587 = CYLINDRICAL_SURFACE ( 'NONE', #1161, 5.499999999999996447 ) ;
+#1588 = AXIS2_PLACEMENT_3D ( 'NONE', #444, #1297, #428 ) ;
+#1589 = VERTEX_POINT ( 'NONE', #272 ) ;
+#1590 = CARTESIAN_POINT ( 'NONE', ( -33.03257378525533738, 31.99619020741757680, 4.500000000000000888 ) ) ;
+#1591 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, 20.50000000000000000 ) ) ;
+#1592 = CARTESIAN_POINT ( 'NONE', ( -32.15342044700649637, 43.76518374714867576, 14.18533669406339648 ) ) ;
+#1593 = EDGE_CURVE ( 'NONE', #2155, #1213, #2314, .T. ) ;
+#1594 = CARTESIAN_POINT ( 'NONE', ( -32.02649951626669633, 32.37823574882894206, -13.04972978295509556 ) ) ;
+#1595 = EDGE_CURVE ( 'NONE', #699, #290, #2165, .T. ) ;
+#1596 = CARTESIAN_POINT ( 'NONE', ( -30.62729709082692864, 33.79276614032308146, -10.17409299487224317 ) ) ;
+#1597 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1598 = CARTESIAN_POINT ( 'NONE', ( -35.59828801170483104, 33.57256264274568025, -17.69015334906597303 ) ) ;
+#1599 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -6.532393833667063276 ) ) ;
+#1600 = ORIENTED_EDGE ( 'NONE', *, *, #828, .T. ) ;
+#1601 = AXIS2_PLACEMENT_3D ( 'NONE', #746, #552, #1410 ) ;
+#1602 = CARTESIAN_POINT ( 'NONE', ( -42.27964029246378175, 14.35354525096438749, 9.216698446042197901 ) ) ;
+#1603 = CIRCLE ( 'NONE', #710, 30.00000000000000711 ) ;
+#1604 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 0.000000000000000000, 20.50000000000000000 ) ) ;
+#1605 = VECTOR ( 'NONE', #1597, 1000.000000000000000 ) ;
+#1606 = CARTESIAN_POINT ( 'NONE', ( -32.60311442061345133, 42.45904613731072885, 15.38048284069858163 ) ) ;
+#1607 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1608 = CARTESIAN_POINT ( 'NONE', ( -35.49713565893312506, 33.72827515132273390, -7.350494171305977709 ) ) ;
+#1609 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, 17.99999999999999645 ) ) ;
+#1610 = EDGE_CURVE ( 'NONE', #2404, #2692, #2596, .T. ) ;
+#1611 = AXIS2_PLACEMENT_3D ( 'NONE', #1559, #2612, #1307 ) ;
+#1612 = CARTESIAN_POINT ( 'NONE', ( -32.50468978478926374, 34.95322199951620235, -8.135956506852453529 ) ) ;
+#1613 = CARTESIAN_POINT ( 'NONE', ( 33.27732909717450127, 40.50078741505620172, 16.46493364688651440 ) ) ;
+#1614 = VERTEX_POINT ( 'NONE', #102 ) ;
+#1615 = CARTESIAN_POINT ( 'NONE', ( -44.77913493337644724, 7.093754932011393954, 11.02328810641593471 ) ) ;
+#1616 = ORIENTED_EDGE ( 'NONE', *, *, #504, .T. ) ;
+#1617 = LINE ( 'NONE', #2484, #599 ) ;
+#1618 = CARTESIAN_POINT ( 'NONE', ( -33.35637253533404589, 39.38759000239074481, 16.13454712838606753 ) ) ;
+#1619 = CARTESIAN_POINT ( 'NONE', ( -44.92483465240199081, 6.670569623932487424, -11.96470875749483653 ) ) ;
+#1620 = FACE_OUTER_BOUND ( 'NONE', #133, .T. ) ;
+#1621 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#1622 = CARTESIAN_POINT ( 'NONE', ( 44.85957031200508993, 6.860130113013416242, 11.44104705821817980 ) ) ;
+#1623 = EDGE_CURVE ( 'NONE', #1174, #1838, #1065, .T. ) ;
+#1624 = CARTESIAN_POINT ( 'NONE', ( -33.91003494827299392, 34.88364372896455023, -7.279559714055275244 ) ) ;
+#1625 = CARTESIAN_POINT ( 'NONE', ( -42.88477153647213669, 6.680457565318644875, -13.62564742563747622 ) ) ;
+#1626 = VERTEX_POINT ( 'NONE', #1647 ) ;
+#1627 = CIRCLE ( 'NONE', #1456, 2.750000000000002665 ) ;
+#1628 = CARTESIAN_POINT ( 'NONE', ( -40.14688991242673666, 20.54812567066662510, 6.169177601812665834 ) ) ;
+#1629 = ORIENTED_EDGE ( 'NONE', *, *, #2010, .F. ) ;
+#1630 = VERTEX_POINT ( 'NONE', #2219 ) ;
+#1631 = CARTESIAN_POINT ( 'NONE', ( -34.13761404710683678, 38.00208697824251658, 4.130593500387957029 ) ) ;
+#1632 = LINE ( 'NONE', #1805, #2015 ) ;
+#1633 = ORIENTED_EDGE ( 'NONE', *, *, #2437, .T. ) ;
+#1634 = FACE_OUTER_BOUND ( 'NONE', #2278, .T. ) ;
+#1635 = CARTESIAN_POINT ( 'NONE', ( -34.15781794637832292, 34.83372801922882189, -16.87284634255418325 ) ) ;
+#1636 = FACE_OUTER_BOUND ( 'NONE', #2419, .T. ) ;
+#1637 = CARTESIAN_POINT ( 'NONE', ( -35.56856678408814076, 33.63960453043719667, -6.620387693440302534 ) ) ;
+#1638 = CARTESIAN_POINT ( 'NONE', ( -44.87938373321222940, 6.802581966635887767, -13.43458640802096049 ) ) ;
+#1639 = ORIENTED_EDGE ( 'NONE', *, *, #2183, .T. ) ;
+#1640 = CARTESIAN_POINT ( 'NONE', ( -43.57608948206922861, 10.58800436056085736, 12.02167594496184932 ) ) ;
+#1641 = ORIENTED_EDGE ( 'NONE', *, *, #390, .F. ) ;
+#1642 = VERTEX_POINT ( 'NONE', #1442 ) ;
+#1643 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#1644 = EDGE_CURVE ( 'NONE', #2706, #2197, #1650, .T. ) ;
+#1645 = CARTESIAN_POINT ( 'NONE', ( -32.55641871737685733, 42.13350728233731957, 14.93733163443755352 ) ) ;
+#1646 = AXIS2_PLACEMENT_3D ( 'NONE', #497, #33, #658 ) ;
+#1647 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, -17.99999999999999645 ) ) ;
+#1648 = AXIS2_PLACEMENT_3D ( 'NONE', #186, #841, #1706 ) ;
+#1649 = ORIENTED_EDGE ( 'NONE', *, *, #686, .T. ) ;
+#1650 = CIRCLE ( 'NONE', #179, 2.750000000000002665 ) ;
+#1651 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1652 = CARTESIAN_POINT ( 'NONE', ( -34.05321614581736611, 33.96036649347200864, -16.78762747368598696 ) ) ;
+#1653 = CARTESIAN_POINT ( 'NONE', ( 42.26572163226845191, 8.805405019716271653, 9.748583515298605562 ) ) ;
+#1654 = CARTESIAN_POINT ( 'NONE', ( 32.17590843201798378, 43.43964489217341907, 11.07394587425366161 ) ) ;
+#1655 = CARTESIAN_POINT ( 'NONE', ( -44.67819521171887232, 7.386934682066843116, -14.34981364217849276 ) ) ;
+#1656 = VERTEX_POINT ( 'NONE', #2310 ) ;
+#1657 = AXIS2_PLACEMENT_3D ( 'NONE', #2166, #921, #906 ) ;
+#1658 = CARTESIAN_POINT ( 'NONE', ( -43.34545552388709666, 11.25788144268017632, 13.91252425953104677 ) ) ;
+#1659 = ORIENTED_EDGE ( 'NONE', *, *, #485, .F. ) ;
+#1660 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1661 = CARTESIAN_POINT ( 'NONE', ( -30.07468478458828542, 32.68077376088574937, -12.63306344009754589 ) ) ;
+#1662 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#1663 = EDGE_CURVE ( 'NONE', #2512, #851, #1455, .T. ) ;
+#1664 = EDGE_LOOP ( 'NONE', ( #247, #985, #8, #2320 ) ) ;
+#1665 = CARTESIAN_POINT ( 'NONE', ( 4.000000000000001776, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1666 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -12.50000000000000000 ) ) ;
+#1667 = CARTESIAN_POINT ( 'NONE', ( 43.34545552388527057, 11.25788144268264901, 13.91252425952919936 ) ) ;
+#1668 = CARTESIAN_POINT ( 'NONE', ( 32.03545348354649036, 43.92176981164495686, 11.78006058356892360 ) ) ;
+#1669 = CARTESIAN_POINT ( 'NONE', ( -43.89767380833999511, 9.653961638331329809, -16.04906812554881768 ) ) ;
+#1670 = ORIENTED_EDGE ( 'NONE', *, *, #2247, .F. ) ;
+#1671 = CYLINDRICAL_SURFACE ( 'NONE', #775, 4.000000000000000000 ) ;
+#1672 = ORIENTED_EDGE ( 'NONE', *, *, #1251, .F. ) ;
+#1673 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#1674 = CARTESIAN_POINT ( 'NONE', ( 15.50000000000002132, 5.000000000000000000, 79.70475956827036157 ) ) ;
+#1675 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1676 = AXIS2_PLACEMENT_3D ( 'NONE', #1904, #838, #183 ) ;
+#1677 = VERTEX_POINT ( 'NONE', #521 ) ;
+#1678 = CARTESIAN_POINT ( 'NONE', ( -42.11335358391553996, 9.328422783357549264, -9.496942355304755168 ) ) ;
+#1679 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, -0.5000000000000004441 ) ) ;
+#1680 = EDGE_CURVE ( 'NONE', #416, #2102, #1783, .T. ) ;
+#1681 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, -135.3085362679971126 ) ) ;
+#1682 = CARTESIAN_POINT ( 'NONE', ( 41.70300804589821553, 16.02837548772470555, 6.859094606483393086 ) ) ;
+#1683 = CARTESIAN_POINT ( 'NONE', ( -39.54706189465257893, 22.29032810038464874, -17.75361044965400836 ) ) ;
+#1684 = EDGE_LOOP ( 'NONE', ( #692, #2290, #1266, #2236 ) ) ;
+#1685 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#1686 = ORIENTED_EDGE ( 'NONE', *, *, #1548, .F. ) ;
+#1687 = FACE_BOUND ( 'NONE', #1326, .T. ) ;
+#1688 = FACE_OUTER_BOUND ( 'NONE', #812, .T. ) ;
+#1689 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, -15.25000000000000355 ) ) ;
+#1690 = ORIENTED_EDGE ( 'NONE', *, *, #1927, .F. ) ;
+#1691 = CARTESIAN_POINT ( 'NONE', ( -42.66701332672604252, 7.427933254001340302, -10.61192693247562602 ) ) ;
+#1692 = CARTESIAN_POINT ( 'NONE', ( -42.99999999999999289, 6.284924945759550852, -15.72182540694797659 ) ) ;
+#1693 = CIRCLE ( 'NONE', #2191, 2.750000000000002665 ) ;
+#1694 = AXIS2_PLACEMENT_3D ( 'NONE', #2151, #1770, #905 ) ;
+#1695 = DIRECTION ( 'NONE', ( 1.224646799147352714E-16, -3.556990613648025458E-16, -1.000000000000000000 ) ) ;
+#1696 = CARTESIAN_POINT ( 'NONE', ( 35.17200529168162859, 34.99769424202343515, 6.820388475540319462 ) ) ;
+#1697 = CARTESIAN_POINT ( 'NONE', ( 40.72182540694797126, 14.10497510537461352, 18.00000000000000000 ) ) ;
+#1698 = VERTEX_POINT ( 'NONE', #372 ) ;
+#1699 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1700 = VERTEX_POINT ( 'NONE', #580 ) ;
+#1701 = AXIS2_PLACEMENT_3D ( 'NONE', #719, #2210, #300 ) ;
+#1702 = CARTESIAN_POINT ( 'NONE', ( -33.06726107370904089, 33.84983563589852196, -9.235563272937660884 ) ) ;
+#1703 = CARTESIAN_POINT ( 'NONE', ( -33.05945178009036312, 33.84573068575826937, -15.75357449116700259 ) ) ;
+#1704 = EDGE_LOOP ( 'NONE', ( #566, #302, #2079, #129 ) ) ;
+#1705 = CARTESIAN_POINT ( 'NONE', ( -41.04433338031164880, 17.94149819751921981, -8.028179518177136487 ) ) ;
+#1706 = DIRECTION ( 'NONE', ( -0.3348729792147795981, -0.9422632794457278527, 0.000000000000000000 ) ) ;
+#1707 = ORIENTED_EDGE ( 'NONE', *, *, #413, .F. ) ;
+#1708 = CARTESIAN_POINT ( 'NONE', ( 44.94291777066060689, 6.618047148100196786, 12.71271199270693408 ) ) ;
+#1709 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1710 = CARTESIAN_POINT ( 'NONE', ( -40.72182540694797126, 14.10497510537457089, 7.000000000000002665 ) ) ;
+#1711 = EDGE_CURVE ( 'NONE', #2690, #2325, #1365, .T. ) ;
+#1712 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1713 = ORIENTED_EDGE ( 'NONE', *, *, #2216, .F. ) ;
+#1714 = EDGE_CURVE ( 'NONE', #758, #1516, #664, .T. ) ;
+#1715 = FACE_BOUND ( 'NONE', #2614, .T. ) ;
+#1716 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -11.24462381489573914 ) ) ;
+#1717 = CARTESIAN_POINT ( 'NONE', ( -34.40849682337891124, 34.70762474206001258, -17.05617626833258171 ) ) ;
+#1718 = ORIENTED_EDGE ( 'NONE', *, *, #952, .F. ) ;
+#1719 = CARTESIAN_POINT ( 'NONE', ( -40.90041223295983741, 13.49195890306038059, -8.153421727786044571 ) ) ;
+#1720 = CARTESIAN_POINT ( 'NONE', ( -43.05645483273637097, 12.09728489066157842, 11.76217390973335242 ) ) ;
+#1721 = AXIS2_PLACEMENT_3D ( 'NONE', #1590, #2017, #926 ) ;
+#1722 = AXIS2_PLACEMENT_3D ( 'NONE', #1841, #526, #1827 ) ;
+#1723 = ORIENTED_EDGE ( 'NONE', *, *, #376, .F. ) ;
+#1724 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#1725 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, 4.500000000000003553 ) ) ;
+#1726 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1727 = CARTESIAN_POINT ( 'NONE', ( -44.94138669699428590, 6.622494156546728128, -12.76790151328784972 ) ) ;
+#1728 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, -135.3085362679971126 ) ) ;
+#1729 = VERTEX_POINT ( 'NONE', #957 ) ;
+#1730 = EDGE_CURVE ( 'NONE', #1098, #1438, #1971, .T. ) ;
+#1731 = FILL_AREA_STYLE ('',( #880 ) ) ;
+#1732 = CARTESIAN_POINT ( 'NONE', ( -34.00738616944651227, 33.97034441269364180, -8.249432599336657290 ) ) ;
+#1733 = CARTESIAN_POINT ( 'NONE', ( -32.53716171719081984, 33.42888418853354437, -14.87330331384644566 ) ) ;
+#1734 = CARTESIAN_POINT ( 'NONE', ( 42.26572163226823164, 8.805405019717014170, 15.25141648470180122 ) ) ;
+#1735 = EDGE_CURVE ( 'NONE', #2620, #988, #946, .T. ) ;
+#1736 = ORIENTED_EDGE ( 'NONE', *, *, #1016, .T. ) ;
+#1737 = CARTESIAN_POINT ( 'NONE', ( -43.58712382716768730, 10.55595506930876759, -8.514304274908694126 ) ) ;
+#1738 = CARTESIAN_POINT ( 'NONE', ( -37.65733024777571814, 27.77905981872053687, 18.11655635681465526 ) ) ;
+#1739 = CARTESIAN_POINT ( 'NONE', ( 43.34545552388534162, 11.25788144268420155, 11.08747574047231943 ) ) ;
+#1740 = CARTESIAN_POINT ( 'NONE', ( -36.01076853932009669, 30.27612420079753619, -7.152262647838539600 ) ) ;
+#1741 = CIRCLE ( 'NONE', #748, 1.000000000000000888 ) ;
+#1742 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, 4.500000000000000888 ) ) ;
+#1743 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371286370, 44.36900610829383140, 12.50000000000000000 ) ) ;
+#1744 = VECTOR ( 'NONE', #1242, 1000.000000000000000 ) ;
+#1745 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000000000, -12.50000000000000000 ) ) ;
+#1746 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, 20.50000000000000000 ) ) ;
+#1747 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371482125, 44.36900610828815417, 12.07457116983854739 ) ) ;
+#1748 = CARTESIAN_POINT ( 'NONE', ( 4.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#1749 = CARTESIAN_POINT ( 'NONE', ( 42.57530924775149117, 13.49477380810269445, 15.37803231393592363 ) ) ;
+#1750 = AXIS2_PLACEMENT_3D ( 'NONE', #31, #420, #1261 ) ;
+#1751 = CARTESIAN_POINT ( 'NONE', ( -35.23995038522426171, 32.88448682190679051, -17.51455095785150462 ) ) ;
+#1752 = CARTESIAN_POINT ( 'NONE', ( -41.64362746466510856, 10.94080219668963316, 16.13454712838690952 ) ) ;
+#1753 = EDGE_CURVE ( 'NONE', #630, #387, #1229, .T. ) ;
+#1754 = CARTESIAN_POINT ( 'NONE', ( -34.79356443061850257, 34.45866161749017209, -7.699244109364620670 ) ) ;
+#1755 = LINE ( 'NONE', #16, #2496 ) ;
+#1756 = CYLINDRICAL_SURFACE ( 'NONE', #1562, 2.750000000000002665 ) ;
+#1757 = LINE ( 'NONE', #876, #97 ) ;
+#1758 = ORIENTED_EDGE ( 'NONE', *, *, #519, .F. ) ;
+#1759 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -18.46760616633223506 ) ) ;
+#1760 = CARTESIAN_POINT ( 'NONE', ( -34.11999303975387221, 36.76639026393849008, 8.101832367194113260 ) ) ;
+#1761 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1762 = CARTESIAN_POINT ( 'NONE', ( 43.61372823025177325, 10.47868249396899287, 16.46493364688598859 ) ) ;
+#1763 = FACE_OUTER_BOUND ( 'NONE', #784, .T. ) ;
+#1764 = CARTESIAN_POINT ( 'NONE', ( -41.32601150491565534, 17.12336316791652635, 18.34241424289258404 ) ) ;
+#1765 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #2464, #1206, #1798, #1609 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 0.000000000000000000, 1.570796326794896780 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1766 = ORIENTED_EDGE ( 'NONE', *, *, #1714, .T. ) ;
+#1767 = EDGE_CURVE ( 'NONE', #2664, #1041, #1354, .T. ) ;
+#1768 = CARTESIAN_POINT ( 'NONE', ( -30.09372127563741373, 32.72733336854117425, -11.97801004061737551 ) ) ;
+#1769 = CARTESIAN_POINT ( 'NONE', ( -32.51811363536528177, 35.24038309588284790, -10.08858819733316636 ) ) ;
+#1770 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1771 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1772 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688396697, 32.64726791736432432, -7.450487090025976755 ) ) ;
+#1773 = ADVANCED_FACE ( 'NONE', ( #728 ), #1808, .F. ) ;
+#1774 = FACE_OUTER_BOUND ( 'NONE', #1095, .T. ) ;
+#1775 = PRODUCT ( 'jsk_fetch_l515headmount', 'jsk_fetch_l515headmount', '', ( #2653 ) ) ;
+#1776 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, 15.25000000000000355 ) ) ;
+#1777 = CARTESIAN_POINT ( 'NONE', ( -34.92363111268105058, 32.64726791736432432, -18.46760616633223506 ) ) ;
+#1778 = CARTESIAN_POINT ( 'NONE', ( 43.57608948206799226, 10.58800436056431593, 12.97832405503685571 ) ) ;
+#1779 = CARTESIAN_POINT ( 'NONE', ( -33.21551960202715748, 33.91540280681631003, -15.95742383256837016 ) ) ;
+#1780 = CARTESIAN_POINT ( 'NONE', ( -42.85920530509989845, 6.768216077038087697, 13.74952544841743141 ) ) ;
+#1781 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 7.000000000000004441 ) ) ;
+#1782 = CARTESIAN_POINT ( 'NONE', ( -34.53979528784477537, 34.63182575294872834, -7.855695000733974531 ) ) ;
+#1783 = LINE ( 'NONE', #1168, #2016 ) ;
+#1784 = CYLINDRICAL_SURFACE ( 'NONE', #1426, 4.000000000000000000 ) ;
+#1785 = CARTESIAN_POINT ( 'NONE', ( 32.85740444909578173, 41.72045992200722253, 15.48591936326437768 ) ) ;
+#1786 = AXIS2_PLACEMENT_3D ( 'NONE', #2684, #113, #92 ) ;
+#1787 = EDGE_CURVE ( 'NONE', #416, #1589, #2443, .T. ) ;
+#1788 = VERTEX_POINT ( 'NONE', #739 ) ;
+#1789 = CIRCLE ( 'NONE', #848, 2.750000000000002665 ) ;
+#1790 =( NAMED_UNIT ( * ) PLANE_ANGLE_UNIT ( ) SI_UNIT ( $, .RADIAN. ) );
+#1791 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#1792 = CYLINDRICAL_SURFACE ( 'NONE', #1104, 5.000000000000000000 ) ;
+#1793 = CARTESIAN_POINT ( 'NONE', ( -42.91187591077668628, 6.587419232237197164, 13.48473243242434272 ) ) ;
+#1794 = CARTESIAN_POINT ( 'NONE', ( -30.10498433482840142, 32.75494340607369992, -11.85069633030392566 ) ) ;
+#1795 = AXIS2_PLACEMENT_3D ( 'NONE', #2526, #356, #1214 ) ;
+#1796 = CARTESIAN_POINT ( 'NONE', ( -34.62947619760160478, 34.54316338245381246, -6.960556498956810501 ) ) ;
+#1797 = CARTESIAN_POINT ( 'NONE', ( 4.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#1798 = CARTESIAN_POINT ( 'NONE', ( -40.72182540694797126, 14.10497510537457089, 18.00000000000000000 ) ) ;
+#1799 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1800 = VERTEX_POINT ( 'NONE', #168 ) ;
+#1801 = EDGE_CURVE ( 'NONE', #1213, #620, #2163, .T. ) ;
+#1802 = SURFACE_STYLE_USAGE ( .BOTH. , #2039 ) ;
+#1803 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#1804 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, -9.749999999999998224 ) ) ;
+#1805 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -17.99999999999999645 ) ) ;
+#1806 = CARTESIAN_POINT ( 'NONE', ( -36.87935591080476172, 30.03868881233102428, -18.83120745670911234 ) ) ;
+#1807 = CARTESIAN_POINT ( 'NONE', ( -32.85153238304921075, 35.18209223698067234, -9.526695088618611251 ) ) ;
+#1808 = CYLINDRICAL_SURFACE ( 'NONE', #2326, 2.750000000000002665 ) ;
+#1809 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 10.00000000000000178, 4.500000000000000888 ) ) ;
+#1810 = CIRCLE ( 'NONE', #2523, 3.000000000000002665 ) ;
+#1811 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 25.16419609954003533, 7.000000000000004441 ) ) ;
+#1812 = ORIENTED_EDGE ( 'NONE', *, *, #1623, .F. ) ;
+#1813 = UNCERTAINTY_MEASURE_WITH_UNIT (LENGTH_MEASURE( 1.000000000000000082E-05 ), #1396, 'distance_accuracy_value', 'NONE');
+#1814 = CARTESIAN_POINT ( 'NONE', ( -43.16529390050359183, 11.78116146411781706, 13.64926868012829431 ) ) ;
+#1815 = CARTESIAN_POINT ( 'NONE', ( -32.01717275684787012, 32.35121551323180711, -12.94835367935805870 ) ) ;
+#1816 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1817 = ADVANCED_FACE ( 'NONE', ( #1891 ), #1269, .F. ) ;
+#1818 = CARTESIAN_POINT ( 'NONE', ( -30.07459780443158692, 32.68056142076170545, -12.37086482429206669 ) ) ;
+#1819 = SURFACE_STYLE_USAGE ( .BOTH. , #2034 ) ;
+#1820 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1821 = CARTESIAN_POINT ( 'NONE', ( -31.22901909779051266, 34.39715868866920800, -9.318116878902950262 ) ) ;
+#1822 = CARTESIAN_POINT ( 'NONE', ( 36.78005168385927703, 27.63548691358506915, 18.00000000000000000 ) ) ;
+#1823 = VERTEX_POINT ( 'NONE', #1915 ) ;
+#1824 = CIRCLE ( 'NONE', #2763, 1.000000000000000888 ) ;
+#1825 = CARTESIAN_POINT ( 'NONE', ( -44.92465409814774091, 6.671094044351557173, 11.96873245554875886 ) ) ;
+#1826 = ORIENTED_EDGE ( 'NONE', *, *, #1561, .F. ) ;
+#1827 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1828 = COLOUR_RGB ( '',0.7921568627450980005, 0.8196078431372548767, 0.9333333333333333481 ) ;
+#1829 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1830 = ADVANCED_FACE ( 'NONE', ( #402 ), #422, .F. ) ;
+#1831 = CARTESIAN_POINT ( 'NONE', ( -1.750000000000000888, 24.99999999999999645, 4.500000000000000888 ) ) ;
+#1832 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1833 = CARTESIAN_POINT ( 'NONE', ( -35.17060090378452486, 34.14936576743428986, -17.50565766128448431 ) ) ;
+#1834 = CIRCLE ( 'NONE', #1978, 1.750000000000000888 ) ;
+#1835 =( LENGTH_UNIT ( ) NAMED_UNIT ( * ) SI_UNIT ( .MILLI., .METRE. ) );
+#1836 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -11.01637359942223959 ) ) ;
+#1837 = CARTESIAN_POINT ( 'NONE', ( -44.78057694612991924, 7.089566601276006352, -13.96120021518454912 ) ) ;
+#1838 = VERTEX_POINT ( 'NONE', #1051 ) ;
+#1839 = CARTESIAN_POINT ( 'NONE', ( -42.98895923545501319, 6.322823416230565208, 12.14024265315041617 ) ) ;
+#1840 = ORIENTED_EDGE ( 'NONE', *, *, #74, .T. ) ;
+#1841 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 15.00000000000000000, -20.50000000000000355 ) ) ;
+#1842 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#1843 = ORIENTED_EDGE ( 'NONE', *, *, #823, .F. ) ;
+#1844 = CARTESIAN_POINT ( 'NONE', ( -43.55646050725465557, 10.64501678178904953, -13.79302111228574645 ) ) ;
+#1845 = FACE_BOUND ( 'NONE', #1537, .T. ) ;
+#1846 = CARTESIAN_POINT ( 'NONE', ( -4.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#1847 = ADVANCED_FACE ( 'NONE', ( #2570 ), #1064, .F. ) ;
+#1848 = CARTESIAN_POINT ( 'NONE', ( 42.99779078280186440, 6.292508293127117902, 12.32001292924790370 ) ) ;
+#1849 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759544634, -12.50000000000000000 ) ) ;
+#1850 = CARTESIAN_POINT ( 'NONE', ( -44.80934957045266742, 7.005996420292152749, -13.83031059393524309 ) ) ;
+#1851 = ORIENTED_EDGE ( 'NONE', *, *, #785, .T. ) ;
+#1852 = AXIS2_PLACEMENT_3D ( 'NONE', #425, #1943, #2319 ) ;
+#1853 = CARTESIAN_POINT ( 'NONE', ( -43.96825760039320130, 9.448950784602933695, 9.065657051501865737 ) ) ;
+#1854 = ORIENTED_EDGE ( 'NONE', *, *, #2643, .F. ) ;
+#1855 = ORIENTED_EDGE ( 'NONE', *, *, #314, .T. ) ;
+#1856 = CARTESIAN_POINT ( 'NONE', ( -33.03257378525533738, 31.99619020741757680, 4.500000000000000888 ) ) ;
+#1857 = FACE_OUTER_BOUND ( 'NONE', #137, .T. ) ;
+#1858 = CARTESIAN_POINT ( 'NONE', ( -34.84611884792062853, 33.49440101975844186, -7.681646398363541905 ) ) ;
+#1859 = ORIENTED_EDGE ( 'NONE', *, *, #1189, .T. ) ;
+#1860 = CARTESIAN_POINT ( 'NONE', ( -43.24436591354764658, 11.55149654490020339, -13.81595327377998039 ) ) ;
+#1861 = CARTESIAN_POINT ( 'NONE', ( -33.67957420515575961, 35.01001685161236310, -16.45949862367574212 ) ) ;
+#1862 = B_SPLINE_CURVE_WITH_KNOTS ( 'NONE', 3,
+ ( #2018, #975, #723, #98, #773, #120, #1631, #954, #1398, #2279, #2240, #2251 ),
+ .UNSPECIFIED., .F., .F.,
+ ( 4, 2, 2, 2, 2, 4 ),
+ ( 0.000000000000000000, 0.001161146787050921017, 0.001741720180576390633, 0.002322293574101860682, 0.004644587148203704885, 0.009289174296407392423 ),
+ .UNSPECIFIED. ) ;
+#1863 = CARTESIAN_POINT ( 'NONE', ( 44.27828862574259006, 8.548464662502123446, 9.623516448636827647 ) ) ;
+#1864 = CARTESIAN_POINT ( 'NONE', ( 32.20724408224923252, 43.60885282341502744, 10.94862032847915323 ) ) ;
+#1865 = CARTESIAN_POINT ( 'NONE', ( -42.94403121265324330, 6.477043111662483810, -13.29080388371014187 ) ) ;
+#1866 = ORIENTED_EDGE ( 'NONE', *, *, #390, .T. ) ;
+#1867 = EDGE_CURVE ( 'NONE', #887, #2555, #829, .T. ) ;
+#1868 = CARTESIAN_POINT ( 'NONE', ( -44.07774513821160411, 9.130943874690093054, 9.248325972625037750 ) ) ;
+#1869 = VERTEX_POINT ( 'NONE', #1689 ) ;
+#1870 = VERTEX_POINT ( 'NONE', #198 ) ;
+#1871 = CYLINDRICAL_SURFACE ( 'NONE', #2022, 3.000000000000002665 ) ;
+#1872 = AXIS2_PLACEMENT_3D ( 'NONE', #1451, #2580, #2377 ) ;
+#1873 = CARTESIAN_POINT ( 'NONE', ( -30.09345630165302765, 32.72668401948667594, -11.98152477718937092 ) ) ;
+#1874 = AXIS2_PLACEMENT_3D ( 'NONE', #499, #1820, #141 ) ;
+#1875 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, 12.50000000000000000 ) ) ;
+#1876 = ORIENTED_EDGE ( 'NONE', *, *, #314, .F. ) ;
+#1877 = CARTESIAN_POINT ( 'NONE', ( -42.77379477138983788, 7.061395827092919575, -10.93477307200270232 ) ) ;
+#1878 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732930828, 12.50000000000000000 ) ) ;
+#1879 = CARTESIAN_POINT ( 'NONE', ( -33.34895536105618419, 35.09410072600955743, -16.11356605787576513 ) ) ;
+#1880 =( NAMED_UNIT ( * ) SI_UNIT ( $, .STERADIAN. ) SOLID_ANGLE_UNIT ( ) );
+#1881 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725331898682, 12.14002175909385883 ) ) ;
+#1882 = CARTESIAN_POINT ( 'NONE', ( -40.06395933689310596, 20.78899779720995156, -17.53901762858859925 ) ) ;
+#1883 = EDGE_LOOP ( 'NONE', ( #461, #105, #1379, #833, #2345, #769 ) ) ;
+#1884 = CIRCLE ( 'NONE', #1750, 5.000000000000000000 ) ;
+#1885 = CIRCLE ( 'NONE', #1795, 5.000000000000000888 ) ;
+#1886 = CARTESIAN_POINT ( 'NONE', ( -34.92363111268105769, 32.64726791736432432, -6.532393833667062388 ) ) ;
+#1887 = EDGE_LOOP ( 'NONE', ( #2372, #1497 ) ) ;
+#1888 = CARTESIAN_POINT ( 'NONE', ( -4.000000000000001776, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#1889 = EDGE_CURVE ( 'NONE', #1614, #1947, #964, .T. ) ;
+#1890 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, -1.224646799147353207E-16 ) ) ;
+#1891 = FACE_OUTER_BOUND ( 'NONE', #1704, .T. ) ;
+#1892 = EDGE_CURVE ( 'NONE', #776, #2749, #1274, .T. ) ;
+#1893 = EDGE_LOOP ( 'NONE', ( #35, #369 ) ) ;
+#1894 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#1895 = CARTESIAN_POINT ( 'NONE', ( -42.98233690606029000, 6.345555189378104366, 12.94953407607416374 ) ) ;
+#1896 = CARTESIAN_POINT ( 'NONE', ( 4.000000000000001776, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1897 = CARTESIAN_POINT ( 'NONE', ( 41.13454712838648675, 12.68826868899496318, 8.356372535334470442 ) ) ;
+#1898 = CARTESIAN_POINT ( 'NONE', ( -43.55993684666788823, 10.63491974264957030, -13.07039899048681342 ) ) ;
+#1899 = ORIENTED_EDGE ( 'NONE', *, *, #1141, .F. ) ;
+#1900 = DIRECTION ( 'NONE', ( 0.9455286637128581528, 0.3255388549733763681, -0.000000000000000000 ) ) ;
+#1901 = ORIENTED_EDGE ( 'NONE', *, *, #2671, .F. ) ;
+#1902 = PLANE ( 'NONE', #678 ) ;
+#1903 = CARTESIAN_POINT ( 'NONE', ( -30.14861977122593473, 32.86258940435795495, -13.52432456679505535 ) ) ;
+#1904 = CARTESIAN_POINT ( 'NONE', ( -31.17158371284658358, 32.72879920981659296, -11.01637359942223959 ) ) ;
+#1905 = ORIENTED_EDGE ( 'NONE', *, *, #1201, .T. ) ;
+#1906 = CARTESIAN_POINT ( 'NONE', ( -37.96348091318344586, 26.88984421472649444, -6.923752528081378088 ) ) ;
+#1907 = CARTESIAN_POINT ( 'NONE', ( -44.92465409814780486, 6.671094044351380425, 13.03126754445123403 ) ) ;
+#1908 = CARTESIAN_POINT ( 'NONE', ( 44.93248048743234335, 6.648362271204079299, 12.92516777354952673 ) ) ;
+#1909 = CARTESIAN_POINT ( 'NONE', ( 42.57530924774187753, 13.49477380811571336, 9.621967686071759118 ) ) ;
+#1910 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371285660, 6.610463800732987671, 12.50000000000000000 ) ) ;
+#1911 = EDGE_LOOP ( 'NONE', ( #1439, #1766, #206, #1659, #217 ) ) ;
+#1912 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1913 = ORIENTED_EDGE ( 'NONE', *, *, #1070, .F. ) ;
+#1914 = CYLINDRICAL_SURFACE ( 'NONE', #2588, 2.750000000000002665 ) ;
+#1915 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -0.4999999999999995559 ) ) ;
+#1916 = ORIENTED_EDGE ( 'NONE', *, *, #736, .T. ) ;
+#1917 = CARTESIAN_POINT ( 'NONE', ( -44.29376301731586096, 8.503519241817201646, -9.650451582590376276 ) ) ;
+#1918 = VECTOR ( 'NONE', #69, 1000.000000000000114 ) ;
+#1919 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1920 = CIRCLE ( 'NONE', #1966, 4.499999999999997335 ) ;
+#1921 = EDGE_CURVE ( 'NONE', #1800, #1788, #1627, .T. ) ;
+#1922 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #2748, #834, #1265, #1310 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 3.141592653589793116, 4.712388980384689674 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.8047378541243649375, 0.8047378541243649375, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#1923 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1924 = CARTESIAN_POINT ( 'NONE', ( 38.21994831615298693, 22.69290528549761987, 7.000000000000002665 ) ) ;
+#1925 = FACE_BOUND ( 'NONE', #576, .T. ) ;
+#1926 = ORIENTED_EDGE ( 'NONE', *, *, #2226, .T. ) ;
+#1927 = EDGE_CURVE ( 'NONE', #2740, #620, #248, .T. ) ;
+#1928 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1929 = ORIENTED_EDGE ( 'NONE', *, *, #1767, .F. ) ;
+#1930 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, 17.99999999999999645 ) ) ;
+#1931 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#1932 = CARTESIAN_POINT ( 'NONE', ( -30.28348284022550274, 33.20279433083954501, -14.10418142956314469 ) ) ;
+#1933 = VERTEX_POINT ( 'NONE', #2117 ) ;
+#1934 = CARTESIAN_POINT ( 'NONE', ( -42.70553305318818360, 13.11653834140497921, -9.802824372074068648 ) ) ;
+#1935 = CARTESIAN_POINT ( 'NONE', ( -43.01186382226871530, 12.22679962567663914, 12.11340478804969578 ) ) ;
+#1936 = ORIENTED_EDGE ( 'NONE', *, *, #1016, .F. ) ;
+#1937 = CARTESIAN_POINT ( 'NONE', ( 44.92465409814774091, 6.671094044351608687, 13.03126754445124114 ) ) ;
+#1938 = EDGE_CURVE ( 'NONE', #387, #1264, #2568, .T. ) ;
+#1939 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1940 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#1941 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1942 = EDGE_CURVE ( 'NONE', #550, #1642, #515, .T. ) ;
+#1943 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1944 = EDGE_LOOP ( 'NONE', ( #2529, #1186, #1484, #1876 ) ) ;
+#1945 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1946 = CARTESIAN_POINT ( 'NONE', ( -34.03521183783485782, 34.88566710271816618, -16.77526415812417326 ) ) ;
+#1947 = VERTEX_POINT ( 'NONE', #2738 ) ;
+#1948 = CARTESIAN_POINT ( 'NONE', ( -44.03943411685308007, 9.242218360906406360, -9.178856669887151654 ) ) ;
+#1949 = CARTESIAN_POINT ( 'NONE', ( -40.14688991242650218, 20.54812567066732854, 18.83082239818493520 ) ) ;
+#1950 = CIRCLE ( 'NONE', #1721, 8.000000000000000000 ) ;
+#1951 = ORIENTED_EDGE ( 'NONE', *, *, #581, .T. ) ;
+#1952 = CARTESIAN_POINT ( 'NONE', ( 31.00000000000000355, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#1953 = DIRECTION ( 'NONE', ( -0.9422632794457277416, 0.3348729792147797646, -0.000000000000000000 ) ) ;
+#1954 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, -12.50000000000000000 ) ) ;
+#1955 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, -20.50000000000000000 ) ) ;
+#1956 = VERTEX_POINT ( 'NONE', #824 ) ;
+#1957 = EDGE_CURVE ( 'NONE', #1000, #1369, #2528, .T. ) ;
+#1958 = CARTESIAN_POINT ( 'NONE', ( -31.57655150778580122, 34.62265474847123414, -16.06343561369301653 ) ) ;
+#1959 = CARTESIAN_POINT ( 'NONE', ( -32.17590843201791984, 43.43964489217512437, 11.07394587425399379 ) ) ;
+#1960 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, -0.4999999999999995559 ) ) ;
+#1961 = CARTESIAN_POINT ( 'NONE', ( 34.45097498342184394, 37.09192911890503552, 17.69783447513438546 ) ) ;
+#1962 = VERTEX_POINT ( 'NONE', #1257 ) ;
+#1963 = CARTESIAN_POINT ( 'NONE', ( -41.56879248528925785, 16.41820502030821061, -6.774416357035755176 ) ) ;
+#1964 = CARTESIAN_POINT ( 'NONE', ( -42.71092435547762278, 7.277204487012604694, 14.26880262041526670 ) ) ;
+#1965 = ORIENTED_EDGE ( 'NONE', *, *, #564, .F. ) ;
+#1966 = AXIS2_PLACEMENT_3D ( 'NONE', #1566, #24, #895 ) ;
+#1967 = CARTESIAN_POINT ( 'NONE', ( -32.80202257256530629, 33.68525208390452974, -9.630770214105424998 ) ) ;
+#1968 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#1969 = AXIS2_PLACEMENT_3D ( 'NONE', #1954, #1352, #2416 ) ;
+#1970 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, -15.25000000000000355 ) ) ;
+#1971 = CIRCLE ( 'NONE', #896, 4.499999999999997335 ) ;
+#1972 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#1973 = CARTESIAN_POINT ( 'NONE', ( -33.90682669663748783, 34.88402129174354371, -17.71860934597597748 ) ) ;
+#1974 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725331308221, 12.14002175909410930 ) ) ;
+#1975 = EDGE_CURVE ( 'NONE', #2058, #1823, #1810, .T. ) ;
+#1976 = FACE_OUTER_BOUND ( 'NONE', #2177, .T. ) ;
+#1977 = CARTESIAN_POINT ( 'NONE', ( 43.65830555055661222, 10.34920752206666883, 12.45125492979268245 ) ) ;
+#1978 = AXIS2_PLACEMENT_3D ( 'NONE', #62, #1996, #507 ) ;
+#1979 = CARTESIAN_POINT ( 'NONE', ( -34.00642026789550698, 33.97049140113600885, -16.74974570788206663 ) ) ;
+#1980 = CARTESIAN_POINT ( 'NONE', ( -44.07774513821154017, 9.130943874690295559, 15.75167402737479705 ) ) ;
+#1981 = EDGE_CURVE ( 'NONE', #175, #2211, #10, .T. ) ;
+#1982 = CARTESIAN_POINT ( 'NONE', ( -32.06470902937228118, 32.48963762489935192, -13.36790182820358730 ) ) ;
+#1983 = CARTESIAN_POINT ( 'NONE', ( -31.20068707187684964, 34.38402335589851333, -9.344251165861859221 ) ) ;
+#1984 = DIRECTION ( 'NONE', ( -0.9422632794457277416, 0.3348729792147797646, 0.000000000000000000 ) ) ;
+#1985 = FACE_BOUND ( 'NONE', #1355, .T. ) ;
+#1986 = CARTESIAN_POINT ( 'NONE', ( 31.98742823517704892, 44.24730866661698769, 13.35083749214626891 ) ) ;
+#1987 = ORIENTED_EDGE ( 'NONE', *, *, #2115, .T. ) ;
+#1988 = CARTESIAN_POINT ( 'NONE', ( -35.45182304767064352, 33.77936032244413411, -18.34026773097174257 ) ) ;
+#1989 = LINE ( 'NONE', #2406, #1505 ) ;
+#1990 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#1991 = CARTESIAN_POINT ( 'NONE', ( 41.62144033389574815, 16.26528917268565166, 16.48460089004631612 ) ) ;
+#1992 = CARTESIAN_POINT ( 'NONE', ( -34.84571189710230499, 33.49490940725474530, -17.31814595735079720 ) ) ;
+#1993 = CARTESIAN_POINT ( 'NONE', ( -43.54841234349528634, 10.66839269962361314, 11.16680329600029253 ) ) ;
+#1994 = EDGE_CURVE ( 'NONE', #30, #1589, #1950, .T. ) ;
+#1995 = AXIS2_PLACEMENT_3D ( 'NONE', #1252, #604, #1061 ) ;
+#1996 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#1997 = CARTESIAN_POINT ( 'NONE', ( -33.62933954372219603, 34.94128602345796963, -7.429681065369031501 ) ) ;
+#1998 = FACE_OUTER_BOUND ( 'NONE', #1436, .T. ) ;
+#1999 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 15.00000000000000000, 79.70475956827036157 ) ) ;
+#2000 = CARTESIAN_POINT ( 'NONE', ( 32.65564714380937517, 42.30646467182854309, 15.14364952153606758 ) ) ;
+#2001 = ORIENTED_EDGE ( 'NONE', *, *, #963, .T. ) ;
+#2002 = CARTESIAN_POINT ( 'NONE', ( -30.45604614282525446, 33.55384789772586629, -14.51865482684744357 ) ) ;
+#2003 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -7.000000000000004441 ) ) ;
+#2004 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -13.75537618510426086 ) ) ;
+#2005 = CARTESIAN_POINT ( 'NONE', ( -42.51245935571762402, 7.958454404286162465, 14.77104525622273634 ) ) ;
+#2006 = CARTESIAN_POINT ( 'NONE', ( -31.71044961685591446, 36.78254263397548840, -11.54477014384589850 ) ) ;
+#2007 = CARTESIAN_POINT ( 'NONE', ( -34.33891446344943432, 33.86159807794278720, -7.997740211241990416 ) ) ;
+#2008 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 5.000000000000000888, 4.500000000000003553 ) ) ;
+#2009 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2010 = EDGE_CURVE ( 'NONE', #440, #630, #2699, .T. ) ;
+#2011 = CARTESIAN_POINT ( 'NONE', ( 32.03545348354654720, 43.92176981164326577, 13.21993941643139436 ) ) ;
+#2012 = AXIS2_PLACEMENT_3D ( 'NONE', #1999, #2578, #1132 ) ;
+#2013 = ORIENTED_EDGE ( 'NONE', *, *, #2183, .F. ) ;
+#2014 = EDGE_CURVE ( 'NONE', #2155, #116, #83, .T. ) ;
+#2015 = VECTOR ( 'NONE', #151, 1000.000000000000000 ) ;
+#2016 = VECTOR ( 'NONE', #1662, 1000.000000000000000 ) ;
+#2017 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#2018 = CARTESIAN_POINT ( 'NONE', ( -33.45489901264950561, 39.98503501767059021, 4.500000000000003553 ) ) ;
+#2019 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2020 = CIRCLE ( 'NONE', #809, 2.750000000000002665 ) ;
+#2021 = CARTESIAN_POINT ( 'NONE', ( -32.12337896459407460, 32.66333205226038672, -11.33764277245682450 ) ) ;
+#2022 = AXIS2_PLACEMENT_3D ( 'NONE', #1856, #150, #1004 ) ;
+#2023 = CARTESIAN_POINT ( 'NONE', ( -32.40283745485356803, 33.25449857238987050, -10.43096154852584334 ) ) ;
+#2024 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2025 = CARTESIAN_POINT ( 'NONE', ( -35.70923006206639627, 33.36694497305119000, -18.42096702102175954 ) ) ;
+#2026 = EDGE_CURVE ( 'NONE', #1315, #2211, #939, .T. ) ;
+#2027 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, -7.000000000000004441 ) ) ;
+#2028 = ORIENTED_EDGE ( 'NONE', *, *, #284, .T. ) ;
+#2029 = CARTESIAN_POINT ( 'NONE', ( -43.20911154149832356, 11.65389298324840794, 13.74738253265919496 ) ) ;
+#2030 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, -12.50000000000000000 ) ) ;
+#2031 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #813, #1400, #332 ),
+ ( #1460, #2088, #596 ),
+ ( #2682, #170, #1191 ),
+ ( #1619, #122, #1444 ),
+ ( #761, #2504, #2267 ),
+ ( #345, #1860, #1204 ),
+ ( #2074, #1431, #583 ),
+ ( #567, #2297, #1231 ),
+ ( #2312, #154, #2104 ),
+ ( #139, #1844, #1024 ),
+ ( #993, #1244, #1877 ),
+ ( #185, #2696, #1503 ),
+ ( #1301, #201, #1691 ),
+ ( #255, #448, #42 ),
+ ( #1917, #2545, #1068 ),
+ ( #1948, #2573, #2 ),
+ ( #2161, #239, #1678 ),
+ ( #1737, #624, #610 ),
+ ( #1473, #1096, #884 ),
+ ( #2144, #1934, #1054 ),
+ ( #1113, #2174, #1719 ),
+ ( #1963, #28, #855 ),
+ ( #2366, #1705, #2530 ),
+ ( #1272, #2589, #1489 ),
+ ( #840, #13, #2327 ),
+ ( #1286, #669, #1535 ),
+ ( #2384, #1906, #2340 ),
+ ( #870, #417, #462 ),
+ ( #1316, #1517, #1259 ),
+ ( #404, #2755, #226 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 0.000000000000000000, 0.06182343355040228333, 0.1236468671008045667, 0.1854703006512068431, 0.2472937342016091333, 0.3091171677520114236, 0.3709406013024136861, 0.4945874684032182667, 0.6182343355040228472, 0.7418812026048273722, 0.9891749368064365333, 1.236468671008045694, 1.483762405209654744, 1.731056139411264017, 1.978349873612873067 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.1650323245415012685, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1650295672437424033, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1659522367849533553, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1695406270279371519, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1722066456629676168, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1790482828115376179, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1832210536907214193, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1927776193290985096, 1.000000000000000000),
+ ( 1.000000000000000000, 0.1981596860165199536, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2098496721005897458, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2161570347703708483, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2294594316546308965, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2364545543617102563, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2581920883496749419, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2736703402487685732, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3056035870501948581, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3220670159437838964, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3554073528904945767, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3722889375824524616, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4230301067165325168, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4569611680061238523, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5233918139849701445, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5559131927636226100, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6185992827450953468, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6487707199100192224, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7060804060503472757, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7332210067120173980, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7839358397906480702, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8075124374852987730, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8290880361187438208, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#2032 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2033 = CARTESIAN_POINT ( 'NONE', ( -31.80139523032111271, 36.96912281376587828, -11.61668358927950706 ) ) ;
+#2034 = SURFACE_SIDE_STYLE ('',( #323 ) ) ;
+#2035 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2036 = VECTOR ( 'NONE', #2112, 1000.000000000000000 ) ;
+#2037 = CARTESIAN_POINT ( 'NONE', ( 42.65021550190652277, 7.485593379377545986, 14.43847249979323522 ) ) ;
+#2038 = ORIENTED_EDGE ( 'NONE', *, *, #1867, .F. ) ;
+#2039 = SURFACE_SIDE_STYLE ('',( #2260 ) ) ;
+#2040 = CARTESIAN_POINT ( 'NONE', ( -42.95367559951914416, 6.443937839889548158, 11.78200188557550376 ) ) ;
+#2041 = ORIENTED_EDGE ( 'NONE', *, *, #2348, .F. ) ;
+#2042 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2043 = EDGE_CURVE ( 'NONE', #2761, #2706, #1989, .T. ) ;
+#2044 = CARTESIAN_POINT ( 'NONE', ( -32.80462327031899150, 41.87376302754497459, 15.75747938236831125 ) ) ;
+#2045 = CARTESIAN_POINT ( 'NONE', ( -34.27817459305202874, 36.22341709370545715, 7.000000000000002665 ) ) ;
+#2046 = CARTESIAN_POINT ( 'NONE', ( -35.09071288550256895, 34.18825145781231356, -6.783385553241092936 ) ) ;
+#2047 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2048 = CARTESIAN_POINT ( 'NONE', ( 42.98895923545502029, 6.322823416230407112, 12.14024265315049966 ) ) ;
+#2049 = CARTESIAN_POINT ( 'NONE', ( 41.64362746466424881, 10.94080219669020870, 16.13454712838777283 ) ) ;
+#2050 = EDGE_LOOP ( 'NONE', ( #892, #194, #1047, #1303 ) ) ;
+#2051 = CIRCLE ( 'NONE', #2718, 4.000000000000001776 ) ;
+#2052 = CARTESIAN_POINT ( 'NONE', ( -44.60389381109560958, 7.602743341985648051, 10.40959690314558017 ) ) ;
+#2053 = EDGE_LOOP ( 'NONE', ( #426, #1091, #1916, #162, #1418 ) ) ;
+#2054 = LINE ( 'NONE', #1591, #965 ) ;
+#2055 = AXIS2_PLACEMENT_3D ( 'NONE', #554, #1832, #2268 ) ;
+#2056 = CARTESIAN_POINT ( 'NONE', ( -32.72692620558953536, 41.54822417257172162, 15.25632870815823772 ) ) ;
+#2057 = EDGE_CURVE ( 'NONE', #1630, #2512, #1323, .T. ) ;
+#2058 = VERTEX_POINT ( 'NONE', #1542 ) ;
+#2059 = ADVANCED_FACE ( 'NONE', ( #1136 ), #329, .T. ) ;
+#2060 = CARTESIAN_POINT ( 'NONE', ( -35.84274608350956726, 33.07029903827023531, -7.219395649130901482 ) ) ;
+#2061 = CARTESIAN_POINT ( 'NONE', ( -28.36585991138575125, 54.76616564920128383, 4.500000000000003553 ) ) ;
+#2062 = CIRCLE ( 'NONE', #2426, 2.750000000000002665 ) ;
+#2063 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000000000, 17.99999999999999645 ) ) ;
+#2064 = CARTESIAN_POINT ( 'NONE', ( -31.69050009878254670, 34.68285970349573688, -8.823244160955214355 ) ) ;
+#2065 = CARTESIAN_POINT ( 'NONE', ( -44.71499975903609680, 7.280035754606219989, -14.22095176989863496 ) ) ;
+#2066 = EDGE_LOOP ( 'NONE', ( #559, #1758, #2347, #1936 ) ) ;
+#2067 = CARTESIAN_POINT ( 'NONE', ( -41.62144033389959930, 16.26528917268317542, 8.515399109952078049 ) ) ;
+#2068 = VERTEX_POINT ( 'NONE', #1090 ) ;
+#2069 = ORIENTED_EDGE ( 'NONE', *, *, #2026, .F. ) ;
+#2070 = FACE_OUTER_BOUND ( 'NONE', #2222, .T. ) ;
+#2071 = CARTESIAN_POINT ( 'NONE', ( -32.17590843201798378, 43.43964489217511726, 13.92605412574633839 ) ) ;
+#2072 = CARTESIAN_POINT ( 'NONE', ( -34.58342906573181352, 33.72076100159020484, -7.835629092645943317 ) ) ;
+#2073 = ORIENTED_EDGE ( 'NONE', *, *, #1519, .F. ) ;
+#2074 = CARTESIAN_POINT ( 'NONE', ( -44.85875296978372972, 6.862504086155298388, -11.43299634048594626 ) ) ;
+#2075 = CARTESIAN_POINT ( 'NONE', ( -30.43907819221767497, 33.50842507884905075, -14.46730229209869911 ) ) ;
+#2076 = CARTESIAN_POINT ( 'NONE', ( 44.92465409814780486, 6.671094044351431940, 11.96873245554876775 ) ) ;
+#2077 = CARTESIAN_POINT ( 'NONE', ( 42.74090254271485634, 13.01380754396811845, 7.602985723577521782 ) ) ;
+#2078 = CARTESIAN_POINT ( 'NONE', ( -44.03943411685344955, 9.242218360905292585, -15.82114333011278973 ) ) ;
+#2079 = ORIENTED_EDGE ( 'NONE', *, *, #1735, .T. ) ;
+#2080 = CIRCLE ( 'NONE', #110, 2.750000000000002665 ) ;
+#2081 = AXIS2_PLACEMENT_3D ( 'NONE', #341, #745, #551 ) ;
+#2082 = CARTESIAN_POINT ( 'NONE', ( -42.41046231078216522, 13.97357227301042570, 7.331800433583932630 ) ) ;
+#2083 = EDGE_LOOP ( 'NONE', ( #2716, #2721, #1008, #641 ) ) ;
+#2084 = ORIENTED_EDGE ( 'NONE', *, *, #721, .F. ) ;
+#2085 = CARTESIAN_POINT ( 'NONE', ( 28.36585991138574769, 54.76616564920129804, 4.500000000000003553 ) ) ;
+#2086 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371285660, 44.36900610829382430, 25.49999999999999645 ) ) ;
+#2087 = EDGE_LOOP ( 'NONE', ( #991, #412, #2307, #1854 ) ) ;
+#2088 = CARTESIAN_POINT ( 'NONE', ( -43.00000000007026557, 12.26125813586037872, -12.74474028311317220 ) ) ;
+#2089 = CARTESIAN_POINT ( 'NONE', ( -33.51955988454129454, 33.98472066379684975, -16.29747460035643414 ) ) ;
+#2090 = CARTESIAN_POINT ( 'NONE', ( 44.36934426592454628, 8.283993259259633035, 9.816037424464214567 ) ) ;
+#2091 = EDGE_CURVE ( 'NONE', #1271, #1869, #2080, .T. ) ;
+#2092 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.21079393477482000, 12.49999999999999822 ) ) ;
+#2093 = CARTESIAN_POINT ( 'NONE', ( -42.11335358391571049, 9.328422783357867232, -15.50305764469500325 ) ) ;
+#2094 = ORIENTED_EDGE ( 'NONE', *, *, #263, .F. ) ;
+#2095 = ADVANCED_FACE ( 'NONE', ( #1496 ), #2125, .F. ) ;
+#2096 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, -7.000000000000004441 ) ) ;
+#2097 = ORIENTED_EDGE ( 'NONE', *, *, #2643, .T. ) ;
+#2098 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#2099 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2100 = CIRCLE ( 'NONE', #1786, 5.499999999999996447 ) ;
+#2101 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 25.16419609954003533, 7.000000000000004441 ) ) ;
+#2102 = VERTEX_POINT ( 'NONE', #2361 ) ;
+#2103 = AXIS2_PLACEMENT_3D ( 'NONE', #1492, #1923, #4 ) ;
+#2104 = CARTESIAN_POINT ( 'NONE', ( -42.86042546973749268, 6.764027746302351929, -11.26359981792067089 ) ) ;
+#2105 = CARTESIAN_POINT ( 'NONE', ( -43.08855886698587057, 12.00403861870638167, 11.60551830606185142 ) ) ;
+#2106 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 12.26125813606941328, 12.49999999999993783 ) ) ;
+#2107 = CARTESIAN_POINT ( 'NONE', ( 39.93733163486432147, 16.79782431294331957, 7.556418717553624376 ) ) ;
+#2108 = CARTESIAN_POINT ( 'NONE', ( -43.46764395096883504, 10.90298475857212246, -11.09020261033075805 ) ) ;
+#2109 = ORIENTED_EDGE ( 'NONE', *, *, #2014, .T. ) ;
+#2110 = AXIS2_PLACEMENT_3D ( 'NONE', #229, #1724, #2373 ) ;
+#2111 = PRODUCT_DEFINITION ( 'UNKNOWN', '', #2235, #743 ) ;
+#2112 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#2113 = EDGE_LOOP ( 'NONE', ( #1533, #523, #2073, #2482 ) ) ;
+#2114 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#2115 = EDGE_CURVE ( 'NONE', #1788, #1800, #2440, .T. ) ;
+#2116 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, 12.50000000000000000 ) ) ;
+#2117 = CARTESIAN_POINT ( 'NONE', ( 1.750000000000000888, 65.00000000000000000, 4.500000000000000888 ) ) ;
+#2118 = ORIENTED_EDGE ( 'NONE', *, *, #80, .F. ) ;
+#2119 = FACE_OUTER_BOUND ( 'NONE', #2567, .T. ) ;
+#2120 = LINE ( 'NONE', #449, #2232 ) ;
+#2121 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 12.26125813606941328, 12.50000000000002132 ) ) ;
+#2122 = CARTESIAN_POINT ( 'NONE', ( 33.59905643722282775, 39.56632930884228472, 8.586160965562099179 ) ) ;
+#2123 = CARTESIAN_POINT ( 'NONE', ( -38.42973826896736966, 21.97278139372983929, -17.93977322889649528 ) ) ;
+#2124 = EDGE_LOOP ( 'NONE', ( #679, #1053, #2041, #165 ) ) ;
+#2125 = CYLINDRICAL_SURFACE ( 'NONE', #1995, 4.000000000000001776 ) ;
+#2126 = ORIENTED_EDGE ( 'NONE', *, *, #598, .F. ) ;
+#2127 = FACE_OUTER_BOUND ( 'NONE', #1944, .T. ) ;
+#2128 = CARTESIAN_POINT ( 'NONE', ( -33.54513916431535847, 33.99549709481389215, -8.669659710838189426 ) ) ;
+#2129 = EDGE_CURVE ( 'NONE', #1614, #410, #2346, .T. ) ;
+#2130 = ORIENTED_EDGE ( 'NONE', *, *, #1680, .T. ) ;
+#2131 = PLANE ( 'NONE', #2333 ) ;
+#2132 = CARTESIAN_POINT ( 'NONE', ( -43.29946974291465267, 11.39144729317233384, 11.12182360864387398 ) ) ;
+#2133 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 6.284924945759610360, 12.49999999999999112 ) ) ;
+#2134 = CARTESIAN_POINT ( 'NONE', ( 39.29637667370440823, 23.01844414046957255, 6.000000000000002665 ) ) ;
+#2135 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371285660, 44.36900610829383851, 25.49999999999999645 ) ) ;
+#2136 = ORIENTED_EDGE ( 'NONE', *, *, #917, .F. ) ;
+#2137 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, -12.50000000000000000 ) ) ;
+#2138 = EDGE_LOOP ( 'NONE', ( #1905, #562, #2608, #720, #1563, #974, #2522, #960, #2572, #1203 ) ) ;
+#2139 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2140 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2141 = CARTESIAN_POINT ( 'NONE', ( -33.62350536456727212, 34.94245463026271636, -17.56719610498104700 ) ) ;
+#2142 = ORIENTED_EDGE ( 'NONE', *, *, #1787, .F. ) ;
+#2143 = ADVANCED_FACE ( 'NONE', ( #218 ), #1671, .F. ) ;
+#2144 = CARTESIAN_POINT ( 'NONE', ( -42.87529148811361779, 12.62347441469825782, -7.713113091876421912 ) ) ;
+#2145 = AXIS2_PLACEMENT_3D ( 'NONE', #1118, #466, #703 ) ;
+#2146 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2147 = AXIS2_PLACEMENT_3D ( 'NONE', #2313, #1504, #1025 ) ;
+#2148 = AXIS2_PLACEMENT_3D ( 'NONE', #1681, #2547, #1057 ) ;
+#2149 = EDGE_CURVE ( 'NONE', #119, #1369, #293, .T. ) ;
+#2150 = FACE_OUTER_BOUND ( 'NONE', #609, .T. ) ;
+#2151 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#2152 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#2153 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2154 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, -12.50000000000000000 ) ) ;
+#2155 = VERTEX_POINT ( 'NONE', #2096 ) ;
+#2156 = VECTOR ( 'NONE', #2253, 1000.000000000000000 ) ;
+#2157 = CARTESIAN_POINT ( 'NONE', ( -32.08407190151646660, 34.85601975930839558, -16.54074713282725639 ) ) ;
+#2158 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 12.50000000000000000 ) ) ;
+#2159 = CARTESIAN_POINT ( 'NONE', ( -33.69087072485709200, 33.99846891094080092, -16.46928847759323844 ) ) ;
+#2160 = ORIENTED_EDGE ( 'NONE', *, *, #94, .F. ) ;
+#2161 = CARTESIAN_POINT ( 'NONE', ( -43.89767380834007326, 9.653961638331107764, -8.950931874450899883 ) ) ;
+#2162 = CYLINDRICAL_SURFACE ( 'NONE', #747, 5.499999999999994671 ) ;
+#2163 = B_SPLINE_CURVE_WITH_KNOTS ( 'NONE', 3,
+ ( #2453, #531, #2245, #359, #1858, #1216, #2072, #152, #2709, #1093, #1732, #637, #2128, #853, #1702, #415, #2324, #2765, #2351, #867, #1530, #1716 ),
+ .UNSPECIFIED., .F., .F.,
+ ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 0.000000000000000000, 0.0007627592689956853248, 0.001144138903493522024, 0.001525518537991358940, 0.001906898172489198242, 0.002288277806987037109, 0.003051037075982718314, 0.003813796344978399085, 0.004576555613974079423, 0.005339314882969760194, 0.006102074151965440965 ),
+ .UNSPECIFIED. ) ;
+#2164 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 20.50000000000000000 ) ) ;
+#2165 = LINE ( 'NONE', #244, #798 ) ;
+#2166 = CARTESIAN_POINT ( 'NONE', ( -33.03257378525533738, 31.99619020741757680, -0.4999999999999995559 ) ) ;
+#2167 = ADVANCED_FACE ( 'NONE', ( #2760 ), #1076, .F. ) ;
+#2168 = PLANE ( 'NONE', #1534 ) ;
+#2169 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -11.01637359942223959 ) ) ;
+#2170 = CARTESIAN_POINT ( 'NONE', ( -32.80462327031782621, 41.87376302754837099, 9.242520617631695856 ) ) ;
+#2171 = CARTESIAN_POINT ( 'NONE', ( -33.20266196594004526, 33.90990497528945014, -15.94086684414193478 ) ) ;
+#2172 = CARTESIAN_POINT ( 'NONE', ( 37.65733024778801763, 27.77905981872672569, 18.11655635681410459 ) ) ;
+#2173 = ORIENTED_EDGE ( 'NONE', *, *, #1088, .F. ) ;
+#2174 = CARTESIAN_POINT ( 'NONE', ( -42.34167783727841083, 14.17335700015280864, -9.275019158363502214 ) ) ;
+#2175 = CARTESIAN_POINT ( 'NONE', ( -42.65021550190652277, 7.485593379376848766, 14.43847249979325120 ) ) ;
+#2176 = CIRCLE ( 'NONE', #923, 32.50000000000000000 ) ;
+#2177 = EDGE_LOOP ( 'NONE', ( #2483, #1866, #21, #61 ) ) ;
+#2178 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 6.284924945759425619, 12.58999354329103681 ) ) ;
+#2179 = LINE ( 'NONE', #657, #2371 ) ;
+#2180 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#2181 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 12.50000000000000000 ) ) ;
+#2182 = ORIENTED_EDGE ( 'NONE', *, *, #2264, .T. ) ;
+#2183 = EDGE_CURVE ( 'NONE', #1213, #839, #644, .T. ) ;
+#2184 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2185 = CARTESIAN_POINT ( 'NONE', ( -34.45097498341976916, 37.09192911891105382, 7.302165524867300306 ) ) ;
+#2186 = CARTESIAN_POINT ( 'NONE', ( -1.750000000000000888, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#2187 = CARTESIAN_POINT ( 'NONE', ( 44.53214698414766559, 7.811132234350878889, 14.79092204521036891 ) ) ;
+#2188 = CARTESIAN_POINT ( 'NONE', ( -35.06775177350758810, 33.21237578291635373, -17.43362278359201767 ) ) ;
+#2189 = CARTESIAN_POINT ( 'NONE', ( -33.54851438728952218, 39.71312885736417542, 16.79537387900164092 ) ) ;
+#2190 = CARTESIAN_POINT ( 'NONE', ( -31.91191708823010487, 37.19759781552239986, -13.13251385117886549 ) ) ;
+#2191 = AXIS2_PLACEMENT_3D ( 'NONE', #837, #1241, #2099 ) ;
+#2192 = CARTESIAN_POINT ( 'NONE', ( -32.36304737523853703, 33.19390749586611378, -10.53192484173048094 ) ) ;
+#2193 = CARTESIAN_POINT ( 'NONE', ( 28.36585991138574769, 54.76616564920129804, 20.50000000000000000 ) ) ;
+#2194 = FACE_OUTER_BOUND ( 'NONE', #355, .T. ) ;
+#2195 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#2196 = VECTOR ( 'NONE', #1953, 1000.000000000000114 ) ;
+#2197 = VERTEX_POINT ( 'NONE', #411 ) ;
+#2198 = CARTESIAN_POINT ( 'NONE', ( -34.67866013361472710, 34.51018141257993932, -18.05938415897110616 ) ) ;
+#2199 = CARTESIAN_POINT ( 'NONE', ( -36.80118201742489248, 30.26574514010100714, 6.931169661504697821 ) ) ;
+#2200 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220313, 33.09510371101608683, -13.98362640057776041 ) ) ;
+#2201 = CARTESIAN_POINT ( 'NONE', ( 40.17685806913529234, 20.46108306199920435, 17.52236565250806777 ) ) ;
+#2202 = EDGE_CURVE ( 'NONE', #410, #2404, #2176, .T. ) ;
+#2203 = CIRCLE ( 'NONE', #319, 5.499999999999996447 ) ;
+#2204 = CARTESIAN_POINT ( 'NONE', ( -34.39467578440337547, 33.83453276706526225, -17.04087496986395323 ) ) ;
+#2205 = CARTESIAN_POINT ( 'NONE', ( -43.61372823025195800, 10.47868249396838536, 16.46493364688603833 ) ) ;
+#2206 = CARTESIAN_POINT ( 'NONE', ( -32.02647746074757151, 32.37817243387397781, -11.95058920248304979 ) ) ;
+#2207 = CARTESIAN_POINT ( 'NONE', ( -32.48921376111908188, 33.37121617934870699, -10.22975609381638229 ) ) ;
+#2208 = FACE_OUTER_BOUND ( 'NONE', #672, .T. ) ;
+#2209 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#2210 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#2211 = VERTEX_POINT ( 'NONE', #193 ) ;
+#2212 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, -9.749999999999998224 ) ) ;
+#2213 = CARTESIAN_POINT ( 'NONE', ( -39.33771734142730736, 22.89837003650002956, 7.131934116793763323 ) ) ;
+#2214 = CARTESIAN_POINT ( 'NONE', ( 43.54841234348915435, 10.66839269963269032, 11.16680329600797528 ) ) ;
+#2215 = CARTESIAN_POINT ( 'NONE', ( -41.87309194091779574, 10.15314363899527805, 15.85494385505698389 ) ) ;
+#2216 = EDGE_CURVE ( 'NONE', #373, #290, #1147, .T. ) ;
+#2217 = AXIS2_PLACEMENT_3D ( 'NONE', #1777, #55, #2602 ) ;
+#2218 = CARTESIAN_POINT ( 'NONE', ( -33.05944098404254561, 33.84561590497992967, -9.246554580333423701 ) ) ;
+#2219 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688394565, 32.64726791736439537, -17.54951290997401614 ) ) ;
+#2220 = LINE ( 'NONE', #313, #2411 ) ;
+#2221 = CARTESIAN_POINT ( 'NONE', ( 32.04197879035449859, 44.08886640157222558, 13.28475262339375895 ) ) ;
+#2222 = EDGE_LOOP ( 'NONE', ( #2726, #399, #2562, #2173 ) ) ;
+#2223 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2224 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2225 = CYLINDRICAL_SURFACE ( 'NONE', #1163, 2.750000000000002665 ) ;
+#2226 = EDGE_CURVE ( 'NONE', #1956, #1002, #20, .T. ) ;
+#2227 = CARTESIAN_POINT ( 'NONE', ( -31.83792765984055251, 37.04464140922890181, -13.30065058924384580 ) ) ;
+#2228 = CARTESIAN_POINT ( 'NONE', ( -34.16219127776945186, 34.83175252575671976, -8.123775874914993977 ) ) ;
+#2229 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, 4.500000000000003553 ) ) ;
+#2230 = CARTESIAN_POINT ( 'NONE', ( -35.22977879528890810, 32.91002671737059160, -17.51004901566757965 ) ) ;
+#2231 = ADVANCED_FACE ( 'NONE', ( #397, #877 ), #2306, .T. ) ;
+#2232 = VECTOR ( 'NONE', #2518, 1000.000000000000114 ) ;
+#2233 = CIRCLE ( 'NONE', #2145, 1.000000000000000888 ) ;
+#2234 = CARTESIAN_POINT ( 'NONE', ( -36.01076853932008248, 30.27612420079759303, -17.84773735216146306 ) ) ;
+#2235 = PRODUCT_DEFINITION_FORMATION_WITH_SPECIFIED_SOURCE ( 'ANY', '', #1775, .NOT_KNOWN. ) ;
+#2236 = ORIENTED_EDGE ( 'NONE', *, *, #199, .T. ) ;
+#2237 = UNCERTAINTY_MEASURE_WITH_UNIT (LENGTH_MEASURE( 1.000000000000000082E-05 ), #1835, 'distance_accuracy_value', 'NONE');
+#2238 = CIRCLE ( 'NONE', #2544, 4.999999999999999112 ) ;
+#2239 = CARTESIAN_POINT ( 'NONE', ( -42.92726601009342602, 6.534591258039863071, 11.60396289541549208 ) ) ;
+#2240 = CARTESIAN_POINT ( 'NONE', ( -35.56052505735149794, 33.86923731822577821, 0.7239554166110148126 ) ) ;
+#2241 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2242 = VECTOR ( 'NONE', #1577, 1000.000000000000000 ) ;
+#2243 = CARTESIAN_POINT ( 'NONE', ( -37.93723037723203362, 26.96608898181698777, -19.00220868657622120 ) ) ;
+#2244 = CARTESIAN_POINT ( 'NONE', ( -32.68649813412481819, 33.58807897161884881, -9.829750986092030018 ) ) ;
+#2245 = CARTESIAN_POINT ( 'NONE', ( -35.14038434858451865, 33.11359712655156073, -7.529260729552952114 ) ) ;
+#2246 = LINE ( 'NONE', #995, #176 ) ;
+#2247 = EDGE_CURVE ( 'NONE', #2746, #1000, #1922, .T. ) ;
+#2248 = CARTESIAN_POINT ( 'NONE', ( -30.45505023850549620, 33.55159498885787883, -10.48396092735532115 ) ) ;
+#2249 = ORIENTED_EDGE ( 'NONE', *, *, #2386, .F. ) ;
+#2250 = CARTESIAN_POINT ( 'NONE', ( -40.85494385521242577, 13.64803341803770920, 8.126908059186071398 ) ) ;
+#2251 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -0.5000000000000004441 ) ) ;
+#2252 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, -12.50000000000000000 ) ) ;
+#2253 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#2254 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639392139, 32.97280677233769097, -6.532393833667017091 ) ) ;
+#2255 = FACE_OUTER_BOUND ( 'NONE', #269, .T. ) ;
+#2256 = CARTESIAN_POINT ( 'NONE', ( 43.67796458834995121, 10.29210778281894001, 12.80896965692306289 ) ) ;
+#2257 = CARTESIAN_POINT ( 'NONE', ( -35.10281040004661435, 34.19759691359718090, -6.777322849519937797 ) ) ;
+#2258 = CARTESIAN_POINT ( 'NONE', ( 35.17200529167838141, 34.99769424203288537, 18.17961152446044437 ) ) ;
+#2259 = ORIENTED_EDGE ( 'NONE', *, *, #1424, .T. ) ;
+#2260 = SURFACE_STYLE_FILL_AREA ( #1731 ) ;
+#2261 = CARTESIAN_POINT ( 'NONE', ( -43.05645483273530516, 12.09728489066292312, 13.23782609026732793 ) ) ;
+#2262 = ORIENTED_EDGE ( 'NONE', *, *, #196, .T. ) ;
+#2263 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732932604, -17.67565352282140623 ) ) ;
+#2264 = EDGE_CURVE ( 'NONE', #1962, #1729, #797, .T. ) ;
+#2265 = CARTESIAN_POINT ( 'NONE', ( -32.28090642499900298, 43.07922933215968442, 14.27218612157844824 ) ) ;
+#2266 = ORIENTED_EDGE ( 'NONE', *, *, #1088, .T. ) ;
+#2267 = CARTESIAN_POINT ( 'NONE', ( -42.97198885086694986, 6.381075869361561459, -11.93415139584733176 ) ) ;
+#2268 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, -1.000000000000000000 ) ) ;
+#2269 = CARTESIAN_POINT ( 'NONE', ( -10.88337182448036522, 14.37644341801383874, -20.50000000000000000 ) ) ;
+#2270 = CARTESIAN_POINT ( 'NONE', ( 43.57608948207057153, 10.58800436055510197, 12.02167594495914926 ) ) ;
+#2271 = CARTESIAN_POINT ( 'NONE', ( -30.92286176549616528, 34.14536489278964382, -9.701579716680802434 ) ) ;
+#2272 = CARTESIAN_POINT ( 'NONE', ( -37.06990144990430025, 26.64055012684498180, -18.00186888864244850 ) ) ;
+#2273 = ORIENTED_EDGE ( 'NONE', *, *, #342, .T. ) ;
+#2274 = CIRCLE ( 'NONE', #307, 1.000000000000000888 ) ;
+#2275 =( NAMED_UNIT ( * ) SI_UNIT ( $, .STERADIAN. ) SOLID_ANGLE_UNIT ( ) );
+#2276 = CARTESIAN_POINT ( 'NONE', ( -42.65021550190652277, 7.485593379376942913, 10.56152750020676656 ) ) ;
+#2277 = EDGE_LOOP ( 'NONE', ( #2038, #2581 ) ) ;
+#2278 = EDGE_LOOP ( 'NONE', ( #2642, #326, #847, #1243, #878 ) ) ;
+#2279 = CARTESIAN_POINT ( 'NONE', ( -35.25154046079260439, 34.76668408449911851, 1.942973882721104051 ) ) ;
+#2280 = CARTESIAN_POINT ( 'NONE', ( -35.86915977639390718, 32.97280677233769808, -18.46760616633223506 ) ) ;
+#2281 = EDGE_CURVE ( 'NONE', #988, #1933, #125, .T. ) ;
+#2282 = ORIENTED_EDGE ( 'NONE', *, *, #2149, .F. ) ;
+#2283 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2284 = CARTESIAN_POINT ( 'NONE', ( -33.13905957460556095, 34.99861909221774425, -17.28973884272261685 ) ) ;
+#2285 = CARTESIAN_POINT ( 'NONE', ( 42.17307833103708958, 9.123411929629037687, 9.594017505116772426 ) ) ;
+#2286 = CARTESIAN_POINT ( 'NONE', ( 36.06038663569744784, 30.10580538339059231, 7.143150278459511782 ) ) ;
+#2287 = CARTESIAN_POINT ( 'NONE', ( -40.07226420255257437, 20.76487628786011896, -18.81591770358692273 ) ) ;
+#2288 = ORIENTED_EDGE ( 'NONE', *, *, #898, .T. ) ;
+#2289 = CARTESIAN_POINT ( 'NONE', ( -43.61372823025177325, 10.47868249396893781, 8.535066353114014959 ) ) ;
+#2290 = ORIENTED_EDGE ( 'NONE', *, *, #598, .T. ) ;
+#2291 = ORIENTED_EDGE ( 'NONE', *, *, #2437, .F. ) ;
+#2292 = LINE ( 'NONE', #2539, #482 ) ;
+#2293 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371285660, 44.36900610829382430, -0.4999999999999935052 ) ) ;
+#2294 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 0.000000000000000000, 12.50000000000000000 ) ) ;
+#2295 = ORIENTED_EDGE ( 'NONE', *, *, #1304, .F. ) ;
+#2296 = ADVANCED_FACE ( 'NONE', ( #2728 ), #2574, .F. ) ;
+#2297 = CARTESIAN_POINT ( 'NONE', ( -43.41506391757393857, 11.05570363667571776, -13.93184824265265398 ) ) ;
+#2298 = CARTESIAN_POINT ( 'NONE', ( -32.98116575626914226, 34.99960826761601851, -17.19097729128900554 ) ) ;
+#2299 = EDGE_CURVE ( 'NONE', #1677, #652, #909, .T. ) ;
+#2300 = CARTESIAN_POINT ( 'NONE', ( 44.84138201281258063, 6.912958087210565594, 11.33622530713484622 ) ) ;
+#2301 = CARTESIAN_POINT ( 'NONE', ( 34.11999303975470355, 36.76639026393851850, 8.101832367193273043 ) ) ;
+#2302 = CARTESIAN_POINT ( 'NONE', ( -38.49829738907315857, 25.33646801997604214, -18.01526548192777355 ) ) ;
+#2303 = ORIENTED_EDGE ( 'NONE', *, *, #2425, .F. ) ;
+#2304 = AXIS2_PLACEMENT_3D ( 'NONE', #2137, #178, #7 ) ;
+#2305 = CIRCLE ( 'NONE', #1457, 2.750000000000002665 ) ;
+#2306 = PLANE ( 'NONE', #662 ) ;
+#2307 = ORIENTED_EDGE ( 'NONE', *, *, #1938, .T. ) ;
+#2308 = FACE_BOUND ( 'NONE', #1339, .T. ) ;
+#2309 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2310 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, 15.25000000000000355 ) ) ;
+#2311 = ORIENTED_EDGE ( 'NONE', *, *, #2532, .F. ) ;
+#2312 = CARTESIAN_POINT ( 'NONE', ( -44.78057694612997608, 7.089566601275853586, -11.03879978481555391 ) ) ;
+#2313 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#2314 =( BOUNDED_CURVE ( ) B_SPLINE_CURVE ( 3, ( #1131, #2623, #1740, #702 ),
+ .UNSPECIFIED., .F., .F. )
+ B_SPLINE_CURVE_WITH_KNOTS ( ( 4, 4 ),
+ ( 1.570796326794896780, 1.978349873612872400 ),
+ .UNSPECIFIED. )
+ CURVE ( ) GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_CURVE ( ( 1.000000000000000000, 0.9862061738295025970, 0.9862061738295025970, 1.000000000000000000 ) )
+ REPRESENTATION_ITEM ( '' ) );
+#2315 = CARTESIAN_POINT ( 'NONE', ( 42.65021550190652277, 7.485593379376897616, 10.56152750020675057 ) ) ;
+#2316 = CARTESIAN_POINT ( 'NONE', ( 41.64362746466552778, 10.94080219668967935, 8.865452871613511476 ) ) ;
+#2317 = CARTESIAN_POINT ( 'NONE', ( -43.66413554073318437, 10.33227429663400798, -11.65534640261178012 ) ) ;
+#2318 = EDGE_LOOP ( 'NONE', ( #2703, #1239 ) ) ;
+#2319 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2320 = ORIENTED_EDGE ( 'NONE', *, *, #1867, .T. ) ;
+#2321 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#2322 = CARTESIAN_POINT ( 'NONE', ( -30.07837841308500160, 32.68977866257936427, -12.24089654967807839 ) ) ;
+#2323 = AXIS2_PLACEMENT_3D ( 'NONE', #1254, #2114, #370 ) ;
+#2324 = CARTESIAN_POINT ( 'NONE', ( -32.68661006668391877, 33.58832361165990932, -9.829381099390571919 ) ) ;
+#2325 = VERTEX_POINT ( 'NONE', #2328 ) ;
+#2326 = AXIS2_PLACEMENT_3D ( 'NONE', #2501, #1586, #2024 ) ;
+#2327 = CARTESIAN_POINT ( 'NONE', ( -38.42973826897114265, 21.97278139373025851, -7.060226771103836896 ) ) ;
+#2328 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 5.000000000000000888, -0.5000000000000004441 ) ) ;
+#2329 = CARTESIAN_POINT ( 'NONE', ( -4.000000000000000000, 24.99999999999999645, 9.500000000000001776 ) ) ;
+#2330 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371286370, 44.36900610829383851, 12.50000000000000000 ) ) ;
+#2331 = CARTESIAN_POINT ( 'NONE', ( -44.59669480201735325, 7.623652887282098334, -14.60501732734054769 ) ) ;
+#2332 = ORIENTED_EDGE ( 'NONE', *, *, #721, .T. ) ;
+#2333 = AXIS2_PLACEMENT_3D ( 'NONE', #211, #2560, #640 ) ;
+#2334 = ADVANCED_FACE ( 'NONE', ( #227 ), #2162, .F. ) ;
+#2335 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2336 = ORIENTED_EDGE ( 'NONE', *, *, #1975, .T. ) ;
+#2337 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#2338 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2339 = EDGE_LOOP ( 'NONE', ( #1466, #1450, #1488, #1299 ) ) ;
+#2340 = CARTESIAN_POINT ( 'NONE', ( -37.06990144989914882, 26.64055012684494628, -6.998131111358390832 ) ) ;
+#2341 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759554404, 12.50000000000000000 ) ) ;
+#2342 = CARTESIAN_POINT ( 'NONE', ( 43.00000000005575629, 12.26125813590289404, 12.30585011635568371 ) ) ;
+#2343 = CARTESIAN_POINT ( 'NONE', ( 42.41046231078231443, 13.97357227301002958, 7.331800433583523180 ) ) ;
+#2344 = CYLINDRICAL_SURFACE ( 'NONE', #1300, 5.000000000000000888 ) ;
+#2345 = ORIENTED_EDGE ( 'NONE', *, *, #2129, .T. ) ;
+#2346 = LINE ( 'NONE', #633, #2036 ) ;
+#2347 = ORIENTED_EDGE ( 'NONE', *, *, #2299, .F. ) ;
+#2348 = EDGE_CURVE ( 'NONE', #2559, #40, #2719, .T. ) ;
+#2349 = AXIS2_PLACEMENT_3D ( 'NONE', #1685, #1033, #1699 ) ;
+#2350 = FACE_OUTER_BOUND ( 'NONE', #1664, .T. ) ;
+#2351 = CARTESIAN_POINT ( 'NONE', ( -32.39801739792145696, 33.25423752648580233, -10.43455839533363161 ) ) ;
+#2352 = CARTESIAN_POINT ( 'NONE', ( -31.80633473863719374, 35.85147799646298239, -12.74769626147816481 ) ) ;
+#2353 = EDGE_CURVE ( 'NONE', #2555, #887, #2370, .T. ) ;
+#2354 = ORIENTED_EDGE ( 'NONE', *, *, #1201, .F. ) ;
+#2355 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, -12.50000000000000000 ) ) ;
+#2356 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, -17.99999999999999645 ) ) ;
+#2357 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2358 = FACE_OUTER_BOUND ( 'NONE', #561, .T. ) ;
+#2359 = CARTESIAN_POINT ( 'NONE', ( 43.77853523924351009, 10.00000000000000000, 4.500000000000003553 ) ) ;
+#2360 = CARTESIAN_POINT ( 'NONE', ( -42.99649525893036639, 6.296955301573404107, -12.72668589585888377 ) ) ;
+#2361 = CARTESIAN_POINT ( 'NONE', ( -28.36585991138575125, 54.76616564920128383, 20.50000000000000000 ) ) ;
+#2362 = ORIENTED_EDGE ( 'NONE', *, *, #1981, .T. ) ;
+#2363 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, 12.50000000000000000 ) ) ;
+#2364 = CARTESIAN_POINT ( 'NONE', ( -33.96085114238744751, 33.97871654396673335, -16.71175975894927745 ) ) ;
+#2365 = EDGE_LOOP ( 'NONE', ( #605, #2598, #366, #1411 ) ) ;
+#2366 = CARTESIAN_POINT ( 'NONE', ( -41.08453677334700416, 17.82472731161974266, -6.535712854909187186 ) ) ;
+#2367 = VECTOR ( 'NONE', #2475, 1000.000000000000114 ) ;
+#2368 = CARTESIAN_POINT ( 'NONE', ( 43.77853523924351009, 10.00000000000000000, 4.500000000000003553 ) ) ;
+#2369 = EDGE_CURVE ( 'NONE', #2102, #2735, #2120, .T. ) ;
+#2370 = CIRCLE ( 'NONE', #712, 1.750000000000000888 ) ;
+#2371 = VECTOR ( 'NONE', #1522, 1000.000000000000000 ) ;
+#2372 = ORIENTED_EDGE ( 'NONE', *, *, #2592, .T. ) ;
+#2373 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2374 = CYLINDRICAL_SURFACE ( 'NONE', #1694, 1.750000000000000888 ) ;
+#2375 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 5.000000000000000000, 20.50000000000000000 ) ) ;
+#2376 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2377 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2378 = AXIS2_PLACEMENT_3D ( 'NONE', #1952, #1695, #830 ) ;
+#2379 = DIRECTION ( 'NONE', ( -0.9455286637128582639, 0.3255388549733759795, 0.000000000000000000 ) ) ;
+#2380 = CARTESIAN_POINT ( 'NONE', ( -9.209006928406457959, 19.08775981524249232, -0.4999999999999995559 ) ) ;
+#2381 = CARTESIAN_POINT ( 'NONE', ( -32.36420686467011620, 33.19576849732460033, -14.47113390788103260 ) ) ;
+#2382 = CARTESIAN_POINT ( 'NONE', ( 42.51245935571760981, 7.958454404285582484, 14.77104525622276476 ) ) ;
+#2383 = ORIENTED_EDGE ( 'NONE', *, *, #1325, .T. ) ;
+#2384 = CARTESIAN_POINT ( 'NONE', ( -37.93723037723144387, 26.96608898181869307, -5.997791313423071813 ) ) ;
+#2385 = CARTESIAN_POINT ( 'NONE', ( -34.50846982257036899, 36.92493517148904658, 17.21047068398206292 ) ) ;
+#2386 = EDGE_CURVE ( 'NONE', #1626, #1380, #117, .T. ) ;
+#2387 = CARTESIAN_POINT ( 'NONE', ( 44.90380467601652015, 6.731651258784676628, 13.24295527983301035 ) ) ;
+#2388 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000000000, 16.99999999999999645 ) ) ;
+#2389 = CARTESIAN_POINT ( 'NONE', ( -37.96348091317739915, 26.88984421473294617, -18.07624747191496439 ) ) ;
+#2390 = DIRECTION ( 'NONE', ( 0.3348729792147799866, 0.9422632794457277416, 0.000000000000000000 ) ) ;
+#2391 = EDGE_CURVE ( 'NONE', #699, #1250, #1202, .T. ) ;
+#2392 = ORIENTED_EDGE ( 'NONE', *, *, #1390, .F. ) ;
+#2393 = CARTESIAN_POINT ( 'NONE', ( -30.75531648230274939, 33.96568033141718246, -15.05013505484490821 ) ) ;
+#2394 = CARTESIAN_POINT ( 'NONE', ( -32.03545348354654720, 43.92176981164326577, 11.78006058356860741 ) ) ;
+#2395 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#2396 = CARTESIAN_POINT ( 'NONE', ( 43.51720173354249255, 10.75904401780113062, 13.24358781162051990 ) ) ;
+#2397 = CARTESIAN_POINT ( 'NONE', ( -32.48587777297380796, 33.37418639456181069, -14.77129521455876926 ) ) ;
+#2398 = CARTESIAN_POINT ( 'NONE', ( -43.47300727646558016, 10.88740696221078430, 11.07271280388263612 ) ) ;
+#2399 = CARTESIAN_POINT ( 'NONE', ( -32.19621406304697331, 32.86985622852959210, -11.04015032658462481 ) ) ;
+#2400 = LINE ( 'NONE', #1086, #850 ) ;
+#2401 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2402 = CARTESIAN_POINT ( 'NONE', ( -44.92483465240206897, 6.670569623932271597, -13.03529124250512261 ) ) ;
+#2403 = EDGE_CURVE ( 'NONE', #1656, #1642, #2561, .T. ) ;
+#2404 = VERTEX_POINT ( 'NONE', #2531 ) ;
+#2405 = DIRECTION ( 'NONE', ( -0.3255388549733759240, -0.9455286637128581528, 0.000000000000000000 ) ) ;
+#2406 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 15.25000000000000355 ) ) ;
+#2407 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, -12.50000000000000000 ) ) ;
+#2408 = CARTESIAN_POINT ( 'NONE', ( 36.06038663570628700, 30.10580538338117407, 17.85684972154414751 ) ) ;
+#2409 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -13.75537618510426086 ) ) ;
+#2410 = CARTESIAN_POINT ( 'NONE', ( -36.74416741500050421, 30.43134423835567404, 18.83082239818915582 ) ) ;
+#2411 = VECTOR ( 'NONE', #1366, 1000.000000000000000 ) ;
+#2412 = CARTESIAN_POINT ( 'NONE', ( -31.71128054985143763, 36.78571494027813316, -13.43558096349155662 ) ) ;
+#2413 = AXIS2_PLACEMENT_3D ( 'NONE', #182, #1890, #2737 ) ;
+#2414 = CARTESIAN_POINT ( 'NONE', ( -33.68994425456530450, 34.00744475573307568, -8.526107425713719934 ) ) ;
+#2415 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2416 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2417 = CARTESIAN_POINT ( 'NONE', ( 32.60311442061276921, 42.45904613731271837, 15.38048284069856386 ) ) ;
+#2418 = VECTOR ( 'NONE', #48, 1000.000000000000000 ) ;
+#2419 = EDGE_LOOP ( 'NONE', ( #1929, #1003, #864, #1812, #2311 ) ) ;
+#2420 = ADVANCED_FACE ( 'NONE', ( #1302 ), #1149, .T. ) ;
+#2421 = PLANE ( 'NONE', #348 ) ;
+#2422 = CARTESIAN_POINT ( 'NONE', ( -37.65733024778801763, 27.77905981872669727, 6.883443643188033256 ) ) ;
+#2423 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2424 = CARTESIAN_POINT ( 'NONE', ( 42.27964029246761157, 14.35354525096313694, 15.78330155396011669 ) ) ;
+#2425 = EDGE_CURVE ( 'NONE', #1869, #1271, #1789, .T. ) ;
+#2426 = AXIS2_PLACEMENT_3D ( 'NONE', #245, #689, #2597 ) ;
+#2427 = CARTESIAN_POINT ( 'NONE', ( -32.80223149720249864, 33.68595592459292476, -15.37014069878409472 ) ) ;
+#2428 = CARTESIAN_POINT ( 'NONE', ( -43.67474508509441478, 10.30145884050160277, 11.70830923837313797 ) ) ;
+#2429 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -11.24462381489573914 ) ) ;
+#2430 = VECTOR ( 'NONE', #1393, 1000.000000000000114 ) ;
+#2431 = CARTESIAN_POINT ( 'NONE', ( -32.25660262397823885, 33.00377268879510950, -10.83624211763857303 ) ) ;
+#2432 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371285660, 6.610463800732987671, -0.4999999999999935052 ) ) ;
+#2433 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2434 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, 12.50000000000000000 ) ) ;
+#2435 = EDGE_LOOP ( 'NONE', ( #1672, #2676, #1253, #1899, #1670 ) ) ;
+#2436 = CARTESIAN_POINT ( 'NONE', ( -32.85740444909299640, 41.72045992200722253, 9.514080636735620544 ) ) ;
+#2437 = EDGE_CURVE ( 'NONE', #219, #2551, #1526, .T. ) ;
+#2438 = CARTESIAN_POINT ( 'NONE', ( -32.10231590476908536, 32.60009903557990185, -13.57251263405132136 ) ) ;
+#2439 = CARTESIAN_POINT ( 'NONE', ( -43.96825760039310893, 9.448950784603200148, 15.93434294849810762 ) ) ;
+#2440 = CIRCLE ( 'NONE', #2304, 2.750000000000002665 ) ;
+#2441 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688393855, 32.64726791736441669, -17.54951290997401259 ) ) ;
+#2442 = CARTESIAN_POINT ( 'NONE', ( -34.79718267054763459, 33.54393479310802917, -7.708834455968716526 ) ) ;
+#2443 = LINE ( 'NONE', #2229, #752 ) ;
+#2444 = CYLINDRICAL_SURFACE ( 'NONE', #1290, 5.499999999999996447 ) ;
+#2445 = ORIENTED_EDGE ( 'NONE', *, *, #1270, .F. ) ;
+#2446 = APPLICATION_PROTOCOL_DEFINITION ( 'draft international standard', 'automotive_design', 1998, #1056 ) ;
+#2447 = CARTESIAN_POINT ( 'NONE', ( -32.33154110754892230, 43.24783170891587503, 10.57274774848070109 ) ) ;
+#2448 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2449 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000000000, 44.04346725331308221, 12.85997824090613406 ) ) ;
+#2450 = ADVANCED_FACE ( 'NONE', ( #2680 ), #1386, .F. ) ;
+#2451 = CARTESIAN_POINT ( 'NONE', ( -32.06738264253668547, 32.49761593359419010, -11.63563047650472981 ) ) ;
+#2452 = CARTESIAN_POINT ( 'NONE', ( -32.28992956916303569, 33.06853971673057657, -10.73456288603651565 ) ) ;
+#2453 = CARTESIAN_POINT ( 'NONE', ( -35.31999555688396697, 32.64726791736432432, -7.450487090025976755 ) ) ;
+#2454 = CARTESIAN_POINT ( 'NONE', ( -35.03661225317585348, 33.25975357281244982, -17.41827260215351458 ) ) ;
+#2455 = MECHANICAL_DESIGN_GEOMETRIC_PRESENTATION_REPRESENTATION ( '', ( #221 ), #88 ) ;
+#2456 = AXIS2_PLACEMENT_3D ( 'NONE', #1960, #38, #899 ) ;
+#2457 = CARTESIAN_POINT ( 'NONE', ( -32.95306044124963307, 35.01023595654707066, -7.820292378271018308 ) ) ;
+#2458 = ORIENTED_EDGE ( 'NONE', *, *, #504, .F. ) ;
+#2459 = VECTOR ( 'NONE', #1575, 1000.000000000000000 ) ;
+#2460 = CARTESIAN_POINT ( 'NONE', ( -43.29946974291550532, 11.39144729316937088, 13.87817639135741921 ) ) ;
+#2461 = LINE ( 'NONE', #2679, #1370 ) ;
+#2462 = CARTESIAN_POINT ( 'NONE', ( -32.00000000000147793, 44.21079393475268660, 12.89178716040438744 ) ) ;
+#2463 = CARTESIAN_POINT ( 'NONE', ( -35.59821368629273763, 33.57263803759811793, -7.309878905903493873 ) ) ;
+#2464 = CARTESIAN_POINT ( 'NONE', ( -43.00000000000000000, 6.284924945759544634, 12.50000000000000000 ) ) ;
+#2465 = CARTESIAN_POINT ( 'NONE', ( 43.01186382226871530, 12.22679962567669243, 12.88659521195030777 ) ) ;
+#2466 = CARTESIAN_POINT ( 'NONE', ( 34.27817459305202163, 36.22341709370546425, 7.000000000000002665 ) ) ;
+#2467 = EDGE_CURVE ( 'NONE', #1626, #1698, #1632, .T. ) ;
+#2468 = CARTESIAN_POINT ( 'NONE', ( 34.11999303975556330, 36.76639026393175413, 16.89816763280757428 ) ) ;
+#2469 = VERTEX_POINT ( 'NONE', #1200 ) ;
+#2470 = CARTESIAN_POINT ( 'NONE', ( -44.93248048743234335, 6.648362271204027785, 12.07483222645047505 ) ) ;
+#2471 = ORIENTED_EDGE ( 'NONE', *, *, #2281, .F. ) ;
+#2472 = CARTESIAN_POINT ( 'NONE', ( -31.94552866371286370, 44.36900610829383851, 12.50000000000000000 ) ) ;
+#2473 = FACE_OUTER_BOUND ( 'NONE', #238, .T. ) ;
+#2474 = CARTESIAN_POINT ( 'NONE', ( -35.03662682140280538, 33.25975546703224239, -7.581717401315367866 ) ) ;
+#2475 = DIRECTION ( 'NONE', ( 0.3255388549733759240, 0.9455286637128581528, 0.000000000000000000 ) ) ;
+#2476 = VECTOR ( 'NONE', #1371, 1000.000000000000000 ) ;
+#2477 = CARTESIAN_POINT ( 'NONE', ( 42.71092435547753752, 7.277204487012656209, 10.73119737958446507 ) ) ;
+#2478 = LINE ( 'NONE', #1172, #2430 ) ;
+#2479 = CARTESIAN_POINT ( 'NONE', ( -41.56879248528904469, 16.41820502030883944, -18.22558364296315503 ) ) ;
+#2480 = VERTEX_POINT ( 'NONE', #990 ) ;
+#2481 = CARTESIAN_POINT ( 'NONE', ( -41.26442097287522159, 17.30225306865531465, 8.207638216925946750 ) ) ;
+#2482 = ORIENTED_EDGE ( 'NONE', *, *, #1975, .F. ) ;
+#2483 = ORIENTED_EDGE ( 'NONE', *, *, #1514, .F. ) ;
+#2484 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, -15.25000000000000355 ) ) ;
+#2485 = DIRECTION ( 'NONE', ( 0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#2486 = EDGE_LOOP ( 'NONE', ( #1077, #1718 ) ) ;
+#2487 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 12.50000000000000000 ) ) ;
+#2488 = CARTESIAN_POINT ( 'NONE', ( -32.80035353576547408, 33.68410357598781246, -15.36662494479539021 ) ) ;
+#2489 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 6.284924945759425619, 12.41000645670904490 ) ) ;
+#2490 = CARTESIAN_POINT ( 'NONE', ( 35.24008933273407251, 34.79994392318622687, 7.395706311575591663 ) ) ;
+#2491 = CARTESIAN_POINT ( 'NONE', ( -43.68618486729789652, 10.26823195585393123, -12.07740465410201658 ) ) ;
+#2492 = ORIENTED_EDGE ( 'NONE', *, *, #2091, .F. ) ;
+#2493 = AXIS2_PLACEMENT_3D ( 'NONE', #2375, #2152, #273 ) ;
+#2494 = CARTESIAN_POINT ( 'NONE', ( -42.26572163226823164, 8.805405019716966208, 9.748583515298200552 ) ) ;
+#2495 = EDGE_LOOP ( 'NONE', ( #787, #27 ) ) ;
+#2496 = VECTOR ( 'NONE', #920, 1000.000000000000000 ) ;
+#2497 = EDGE_LOOP ( 'NONE', ( #1901, #1913, #1075, #1452 ) ) ;
+#2498 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#2499 = ADVANCED_FACE ( 'NONE', ( #1857 ), #2225, .F. ) ;
+#2500 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, 9.749999999999998224 ) ) ;
+#2501 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 12.50000000000000000 ) ) ;
+#2502 = ADVANCED_FACE ( 'NONE', ( #2657 ), #331, .F. ) ;
+#2503 = ORIENTED_EDGE ( 'NONE', *, *, #1304, .T. ) ;
+#2504 = CARTESIAN_POINT ( 'NONE', ( -43.13581435095088779, 11.86678491173349137, -13.57447109993831980 ) ) ;
+#2505 = CARTESIAN_POINT ( 'NONE', ( -30.85352967496542220, 34.06144666639386998, -15.18679774478694888 ) ) ;
+#2506 = VECTOR ( 'NONE', #1643, 1000.000000000000000 ) ;
+#2507 = CARTESIAN_POINT ( 'NONE', ( 43.61372823025153167, 10.47868249396968743, 8.535066353113965221 ) ) ;
+#2508 = CARTESIAN_POINT ( 'NONE', ( 32.04197879035282170, 44.08886640158401349, 11.71524737660156035 ) ) ;
+#2509 = CARTESIAN_POINT ( 'NONE', ( -39.54431025431523494, 22.29832024870347595, -18.92882290687671443 ) ) ;
+#2510 = ORIENTED_EDGE ( 'NONE', *, *, #544, .F. ) ;
+#2511 = CARTESIAN_POINT ( 'NONE', ( -42.91187591077667918, 6.587419232237300193, 11.51526756757560932 ) ) ;
+#2512 = VERTEX_POINT ( 'NONE', #2280 ) ;
+#2513 = CARTESIAN_POINT ( 'NONE', ( 4.000000000000001776, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#2514 = LINE ( 'NONE', #385, #1293 ) ;
+#2515 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371285660, 44.36900610829383851, -0.4999999999999935052 ) ) ;
+#2516 = ORIENTED_EDGE ( 'NONE', *, *, #1711, .T. ) ;
+#2517 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2518 = DIRECTION ( 'NONE', ( -0.3255388549733759240, -0.9455286637128581528, -0.000000000000000000 ) ) ;
+#2519 = CARTESIAN_POINT ( 'NONE', ( 42.81541933757674201, 6.918515559511324575, 11.07567117061645057 ) ) ;
+#2520 = CARTESIAN_POINT ( 'NONE', ( 41.87309194091779574, 10.15314363899672934, 9.145056144943021437 ) ) ;
+#2521 = CARTESIAN_POINT ( 'NONE', ( -44.55199895893468920, 7.753472108975101307, -14.73135907980154258 ) ) ;
+#2522 = ORIENTED_EDGE ( 'NONE', *, *, #2369, .F. ) ;
+#2523 = AXIS2_PLACEMENT_3D ( 'NONE', #2542, #1931, #1487 ) ;
+#2524 = CARTESIAN_POINT ( 'NONE', ( -44.94291777066060689, 6.618047148100145272, 12.28728800729306769 ) ) ;
+#2525 = EDGE_LOOP ( 'NONE', ( #241, #1512, #2273, #536 ) ) ;
+#2526 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 15.00000000000000000, 4.500000000000000888 ) ) ;
+#2527 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371285660, 6.610463800732987671, 25.49999999999999645 ) ) ;
+#2528 = LINE ( 'NONE', #2752, #157 ) ;
+#2529 = ORIENTED_EDGE ( 'NONE', *, *, #1221, .T. ) ;
+#2530 = CARTESIAN_POINT ( 'NONE', ( -39.73300686199834786, 17.49918845664574363, -7.453295492615387374 ) ) ;
+#2531 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664219957, 33.09510371101609394, -20.50000000000000000 ) ) ;
+#2532 = EDGE_CURVE ( 'NONE', #1041, #1174, #1824, .T. ) ;
+#2533 = CARTESIAN_POINT ( 'NONE', ( 45.50000000000002132, 5.000000000000000000, -20.50000000000000000 ) ) ;
+#2534 = CARTESIAN_POINT ( 'NONE', ( 43.08988301296579237, 12.00019263240714196, 10.51163916010018085 ) ) ;
+#2535 = CARTESIAN_POINT ( 'NONE', ( -41.04433338031060430, 17.94149819751710950, -16.97182048182517633 ) ) ;
+#2536 = ORIENTED_EDGE ( 'NONE', *, *, #1593, .T. ) ;
+#2537 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2538 = ORIENTED_EDGE ( 'NONE', *, *, #2353, .T. ) ;
+#2539 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, 0.000000000000000000 ) ) ;
+#2540 =( GEOMETRIC_REPRESENTATION_CONTEXT ( 3 ) GLOBAL_UNCERTAINTY_ASSIGNED_CONTEXT ( ( #1175 ) ) GLOBAL_UNIT_ASSIGNED_CONTEXT ( ( #82, #1790, #470 ) ) REPRESENTATION_CONTEXT ( 'NONE', 'WORKASPACE' ) );
+#2541 = CARTESIAN_POINT ( 'NONE', ( -30.07852830399136934, 32.69014495678720067, -12.76246157593145725 ) ) ;
+#2542 = CARTESIAN_POINT ( 'NONE', ( -33.03257378525533738, 31.99619020741757680, -0.4999999999999995559 ) ) ;
+#2543 = VERTEX_POINT ( 'NONE', #2500 ) ;
+#2544 = AXIS2_PLACEMENT_3D ( 'NONE', #1540, #1321, #1351 ) ;
+#2545 = CARTESIAN_POINT ( 'NONE', ( -43.66728881047318112, 10.32311561441032843, -12.59462259923101435 ) ) ;
+#2546 = CARTESIAN_POINT ( 'NONE', ( 44.78694594894730585, 7.071067811865475505, 4.500000000000001776 ) ) ;
+#2547 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#2548 = ADVANCED_FACE ( 'NONE', ( #1425 ), #357, .F. ) ;
+#2549 = CARTESIAN_POINT ( 'NONE', ( 36.78005168384700596, 27.63548691358245080, 7.000000000000002665 ) ) ;
+#2550 = CARTESIAN_POINT ( 'NONE', ( -43.01892871247724770, 12.20627962907637354, -12.01430770672717863 ) ) ;
+#2551 = VERTEX_POINT ( 'NONE', #99 ) ;
+#2552 = VECTOR ( 'NONE', #2153, 1000.000000000000000 ) ;
+#2553 = EDGE_CURVE ( 'NONE', #603, #758, #301, .T. ) ;
+#2554 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 45.00000000000000000, -20.50000000000000000 ) ) ;
+#2555 = VERTEX_POINT ( 'NONE', #735 ) ;
+#2556 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, -0.000000000000000000 ) ) ;
+#2557 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2558 = CARTESIAN_POINT ( 'NONE', ( -32.12243782233524314, 35.39872147352874521, -13.96340192189375884 ) ) ;
+#2559 = VERTEX_POINT ( 'NONE', #1063 ) ;
+#2560 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2561 = LINE ( 'NONE', #212, #1027 ) ;
+#2562 = ORIENTED_EDGE ( 'NONE', *, *, #1610, .F. ) ;
+#2563 = CARTESIAN_POINT ( 'NONE', ( 42.95367559951915126, 6.443937839889398944, 13.21799811442444117 ) ) ;
+#2564 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 0.000000000000000000, -0.000000000000000000 ) ) ;
+#2565 = CARTESIAN_POINT ( 'NONE', ( -42.99999999999999289, 6.284924945759550852, 9.278174593052025187 ) ) ;
+#2566 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2567 = EDGE_LOOP ( 'NONE', ( #352, #1713 ) ) ;
+#2568 = CIRCLE ( 'NONE', #1188, 4.000000000000000000 ) ;
+#2569 = CARTESIAN_POINT ( 'NONE', ( -30.10493428705314045, 32.75481996492078451, -13.14886959080084772 ) ) ;
+#2570 = FACE_OUTER_BOUND ( 'NONE', #2050, .T. ) ;
+#2571 = CARTESIAN_POINT ( 'NONE', ( -34.12734107192669342, 34.79531642946736980, -17.81961929576890924 ) ) ;
+#2572 = ORIENTED_EDGE ( 'NONE', *, *, #1787, .T. ) ;
+#2573 = CARTESIAN_POINT ( 'NONE', ( -43.55993684666466947, 10.63491974265470930, -11.92960100951705371 ) ) ;
+#2574 = PLANE ( 'NONE', #661 ) ;
+#2575 = ORIENTED_EDGE ( 'NONE', *, *, #2115, .F. ) ;
+#2576 = CARTESIAN_POINT ( 'NONE', ( 44.77913493337640460, 7.093754932011568037, 13.97671189358406707 ) ) ;
+#2577 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, -20.50000000000000000 ) ) ;
+#2578 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 1.000000000000000000 ) ) ;
+#2579 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 6.000000000000005329, 12.50000000000000000 ) ) ;
+#2580 = DIRECTION ( 'NONE', ( -0.000000000000000000, -0.000000000000000000, 1.000000000000000000 ) ) ;
+#2581 = ORIENTED_EDGE ( 'NONE', *, *, #2353, .F. ) ;
+#2582 = CARTESIAN_POINT ( 'NONE', ( -32.96180855177377111, 34.99942511581703997, -17.17851078735381876 ) ) ;
+#2583 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2584 = ADVANCED_FACE ( 'NONE', ( #1441 ), #1914, .F. ) ;
+#2585 = CARTESIAN_POINT ( 'NONE', ( -32.49066575616762265, 33.37302784643443943, -14.77344424567526637 ) ) ;
+#2586 = CARTESIAN_POINT ( 'NONE', ( 32.72692620558952825, 41.54822417257454958, 15.25632870815823061 ) ) ;
+#2587 = VERTEX_POINT ( 'NONE', #1930 ) ;
+#2588 = AXIS2_PLACEMENT_3D ( 'NONE', #2764, #592, #852 ) ;
+#2589 = CARTESIAN_POINT ( 'NONE', ( -40.06395933689159250, 20.78899779720964602, -7.460982371409011549 ) ) ;
+#2590 = TOROIDAL_SURFACE ( 'NONE', #1874, 5.000000000000000000, 5.000000000000000000 ) ;
+#2591 = CARTESIAN_POINT ( 'NONE', ( -35.17200529167902801, 34.99769424203096690, 18.17961152445757023 ) ) ;
+#2592 = EDGE_CURVE ( 'NONE', #1041, #2664, #1429, .T. ) ;
+#2593 = VECTOR ( 'NONE', #287, 1000.000000000000000 ) ;
+#2594 = ORIENTED_EDGE ( 'NONE', *, *, #1221, .F. ) ;
+#2595 = CARTESIAN_POINT ( 'NONE', ( 43.16529390050471449, 11.78116146411336373, 11.35073131986692196 ) ) ;
+#2596 = LINE ( 'NONE', #311, #91 ) ;
+#2597 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2598 = ORIENTED_EDGE ( 'NONE', *, *, #935, .T. ) ;
+#2599 = CIRCLE ( 'NONE', #500, 1.000000000000002665 ) ;
+#2600 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2601 =( BOUNDED_SURFACE ( ) B_SPLINE_SURFACE ( 3, 2, (
+ ( #1181, #346, #763 ),
+ ( #2662, #124, #2650 ),
+ ( #2230, #324, #2025 ),
+ ( #740, #1598, #555 ),
+ ( #2454, #533, #1389 ),
+ ( #981, #1833, #1414 ),
+ ( #2698, #252, #362 ),
+ ( #171, #650, #2571 ),
+ ( #1515, #1717, #1284 ),
+ ( #1652, #1635, #2141 ),
+ ( #2364, #1946, #1313 ),
+ ( #26, #1110, #2284 ),
+ ( #2159, #1861, #2298 ),
+ ( #2089, #1026, #2712 ),
+ ( #445, #1879, #237 ),
+ ( #2171, #882, #1232 ),
+ ( #1703, #779, #155 ),
+ ( #2488, #568, #868 ),
+ ( #1298, #2729, #638 ),
+ ( #1733, #1501, #1094 ),
+ ( #2585, #2558, #2505 ),
+ ( #1080, #666, #1531 ),
+ ( #2381, #795, #584 ),
+ ( #459, #1432, #1220 ),
+ ( #814, #2352, #2075 ),
+ ( #1010, #1446, #375 ),
+ ( #430, #224, #1932 ),
+ ( #11, #682, #2200 ) ),
+ .UNSPECIFIED., .F., .F., .T. )
+ B_SPLINE_SURFACE_WITH_KNOTS ( ( 4, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 2, 4 ),
+ ( 3, 3 ),
+ ( 0.008757071320785850960, 0.009183922389969279704, 0.009610773459152708448, 0.01046447559751956420, 0.01089132666670299121, 0.01131817773588641995, 0.01174502880506984870, 0.01217187987425327744, 0.01302558201262013493, 0.01387928415098699068, 0.01430613522017041769, 0.01473298628935384644, 0.01515983735853727518, 0.01558668842772070393 ),
+ ( 0.000000000000000000, 1.000000000000000000 ),
+ .UNSPECIFIED. )
+ GEOMETRIC_REPRESENTATION_ITEM ( ) RATIONAL_B_SPLINE_SURFACE ( (
+ ( 1.000000000000000000, 0.8290880361187440428, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8283414753042251943, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8272488154433742524, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8237641646937760020, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8213289271647844503, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8113567961825397523, 1.000000000000000000),
+ ( 1.000000000000000000, 0.8007958503489036595, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7781053080360526009, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7693130770049906308, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7498607328506173841, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7391817961567436202, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7159459271895894927, 1.000000000000000000),
+ ( 1.000000000000000000, 0.7033057285803756553, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6765659509163293173, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6625482921293183169, 1.000000000000000000),
+ ( 1.000000000000000000, 0.6186898459787799620, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5869418376578605656, 1.000000000000000000),
+ ( 1.000000000000000000, 0.5195157893409696470, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4845337398371500903, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4302931466504548164, 1.000000000000000000),
+ ( 1.000000000000000000, 0.4119160469453999052, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3745556284437132066, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3555730519643583998, 1.000000000000000000),
+ ( 1.000000000000000000, 0.3169518206573033181, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2972931862310035922, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2575326329851665430, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2373943807889200286, 1.000000000000000000),
+ ( 1.000000000000000000, 0.2168728904164849147, 1.000000000000000000) ) )
+ REPRESENTATION_ITEM ( '' ) SURFACE ( ) );
+#2602 = DIRECTION ( 'NONE', ( 0.9455286637128577087, -0.3255388549733774228, 0.000000000000000000 ) ) ;
+#2603 = CARTESIAN_POINT ( 'NONE', ( -44.94552866371285660, 6.610463800732931716, 12.50000000000000000 ) ) ;
+#2604 = CARTESIAN_POINT ( 'NONE', ( 41.13454712838774441, 12.68826868899451021, 16.64362746466427367 ) ) ;
+#2605 = ADVANCED_FACE ( 'NONE', ( #2127 ), #1454, .F. ) ;
+#2606 = CARTESIAN_POINT ( 'NONE', ( -35.28468642979672154, 32.76846984181587175, -17.53426905273371972 ) ) ;
+#2607 = CARTESIAN_POINT ( 'NONE', ( -39.33771734144060161, 22.89837003650885805, 17.86806588320328260 ) ) ;
+#2608 = ORIENTED_EDGE ( 'NONE', *, *, #263, .T. ) ;
+#2609 = ADVANCED_FACE ( 'NONE', ( #209, #371, #1715 ), #1078, .T. ) ;
+#2610 = CARTESIAN_POINT ( 'NONE', ( -31.40548039598481367, 34.52978301891334212, -9.109092070848220146 ) ) ;
+#2611 = LINE ( 'NONE', #1725, #1294 ) ;
+#2612 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#2613 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, 7.000000000000004441 ) ) ;
+#2614 = EDGE_LOOP ( 'NONE', ( #2471, #2678 ) ) ;
+#2615 = CARTESIAN_POINT ( 'NONE', ( -45.50000000000000000, 5.000000000000000000, -135.3085362679971126 ) ) ;
+#2616 = CARTESIAN_POINT ( 'NONE', ( -34.73009560827659215, 34.67215538705031719, 7.694174863920470564 ) ) ;
+#2617 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 0.000000000000000000, 9.749999999999998224 ) ) ;
+#2618 = CARTESIAN_POINT ( 'NONE', ( 44.72738788084893713, 7.244054414484648596, 14.18329770745364549 ) ) ;
+#2619 = CARTESIAN_POINT ( 'NONE', ( -41.26442097287803534, 17.30225306865554202, 16.79236178307624172 ) ) ;
+#2620 = VERTEX_POINT ( 'NONE', #1499 ) ;
+#2621 = CARTESIAN_POINT ( 'NONE', ( -32.01655176691529192, 32.34941709639529961, -12.94019891333433847 ) ) ;
+#2622 = CARTESIAN_POINT ( 'NONE', ( -31.86181303274648968, 34.77032875699576664, -8.655019049852235469 ) ) ;
+#2623 = CARTESIAN_POINT ( 'NONE', ( -36.74760041787510545, 27.74687895898127366, -7.000000000000003553 ) ) ;
+#2624 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, -9.749999999999998224 ) ) ;
+#2625 = ADVANCED_FACE ( 'NONE', ( #2350 ), #1470, .F. ) ;
+#2626 = CARTESIAN_POINT ( 'NONE', ( 31.94552866371286370, 44.36900610829383851, 12.50000000000000000 ) ) ;
+#2627 = PRODUCT_DEFINITION_SHAPE ( 'NONE', 'NONE', #2111 ) ;
+#2628 = EDGE_LOOP ( 'NONE', ( #1859, #1296, #924, #771, #1408 ) ) ;
+#2629 = CARTESIAN_POINT ( 'NONE', ( -33.43177830774572357, 34.97387511022177620, -17.46161727953160536 ) ) ;
+#2630 = CARTESIAN_POINT ( 'NONE', ( -35.17200529168098200, 34.99769424202530388, 6.820388475540610784 ) ) ;
+#2631 = CARTESIAN_POINT ( 'NONE', ( -32.14518621856249325, 32.72879920981659296, -11.24462381489573914 ) ) ;
+#2632 = CARTESIAN_POINT ( 'NONE', ( 42.43541227556363538, 8.222925807528202569, 14.93394762038420787 ) ) ;
+#2633 = CARTESIAN_POINT ( 'NONE', ( -35.13191470449255149, 33.10592325286900461, -17.46444534699889317 ) ) ;
+#2634 = CARTESIAN_POINT ( 'NONE', ( -42.26572163226845191, 8.805405019716850745, 15.25141648470139621 ) ) ;
+#2635 = CIRCLE ( 'NONE', #43, 1.750000000000000888 ) ;
+#2636 = CARTESIAN_POINT ( 'NONE', ( -30.07847176690764357, 32.69000639195882485, -12.23827161217920434 ) ) ;
+#2637 = VECTOR ( 'NONE', #2566, 1000.000000000000000 ) ;
+#2638 = CARTESIAN_POINT ( 'NONE', ( -32.00623955549127686, 35.50097029340265209, -11.40582650544678067 ) ) ;
+#2639 = DIRECTION ( 'NONE', ( 0.000000000000000000, -1.000000000000000000, 0.000000000000000000 ) ) ;
+#2640 = FACE_OUTER_BOUND ( 'NONE', #869, .T. ) ;
+#2641 = CARTESIAN_POINT ( 'NONE', ( 32.20724408224754143, 43.60885282342698588, 14.05137967152550083 ) ) ;
+#2642 = ORIENTED_EDGE ( 'NONE', *, *, #1171, .T. ) ;
+#2643 = EDGE_CURVE ( 'NONE', #440, #1264, #2514, .T. ) ;
+#2644 = CARTESIAN_POINT ( 'NONE', ( -32.28090642499899587, 43.07922933215529326, 10.72781387842156953 ) ) ;
+#2645 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 10.00000000000000000, -0.5000000000000004441 ) ) ;
+#2646 = CARTESIAN_POINT ( 'NONE', ( -42.41046231078231443, 13.97357227300998872, 17.66819956641551315 ) ) ;
+#2647 = CARTESIAN_POINT ( 'NONE', ( -31.99997465284142706, 32.30150177216948038, -12.39073177440097062 ) ) ;
+#2648 = CARTESIAN_POINT ( 'NONE', ( -34.12997056330182488, 34.79430200687454544, -7.179147523306513179 ) ) ;
+#2649 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#2650 = CARTESIAN_POINT ( 'NONE', ( -35.79910006918283472, 33.17629541993650122, -18.44744178313778349 ) ) ;
+#2651 = DIRECTION ( 'NONE', ( -0.000000000000000000, 1.000000000000000000, -0.000000000000000000 ) ) ;
+#2652 = EDGE_LOOP ( 'NONE', ( #81, #647 ) ) ;
+#2653 = PRODUCT_CONTEXT ( 'NONE', #478, 'mechanical' ) ;
+#2654 = CARTESIAN_POINT ( 'NONE', ( -32.20724408224754143, 43.60885282342698588, 10.94862032847449917 ) ) ;
+#2655 = DIRECTION ( 'NONE', ( -0.000000000000000000, -1.000000000000000000, -0.000000000000000000 ) ) ;
+#2656 = EDGE_CURVE ( 'NONE', #2692, #2740, #192, .T. ) ;
+#2657 = FACE_OUTER_BOUND ( 'NONE', #1226, .T. ) ;
+#2658 = AXIS2_PLACEMENT_3D ( 'NONE', #881, #1675, #2583 ) ;
+#2659 = CARTESIAN_POINT ( 'NONE', ( -32.10328509828497090, 32.60361429427916846, -11.43419781602488960 ) ) ;
+#2660 = CARTESIAN_POINT ( 'NONE', ( -33.46221696823123182, 34.96965691954395083, -7.521081067193763126 ) ) ;
+#2661 = DIRECTION ( 'NONE', ( -0.3255388549733759240, -0.9455286637128581528, 0.000000000000000000 ) ) ;
+#2662 = CARTESIAN_POINT ( 'NONE', ( -35.28047570514758746, 32.78292353033875628, -17.53245117473092307 ) ) ;
+#2663 = CARTESIAN_POINT ( 'NONE', ( 31.99999999999999289, 44.04346725332046475, 9.278174593052023411 ) ) ;
+#2664 = VERTEX_POINT ( 'NONE', #808 ) ;
+#2665 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2666 = CARTESIAN_POINT ( 'NONE', ( -43.29723134156039066, 11.39794873719420565, 11.00854648015628356 ) ) ;
+#2667 = CARTESIAN_POINT ( 'NONE', ( -31.99996665789103645, 32.30147870511411412, -12.38919095465474562 ) ) ;
+#2668 = CARTESIAN_POINT ( 'NONE', ( -32.20724408224923252, 43.60885282341672564, 14.05137967152085032 ) ) ;
+#2669 = EDGE_CURVE ( 'NONE', #1656, #484, #1693, .T. ) ;
+#2670 = CARTESIAN_POINT ( 'NONE', ( -31.91184017190819944, 37.19743678734352699, -11.86713072258852186 ) ) ;
+#2671 = EDGE_CURVE ( 'NONE', #1870, #1438, #977, .T. ) ;
+#2672 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 5.000000000000004441, 9.749999999999998224 ) ) ;
+#2673 = CARTESIAN_POINT ( 'NONE', ( 43.20911154149919042, 11.65389298324529044, 13.74738253266046684 ) ) ;
+#2674 = CARTESIAN_POINT ( 'NONE', ( -32.35763824740754302, 34.92288471850496734, -8.246680545295799192 ) ) ;
+#2675 = CARTESIAN_POINT ( 'NONE', ( 39.29637667370386112, 23.01844414047112508, 19.00000000000000000 ) ) ;
+#2676 = ORIENTED_EDGE ( 'NONE', *, *, #2592, .F. ) ;
+#2677 = CARTESIAN_POINT ( 'NONE', ( -43.08855886698635373, 12.00403861870095135, 13.39448169394137267 ) ) ;
+#2678 = ORIENTED_EDGE ( 'NONE', *, *, #1325, .F. ) ;
+#2679 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 75.00000000000001421, -9.749999999999998224 ) ) ;
+#2680 = FACE_OUTER_BOUND ( 'NONE', #1883, .T. ) ;
+#2681 = TOROIDAL_SURFACE ( 'NONE', #1038, 4.499999999999996447, 1.000000000000000000 ) ;
+#2682 = CARTESIAN_POINT ( 'NONE', ( -44.94138669699436406, 6.622494156546505195, -12.23209848671220712 ) ) ;
+#2683 = CARTESIAN_POINT ( 'NONE', ( -34.94235511436430386, 34.31780708879535524, -6.837713445772488186 ) ) ;
+#2684 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000000000, 12.50000000000000000 ) ) ;
+#2685 = ADVANCED_FACE ( 'NONE', ( #1688 ), #822, .F. ) ;
+#2686 = CLOSED_SHELL ( 'NONE', ( #1374, #2420, #1830, #1036, #648, #2095, #1498, #1538, #2334, #528, #859, #2605, #1773, #671, #2584, #1394, #2231, #2450, #1309, #156, #749, #467, #2296, #316, #2739, #2609, #295, #1847, #2499, #2167, #160, #327, #2502, #1358, #429, #1320, #724, #19, #2625, #1817, #950, #2548, #1067, #2143, #2685, #635, #807, #614, #1154, #396, #177, #534, #938, #996, #1340, #879, #2059, #1233 ) ) ;
+#2687 = CARTESIAN_POINT ( 'NONE', ( 43.00000000000000000, 6.284924945759610360, 12.50000000000000000 ) ) ;
+#2688 = CARTESIAN_POINT ( 'NONE', ( -30.74911587642074906, 33.95800866149480868, -9.960050070298168023 ) ) ;
+#2689 = CARTESIAN_POINT ( 'NONE', ( -43.41506391757481964, 11.05570363667380995, -11.06815175734728918 ) ) ;
+#2690 = VERTEX_POINT ( 'NONE', #1485 ) ;
+#2691 = CARTESIAN_POINT ( 'NONE', ( -41.64362746466424881, 10.94080219669100629, 8.865452871612232499 ) ) ;
+#2692 = VERTEX_POINT ( 'NONE', #1108 ) ;
+#2693 = DIRECTION ( 'NONE', ( 1.000000000000000000, 0.000000000000000000, 0.000000000000000000 ) ) ;
+#2694 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 5.000000000000004441, -12.50000000000000000 ) ) ;
+#2695 = ORIENTED_EDGE ( 'NONE', *, *, #2669, .F. ) ;
+#2696 = CARTESIAN_POINT ( 'NONE', ( -43.64654375114167095, 10.38336970674818716, -13.47706415008192060 ) ) ;
+#2697 = CARTESIAN_POINT ( 'NONE', ( -35.10826710052241140, 33.14810076276800288, -7.546809860019490834 ) ) ;
+#2698 = CARTESIAN_POINT ( 'NONE', ( -34.61899141748324382, 33.70810724951781623, -17.19051702500752654 ) ) ;
+#2699 = CIRCLE ( 'NONE', #734, 4.000000000000000000 ) ;
+#2700 = CARTESIAN_POINT ( 'NONE', ( 43.64736563080724352, 10.38098255457818553, 13.50780290469519329 ) ) ;
+#2701 = EDGE_CURVE ( 'NONE', #2512, #2692, #257, .T. ) ;
+#2702 = CARTESIAN_POINT ( 'NONE', ( -43.30319550006222329, 11.38062581941505158, -11.11271680004639428 ) ) ;
+#2703 = ORIENTED_EDGE ( 'NONE', *, *, #199, .F. ) ;
+#2704 = CARTESIAN_POINT ( 'NONE', ( -43.51720173354104304, 10.75904401780104180, 11.75641218837916036 ) ) ;
+#2705 = ORIENTED_EDGE ( 'NONE', *, *, #2026, .T. ) ;
+#2706 = VERTEX_POINT ( 'NONE', #1776 ) ;
+#2707 = FACE_OUTER_BOUND ( 'NONE', #1506, .T. ) ;
+#2708 = CARTESIAN_POINT ( 'NONE', ( -32.03545348354649036, 43.92176981164325866, 13.21993941643107640 ) ) ;
+#2709 = CARTESIAN_POINT ( 'NONE', ( -34.29859475771816335, 33.87937285550358268, -8.026125860938119416 ) ) ;
+#2710 = ORIENTED_EDGE ( 'NONE', *, *, #1159, .T. ) ;
+#2711 = CARTESIAN_POINT ( 'NONE', ( 1.750000000000000888, 24.99999999999999645, 20.50000000000000000 ) ) ;
+#2712 = CARTESIAN_POINT ( 'NONE', ( -32.67747835695446668, 34.97898588707287360, -16.98792452810606335 ) ) ;
+#2713 = CARTESIAN_POINT ( 'NONE', ( 43.54841234349629531, 10.66839269962366465, 13.83319670399970747 ) ) ;
+#2714 = CARTESIAN_POINT ( 'NONE', ( 34.45097498341975495, 37.09192911891108224, 7.302165524865620760 ) ) ;
+#2715 = CARTESIAN_POINT ( 'NONE', ( -41.85058052292291109, 10.23041621433535120, -15.87251176738538305 ) ) ;
+#2716 = ORIENTED_EDGE ( 'NONE', *, *, #979, .F. ) ;
+#2717 = EDGE_CURVE ( 'NONE', #1438, #1098, #1920, .T. ) ;
+#2718 = AXIS2_PLACEMENT_3D ( 'NONE', #280, #1137, #2423 ) ;
+#2719 = CIRCLE ( 'NONE', #1648, 5.000000000000000888 ) ;
+#2720 = CARTESIAN_POINT ( 'NONE', ( -42.85920530509991266, 6.768216077037892298, 11.25047455158265031 ) ) ;
+#2721 = ORIENTED_EDGE ( 'NONE', *, *, #2701, .F. ) ;
+#2722 = ORIENTED_EDGE ( 'NONE', *, *, #447, .T. ) ;
+#2723 = FACE_BOUND ( 'NONE', #275, .T. ) ;
+#2724 = CARTESIAN_POINT ( 'NONE', ( -30.24108867664220668, 33.09510371101609394, -11.01637359942223959 ) ) ;
+#2725 = CARTESIAN_POINT ( 'NONE', ( -1.750000000000000888, 65.00000000000000000, 20.50000000000000000 ) ) ;
+#2726 = ORIENTED_EDGE ( 'NONE', *, *, #1663, .F. ) ;
+#2727 = LINE ( 'NONE', #2711, #757 ) ;
+#2728 = FACE_OUTER_BOUND ( 'NONE', #1230, .T. ) ;
+#2729 = CARTESIAN_POINT ( 'NONE', ( -32.37073422028953473, 35.27469942497417321, -14.60481899148886953 ) ) ;
+#2730 = AXIS2_PLACEMENT_3D ( 'NONE', #361, #1651, #1219 ) ;
+#2731 = CARTESIAN_POINT ( 'NONE', ( 34.50846982257023399, 36.92493517149642202, 7.789529316018065863 ) ) ;
+#2732 = CARTESIAN_POINT ( 'NONE', ( -38.87646853286651805, 20.43933743288788207, -17.84423805688090781 ) ) ;
+#2733 = ORIENTED_EDGE ( 'NONE', *, *, #1644, .T. ) ;
+#2734 = CARTESIAN_POINT ( 'NONE', ( 0.000000000000000000, 65.00000000000000000, 9.500000000000001776 ) ) ;
+#2735 = VERTEX_POINT ( 'NONE', #1746 ) ;
+#2736 = CARTESIAN_POINT ( 'NONE', ( 17.22413793103449464, 15.00000000000000000, -0.5000000000000004441 ) ) ;
+#2737 = DIRECTION ( 'NONE', ( 0.000000000000000000, 0.000000000000000000, -1.000000000000000000 ) ) ;
+#2738 = CARTESIAN_POINT ( 'NONE', ( -9.209006928406465065, 19.08775981524247456, 4.500000000000000888 ) ) ;
+#2739 = ADVANCED_FACE ( 'NONE', ( #457, #1341 ), #2421, .T. ) ;
+#2740 = VERTEX_POINT ( 'NONE', #2169 ) ;
+#2741 = CARTESIAN_POINT ( 'NONE', ( -37.50000000000000000, 25.16419609954000691, 7.000000000000004441 ) ) ;
+#2742 = CARTESIAN_POINT ( 'NONE', ( -44.93248048743244283, 6.648362271203721363, 12.92516777354943258 ) ) ;
+#2743 = LINE ( 'NONE', #2164, #904 ) ;
+#2744 = CARTESIAN_POINT ( 'NONE', ( 32.80462327031869307, 41.87376302754584856, 9.242520617630988866 ) ) ;
+#2745 = CARTESIAN_POINT ( 'NONE', ( -43.64654375114278650, 10.38336970674933646, -11.52293584991913455 ) ) ;
+#2746 = VERTEX_POINT ( 'NONE', #501 ) ;
+#2747 = AXIS2_PLACEMENT_3D ( 'NONE', #1886, #863, #1048 ) ;
+#2748 = CARTESIAN_POINT ( 'NONE', ( 32.00000000000000000, 44.04346725332046475, 12.50000000000000000 ) ) ;
+#2749 = VERTEX_POINT ( 'NONE', #2212 ) ;
+#2750 = CARTESIAN_POINT ( 'NONE', ( -40.62089237294614463, 19.17138398148123457, -20.51905119829299906 ) ) ;
+#2751 = DIRECTION ( 'NONE', ( 0.9422632794457277416, -0.3348729792147797646, 0.000000000000000000 ) ) ;
+#2752 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, 17.99999999999999645 ) ) ;
+#2753 = ORIENTED_EDGE ( 'NONE', *, *, #1561, .T. ) ;
+#2754 = EDGE_CURVE ( 'NONE', #630, #440, #41, .T. ) ;
+#2755 = CARTESIAN_POINT ( 'NONE', ( -35.89971384576189450, 32.88406237842237090, -7.200207811996365059 ) ) ;
+#2756 = CARTESIAN_POINT ( 'NONE', ( 44.94552866371287791, 6.610463800732935269, 12.60635600570758896 ) ) ;
+#2757 = CARTESIAN_POINT ( 'NONE', ( 33.35637253533361957, 39.38759000238739105, 8.865452871614355246 ) ) ;
+#2758 = DIRECTION ( 'NONE', ( -1.000000000000000000, 0.000000000000000000, -0.000000000000000000 ) ) ;
+#2759 = ORIENTED_EDGE ( 'NONE', *, *, #434, .F. ) ;
+#2760 = FACE_OUTER_BOUND ( 'NONE', #1684, .T. ) ;
+#2761 = VERTEX_POINT ( 'NONE', #1327 ) ;
+#2762 = CIRCLE ( 'NONE', #1646, 4.000000000000000000 ) ;
+#2763 = AXIS2_PLACEMENT_3D ( 'NONE', #774, #2485, #565 ) ;
+#2764 = CARTESIAN_POINT ( 'NONE', ( 37.50000000000000000, 75.00000000000001421, -12.50000000000000000 ) ) ;
+#2765 = CARTESIAN_POINT ( 'NONE', ( -32.48400049863987959, 33.37196717446787630, -10.23269389697909482 ) ) ;
+ENDSEC;
+END-ISO-10303-21;
diff --git a/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount.stl b/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount.stl
new file mode 100755
index 0000000000..a266ec7d48
Binary files /dev/null and b/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount.stl differ
diff --git a/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount_simplified.stl b/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount_simplified.stl
new file mode 100755
index 0000000000..62ef2ca512
Binary files /dev/null and b/jsk_fetch_robot/jsk_fetch_accessories/head_l515/fetch_head_l515_mount_simplified.stl differ
diff --git a/jsk_fetch_robot/jsk_fetch_startup/CMakeLists.txt b/jsk_fetch_robot/jsk_fetch_startup/CMakeLists.txt
index be9259dac6..20cade468e 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/CMakeLists.txt
+++ b/jsk_fetch_robot/jsk_fetch_startup/CMakeLists.txt
@@ -10,7 +10,19 @@ endif()
## Find catkin macros and libraries
## if COMPONENTS list like find_package(catkin REQUIRED COMPONENTS xyz)
## is used, also find other catkin packages
-find_package(catkin)
+find_package(catkin REQUIRED COMPONENTS
+ roscpp
+ std_msgs
+ sensor_msgs
+ geometry_msgs
+ tf
+ tf2
+ tf2_geometry_msgs
+ tf2_ros
+ tf2_eigen
+ tf2_msgs
+)
+find_package(Boost REQUIRED COMPONENTS)
###################################
## catkin specific configuration ##
@@ -20,6 +32,18 @@ catkin_package()
catkin_add_env_hooks(99.jsk_fetch_startup SHELLS bash zsh sh
DIRECTORY ${CMAKE_CURRENT_SOURCE_DIR}/env-hooks)
+###########
+## Build ##
+###########
+include_directories(
+ ${catkin_INCLUDE_DIRS}
+ ${Boost_INCLUDE_DIRS}
+)
+add_executable(trashbins_pose_estimator src/trashbins_pose_estimator.cpp)
+target_link_libraries(trashbins_pose_estimator
+ ${catkin_LIBRARIES}
+)
+
#############
## Install ##
#############
@@ -51,9 +75,25 @@ macro(configure_icon_files icol iname)
endif()
endmacro(configure_icon_files)
+macro(configure_dialogflow_hotword_yaml dname)
+ set(FETCH_NAME ${dname})
+ if (${FETCH_NAME} STREQUAL "fetch15")
+ set(ROBOT_NICKNAME_KANA "ヘンゼル")
+ set(ROBOT_NICKNAME_HIRA "へんぜる")
+ configure_file(${CMAKE_CURRENT_SOURCE_DIR}/config/dialogflow_hotword.yaml.in
+ ${CMAKE_CURRENT_SOURCE_DIR}/config/${FETCH_NAME}_dialogflow_hotword.yaml)
+ elseif (${FETCH_NAME} STREQUAL "fetch1075")
+ set(ROBOT_NICKNAME_KANA "グレーテル")
+ set(ROBOT_NICKNAME_HIRA "ぐれーてる")
+ configure_file(${CMAKE_CURRENT_SOURCE_DIR}/config/dialogflow_hotword.yaml.in
+ ${CMAKE_CURRENT_SOURCE_DIR}/config/${FETCH_NAME}_dialogflow_hotword.yaml)
+ endif()
+endmacro(configure_dialogflow_hotword_yaml)
+
configure_icon_files(blue fetch15)
configure_icon_files(red fetch1075)
-
+configure_dialogflow_hotword_yaml(fetch15)
+configure_dialogflow_hotword_yaml(fetch1075)
#############
## Testing ##
diff --git a/jsk_fetch_robot/jsk_fetch_startup/README.md b/jsk_fetch_robot/jsk_fetch_startup/README.md
index a509050a34..d23cac2634 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/README.md
+++ b/jsk_fetch_robot/jsk_fetch_startup/README.md
@@ -1,5 +1,28 @@
# jsk_fetch_startup
+- [jsk_fetch_startup](#jsk_fetch_startup)
+ - [SetUp (Running following commands in the first time)](#setup-running-following-commands-in-the-first-time)
+ - [Install a udev rule](#install-a-udev-rule)
+ - [For realsense](#for-realsense)
+ - [supervisor](#supervisor)
+ - [cron](#cron)
+ - [mongodb](#mongodb)
+ - [Teleoperation](#teleoperation)
+ - [Maintenance](#maintenance)
+ - [re-roslaunch jsk_fetch_startup fetch_bringup.launch](#re-roslaunch-jsk_fetch_startup-fetch_bringuplaunch)
+ - [re-roslaunch fetch_bringup fetch.launch](#re-roslaunch-fetch_bringup-fetchlaunch)
+ - [Clock Synchronization](#clock-synchronization)
+ - [Network](#network)
+ - [General description](#general-description)
+ - [Case description](#case-description)
+ - [Access point](#access-point)
+ - [Log](#log)
+ - [Apps](#apps)
+ - [Note](#note)
+ - [Add fetch to rwt_app_chooser](#add-fetch-to-rwt_app_chooser)
+ - [Execute demos](#execute-demos)
+ - [Administration](#administration)
+
## SetUp (Running following commands in the first time)
### Install a udev rule
@@ -7,80 +30,137 @@
rosrun jsk_fetch_startup install_udev.sh
```
-#### For realsense
-udev rule have to be manually installed according to [this issue](https://github.com/IntelRealSense/realsense-ros/issues/1426) when using realsense-ros from ROS repository.
+### For realsense
+
+Before start this, remove `librealsense2` and `realsense2-ros` from ROS repository and set not to be installed.
+
+```bash
+sudo apt purge ros-melodic-librealsense2* ros-melodic-realsense*
+sudo apt-mark hold ros-melodic-librealsense2 ros-melodic-realsense2-camera
+```
+
+After that, please add Intel repository and install `librealsense2` packages. (see [this page](https://github.com/IntelRealSense/librealsense/blob/master/doc/distribution_linux.md#installing-the-packages))
+
+```bash
+sudo apt-key adv --keyserver keyserver.ubuntu.com --recv-key F6E65AC044F831AC80A06380C8B3A55A6F3EFCDE || sudo apt-key adv --keyserver hkp://keyserver.ubuntu.com:80 --recv-key F6E65AC044F831AC80A06380C8B3A55A6F3EFCDE
+sudo add-apt-repository "deb https://librealsense.intel.com/Debian/apt-repo $(lsb_release -cs) main" -u
+sudo apt install librealsense2=2.45.0-0~realsense0.4551
+sudo apt install librealsense2-dev=2.45.0-0~realsense0.4551
+sudo apt-mark hold librealsense2 librealsense2-dev
+```
+
+### Install config.bash
+
+JSK fetch system uses some enviroment variables. To set them, copy `config.bash` to `/var/lib/robot/config.bash` and modify it.
+
+```bash
+roscd jsk_fetch_startup
+sudo cp config/config.bash /var/lib/robot/config.bash
+```
+
+descriptions of each variable are below.
+
+- `USE_BASE_CAMERA_MOUNT`
+ + Flag for robot model
+- `USE_HEAD_BOX`
+ + Flag for robot model
+- `USE_HEAD_L515`
+ + Flag for robot model
+- `USE_INSTA360_STAND`
+ + Flag for robot model
+- `RS_SERIAL_NO_T265`
+ + Serial number of Realsense T265 for visual odometry.
+ + realsense will not be launched when this is blank.
+- `RS_SERIAL_NO_D435_FRONTRIGHT`
+ + Serial number of Realsense D435 on the base
+ + realsense will not be launched when this is blank.
+- `RS_SERIAL_NO_D435_FRONTLEFT`
+ + Serial number of Realsense D435 on the base
+ + realsense will not be launched when this is blank.
+- `RS_SERIAL_NO_L515_HEAD`
+ + Serial number of Realsense L515 on the head
+ + realsense will not be launched when this is blank.
+- `NETWORK_DEFAULT_WIFI_INTERFACE`
+ + Wi-Fi network interface for network management scripts (e.g. `network_monitor.py` and `network-log-wifi.sh`)
+- `NETWORK_DEFAULT_PROFILE_ID`
+ + Network manager profile ID for network management scripts (`network_monitor.py`)
++ `NETWORK_DEFAULT_ROS_INTERFACE`
+ + Network interface or IP address or hostname which is used for ROS connection.
+ + `rossetclient $NETWORK_DEFAULT_ROS_INTERFACE` is executed to set `ROS_IP` or `ROS_HOSTNAME`.
+
+It is also recommended to add lines below to each users's bashrc in the robot PC.
```bash
-wget https://github.com/IntelRealSense/librealsense/raw/master/config/99-realsense-libusb.rules
-sudo mv 99-realsense-libusb.rules /etc/udev/rules.d/
-sudo udevadm control --reload-rules && sudo udevadm trigger
+source /var/lib/robot/config.bash
+rossetmaster localhost
+rossetip $NETWORK_DEFAULT_ROS_INTERFACE
```
### supervisor
+
Important jobs for fetch operation are managed by supervisor.
Here is a list of jobs that are managed by supervisor.
- - roscore
+- roscore
Start roscore
- - robot
+- robot
Launch Minimum ROS programs to run fetch
- - jsk-fetch-startup
+- jsk-fetch-startup
Launch ROS programs extended by JSK
- - jsk-network-monitor
+- jsk-network-monitor
Restart the network manager automatically if ping does not work.
- - jsk-log-wifi
+- jsk-log-wifi
Monitor network condition
- - jsk-app-scheduler
+- jsk-app-scheduler
Scheduler to launch [app](https://github.com/knorth55/app_manager_utils/tree/master/app_scheduler) at a fixed time
- - jsk-object-detector
+- jsk-object-detector
Object detection using fetch's head camera and [coral_usb_ros](https://github.com/knorth55/coral_usb_ros)
- - jsk-panorama-object-detector:
+- jsk-panorama-object-detector:
Object detection using fetch's 360 camera and [coral_usb_ros](https://github.com/knorth55/coral_usb_ros)
- - jsk-human-pose-estimator
+- jsk-human-pose-estimator
Human pose estimation using fetch's head camera and [coral_usb_ros](https://github.com/knorth55/coral_usb_ros)
- - jsk-panorama-human-pose-estimator
+- jsk-panorama-human-pose-estimator
Human pose estimation using fetch's 360 camera and [coral_usb_ros](https://github.com/knorth55/coral_usb_ros)
- - jsk-dialog
+- jsk-dialog
Launch [dialogflow_task_exective](https://github.com/jsk-ros-pkg/jsk_3rdparty/tree/master/dialogflow_task_executive)
- - jsk-gdrive
+- jsk-gdrive
Launch [app](https://github.com/knorth55/app_manager_utils/tree/master/app_uploader) to upload data to Goole Drive
- - jsk-dstat
+- jsk-dstat
Monitor fetch's resource using dstat command
- - jsk-lifelog
+- jsk-lifelog
Launch program to save fetch's [lifelog](https://github.com/jsk-ros-pkg/jsk_robot/tree/master/jsk_robot_common/jsk_robot_startup/lifelog)
-
Install supervisor config files. e.g. `robot.conf`, `jsk_fetch_startup.conf` ...
-```
+```bash
su -c 'rosrun jsk_fetch_startup install_supervisor.sh'
```
@@ -90,17 +170,17 @@ Previously, upstart was used, but it has been moved to supervisor. This is becau
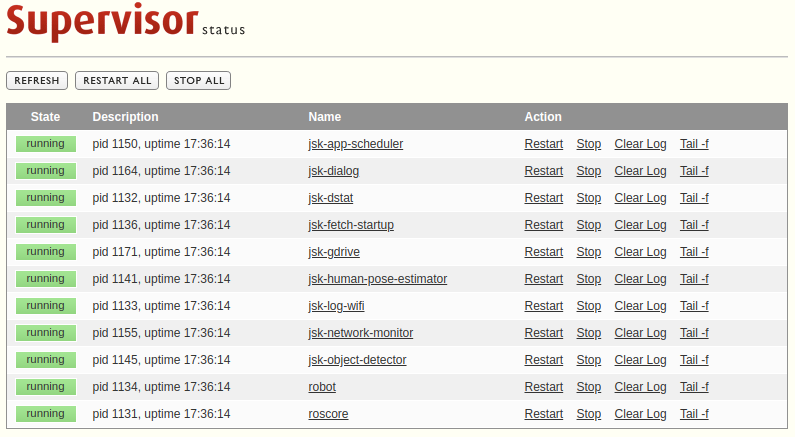
-
### cron
+
Install cron jobs for root user and fetch user. e.g. `shutdown`, `update_workspace`.
-```
+```bash
su -c 'rosrun jsk_fetch_startup install_cron.sh'
```
### mongodb
-```
+```bash
sudo mkdir -p /var/lib/robot/mongodb_store/
# to see the db items from http://lcoalhost/rockmongo
@@ -112,11 +192,7 @@ sudo mv rockmongo-1.1.7 /var/www/html/rockmongo
# $MONGO["servers"][$i]["control_auth"] = false; // true;//enable control users, works only if mongo_auth=false
```
-### Coral Edge TPU
-Create ROS workspace for Coral Edge TPU. Please see:
-https://github.com/knorth55/coral_usb_ros
-
-### Teleoperation
+## Teleoperation
For the JSK safe teleop system, please see [data flow diagram of safe_teleop.launch](https://github.com/jsk-ros-pkg/jsk_robot/tree/master/jsk_robot_common/jsk_robot_startup#launchsafe_teleoplaunch)
@@ -128,19 +204,22 @@ The numbers assigned to the joystick are as follows.
## Maintenance
### re-roslaunch jsk_fetch_startup fetch_bringup.launch
-```
+
+```bash
sudo supervisorctl restart jsk-fetch-startup
```
### re-roslaunch fetch_bringup fetch.launch
-```
+
+```bash
sudo supervisorctl restart robot
```
### [Clock Synchronization](https://github.com/fetchrobotics/docs/blob/0c1c63ab47952063bf60280e74b4ff3ae07fd914/source/computer.rst)
install `chrony` and add ```server `gethostip -d fetch15` offline minpoll 8``` to /etc/chrony/chrony.conf, restart chronyd by `sudo /etc/init.d/chrony restart` and wait for few seconds, if you get
-```
+
+```bash
$ chronyc tracking
Reference ID : 133.11.216.145 (fetch15.jsk.imi.i.u-tokyo.ac.jp)
Stratum : 4
@@ -156,21 +235,22 @@ Root dispersion : 0.018803 seconds
Update interval : 2.1 seconds
Leap status : Normal
```
-it works, if you get `127.127.1.1` for `Reference ID`, something wrong
+it works, if you get `127.127.1.1` for `Reference ID`, something wrong
## Network
+
### General description
+
Fetch has wired and wireless network connections.
If we use both of wired and wireless connections as DHCP, DNS holds two IP addresses for same hostname (fetch15 in this case).
This cause problems in network such as ROS communication or ssh connection.
-The solution we take now (2016/11/01) is using wired connection as static IP.
-By doing so, DNS holds only one IP adress (for wireless connection) for fetch hostname.
-
### Case description
+
If you see the following result, it is OK.
-```
+
+```bash
$ nslookup fetch15.jsk.imi.i.u-tokyo.ac.jp
Server: 127.0.1.1
Address: 127.0.1.1#53
@@ -180,24 +260,70 @@ Address: 133.11.216.145
```
If two or more IP addresses apper, something is wrong.
-Please connect display, open a window of network manager, and check that wired connection uses static IP.
+Please connect display, open a window of network manager.
-### Access point
-Define access point setting, such as ssid:
-```
+### Network configuration
+
+Ubuntu 18.04 uses [NetworkManager](https://wiki.archlinux.jp/index.php/NetworkManager) for network configuration.
+Network manager's profiles are in `/etc/NetworkManager/system-connections`.
+
+```bash
cd /etc/NetworkManager/system-connections
```
-### Log
+You can also see profiles with `nmcli`, `nmtui` or `nm-connection-editor` (GUI) command.
+
+To see available connection profiles.
+
+```bash
+fetch@fetch1075:~$ nmcli connection
+NAME UUID TYPE DEVICE
+netplan-eth1 433e484b-3493-3640-9368-395c0c752304 ethernet eth1
+sanshiro-73B2 1f0f9db6-e448-41ef-9d27-e1ed4e48a4f5 wifi wlan1
+Wired connection 1 eb557bda-e1bf-3ab7-89e9-1f839cd6b88a ethernet --
+fetch1075-hotspot b85c6a8f-9d28-4cf3-95a0-6dc37229863d wifi --
+sanshiro-outside d7ee2165-c93a-4050-862d-d0c381a546ab wifi --
+fetch@fetch1075:~$ nmcli c show sanshiro-73B2
+```
+
+To activate some connection.
+
+```bash
+fetch@fetch1075:~$ sudo nmcli c up sanshiro-outside
+[sudo] password for fetch:
+Connection successfully activated (D-Bus active path: /org/freedesktop/NetworkManager/ActiveConnection/5)
+fetch@fetch1075:~$ sudo nmcli c up sanshiro-73B2
+Connection successfully activated (D-Bus active path: /org/freedesktop/NetworkManager/ActiveConnection/6)
+```
+
+To edit connection profiles, use `nmtui` or `nm-connection-editor`
+
+### Default connection profiles
+
+fetch15 and fetch1075 has `sanshiro-73B2` and `sanshiro-outside` connections by default. (If not, please make them)
+
+- `sanshiro-73B2` (Connect `sanshiro` with fixed BSSID)
+- `sanshiro-outside` (Connect `sanshiro` without fixed BSSID)
+
+By default, `sanshiro-73B2` is activated for stable connection in 73B2.
+If you want to use fetch outside of 73B2, it is recommended to activate `sanshiro-outside`.
+
+```bash
+sudo nmcli c up sanshiro-outside
+```
+
+## Log
tmuxinator makes it easy to check the important logs of fetch from command line. Currently, it shows the logs of the supervisor jobs.
Install tmuxinator config.
+
```bash
rosrun jsk_fetch_startup install_tmuxinator.sh
```
Show logs
+
```bash
tmuxinator log
```
@@ -234,6 +360,7 @@ tmuxinator log
You can not run this on Firefox. Please use Google Chrome.
### Add fetch to rwt_app_chooser
+
1. Access [http://tork-a.github.io/visualization_rwt/rwt_app_chooser](http://tork-a.github.io/visualization_rwt/rwt_app_chooser "website").
- Be careful to access the site via http, not https, to to enable websocket communication.
- Modern browsers may automatically redirect from http to https.
@@ -244,7 +371,27 @@ You can not run this on Firefox. Please use Google Chrome.
1. Click `ADD ROBOT` button
### Execute demos
+
1. Click `fetch15` at `Select Robot` window
1. Select task which are shown with icons.
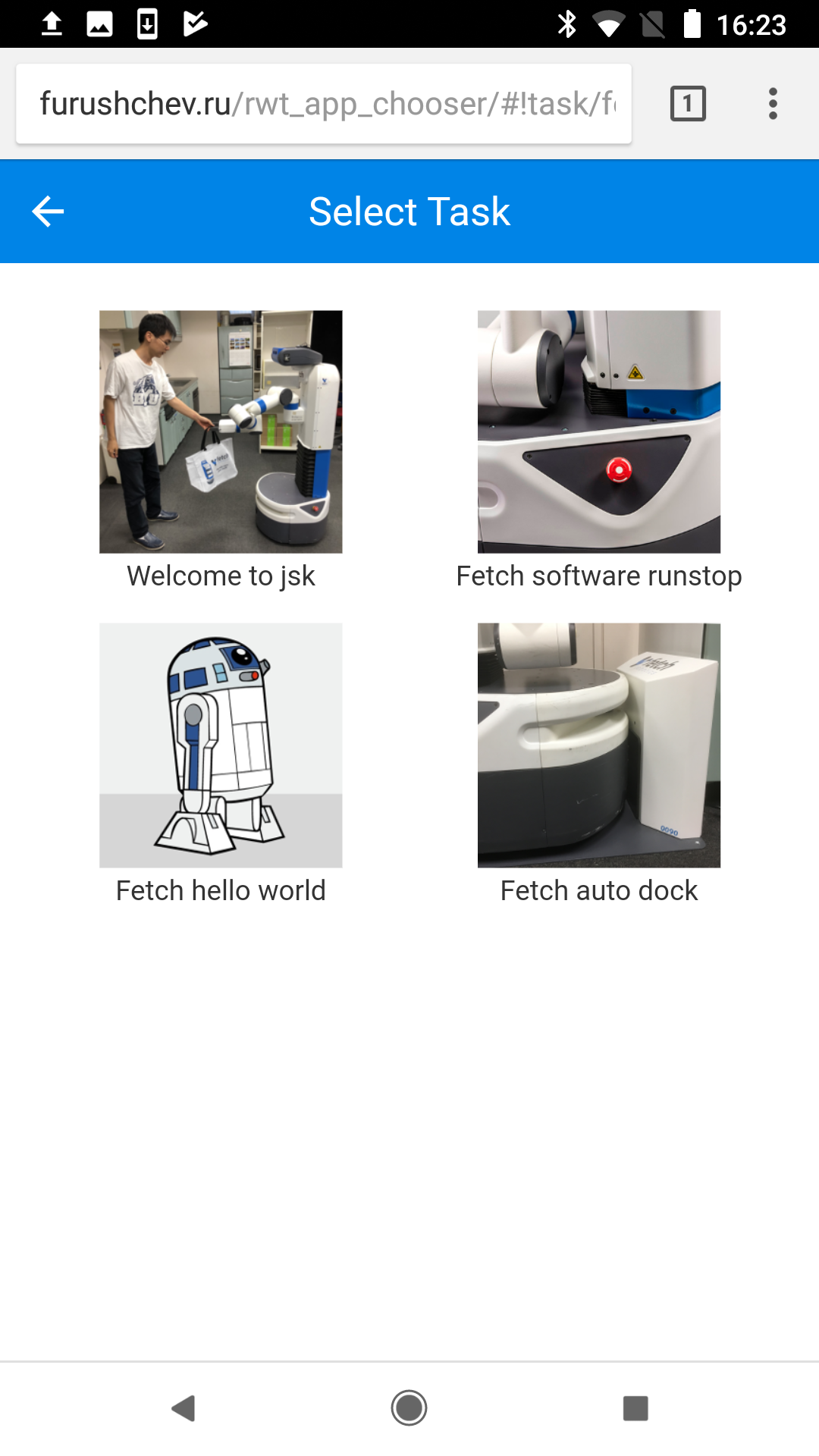
+
+## Administration
+
+- 2016/10/26 add `allow 133.11.216/8` to /etc/chrony/chrony.conf
+- 2018/08/26 add `0 10 * * 1-5 /home/fetch/ros/indigo_robot/devel/env.sh rosservice call /fetch15/start_app "name: 'jsk_fetch_startup/go_to_kitchen'"` to crontab
+ - `fetch` goes to 73B2 kitchen at 10:00 AM from Monday to Friday.
+- 2019/04/19: add `fetch` user in `pulse-access` group.
+- 2019/04/19: set `start on runlevel [2345]` in `/etc/init/pulseaudio.conf`.
+ - this modification is needed for starting `pulseaudio` in boot.
+ - `pulseaudio` is required to register USB speaker on head in boot.
+- 2019/04/19: set `env DISALLOW_MODULE_LOADING=0` in `/etc/init/pulseaudio.conf`.
+ - this modification is needed for overriding default speaker setting in `/etc/init/jsk-fetch-startup.conf`
+ - overriding default speaker setting to use USB speaker on head is done with `pactl set-default-sink $AUDIO_DEVICE` in `/etc/init/jsk-fetch-startup.conf`
+- 2019/04/19: launch `jsk_fetch_startup/fetch_bringup.launch` by `fetch` user in `/etc/init/jsk-fetch-startup.conf`
+ - some nodes save files by `fetch` user
+- 2019/04/19: add arg `launch_teleop` in `/etc/ros/indigo/robot.launch`.
+ - We sent PR to upstream [fetchrobotics/fetch_robots PR#40](https://github.com/fetchrobotics/fetch_robots/pull/40).
+- 2019/04/19: run `/etc/ros/indigo/robot.launch` with `arg` `launch_teleop:=false`.
+ - `teleop` in `/etc/ros/indigo/robot.launch` nodes were conflicted with `teleop` nodes in [jsk_fetch_startup/launch/fetch_teleop.xml](https://github.com/jsk-ros-pkg/jsk_robot/blob/master/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_teleop.xml)
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/auto_dock/auto_dock.app b/jsk_fetch_robot/jsk_fetch_startup/apps/auto_dock/auto_dock.app
index d628e8e57a..fd09baa3eb 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/auto_dock/auto_dock.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/auto_dock/auto_dock.app
@@ -1,5 +1,5 @@
display: Fetch auto dock
platform: fetch
-launch: jsk_fetch_startup/auto_dock.xml
+run: jsk_fetch_startup/auto-dock.l
interface: jsk_fetch_startup/auto_dock.interface
icon: jsk_fetch_startup/auto_dock.png
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/call_k_okada/call_k_okada.app b/jsk_fetch_robot/jsk_fetch_startup/apps/call_k_okada/call_k_okada.app
index acfcb25162..4577ac55ae 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/call_k_okada/call_k_okada.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/call_k_okada/call_k_okada.app
@@ -1,5 +1,5 @@
display: Call k-okada
platform: fetch
-launch: jsk_fetch_startup/call_k_okada.xml
+run: jsk_fetch_startup/call-k-okada.l
interface: jsk_fetch_startup/call_k_okada.interface
icon: jsk_fetch_startup/call_k_okada.png
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/dock/dock.app b/jsk_fetch_robot/jsk_fetch_startup/apps/dock/dock.app
index 916d8fb34c..6c034bd9aa 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/dock/dock.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/dock/dock.app
@@ -1,5 +1,5 @@
display: Fetch dock
platform: fetch
-launch: jsk_fetch_startup/dock.xml
+run: jsk_fetch_startup/dock.l
interface: jsk_fetch_startup/dock.interface
icon: jsk_fetch_startup/dock.png
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/fetch_apps.installed b/jsk_fetch_robot/jsk_fetch_startup/apps/fetch_apps.installed
index e47207b282..c1bad9ee46 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/fetch_apps.installed
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/fetch_apps.installed
@@ -29,3 +29,9 @@ apps:
display: light on
- app: jsk_fetch_startup/light_off
display: light off
+ - app: jsk_fetch_startup/upload_notification
+ display: Upload notification
+ - app: jsk_fetch_startup/plug_spot_power_connector
+ display: Plug Spot Power Connector
+ - app: jsk_fetch_startup/unplug_spot_power_connector
+ display: Unplug Spot Power Connector
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.app b/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.app
index e81df30945..48e797cb66 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.app
@@ -3,53 +3,18 @@ platform: fetch
launch: jsk_fetch_startup/go_to_kitchen.xml
interface: jsk_fetch_startup/go_to_kitchen.interface
icon: jsk_fetch_startup/go_to_kitchen.png
+timeout: 1200
plugins:
- name: service_notification_saver_plugin
type: app_notification_saver/service_notification_saver
- name: smach_notification_saver_plugin
type: app_notification_saver/smach_notification_saver
- - name: head_camera_video_recorder_plugin
- type: app_recorder/audio_video_recorder_plugin
- launch_args:
- video_path: /tmp
- video_title: go_to_kitchen_head_camera.avi
- audio_topic_name: /audio
- audio_channels: 1
- audio_sample_rate: 16000
- audio_format: wave
- audio_sample_format: S16LE
- video_topic_name: /head_camera/rgb/image_rect_color
- video_height: 480
- video_width: 640
- video_framerate: 30
- video_encoding: RGB
- - name: object_detection_video_recorder_plugin
- type: app_recorder/video_recorder_plugin
- launch_args:
- video_path: /tmp
- video_title: go_to_kitchen_object_detection.avi
- video_topic_name: /edgetpu_object_detector_visualization/output
- video_fps: 5.0
- - name: panorama_video_recorder_plugin
- type: app_recorder/video_recorder_plugin
- launch_args:
- video_path: /tmp
- video_title: go_to_kitchen_panorama.avi
- video_topic_name: /dual_fisheye_to_panorama/output
- video_fps: 1.0
- - name: respeaker_audio_recorder_plugin
- type: app_recorder/audio_recorder_plugin
- launch_args:
- audio_path: /tmp
- audio_title: go_to_kitchen_audio.wav
- audio_topic_name: /audio
- audio_format: wave
- name: rosbag_recorder_plugin
type: app_recorder/rosbag_recorder_plugin
launch_args:
rosbag_path: /tmp
rosbag_title: go_to_kitchen_rosbag.bag
- compress: true
+ compress: false
rosbag_topic_names:
- /rosout
- /tf
@@ -70,6 +35,8 @@ plugins:
- /move_base/global_costmap/costmap
- /particlecloud
- /base_scan/throttled
+ - /dual_fisheye_to_panorama/quater/output/compressed
+ - /edgetpu_object_detector/output/image/compressed
- /head_camera/rgb/throttled/camera_info
- /head_camera/depth_registered/throttled/camera_info
- /head_camera/rgb/throttled/image_rect_color/compressed
@@ -80,6 +47,53 @@ plugins:
- /server_name/smach/container_structure
- /audio
- /rviz/throttled/image/compressed
+ - name: head_camera_converter_plugin
+ type: app_recorder/rosbag_video_converter_plugin
+ plugin_args:
+ rosbag_path: /tmp
+ rosbag_title: go_to_kitchen_rosbag.bag
+ image_topic_name: /head_camera/rgb/throttled/image_rect_color/compressed
+ image_fps: 5
+ audio_topic_name: /audio
+ audio_sample_rate: 16000
+ audio_channels: 1
+ video_path: /tmp/go_to_kitchen_head_camera.mp4
+ - name: object_detection_converter_plugin
+ type: app_recorder/rosbag_video_converter_plugin
+ plugin_args:
+ rosbag_path: /tmp
+ rosbag_title: go_to_kitchen_rosbag.bag
+ image_topic_name: /edgetpu_object_detector/output/image/compressed
+ image_fps: 5
+ audio_topic_name: /audio
+ audio_sample_rate: 16000
+ audio_channels: 1
+ video_path: /tmp/go_to_kitchen_object_detection.mp4
+ - name: panorama_converter_plugin
+ type: app_recorder/rosbag_video_converter_plugin
+ plugin_args:
+ rosbag_path: /tmp
+ rosbag_title: go_to_kitchen_rosbag.bag
+ image_topic_name: /dual_fisheye_to_panorama/quater/output/compressed
+ image_fps: 1
+ video_path: /tmp/go_to_kitchen_panorama.mp4
+ - name: rviz_converter_plugin
+ type: app_recorder/rosbag_video_converter_plugin
+ plugin_args:
+ rosbag_path: /tmp
+ rosbag_title: go_to_kitchen_rosbag.bag
+ image_topic_name: /rviz/throttled/image/compressed
+ image_fps: 5
+ video_path: /tmp/go_to_kitchen_rviz.mp4
+ - name: respeaker_audio_converter_plugin
+ type: app_recorder/rosbag_audio_converter_plugin
+ plugin_args:
+ rosbag_path: /tmp
+ rosbag_title: go_to_kitchen_rosbag.bag
+ audio_topic_name: /audio
+ audio_sample_rate: 16000
+ audio_channels: 1
+ audio_path: /tmp/go_to_kitchen_audio.wav
- name: result_recorder_plugin
type: app_recorder/result_recorder_plugin
plugin_args:
@@ -90,20 +104,31 @@ plugins:
plugin_args:
upload_file_paths:
- /tmp/go_to_kitchen_result.yaml
- - /tmp/go_to_kitchen_head_camera.avi
- - /tmp/go_to_kitchen_object_detection.avi
- - /tmp/go_to_kitchen_panorama.avi
+ - /tmp/go_to_kitchen_head_camera.mp4
+ - /tmp/go_to_kitchen_object_detection.mp4
+ - /tmp/go_to_kitchen_panorama.mp4
+ - /tmp/go_to_kitchen_rviz.mp4
- /tmp/go_to_kitchen_audio.wav
- /tmp/go_to_kitchen_rosbag.bag
+ - /tmp/trashcan_inside.jpg
upload_file_titles:
- go_to_kitchen_result.yaml
- - go_to_kitchen_head_camera.avi
- - go_to_kitchen_object_detection.avi
- - go_to_kitchen_panorama.avi
+ - go_to_kitchen_head_camera.mp4
+ - go_to_kitchen_object_detection.mp4
+ - go_to_kitchen_panorama.mp4
+ - go_to_kitchen_rviz.mp4
- go_to_kitchen_audio.wav
- go_to_kitchen_rosbag.bag
+ - trashcan_inside.jpg
upload_parents_path: fetch_go_to_kitchen
upload_server_name: /gdrive_server
+ - name: tweet_notifier_plugin
+ type: app_notifier/tweet_notifier_plugin
+ plugin_args:
+ client_name: /tweet_image_server/tweet
+ image: true
+ image_topic_name: /edgetpu_object_detector/output/image
+ warning: false
- name: speech_notifier_plugin
type: app_notifier/speech_notifier_plugin
plugin_args:
@@ -136,13 +161,15 @@ plugin_order:
- move_base_cancel_plugin
- service_notification_saver_plugin
- smach_notification_saver_plugin
- - head_camera_video_recorder_plugin
- - object_detection_video_recorder_plugin
- - panorama_video_recorder_plugin
- - respeaker_audio_recorder_plugin
- rosbag_recorder_plugin
+ - head_camera_converter_plugin
+ - object_detection_converter_plugin
+ - panorama_converter_plugin
+ - rviz_converter_plugin
+ - respeaker_audio_converter_plugin
- result_recorder_plugin
- gdrive_uploader_plugin
+ - tweet_notifier_plugin
- speech_notifier_plugin
- mail_notifier_plugin
- shutdown_plugin
@@ -150,13 +177,15 @@ plugin_order:
- move_base_cancel_plugin
- service_notification_saver_plugin
- smach_notification_saver_plugin
- - head_camera_video_recorder_plugin
- - object_detection_video_recorder_plugin
- - panorama_video_recorder_plugin
- - respeaker_audio_recorder_plugin
- rosbag_recorder_plugin
+ - head_camera_converter_plugin
+ - object_detection_converter_plugin
+ - panorama_converter_plugin
+ - rviz_converter_plugin
+ - respeaker_audio_converter_plugin
- result_recorder_plugin
- gdrive_uploader_plugin
+ - tweet_notifier_plugin
- speech_notifier_plugin
- mail_notifier_plugin
- shutdown_plugin
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.xml b/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.xml
index 4cba12ab53..33dcfe3d80 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.xml
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/go_to_kitchen/go_to_kitchen.xml
@@ -1,8 +1,22 @@
-
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/hello_world/hello_world.app b/jsk_fetch_robot/jsk_fetch_startup/apps/hello_world/hello_world.app
index 7b9b90ea81..a2dc73bd13 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/hello_world/hello_world.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/hello_world/hello_world.app
@@ -1,5 +1,5 @@
display: Fetch hello world
platform: fetch
-launch: jsk_fetch_startup/hello_world.xml
+run: jsk_robot_startup/boot_sound.py
interface: jsk_fetch_startup/hello_world.interface
icon: jsk_fetch_startup/hello_world.png
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/light_off/light_off.app b/jsk_fetch_robot/jsk_fetch_startup/apps/light_off/light_off.app
index daf7f86708..f8aeb69c3c 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/light_off/light_off.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/light_off/light_off.app
@@ -1,6 +1,6 @@
display: Fetch light off
platform: fetch
-launch: jsk_fetch_startup/light_off.xml
+run: jsk_fetch_startup/light-off.l
interface: jsk_fetch_startup/light_off.interface
icon: jsk_fetch_startup/light_off.png
plugins:
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/light_on/light_on.app b/jsk_fetch_robot/jsk_fetch_startup/apps/light_on/light_on.app
index ef63123502..eb66297f74 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/light_on/light_on.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/light_on/light_on.app
@@ -1,6 +1,6 @@
display: Fetch light on
platform: fetch
-launch: jsk_fetch_startup/light_on.xml
+run: jsk_fetch_startup/light-on.l
interface: jsk_fetch_startup/light_on.interface
icon: jsk_fetch_startup/light_on.png
plugins:
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.app b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.app
new file mode 100644
index 0000000000..10982ad16c
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.app
@@ -0,0 +1,75 @@
+display: Plug Spot Power Connector
+platform: fetch
+launch: jsk_fetch_startup/plug_spot_power_connector.xml
+interface: jsk_fetch_startup/plug_spot_power_connector.interface
+icon: jsk_fetch_startup/plug_spot_power_connector.png
+plugins:
+ - name: service_notification_saver_plugin
+ type: app_notification_saver/service_notification_saver
+ - name: head_camera_video_recorder_plugin
+ type: app_recorder/audio_video_recorder_plugin
+ launch_args:
+ video_path: /tmp
+ video_title: plug_spot_power_connector_head_camera.avi
+ audio_topic_name: /audio
+ audio_channels: 1
+ audio_sample_rate: 16000
+ audio_format: wave
+ audio_sample_format: S16LE
+ video_topic_name: /l515_head/color/image_raw
+ video_height: 1080
+ video_width: 1920
+ video_framerate: 30
+ video_encoding: RGB
+ - name: panorama_video_recorder_plugin
+ type: app_recorder/video_recorder_plugin
+ launch_args:
+ video_path: /tmp
+ video_title: plug_spot_power_connector_panorama.avi
+ video_topic_name: /dual_fisheye_to_panorama/output
+ video_fps: 1.0
+ - name: result_recorder_plugin
+ type: app_recorder/result_recorder_plugin
+ plugin_args:
+ result_path: /tmp
+ result_title: plug_spot_power_connector_result.yaml
+ - name: gdrive_uploader_plugin
+ type: app_uploader/gdrive_uploader_plugin
+ plugin_args:
+ upload_file_paths:
+ - /tmp/plug_spot_power_connector_result.yaml
+ - /tmp/plug_spot_power_connector_head_camera.avi
+ - /tmp/plug_spot_power_connector_panorama.avi
+ upload_file_titles:
+ - plug_spot_power_connector_result.yaml
+ - plug_spot_power_connector_head_camera.avi
+ - plug_spot_power_connector_panorama.avi
+ upload_parents_path: fetch_plug_spot_power_connector
+ upload_server_name: /gdrive_server
+ - name: speech_notifier_plugin
+ type: app_notifier/speech_notifier_plugin
+ plugin_args:
+ client_name: /sound_play
+ - name: mail_notifier_plugin
+ type: app_notifier/mail_notifier_plugin
+ plugin_args:
+ mail_title: Fetch Plug Spot Power Connector Demo
+ use_timestamp_title: true
+ plugin_arg_yaml: /var/lib/robot/fetch_mail_notifier_plugin.yaml
+plugin_order:
+ start_plugin_order:
+ - service_notification_saver_plugin
+ - head_camera_video_recorder_plugin
+ - panorama_video_recorder_plugin
+ - result_recorder_plugin
+ - gdrive_uploader_plugin
+ - speech_notifier_plugin
+ - mail_notifier_plugin
+ stop_plugin_order:
+ - service_notification_saver_plugin
+ - head_camera_video_recorder_plugin
+ - panorama_video_recorder_plugin
+ - result_recorder_plugin
+ - gdrive_uploader_plugin
+ - speech_notifier_plugin
+ - mail_notifier_plugin
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.interface b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.interface
new file mode 100644
index 0000000000..044105d644
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.interface
@@ -0,0 +1,2 @@
+published_topics: {}
+subscribed_topics: {}
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.png b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.png
new file mode 100644
index 0000000000..d1b1329d2f
Binary files /dev/null and b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.png differ
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.xml b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.xml
new file mode 100644
index 0000000000..ad05e91977
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/plug_spot_power_connector/plug_spot_power_connector.xml
@@ -0,0 +1,3 @@
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/software_runstop/software_runstop.app b/jsk_fetch_robot/jsk_fetch_startup/apps/software_runstop/software_runstop.app
index 6c86c77347..ed4a25c745 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/software_runstop/software_runstop.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/software_runstop/software_runstop.app
@@ -1,5 +1,6 @@
display: Fetch software runstop
platform: fetch
-launch: jsk_fetch_startup/software_runstop.xml
+run: rostopic/rostopic
+run_args: "pub -1 /enable_software_runstop std_msgs/Bool 'data: true'"
interface: jsk_fetch_startup/software_runstop.interface
icon: jsk_fetch_startup/software_runstop.png
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/speak_battery/speak_battery.app b/jsk_fetch_robot/jsk_fetch_startup/apps/speak_battery/speak_battery.app
index 0a48645098..2dc162d470 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/speak_battery/speak_battery.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/speak_battery/speak_battery.app
@@ -1,6 +1,6 @@
display: Speak battery
platform: fetch
-launch: jsk_fetch_startup/speak_battery.xml
+run: jsk_fetch_startup/speak-battery.l
interface: jsk_fetch_startup/speak_battery.interface
icon: jsk_fetch_startup/speak_battery.png
plugins:
@@ -8,6 +8,7 @@ plugins:
type: app_notifier/user_speech_notifier_plugin
plugin_args:
client_name: /sound_play
+ warning: true
plugin_order:
start_plugin_order:
- user_speech_notifier_plugin
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/time_signal/time_signal.app b/jsk_fetch_robot/jsk_fetch_startup/apps/time_signal/time_signal.app
index d1c5e17227..6137ee226b 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/time_signal/time_signal.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/time_signal/time_signal.app
@@ -1,10 +1,17 @@
display: Speak time signal
platform: fetch
-launch: jsk_fetch_startup/time_signal.xml
+run: jsk_fetch_startup/time_signal.py
interface: jsk_fetch_startup/time_signal.interface
icon: jsk_fetch_startup/time_signal.png
-timeout: 10
+timeout: 120
plugins:
+ - name: tweet_notifier_plugin
+ type: app_notifier/tweet_notifier_plugin
+ plugin_args:
+ client_name: /tweet_image_server/tweet
+ image: true
+ image_topic_name: /edgetpu_object_detector/output/image
+ warning: false
- name: user_speech_notifier_plugin
type: app_notifier/user_speech_notifier_plugin
plugin_args:
@@ -12,6 +19,8 @@ plugins:
warning: false
plugin_order:
start_plugin_order:
+ - tweet_notifier_plugin
- user_speech_notifier_plugin
stop_plugin_order:
+ - tweet_notifier_plugin
- user_speech_notifier_plugin
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/tweet/tweet.app b/jsk_fetch_robot/jsk_fetch_startup/apps/tweet/tweet.app
index da0197d1b9..28d7cc4bf1 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/tweet/tweet.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/tweet/tweet.app
@@ -1,6 +1,6 @@
display: Fetch tweet
platform: fetch
-launch: jsk_fetch_startup/tweet.xml
+run: jsk_fetch_startup/tweet.l
interface: jsk_fetch_startup/tweet.interface
icon: jsk_fetch_startup/tweet.png
plugins:
@@ -8,6 +8,7 @@ plugins:
type: app_notifier/user_speech_notifier_plugin
plugin_args:
client_name: /sound_play
+ warning: true
plugin_order:
start_plugin_order:
- user_speech_notifier_plugin
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/undock/undock.app b/jsk_fetch_robot/jsk_fetch_startup/apps/undock/undock.app
index da845f0a25..78a4b30891 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/apps/undock/undock.app
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/undock/undock.app
@@ -1,5 +1,5 @@
display: Fetch undock
platform: fetch
-launch: jsk_fetch_startup/undock.xml
+run: jsk_fetch_startup/undock.l
interface: jsk_fetch_startup/undock.interface
icon: jsk_fetch_startup/undock.png
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.app b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.app
new file mode 100644
index 0000000000..680166f454
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.app
@@ -0,0 +1,75 @@
+display: Unplug Spot Power Connector
+platform: fetch
+launch: jsk_fetch_startup/unplug_spot_power_connector.xml
+interface: jsk_fetch_startup/unplug_spot_power_connector.interface
+icon: jsk_fetch_startup/unplug_spot_power_connector.png
+plugins:
+ - name: service_notification_saver_plugin
+ type: app_notification_saver/service_notification_saver
+ - name: head_camera_video_recorder_plugin
+ type: app_recorder/audio_video_recorder_plugin
+ launch_args:
+ video_path: /tmp
+ video_title: unplug_spot_power_connector_head_camera.avi
+ audio_topic_name: /audio
+ audio_channels: 1
+ audio_sample_rate: 16000
+ audio_format: wave
+ audio_sample_format: S16LE
+ video_topic_name: /l515_head/color/image_raw
+ video_height: 1080
+ video_width: 1920
+ video_framerate: 30
+ video_encoding: RGB
+ - name: panorama_video_recorder_plugin
+ type: app_recorder/video_recorder_plugin
+ launch_args:
+ video_path: /tmp
+ video_title: unplug_spot_power_connector_panorama.avi
+ video_topic_name: /dual_fisheye_to_panorama/output
+ video_fps: 1.0
+ - name: result_recorder_plugin
+ type: app_recorder/result_recorder_plugin
+ plugin_args:
+ result_path: /tmp
+ result_title: unplug_spot_power_connector_result.yaml
+ - name: gdrive_uploader_plugin
+ type: app_uploader/gdrive_uploader_plugin
+ plugin_args:
+ upload_file_paths:
+ - /tmp/unplug_spot_power_connector_result.yaml
+ - /tmp/unplug_spot_power_connector_head_camera.avi
+ - /tmp/unplug_spot_power_connector_panorama.avi
+ upload_file_titles:
+ - unplug_spot_power_connector_result.yaml
+ - unplug_spot_power_connector_head_camera.avi
+ - unplug_spot_power_connector_panorama.avi
+ upload_parents_path: fetch_unplug_spot_power_connector
+ upload_server_name: /gdrive_server
+ - name: speech_notifier_plugin
+ type: app_notifier/speech_notifier_plugin
+ plugin_args:
+ client_name: /sound_play
+ - name: mail_notifier_plugin
+ type: app_notifier/mail_notifier_plugin
+ plugin_args:
+ mail_title: Fetch Unplug Spot Power Connector Demo
+ use_timestamp_title: true
+ plugin_arg_yaml: /var/lib/robot/fetch_mail_notifier_plugin.yaml
+plugin_order:
+ start_plugin_order:
+ - service_notification_saver_plugin
+ - head_camera_video_recorder_plugin
+ - panorama_video_recorder_plugin
+ - result_recorder_plugin
+ - gdrive_uploader_plugin
+ - speech_notifier_plugin
+ - mail_notifier_plugin
+ stop_plugin_order:
+ - service_notification_saver_plugin
+ - head_camera_video_recorder_plugin
+ - panorama_video_recorder_plugin
+ - result_recorder_plugin
+ - gdrive_uploader_plugin
+ - speech_notifier_plugin
+ - mail_notifier_plugin
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.interface b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.interface
new file mode 100644
index 0000000000..044105d644
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.interface
@@ -0,0 +1,2 @@
+published_topics: {}
+subscribed_topics: {}
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.png b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.png
new file mode 100644
index 0000000000..3098183bc3
Binary files /dev/null and b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.png differ
diff --git a/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.xml b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.xml
new file mode 100644
index 0000000000..d61fc63f62
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/apps/unplug_spot_power_connector/unplug_spot_power_connector.xml
@@ -0,0 +1,3 @@
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/.gitignore b/jsk_fetch_robot/jsk_fetch_startup/config/.gitignore
new file mode 100644
index 0000000000..97ed018fbb
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/.gitignore
@@ -0,0 +1 @@
+fetch*_dialogflow_hotword.yaml
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/config.bash b/jsk_fetch_robot/jsk_fetch_startup/config/config.bash
new file mode 100644
index 0000000000..4f7057cc68
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/config.bash
@@ -0,0 +1,46 @@
+# This file is bash configuration for robot.conf
+# This file must be at /var/lib/robot/config.bash
+
+if [ $(hostname) = 'fetch15' ]; then
+ export DEFAULT_SPEAKER=13;
+ export DEFAULT_ENGLISH_SPEAKER=cmu_us_bdl.flitevox;
+ export DEFAULT_WARNING_SPEAKER=cmu_us_fem.flitevox;
+
+ export USE_BASE_CAMERA_MOUNT=true;
+ export USE_HEAD_BOX=false;
+ export USE_HEAD_L515=true;
+ export USE_INSTA360_STAND=true;
+
+ export RS_SERIAL_NO_T265="925122110450";
+ export RS_SERIAL_NO_D435_FRONTRIGHT="";
+ export RS_SERIAL_NO_D435_FRONTLEFT="";
+ # Disable L515 to reduce fetch's CPU usage
+ # Use L515 after finding a way to reduce L515 CPU usage like ConnectionBasedTransport
+ # export RS_SERIAL_NO_L515_HEAD="f0211890";
+ export RS_SERIAL_NO_L515_HEAD="";
+
+ export NETWORK_DEFAULT_WIFI_INTERFACE="wlan0";
+ export NETWORK_DEFAULT_ROS_INTERFACE="fetch15";
+ export NETWORK_DEFAULT_PROFILE_ID="sanshiro-73B2";
+elif [ $(hostname) = 'fetch1075' ]; then
+ export DEFAULT_SPEAKER=2;
+ export DEFAULT_ENGLISH_SPEAKER=cmu_us_slt.flitevox;
+ export DEFAULT_WARNING_SPEAKER=cmu_us_lnh.flitevox;
+
+ export USE_BASE_CAMERA_MOUNT=false;
+ export USE_HEAD_BOX=false;
+ export USE_HEAD_L515=true;
+ export USE_INSTA360_STAND=true;
+
+ export RS_SERIAL_NO_T265="";
+ export RS_SERIAL_NO_D435_FRONTRIGHT="";
+ export RS_SERIAL_NO_D435_FRONTLEFT="";
+ # Disable L515 to reduce fetch's CPU usage
+ # Use L515 after finding a way to reduce L515 CPU usage like ConnectionBasedTransport
+ export RS_SERIAL_NO_L515_HEAD="f0232270";
+ #export RS_SERIAL_NO_L515_HEAD="";
+
+ export NETWORK_DEFAULT_WIFI_INTERFACE="wlan0";
+ export NETWORK_DEFAULT_ROS_INTERFACE="fetch1075";
+ export NETWORK_DEFAULT_PROFILE_ID="sanshiro-73B2";
+fi
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/config_outside.bash b/jsk_fetch_robot/jsk_fetch_startup/config/config_outside.bash
new file mode 100644
index 0000000000..007560b460
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/config_outside.bash
@@ -0,0 +1,12 @@
+# This file is bash configuration for robot.conf
+# This file must be at /var/lib/robot/config.bash
+
+SCRIPT_DIR="$( cd -- "$( dirname -- "${BASH_SOURCE[0]:-$0}"; )" &> /dev/null && pwd 2> /dev/null; )";
+. $SCRIPT_DIR/config.bash
+
+if [ $(hostname) = 'fetch15' ]; then
+ export NETWORK_DEFAULT_PROFILE_ID="sanshiro-outside";
+elif [ $(hostname) = 'fetch1075' ]; then
+ export NETWORK_DEFAULT_ROS_INTERFACE="fetch1075.local";
+ export NETWORK_DEFAULT_PROFILE_ID="sanshiro-outside";
+fi
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/dialogflow_hotword.yaml.in b/jsk_fetch_robot/jsk_fetch_startup/config/dialogflow_hotword.yaml.in
new file mode 100644
index 0000000000..73714a65a9
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/dialogflow_hotword.yaml.in
@@ -0,0 +1,5 @@
+- ねえねえ
+- こんにちわ
+- やあ
+- @ROBOT_NICKNAME_KANA@
+- @ROBOT_NICKNAME_HIRA@
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/fetch_analyzers.yaml b/jsk_fetch_robot/jsk_fetch_startup/config/fetch_analyzers.yaml
index f4847a4cba..d6b18c8111 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/config/fetch_analyzers.yaml
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/fetch_analyzers.yaml
@@ -102,7 +102,7 @@ analyzers:
path: Head Camera
timeout: 5.0
startswith: 'head_camera'
- num_items: 2
+ num_items: 4
imu:
type: diagnostic_aggregator/GenericAnalyzer
path: IMU
@@ -154,18 +154,6 @@ analyzers:
contains: '/audio'
timeout: 100
discard_stale: true
- head_depth_image:
- type: diagnostic_aggregator/GenericAnalyzer
- path: HeadDepthImage
- contains: '/head_camera/depth/image_raw'
- timeout: 100
- discard_stale: true
- head_rgb_image:
- type: diagnostic_aggregator/GenericAnalyzer
- path: HeadRGBImage
- contains: '/head_camera/rgb/image_raw'
- timeout: 100
- discard_stale: true
nodes:
type: diagnostic_aggregator/AnalyzerGroup
path: Nodes
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/install_cron.sh b/jsk_fetch_robot/jsk_fetch_startup/config/install_cron.sh
new file mode 100755
index 0000000000..186b8dc4cc
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/install_cron.sh
@@ -0,0 +1,15 @@
+#!/bin/bash
+
+jsk_fetch_startup=$(builtin cd "`dirname "${BASH_SOURCE[0]}"`"/.. > /dev/null && pwd)
+
+IFS=':' read -r -a prefix_paths <<< "$CMAKE_PREFIX_PATH"
+current_prefix_path="${prefix_paths[0]}"
+
+set -x
+
+cd $jsk_fetch_startup/cron_scripts
+sudo -u fetch crontab cron_fetch.conf
+echo "Set cron jobs for fetch user"
+sudo -u root crontab cron_root.conf
+echo "Set cron jobs for root user"
+set +x
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/install_supervisor.sh b/jsk_fetch_robot/jsk_fetch_startup/config/install_supervisor.sh
index 9f77c428b9..6a35d559c0 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/config/install_supervisor.sh
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/install_supervisor.sh
@@ -8,11 +8,14 @@ current_prefix_path="${prefix_paths[0]}"
set -x
cd $jsk_fetch_startup/supervisor_scripts
-for file in $(ls ./*.conf); do
- sudo cp $file /etc/supervisor/conf.d/
- sudo chown root:root /etc/supervisor/conf.d/$file
- sudo chmod 644 /etc/supervisor/conf.d/$file
- echo "copied $file to /etc/supervisor/conf.d"
+for file in $(ls *.conf); do
+ sudo ln -sf $jsk_fetch_startup/supervisor_scripts/$file /etc/supervisor/conf.d/$file
done
+sudo supervisorctl reread
+# Enable jsk_dstat job to save the csv log under /var/log
+sudo ln -sf /home/fetch/Documents/jsk_dstat.csv /var/log/ros/jsk-dstat.csv
+
+sudo ln -sf $jsk_fetch_startup/config/config.bash /var/lib/robot/config.bash
+sudo ln -sf $jsk_fetch_startup/config/config_outside.bash /var/lib/robot/config_outside.bash
set +x
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/install_udev.sh b/jsk_fetch_robot/jsk_fetch_startup/config/install_udev.sh
index b079e114a4..5e8e1cec43 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/config/install_udev.sh
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/install_udev.sh
@@ -1,17 +1,12 @@
#!/bin/bash
+# 99-edgetpu-accelerator.rules and 99-realsense-libusb.rules are not tracked in jsk_fetch_startup
+# because they are installed by apt
+
jsk_fetch_startup=$(builtin cd "`dirname "${BASH_SOURCE[0]}"`"/.. > /dev/null && pwd)
cd $jsk_fetch_startup/udev_rules
for file in $(ls *.rules); do
- if [ -e /etc/udev/rules.d/$file ]; then
- file_bk=$file.$(date "+%Y%m%d_%H%M%S")
- sudo cp /etc/udev/rules.d/$file /etc/udev/rules.d/$file_bk
- echo "backup /etc/udev/rules.d/$file to /etc/udev/rules.d/$file_bk"
- fi
-
- sudo cp $file /etc/udev/rules.d/
- sudo chown root:root /etc/udev/rules.d/$file
- sudo chmod 644 /etc/udev/rules.d/$file
- echo -e "copied jsk_fetch_startup/udev_rules/$file to /etc/udev/rules.d/$file\n"
+ sudo ln -sf $jsk_fetch_startup/udev_rules/$file /etc/udev/rules.d/$file
+ echo -e "Create symbolic link from jsk_fetch_startup/udev_rules/$file to /etc/udev/rules.d/$file\n"
done
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/jsk_startup.rviz b/jsk_fetch_robot/jsk_fetch_startup/config/jsk_startup.rviz
index 7d5b36018d..9fa5277bc9 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/config/jsk_startup.rviz
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/jsk_startup.rviz
@@ -5,7 +5,7 @@ Panels:
Property Tree Widget:
Expanded: ~
Splitter Ratio: 0.4187380373477936
- Tree Height: 595
+ Tree Height: 477
- Class: rviz/Selection
Name: Selection
- Class: rviz/Tool Properties
@@ -27,7 +27,7 @@ Panels:
SyncSource: BaseScan
- Class: jsk_rviz_plugin/CancelAction
Name: CancelAction
- Topic: /move_base
+ Topic: ""
topics:
- /move_base
Preferences:
@@ -65,6 +65,11 @@ Visualization Manager:
Expand Link Details: false
Expand Tree: false
Link Tree Style: Links in Alphabetic Order
+ base_camera_mount:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
base_link:
Alpha: 1
Show Axes: false
@@ -80,6 +85,14 @@ Visualization Manager:
Show Axes: false
Show Trail: false
Value: true
+ d435_front_left_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ d435_front_right_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
elbow_flex_link:
Alpha: 1
Show Axes: false
@@ -100,6 +113,11 @@ Visualization Manager:
Show Axes: false
Show Trail: false
Value: true
+ head_box_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
head_camera_depth_frame:
Alpha: 1
Show Axes: false
@@ -120,6 +138,15 @@ Visualization Manager:
Alpha: 1
Show Axes: false
Show Trail: false
+ head_l515_mount_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ head_l515_virtual_mount_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
head_pan_link:
Alpha: 1
Show Axes: false
@@ -130,6 +157,14 @@ Visualization Manager:
Show Axes: false
Show Trail: false
Value: true
+ insta360_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ l515_head_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
l_gripper_finger_link:
Alpha: 1
Show Axes: false
@@ -229,7 +264,7 @@ Visualization Manager:
Head Length: 0.20000000298023224
Length: 0.30000001192092896
Line Style: Billboards
- Line Width: 0.3
+ Line Width: 0.30000001192092896
Name: NavigationPath
Offset:
X: 0
@@ -252,7 +287,7 @@ Visualization Manager:
Head Length: 0.20000000298023224
Length: 0.30000001192092896
Line Style: Billboards
- Line Width: 0.1
+ Line Width: 0.10000000149011612
Name: GlobalPath
Offset:
X: 0
@@ -317,12 +352,12 @@ Visualization Manager:
Class: rviz/Map
Color Scheme: costmap
Draw Behind: false
- Enabled: false
+ Enabled: true
Name: SafeCostMap
Topic: /safe_teleop_base/local_costmap/costmap
Unreliable: false
Use Timestamp: false
- Value: false
+ Value: true
- Alpha: 0.3499999940395355
Class: rviz/Map
Color Scheme: costmap
@@ -374,9 +409,7 @@ Visualization Manager:
Enabled: true
Invert Rainbow: false
Max Color: 255; 255; 255
- Max Intensity: 4096
Min Color: 0; 0; 0
- Min Intensity: 0
Name: BaseScan
Position Transformer: XYZ
Queue Size: 10
@@ -404,9 +437,7 @@ Visualization Manager:
Enabled: true
Invert Rainbow: false
Max Color: 255; 255; 255
- Max Intensity: 4096
Min Color: 0; 0; 0
- Min Intensity: 0
Name: XtionPointCloud
Position Transformer: XYZ
Queue Size: 10
@@ -419,19 +450,120 @@ Visualization Manager:
Use Fixed Frame: true
Use rainbow: true
Value: true
- - Class: jsk_rviz_plugin/OverlayImage
+ - Alpha: 1
+ Autocompute Intensity Bounds: true
+ Autocompute Value Bounds:
+ Max Value: 10
+ Min Value: -10
+ Value: true
+ Axis: Z
+ Channel Name: intensity
+ Class: rviz/PointCloud2
+ Color: 255; 255; 255
+ Color Transformer: RGB8
+ Decay Time: 0
+ Enabled: false
+ Invert Rainbow: false
+ Max Color: 255; 255; 255
+ Min Color: 0; 0; 0
+ Name: HeadL515PointCloud
+ Position Transformer: XYZ
+ Queue Size: 10
+ Selectable: true
+ Size (Pixels): 3
+ Size (m): 0.009999999776482582
+ Style: Flat Squares
+ Topic: /l515_head/depth/color/points
+ Unreliable: false
+ Use Fixed Frame: true
+ Use rainbow: true
+ Value: false
+ - Class: rviz/Group
+ Displays:
+ - Class: jsk_rviz_plugin/OverlayImage
+ Enabled: true
+ Name: XtionRGB
+ Topic: /head_camera/rgb/quater/image_rect_color
+ Value: true
+ alpha: 0.800000011920929
+ height: 128
+ keep aspect ratio: true
+ left: 10
+ overwrite alpha value: false
+ top: 10
+ transport hint: raw
+ width: 320
+ - Class: jsk_rviz_plugin/OverlayImage
+ Enabled: false
+ Name: XtionRGBHumanPoseEstimator
+ Topic: /edgetpu_human_pose_estimator/output/image
+ Value: false
+ alpha: 0.800000011920929
+ height: 128
+ keep aspect ratio: true
+ left: 10
+ overwrite alpha value: false
+ top: 10
+ transport hint: raw
+ width: 320
+ - Class: jsk_rviz_plugin/OverlayImage
+ Enabled: false
+ Name: XtionRGBObjectDetector
+ Topic: /edgetpu_human_pose_estimator/output/image
+ Value: false
+ alpha: 0.800000011920929
+ height: 128
+ keep aspect ratio: true
+ left: 10
+ overwrite alpha value: false
+ top: 10
+ transport hint: raw
+ width: 320
Enabled: true
Name: XtionRGB
- Topic: /head_camera/rgb/quater/image_rect_color
- Value: true
- alpha: 0.800000011920929
- height: 128
- keep aspect ratio: true
- left: 10
- overwrite alpha value: false
- top: 10
- transport hint: raw
- width: 320
+ - Class: rviz/Group
+ Displays:
+ - Class: jsk_rviz_plugin/OverlayImage
+ Enabled: true
+ Name: PanoramaImage
+ Topic: /dual_fisheye_to_panorama/quater/output
+ Value: true
+ alpha: 0.800000011920929
+ height: 128
+ keep aspect ratio: true
+ left: 340
+ overwrite alpha value: false
+ top: 10
+ transport hint: raw
+ width: 480
+ - Class: jsk_rviz_plugin/OverlayImage
+ Enabled: false
+ Name: PanoramaHumanPoseEstimator
+ Topic: /edgetpu_panorama_human_pose_estimator/output/image
+ Value: false
+ alpha: 0.800000011920929
+ height: 128
+ keep aspect ratio: true
+ left: 340
+ overwrite alpha value: false
+ top: 10
+ transport hint: raw
+ width: 480
+ - Class: jsk_rviz_plugin/OverlayImage
+ Enabled: false
+ Name: PanoramaObjectDetector
+ Topic: /edgetpu_panorama_object_detector/output/image
+ Value: false
+ alpha: 0.800000011920929
+ height: 128
+ keep aspect ratio: true
+ left: 340
+ overwrite alpha value: false
+ top: 10
+ transport hint: raw
+ width: 480
+ Enabled: true
+ Name: PanoramaImage
Enabled: true
Global Options:
Background Color: 48; 48; 48
@@ -472,23 +604,25 @@ Visualization Manager:
X: 0
Y: 0
Z: 0
- Focal Shape Fixed Size: true
+ Focal Shape Fixed Size: false
Focal Shape Size: 0.05000000074505806
Invert Z Axis: false
Name: Current View
Near Clip Distance: 0.009999999776482582
- Pitch: 0.7897970676422119
+ Pitch: 0.7853981852531433
Target Frame: base_link
Value: Orbit (rviz)
- Yaw: 5.593582630157471
+ Yaw: 0.7853981852531433
Saved: ~
Window Geometry:
+ CancelAction:
+ collapsed: false
Displays:
collapsed: false
- Height: 1052
+ Height: 1025
Hide Left Dock: false
Hide Right Dock: true
- QMainWindow State: 000000ff00000000fd0000000400000000000001f80000037efc020000000afb0000001200530065006c0065006300740069006f006e00000001e10000009b0000005c00fffffffb0000001e0054006f006f006c002000500072006f0070006500720074006900650073020000019b000001df00000185000000a3fb000000120056006900650077007300200054006f006f02000001df000002110000018500000122fb000000200054006f006f006c002000500072006f0070006500720074006900650073003203000002880000011d000002210000017afb000000100044006900730070006c006100790073010000003d0000037e000000c900fffffffb00000018004b0069006e00650063007400430061006d0065007200610000000373000000160000000000000000fb0000002000730065006c0065006300740069006f006e00200062007500660066006500720200000138000000aa0000023a00000294fb00000014005700690064006500530074006500720065006f02000000e6000000d2000003ee0000030bfb0000000c004b0069006e0065006300740200000186000001060000030c00000261fb00000018004e006100720072006f007700430061006d0065007200610000000244000001460000000000000000000000010000010f00000463fc0200000003fb0000001e0054006f006f006c002000500072006f00700065007200740069006500730100000041000000780000000000000000fb0000000a00560069006500770073000000002800000463000000a400fffffffb0000001200530065006c0065006300740069006f006e010000025a000000b200000000000000000000000200000780000000a9fc0100000001fb0000000a00560069006500770073030000004e00000080000002e100000197000000030000073d0000003efc0100000002fb0000000800540069006d006501000000000000073d000002eb00fffffffb0000000800540069006d006501000000000000045000000000000000000000053f0000037e00000004000000040000000800000008fc0000000100000002000000010000000a0054006f006f006c00730100000000ffffffff0000000000000000
+ QMainWindow State: 000000ff00000000fd0000000400000000000001f800000361fc020000000bfb0000001200530065006c0065006300740069006f006e00000001e10000009b0000005c00fffffffb0000001e0054006f006f006c002000500072006f0070006500720074006900650073020000019b000001df00000185000000a3fb000000120056006900650077007300200054006f006f02000001df000002110000018500000122fb000000200054006f006f006c002000500072006f0070006500720074006900650073003203000002880000011d000002210000017afb000000100044006900730070006c006100790073010000003e00000269000000ca00fffffffb00000018004b0069006e00650063007400430061006d0065007200610000000373000000160000000000000000fb0000002000730065006c0065006300740069006f006e00200062007500660066006500720200000138000000aa0000023a00000294fb00000014005700690064006500530074006500720065006f02000000e6000000d2000003ee0000030bfb0000000c004b0069006e0065006300740200000186000001060000030c00000261fb00000018004e006100720072006f007700430061006d0065007200610000000244000001460000000000000000fb0000001800430061006e00630065006c0041006300740069006f006e01000002ad000000f20000008200ffffff000000010000010f00000463fc0200000003fb0000001e0054006f006f006c002000500072006f00700065007200740069006500730100000041000000780000000000000000fb0000000a00560069006500770073000000002800000463000000a600fffffffb0000001200530065006c0065006300740069006f006e010000025a000000b200000000000000000000000200000780000000a9fc0100000001fb0000000a00560069006500770073030000004e00000080000002e10000019700000003000007800000003efc0100000002fb0000000800540069006d00650100000000000007800000026200fffffffb0000000800540069006d00650100000000000004500000000000000000000005820000036100000004000000040000000800000008fc0000000100000002000000010000000a0054006f006f006c00730100000000ffffffff0000000000000000
Selection:
collapsed: false
Time:
@@ -497,6 +631,6 @@ Window Geometry:
collapsed: false
Views:
collapsed: true
- Width: 1853
- X: 67
+ Width: 1920
+ X: 0
Y: 27
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/sanity_targets.yaml b/jsk_fetch_robot/jsk_fetch_startup/config/sanity_targets.yaml
index 6b8ce4ff93..24a3521055 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/config/sanity_targets.yaml
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/sanity_targets.yaml
@@ -2,8 +2,6 @@ topics:
- /audio
- /edgetpu_human_pose_estimator/output/image/compressed
- /edgetpu_object_detector/output/image/compressed
- - /head_camera/depth/image_raw
- - /head_camera/rgb/image_raw
nodes:
- /head_camera/head_camera_nodelet_manager
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/view_model.rviz b/jsk_fetch_robot/jsk_fetch_startup/config/view_model.rviz
new file mode 100644
index 0000000000..e8978465a4
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/view_model.rviz
@@ -0,0 +1,426 @@
+Panels:
+ - Class: rviz/Displays
+ Help Height: 78
+ Name: Displays
+ Property Tree Widget:
+ Expanded: ~
+ Splitter Ratio: 0.5
+ Tree Height: 728
+ - Class: rviz/Selection
+ Name: Selection
+ - Class: rviz/Tool Properties
+ Expanded:
+ - /2D Pose Estimate1
+ - /2D Nav Goal1
+ - /Publish Point1
+ Name: Tool Properties
+ Splitter Ratio: 0.5886790156364441
+ - Class: rviz/Views
+ Expanded:
+ - /Current View1
+ Name: Views
+ Splitter Ratio: 0.5
+ - Class: rviz/Time
+ Experimental: false
+ Name: Time
+ SyncMode: 0
+ SyncSource: ""
+Preferences:
+ PromptSaveOnExit: true
+Toolbars:
+ toolButtonStyle: 2
+Visualization Manager:
+ Class: ""
+ Displays:
+ - Alpha: 0.5
+ Cell Size: 1
+ Class: rviz/Grid
+ Color: 160; 160; 164
+ Enabled: true
+ Line Style:
+ Line Width: 0.029999999329447746
+ Value: Lines
+ Name: Grid
+ Normal Cell Count: 0
+ Offset:
+ X: 0
+ Y: 0
+ Z: 0
+ Plane: XY
+ Plane Cell Count: 10
+ Reference Frame:
+ Value: true
+ - Alpha: 0.5
+ Class: rviz/RobotModel
+ Collision Enabled: false
+ Enabled: true
+ Links:
+ All Links Enabled: true
+ Expand Joint Details: false
+ Expand Link Details: false
+ Expand Tree: false
+ Link Tree Style: Links in Alphabetic Order
+ base_camera_mount:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ base_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ bellows_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ bellows_link2:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ d435_front_left_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ d435_front_right_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ elbow_flex_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ estop_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ forearm_roll_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ gripper_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ head_box_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ head_camera_depth_frame:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ head_camera_depth_optical_frame:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ head_camera_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ head_camera_rgb_frame:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ head_camera_rgb_optical_frame:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ head_l515_mount_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ head_l515_virtual_mount_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ head_pan_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ head_tilt_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ insta360_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ l515_head_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ l_gripper_finger_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ l_wheel_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ laser_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ r_gripper_finger_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ r_wheel_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ shoulder_lift_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ shoulder_pan_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ torso_fixed_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ torso_lift_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ upperarm_roll_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ wrist_flex_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ wrist_roll_link:
+ Alpha: 1
+ Show Axes: false
+ Show Trail: false
+ Value: true
+ Name: RobotModel
+ Robot Description: robot_description
+ TF Prefix: ""
+ Update Interval: 0
+ Value: true
+ Visual Enabled: true
+ - Class: rviz/TF
+ Enabled: true
+ Frame Timeout: 15
+ Frames:
+ All Enabled: true
+ base_camera_mount:
+ Value: true
+ base_link:
+ Value: true
+ bellows_link:
+ Value: true
+ bellows_link2:
+ Value: true
+ d435_front_left_link:
+ Value: true
+ d435_front_right_link:
+ Value: true
+ elbow_flex_link:
+ Value: true
+ estop_link:
+ Value: true
+ forearm_roll_link:
+ Value: true
+ gripper_link:
+ Value: true
+ head_box_link:
+ Value: true
+ head_camera_depth_frame:
+ Value: true
+ head_camera_depth_optical_frame:
+ Value: true
+ head_camera_link:
+ Value: true
+ head_camera_rgb_frame:
+ Value: true
+ head_camera_rgb_optical_frame:
+ Value: true
+ head_l515_mount_link:
+ Value: true
+ head_l515_virtual_mount_link:
+ Value: true
+ head_pan_link:
+ Value: true
+ head_tilt_link:
+ Value: true
+ insta360_link:
+ Value: true
+ l515_head_link:
+ Value: true
+ l_gripper_finger_link:
+ Value: true
+ l_wheel_link:
+ Value: true
+ laser_link:
+ Value: true
+ r_gripper_finger_link:
+ Value: true
+ r_wheel_link:
+ Value: true
+ shoulder_lift_link:
+ Value: true
+ shoulder_pan_link:
+ Value: true
+ torso_fixed_link:
+ Value: true
+ torso_lift_link:
+ Value: true
+ upperarm_roll_link:
+ Value: true
+ wrist_flex_link:
+ Value: true
+ wrist_roll_link:
+ Value: true
+ Marker Scale: 0.5
+ Name: TF
+ Show Arrows: true
+ Show Axes: true
+ Show Names: true
+ Tree:
+ base_link:
+ base_camera_mount:
+ {}
+ d435_front_left_link:
+ {}
+ d435_front_right_link:
+ {}
+ estop_link:
+ {}
+ l_wheel_link:
+ {}
+ laser_link:
+ {}
+ r_wheel_link:
+ {}
+ torso_fixed_link:
+ {}
+ torso_lift_link:
+ bellows_link:
+ {}
+ bellows_link2:
+ {}
+ head_pan_link:
+ head_box_link:
+ {}
+ head_tilt_link:
+ head_camera_link:
+ head_camera_depth_frame:
+ head_camera_depth_optical_frame:
+ {}
+ head_camera_rgb_frame:
+ head_camera_rgb_optical_frame:
+ {}
+ head_l515_virtual_mount_link:
+ head_l515_mount_link:
+ l515_head_link:
+ {}
+ insta360_link:
+ {}
+ shoulder_pan_link:
+ shoulder_lift_link:
+ upperarm_roll_link:
+ elbow_flex_link:
+ forearm_roll_link:
+ wrist_flex_link:
+ wrist_roll_link:
+ gripper_link:
+ l_gripper_finger_link:
+ {}
+ r_gripper_finger_link:
+ {}
+ Update Interval: 0
+ Value: true
+ Enabled: true
+ Global Options:
+ Background Color: 48; 48; 48
+ Default Light: true
+ Fixed Frame: base_link
+ Frame Rate: 30
+ Name: root
+ Tools:
+ - Class: rviz/Interact
+ Hide Inactive Objects: true
+ - Class: rviz/MoveCamera
+ - Class: rviz/Select
+ - Class: rviz/FocusCamera
+ - Class: rviz/Measure
+ - Class: rviz/SetInitialPose
+ Theta std deviation: 0.2617993950843811
+ Topic: /initialpose
+ X std deviation: 0.5
+ Y std deviation: 0.5
+ - Class: rviz/SetGoal
+ Topic: /move_base_simple/goal
+ - Class: rviz/PublishPoint
+ Single click: true
+ Topic: /clicked_point
+ Value: true
+ Views:
+ Current:
+ Class: rviz/Orbit
+ Distance: 4.618305206298828
+ Enable Stereo Rendering:
+ Stereo Eye Separation: 0.05999999865889549
+ Stereo Focal Distance: 1
+ Swap Stereo Eyes: false
+ Value: false
+ Focal Point:
+ X: 0
+ Y: 0
+ Z: 0
+ Focal Shape Fixed Size: true
+ Focal Shape Size: 0.05000000074505806
+ Invert Z Axis: false
+ Name: Current View
+ Near Clip Distance: 0.009999999776482582
+ Pitch: 0.564796507358551
+ Target Frame:
+ Value: Orbit (rviz)
+ Yaw: 1.5854246616363525
+ Saved: ~
+Window Geometry:
+ Displays:
+ collapsed: false
+ Height: 1025
+ Hide Left Dock: false
+ Hide Right Dock: false
+ QMainWindow State: 000000ff00000000fd00000004000000000000015600000363fc0200000008fb0000001200530065006c0065006300740069006f006e00000001e10000009b0000005c00fffffffb0000001e0054006f006f006c002000500072006f007000650072007400690065007302000001ed000001df00000185000000a3fb000000120056006900650077007300200054006f006f02000001df000002110000018500000122fb000000200054006f006f006c002000500072006f0070006500720074006900650073003203000002880000011d000002210000017afb000000100044006900730070006c006100790073010000003d00000363000000c900fffffffb0000002000730065006c0065006300740069006f006e00200062007500660066006500720200000138000000aa0000023a00000294fb00000014005700690064006500530074006500720065006f02000000e6000000d2000003ee0000030bfb0000000c004b0069006e0065006300740200000186000001060000030c00000261000000010000010f00000363fc0200000003fb0000001e0054006f006f006c002000500072006f00700065007200740069006500730100000041000000780000000000000000fb0000000a00560069006500770073010000003d00000363000000a400fffffffb0000001200530065006c0065006300740069006f006e010000025a000000b200000000000000000000000200000490000000a9fc0100000001fb0000000a00560069006500770073030000004e00000080000002e10000019700000003000007800000003efc0100000002fb0000000800540069006d0065010000000000000780000002eb00fffffffb0000000800540069006d006501000000000000045000000000000000000000050f0000036300000004000000040000000800000008fc0000000100000002000000010000000a0054006f006f006c00730100000000ffffffff0000000000000000
+ Selection:
+ collapsed: false
+ Time:
+ collapsed: false
+ Tool Properties:
+ collapsed: false
+ Views:
+ collapsed: false
+ Width: 1920
+ X: 0
+ Y: 27
diff --git a/jsk_fetch_robot/jsk_fetch_startup/config/warning_blacklist.yaml b/jsk_fetch_robot/jsk_fetch_startup/config/warning_blacklist.yaml
new file mode 100644
index 0000000000..54dc6a28f6
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/config/warning_blacklist.yaml
@@ -0,0 +1,15 @@
+blacklist:
+ - name: "/CPU/CPU Usage/my_machine CPU Usage"
+ - name: "/Other/amcl: Standard deviation"
+ - name: "/Other/jsk_joy_node: Joystick Driver Status"
+ - name: "/Other/l515_head .*realsense2_camera_.*: Frequency Status"
+ - name: "/Other/rosserial_python"
+ - name: "/Peripherals/PS3 Controller"
+ - name: "/Sensors/IMU/IMU.*"
+ - name: "/SoundPlay/sound_play.*"
+run_stop_blacklist:
+ - name: "/Motor Control Boards/.*_(mcb|breaker)"
+ - name: "/System/Mainboard/Mainboard"
+ message: "Runstop pressed"
+ - name: "/Breakers/.*"
+ message: "Disabled."
diff --git a/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_certbot.conf b/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_certbot.conf
new file mode 100644
index 0000000000..8c7cc3ea3b
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_certbot.conf
@@ -0,0 +1 @@
+0 0 1 Jan,Apr,Jul,Oct * bash -c ". /home/fetch/ros/melodic/devel/setup.bash && systemctl stop haproxy && certbot renew -v --standalone --renew-hook \"$(rospack find jsk_fetch_startup)/scripts/certbot_renewhook.sh\" >> /var/log/certbot_renewal.log"
diff --git a/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_fetch.conf b/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_fetch.conf
new file mode 100644
index 0000000000..03fadaf878
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_fetch.conf
@@ -0,0 +1,2 @@
+0 3 * * * bash -c ". /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup update_workspace.sh &> /home/fetch/ros/melodic/update_workspace.log"
+0 5 * * * bash -c ". /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup storage_warning.sh &> /home/fetch/ros/melodic/storage_warning.log"
diff --git a/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_root.conf b/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_root.conf
new file mode 100644
index 0000000000..da33972f7e
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/cron_scripts/cron_root.conf
@@ -0,0 +1 @@
+0 4 * * * /sbin/shutdown -r now
diff --git a/jsk_fetch_robot/jsk_fetch_startup/data/burnable.png b/jsk_fetch_robot/jsk_fetch_startup/data/burnable.png
new file mode 100644
index 0000000000..6b00521962
Binary files /dev/null and b/jsk_fetch_robot/jsk_fetch_startup/data/burnable.png differ
diff --git a/jsk_fetch_robot/jsk_fetch_startup/data/icmark.png b/jsk_fetch_robot/jsk_fetch_startup/data/icmark.png
new file mode 100644
index 0000000000..125b739436
Binary files /dev/null and b/jsk_fetch_robot/jsk_fetch_startup/data/icmark.png differ
diff --git a/jsk_fetch_robot/jsk_fetch_startup/data/plastic.png b/jsk_fetch_robot/jsk_fetch_startup/data/plastic.png
new file mode 100644
index 0000000000..e1df1b97aa
Binary files /dev/null and b/jsk_fetch_robot/jsk_fetch_startup/data/plastic.png differ
diff --git a/jsk_fetch_robot/jsk_fetch_startup/euslisp/navigation-utils.l b/jsk_fetch_robot/jsk_fetch_startup/euslisp/navigation-utils.l
index 9c3f9d4417..7134603ce2 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/euslisp/navigation-utils.l
+++ b/jsk_fetch_robot/jsk_fetch_startup/euslisp/navigation-utils.l
@@ -10,6 +10,7 @@
(ros::load-ros-manifest "jsk_robot_startup")
(ros::load-ros-manifest "jsk_recognition_msgs")
(ros::load-ros-manifest "power_msgs")
+(ros::load-ros-manifest "sensor_msgs")
(defparameter *dock-action* nil)
(defparameter *undock-action* nil)
@@ -117,7 +118,7 @@
(simple-dock))
-(defun undock ()
+(defun undock (&key (rotate-in-place nil))
(unless *undock-action*
(setq *undock-action*
(instance ros::simple-action-client :init
@@ -126,14 +127,45 @@
(ros::ros-error "/undock action server is not started")
(return-from undock nil))
(send *undock-action* :send-goal
- (instance fetch_auto_dock_msgs::UndockActionGoal :init))
+ (instance fetch_auto_dock_msgs::UndockActionGoal :init
+ :goal (instance fetch_auto_dock_msgs::UndockGoal :rotate_in_place rotate-in-place)))
(unless (send *undock-action* :wait-for-result :timeout 60)
(ros::ros-error "No result returned from /undock action server")
(return-from undock nil))
(send (send *undock-action* :get-result) :undocked))
+(defun wait-until-is-charging (&key (timeout 10))
+ """
+ Wait for updating battery charging state especially when robots dock.
+ Args:
+ - key
+ timeout [sec]: Set waiting time for updating /battery_state topic
+
+ ;; If /battery_state is subscribed before /battery_state becomes true
+ ;; while dock succeeds, the result will not be correct,
+ ;; so wait for a certain time when docking.
+ """
+ (let* ((msg nil)
+ (is-charging nil)
+ (duration 0)
+ (start-time (send (ros::time-now) :sec)))
+ (while (not is-charging)
+ (when (> duration timeout)
+ (ros::ros-warn "Skip waiting for charging state because of timeout")
+ (return-from wait-until-is-charging :timeout))
+ (setq msg (one-shot-subscribe "/battery_state" power_msgs::BatteryState
+ :timeout 1000))
+ (if msg (setq is-charging (send msg :is_charging)))
+ (setq duration (- (send (ros::time-now) :sec) start-time)))
+ :charging))
(defun get-battery-charging-state (&key (timeout 1500))
+ """
+ Get battery charging state
+ Args:
+ - key
+ timeout: Set waiting time for topic.
+ """
(let* ((msg (one-shot-subscribe "/battery_state" power_msgs::batterystate :timeout timeout))
(is-charging (if msg (send msg :is_charging))))
;; You may fail to subscribe /battery_state
@@ -141,7 +173,6 @@
(if (not msg) (return-from get-battery-charging-state nil))
(if is-charging :charging :discharging)))
-
(defun go-to-spot (name &key (relative-pos nil) (relative-rot nil) (undock-rotate nil) (clear-costmap t))
"Move the robot to the spot defined in /spots_marker_array topic. The reason for using relative-pos and relative-rot instead of relative-coords is that it is easier to understand if relative-pos and relative-rot are specified in the different coords (specifically, world (map) and local (spot) coords respectively). For detail, see https://github.com/jsk-ros-pkg/jsk_robot/pull/1458#pullrequestreview-1039654868
Args:
@@ -169,10 +200,7 @@ Args:
(return-from go-to-spot-undock t))
(if (equal battery-charging-state :charging)
(progn
- (setq undock-success (auto-undock :n-trial 3))
- ;; rotate after undock
- (if (and undock-success undock-rotate)
- (send *ri* :go-pos-unsafe 0 0 180)))
+ (setq undock-success (auto-undock :n-trial 3 :rotate-in-place undock-rotate)))
(return-from go-to-spot-undock t))
(if (not undock-success)
(progn
@@ -202,14 +230,14 @@ Args:
success))
-(defun auto-undock (&key (n-trial 1))
+(defun auto-undock (&key (n-trial 1) (rotate-in-place nil))
(let ((success nil))
(unless (boundp '*ri*)
(require :fetch-interface "package://fetcheus/fetch-interface.l")
(fetch-init))
(dotimes (i n-trial)
(ros::ros-info "trying to do undock.")
- (setq success (undock))
+ (setq success (undock :rotate-in-place rotate-in-place))
(when success (return-from auto-undock success)))
(if (not success)
(let ((enable-request (instance power_msgs::BreakerCommandRequest :init :enable t))
@@ -390,6 +418,35 @@ Args:
(format nil "~A is found" label-names))
label-names)))
+(defun notify-co2 (&key (location "kitchen"))
+ (let* ((msg (one-shot-subscribe "/eco2" std_msgs::UInt16
+ :timeout 3000)))
+ (when msg
+ (ros::ros-info
+ (format nil "Notify app that co2 concentration is measured."))
+ (notify-app "CO2 concentration"
+ (ros::time-now)
+ location
+ (format nil "CO2 concentration is ~A ppm" (send msg :data))))))
+
+(defun notify-trashcan-occupancy (&key (location "kitchen"))
+ (let* ((msg (one-shot-subscribe "/trashbin_occupancy_detector/container/occupancies"
+ jsk_recognition_msgs::BoundingBoxArray
+ :timeout 3000))
+ (occupancy (if msg (send (elt (send msg :boxes) 0) :value)))
+ (notify-text (if occupancy (if (> occupancy 1.0)
+ "Trashcan is full."
+ "Trashcan is not full."))))
+ (when occupancy
+ (ros::ros-info
+ (format nil "Notify app that the occupancy of trash can is measured. ~A." occupancy))
+ (notify-app "trashcan occupancy"
+ (ros::time-now)
+ location
+ (format nil "~A Trashcan occupancy is ~A"
+ notify-text
+ occupancy)))))
+
(defun inspect-kitchen (&key (tweet t))
(report-move-to-sink-front-success)
(if tweet
@@ -398,6 +455,7 @@ Args:
(tweet-string "I took a photo at 73B2 Kitchen stove." :warning-time 3
:with-image "/edgetpu_object_detector/output/image" :speak t)
(notify-recognition :location "kitchen stove")
+ (notify-co2 :location "kitchen stove")
(take-photo "kitchen_stove.jpg")
(send *ri* :go-pos-unsafe 0 0 -45)
;; sink
@@ -504,7 +562,7 @@ Args:
(ros::publish "/photo_taken" img)))))
(defun inspect-desk-back (&key (tweet t))
- (report-move-to-tv-desk)
+ (report-move-to-desk-back)
(send *fetch* :angle-vector #f(21.5684 75.5832 80.1877 -11.4819 93.608 -0.04749 95.2016 -0.072829 -1.45585 10.8727))
(send *ri* :angle-vector-raw (send *fetch* :angle-vector) 3000 :head-controller)
(send *ri* :wait-interpolation)
@@ -516,7 +574,7 @@ Args:
(ros::publish "/photo_taken" img)))))
(defun inspect-desk-front (&key (tweet t))
- (report-move-to-tv-desk)
+ (report-move-to-desk-front)
(send *fetch* :angle-vector #f(21.5761 75.5173 80.2097 -11.5478 93.6519 -0.04749 95.1796 -0.006954 -1.11597 10.8288))
(send *ri* :angle-vector-raw (send *fetch* :angle-vector) 3000 :head-controller)
(send *ri* :wait-interpolation)
@@ -528,7 +586,7 @@ Args:
(ros::publish "/photo_taken" img)))))
(defun inspect-kitchen-door-front (&key (tweet t))
- (report-move-to-tv-desk)
+ (report-move-to-kitchen-door-front)
(send *fetch* :angle-vector #f(21.5608 75.5612 80.2316 -11.5039 93.586 -0.04749 95.1576 -0.050857 3.17796 16.3219))
(send *ri* :angle-vector-raw (send *fetch* :angle-vector) 3000 :head-controller)
(send *ri* :wait-interpolation)
@@ -559,6 +617,7 @@ Args:
(ros::publish "/photo_taken" img))
(take-photo "trashcan_inside.jpg")
(notify-recognition :location "trash cans inside")
+ (notify-trashcan-occupancy :location "trash can")
(send *ri* :go-pos-unsafe -0.2 0 0))
(progn
(notify-recognition :location "trash cans"))))
@@ -620,7 +679,8 @@ Args:
;; change the inflation_radius
(restore-params)
(send-kitchen-mail)
- (setq success-battery-charging (equal (get-battery-charging-state) :charging))
+ (setq success-battery-charging (progn (wait-until-is-charging)
+ (equal (get-battery-charging-state) :charging)))
(and success-go-to-kitchen success-auto-dock success-battery-charging)))
@@ -667,13 +727,15 @@ Args:
(:move-to-sink-front -> :inspect-kitchen)
(:move-to-sink-front !-> :report-move-to-sink-front-failure)
;;(:inspect-kitchen -> :auto-dock)
- ;;(:report-move-to-sink-front-failure -> :auto-dock)
+ (:report-move-to-sink-front-failure -> :auto-dock)
(:inspect-kitchen -> :move-to-trashcan-front)
(:move-to-trashcan-front -> :inspect-trashcan)
(:move-to-trashcan-front !-> :report-move-to-trashcan-front-failure)
(:inspect-trashcan -> :auto-dock)
(:report-move-to-trashcan-front-failure -> :auto-dock)
(:auto-dock -> :room-light-off)
+ (:auto-dock !-> :auto-dock-failure)
+ (:auto-dock-failure -> :room-light-off)
(:room-light-off -> :finish)
(:finish -> t)
(:finish !-> nil))
@@ -859,11 +921,11 @@ Args:
success)))
(:inspect-kitchen
'(lambda (userdata)
- (let ((label-names (notify-recognition :location "kitchen"))
- (notify-text (if label-names (format nil "~Aがあったよ" label-names) "")))
- (inspect-kitchen :tweet (cdr (assoc 'tweet userdata)))
- (set-alist 'description (format nil "キッチンの様子を見たよ。~A" notify-text) userdata))
- (set-alist 'image (remove #\newline (base64encode (send *image* :serialize))) userdata)
+ (let* ((label-names (notify-recognition :location "kitchen"))
+ (notify-text (if label-names (format nil "~Aがあったよ" label-names) "")))
+ (inspect-kitchen :tweet (cdr (assoc 'tweet userdata)))
+ (set-alist 'description (format nil "キッチンの様子を見たよ。~A" notify-text) userdata))
+ (set-alist 'image (remove #\newline (base64encode (send *image* :serialize))) userdata)
t))
(:report-move-to-sink-front-failure
'(lambda (userdata)
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/devices/realsense_nodelet.launch.xml b/jsk_fetch_robot/jsk_fetch_startup/launch/devices/realsense_nodelet.launch.xml
index a72dd3f2d1..c5993ed682 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/devices/realsense_nodelet.launch.xml
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/devices/realsense_nodelet.launch.xml
@@ -3,6 +3,8 @@
This launch file is copied from realsense2_camera in realsense_ros https://github.com/IntelRealSense/realsense-ros and then modified
And it is originally distributed under Apache-2.0 License
-->
+
+
@@ -10,6 +12,7 @@
+
@@ -101,11 +104,12 @@
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch.launch
index a09c95a3c8..16dea4af56 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch.launch
@@ -1,11 +1,23 @@
-
-
+
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
@@ -22,10 +34,15 @@
-
+
+
+
+
+
+
@@ -33,6 +50,8 @@
+
+
+ base_camera_mount:=$(arg use_base_camera_mount) head_box:=$(arg use_head_box)
+ head_l515:=$(arg use_head_l515) insta360_stand:=$(arg insta360_stand)" />
@@ -166,6 +186,15 @@
+
+
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_auto_dock.xml b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_auto_dock.xml
new file mode 100644
index 0000000000..be7b25f86c
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_auto_dock.xml
@@ -0,0 +1,14 @@
+
+
+
+
+ duration_timeout_undock: 10.0
+ duration_timeout_preorientation: 20.0
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_bringup.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_bringup.launch
index 41f2e55e75..a587c70d13 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_bringup.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_bringup.launch
@@ -1,18 +1,40 @@
-
-
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
@@ -21,17 +43,17 @@
+ respawn="true" output="screen" if="$(arg battery_warning)">
duration: 180
- charge_level_threshold: 80.0
+ charge_level_threshold: 65.0
shutdown_level_threshold: 60.0
charge_level_step: 10.0
volume: 1.0
-
+
lang: japanese
volume: 0.5
@@ -39,7 +61,7 @@
-
+
@@ -56,16 +78,20 @@
+ respawn="true" if="$(arg launch_sound_play)">
+
+ default_voice: $(arg default_english_speaker)
+ plugin: sound_play/flite_plugin
+
+
-
-
-
+
+
@@ -74,14 +100,15 @@
-
+
+ name="diag_agg" args="CPP" output="screen"
+ clear_params="true" >
@@ -96,7 +123,7 @@
-
+
@@ -109,76 +136,116 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
-
+
-
-
-
-
-
-
-
-
-
+
+
-
+
-
+
interface_name: wlan0
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
-
- tts_action_names:
- - sound_play
- - robotsound_jp
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ tts_action_names:
+ - sound_play
+ - robotsound_jp
+
+
+
+
+
+
+
+
+ default_select: speech_to_text_google
+ patient: 6
+
+
-
-
-
-
-
-
- default_select: speech_to_text_google
- patient: 6
-
-
@@ -243,6 +310,9 @@
+
+ luminance_threshold: 70
+
@@ -277,8 +347,40 @@
+
+
+
+
+ run_stop_topic: robot_state
+ run_stop_condition: "m.runstopped is True"
+ seconds_to_start_speaking: 60
+ speak_interval: 600
+ language: $(arg default_warning_speaker)
+ ignore_time_after_runstop_is_enabled: 5.0
+ ignore_time_after_runstop_is_disabled: 5.0
+
+
+
+
+
+
+
+
+
-
+
duration: 60
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_coral.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_coral.launch
new file mode 100644
index 0000000000..237ef48534
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_coral.launch
@@ -0,0 +1,56 @@
+
+
+
+
+
+
+
+
+
+
+
+ edgetpu_human_node_manager:
+ nodes:
+ - name: edgetpu_human_pose_estimator
+ type: human_pose_estimator
+ - name: edgetpu_panorama_human_pose_estimator
+ type: panorama_human_pose_estimator
+ default: edgetpu_human_pose_estimator
+ edgetpu_human_pose_estimator:
+ input_topic: $(arg input_image)
+ image_transport: raw
+ device_id: 0
+ edgetpu_panorama_human_pose_estimator:
+ input_topic: $(arg input_panorama_image)
+ image_transport: raw
+ device_id: 0
+
+
+
+
+
+
+ edgetpu_object_node_manager:
+ nodes:
+ - name: edgetpu_object_detector
+ type: object_detector
+ - name: edgetpu_panorama_object_detector
+ type: panorama_object_detector
+ default: edgetpu_object_detector
+ edgetpu_object_detector:
+ input_topic: $(arg input_image)
+ image_transport: raw
+ device_id: 1
+ model_file: /etc/ros/jsk_data/coral_usb/mobilenet_v2_ssd_73b2_kitchen_finetuning_20200528.tflite
+ label_file: /etc/ros/jsk_data/coral_usb/73b2_kitchen_labels.txt
+ edgetpu_panorama_object_detector:
+ input_topic: $(arg input_panorama_image)
+ image_transport: raw
+ device_id: 1
+ model_file: /etc/ros/jsk_data/coral_usb/mobilenet_v2_ssd_73b2_kitchen_finetuning_20200528.tflite
+ label_file: /etc/ros/jsk_data/coral_usb/73b2_kitchen_labels.txt
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_dialogflow_task_executive.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_dialogflow_task_executive.launch
new file mode 100644
index 0000000000..a236629a55
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_dialogflow_task_executive.launch
@@ -0,0 +1,32 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_indigo.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_indigo.launch
index 5cd5374aa4..2835f972fd 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_indigo.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_indigo.launch
@@ -1,6 +1,7 @@
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_melodic.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_melodic.launch
index c94523f778..9958ed71f2 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_melodic.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_insta360_melodic.launch
@@ -2,14 +2,18 @@
+
-
-
+
+
+
+
+
@@ -21,8 +25,8 @@
- scale_width: 0.25
- scale_height: 0.25
+ scale_width: 0.5
+ scale_height: 0.5
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_lifelog.xml b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_lifelog.xml
index fc02dea6d2..75c150fbed 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_lifelog.xml
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_lifelog.xml
@@ -20,8 +20,8 @@
-
-
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_realsense_bringup.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_realsense_bringup.launch
index c28fde2de9..1e0ab40556 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_realsense_bringup.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_realsense_bringup.launch
@@ -1,9 +1,36 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
-
+
/tracking_module/enable_mapping: false
/tracking_module/enable_relocalization: false
@@ -13,7 +40,7 @@
-
+
@@ -44,39 +71,131 @@
-
+
-
+
-
-
+
-
+
-
+
-
-
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ /l515_head/motion_module/global_time_enabled: true
+ /l515_head/l500_depth_sensor/global_time_enabled: true
+ /l515_head/rgb_camera/global_time_enabled: true
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ step_x: 4
+ step_y: 4
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_sensors.xml b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_sensors.xml
index 43d79040b6..2649c370e9 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_sensors.xml
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_sensors.xml
@@ -16,6 +16,15 @@
+
+
+
+
+ warning_hz: 5
+
+
+
+
+
+
+ warning_hz: 5
+
+
-
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_tweet.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_tweet.launch
index d8faf71fb8..198a504b64 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_tweet.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/fetch_tweet.launch
@@ -1,11 +1,10 @@
-
-
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/moveit/fetch.srdf.xacro b/jsk_fetch_robot/jsk_fetch_startup/launch/moveit/fetch.srdf.xacro
index 3a3fe90d40..3371641386 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/moveit/fetch.srdf.xacro
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/moveit/fetch.srdf.xacro
@@ -1,7 +1,12 @@
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch1075/fetch_move_base_common_params.yaml b/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch1075/fetch_move_base_common_params.yaml
index e69de29bb2..b7eabb9a39 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch1075/fetch_move_base_common_params.yaml
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch1075/fetch_move_base_common_params.yaml
@@ -0,0 +1,36 @@
+move_base:
+ recovery_behavior_enabled: true
+ recovery_behaviors:
+ - name: "conservative_reset"
+ type: "clear_costmap_recovery/ClearCostmapRecovery"
+ - name: "rotate_recovery0"
+ type: "rotate_recovery/RotateRecovery"
+ - name: "speak_and_wait0"
+ type: "speak_and_wait_recovery/SpeakAndWaitRecovery"
+ - name: "aggressive_reset"
+ type: "clear_costmap_recovery/ClearCostmapRecovery"
+ - name: "rotate_recovery1"
+ type: "rotate_recovery/RotateRecovery"
+ - name: "speak_and_wait1"
+ type: "speak_and_wait_recovery/SpeakAndWaitRecovery"
+ - name: "all_reset"
+ type: "clear_costmap_recovery/ClearCostmapRecovery"
+ - name: "rotate_recovery2"
+ type: "rotate_recovery/RotateRecovery"
+ - name: "move_slow_and_clear"
+ type: "move_slow_and_clear/MoveSlowAndClear"
+ speak_and_wait0:
+ speak_text: "とおれません、みちをあけてください"
+ duration_wait: 5.0
+ duration_timeout: 1.0
+ sound_action: /robotsound_jp
+ speak_and_wait1:
+ speak_text: "とおれません、みちをあけてください"
+ duration_wait: 5.0
+ duration_timeout: 1.0
+ sound_action: /robotsound_jp
+ move_slow_and_clear:
+ planner_namespace: TrajectoryPlannerROS
+ max_trans_param_name: max_vel_x
+ max_rot_param_name: max_vel_theta
+ max_planning_retries: 2
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch15/fetch_move_base_common_params.yaml b/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch15/fetch_move_base_common_params.yaml
index a0a284bfa1..0d3130cdcb 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch15/fetch_move_base_common_params.yaml
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/navigation/fetch15/fetch_move_base_common_params.yaml
@@ -1,119 +1,18 @@
move_base:
- controller_frequency: 20.0
- global_costmap:
- inflater:
- inflation_radius: 1.0 # 0.7
- cost_scaling_factor: 10.0 # 10.0
- obstacles:
- min_obstacle_height: 0.05
- footprint_padding: 0.05
- publish_frequency: 1.0
- local_costmap:
- inflater:
- inflation_radius: 1.0 # 0.7
- cost_scaling_factor: 10.0 # 25.0 default 10, increasing factor decrease the cost value
- obstacles:
- min_obstacle_height: 0.05
- # default 5 (http://wiki.ros.org/navigation/Tutorials/Navigation%20Tuning%20Guide)
- footprint_padding: 0.05
- update_frequency: 5.0
- base_global_planner: global_planner/GlobalPlanner
- base_local_planner: teb_local_planner/TebLocalPlannerROS
- # base_local_planner: base_local_planner/TrajectoryPlannerROS
- GlobalPlanner:
- allow_unknown: true
- default_tolerance: 0.0
- orientation_mode: 1
- visualize_potential: false
- publish_potential: false
- TrajectoryPlannerROS:
- yaw_goal_tolerance: 0.1
- xy_goal_tolerance: 0.17
- max_vel_x: 0.5
- min_vel_x: 0.1
- min_in_place_vel_theta: 0.5
- escape_vel: 0.0
- sim_time: 1.5
- sim_granularity: 0.025
- angular_sim_granularity: 0.025
- vx_samples: 10
- vth_samples: 40
- meter_scoring: true
- pdist_scale: 4.0
- gdist_scale: 2.5
- occdist_scale: 0.00625
- dwa: true
- TebLocalPlannerROS:
- odom_topic: /odom_combined
- map_frame: /odom
- # Trajectory
- teb_autosize: True
- dt_ref: 0.3
- dt_hysteresis: 0.1
- global_plan_overwrite_orientation: True
- max_global_plan_lookahead_dist: 3.0
- feasibility_check_no_poses: 5
- # Robot
- max_vel_x: 0.5
- max_vel_x_backwards: 0.3
- max_vel_theta: 1.0
- acc_lim_x: 1.0
- acc_lim_theta: 1.0
- min_turning_radius: 0.2
- footprint_model:
- type: "circular"
- radius: 0.3
- # GoalTolerance
- xy_goal_tolerance: 0.2
- yaw_goal_tolerance: 0.1
- free_goal_vel: False
- # Obstacles
- min_obstacle_dist: 0.1
- include_costmap_obstacles: True
- costmap_obstacles_behind_robot_dist: 1.0
- obstacle_poses_afected: 30
- costmap_converter_plugin: ""
- costmap_converter_spin_thread: True
- costmap_converter_rate: 5
- # Optimization
- no_inner_iterations: 5
- no_outer_iterations: 4
- optimization_activate: True
- optimization_verbose: False
- penalty_epsilon: 0.1
- weight_max_vel_x: 2
- weight_max_vel_theta: 1
- weight_acc_lim_x: 1
- weight_acc_lim_theta: 1
- weight_kinematics_nh: 1000
- weight_kinematics_forward_drive: 1
- weight_kinematics_turning_radius: 100
- weight_optimaltime: 1
- weight_obstacle: 50
- weight_dynamic_obstacle: 10 # not in use yet
- alternative_time_cost: False # not in use yet
- # Homotopy Class Planner
- enable_homotopy_class_planning: True
- enable_multithreading: True
- simple_exploration: False
- max_number_classes: 4
- roadmap_graph_no_samples: 15
- roadmap_graph_area_width: 5
- h_signature_prescaler: 0.5
- h_signature_threshold: 0.1
- obstacle_keypoint_offset: 0.1
- obstacle_heading_threshold: 0.45
- visualize_hc_graph: False
recovery_behavior_enabled: true
recovery_behaviors:
- name: "conservative_reset"
type: "clear_costmap_recovery/ClearCostmapRecovery"
- name: "rotate_recovery0"
type: "rotate_recovery/RotateRecovery"
+ - name: "speak_and_wait0"
+ type: "speak_and_wait_recovery/SpeakAndWaitRecovery"
- name: "aggressive_reset"
type: "clear_costmap_recovery/ClearCostmapRecovery"
- name: "rotate_recovery1"
type: "rotate_recovery/RotateRecovery"
+ - name: "speak_and_wait1"
+ type: "speak_and_wait_recovery/SpeakAndWaitRecovery"
- name: "all_reset"
type: "clear_costmap_recovery/ClearCostmapRecovery"
- name: "rotate_recovery2"
@@ -125,11 +24,21 @@ move_base:
rotate_recovery0:
frequency: 20.0
sim_granularity: 0.017
+ speak_and_wait0:
+ speak_text: "とおれません、みちをあけてください"
+ duration_wait: 5.0
+ duration_timeout: 1.0
+ sound_action: /robotsound_jp
aggressive_reset:
reset_distance: 1.0 # 0.5
rotate_recovery1:
frequency: 20.0
sim_granularity: 0.017
+ speak_and_wait1:
+ speak_text: "とおれません、みちをあけてください"
+ duration_wait: 5.0
+ duration_timeout: 1.0
+ sound_action: /robotsound_jp
all_reset:
reset_distance: 0.0
rotate_recovery2:
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_play.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_play.launch
index 1fdab69f5f..20abc5d53a 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_play.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_play.launch
@@ -12,6 +12,8 @@
+
+
@@ -104,8 +106,8 @@
+ args="$(arg rosbag) $(arg loop_flag) --clock --start $(arg start_time) $(arg duration) -r $(arg rate)" output="screen" />
+ args="-d $(arg rviz_config)" />
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_record.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_record.launch
index 43b5a923ce..12dd846fd3 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_record.launch
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/rosbag_record.launch
@@ -35,6 +35,9 @@
/head_camera/depth_registered/throttled/camera_info
/head_camera/rgb/throttled/image_rect_color/compressed
/head_camera/depth_registered/throttled/image_rect/compressedDepth
+ /server_name/smach/container_init
+ /server_name/smach/container_status
+ /server_name/smach/container_structure
/audio
$(arg other_topics)
$(arg regex_flag)
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/trashbin_occupancy_detector.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/trashbin_occupancy_detector.launch
new file mode 100644
index 0000000000..76030da7cd
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/trashbin_occupancy_detector.launch
@@ -0,0 +1,176 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ use_indices: false
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ tolerance: 0.02
+ min_size: 20
+ vital_rate: 0.1
+
+
+
+
+
+
+
+ align_boxes: true
+ align_boxes_with_plane: false
+ target_frame_id: base_link
+ use_pca: true
+ force_to_flip_z_axis: false
+ vital_rate: 0.1
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ trashbin_l: 0.3
+ trashbin_w: 0.2
+ trashbin_h: 0.49
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/launch/view_model.launch b/jsk_fetch_robot/jsk_fetch_startup/launch/view_model.launch
new file mode 100644
index 0000000000..4f8ef6e3f9
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/launch/view_model.launch
@@ -0,0 +1,17 @@
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/package.xml b/jsk_fetch_robot/jsk_fetch_startup/package.xml
index a89d0c4dea..0a031ea04e 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/package.xml
+++ b/jsk_fetch_robot/jsk_fetch_startup/package.xml
@@ -29,12 +29,15 @@
pr2_computer_monitor
robot_pose_publisher
rviz
+ switchbot_ros
tf
touchegg
sound_play
switchbot_ros
tf2_ros
voice_text
+ tf2
+ tf2_geometry_msgs
amcl
@@ -64,9 +67,16 @@
julius_ros
respeaker_ros
+
+ dialogflow_task_executive
+ google_chat_ros
+
robot_pose_publisher
+
+ jsk_pcl_ros
+
rostest
diff --git a/jsk_fetch_robot/jsk_fetch_startup/robots/head_l515.srdf.xacro b/jsk_fetch_robot/jsk_fetch_startup/robots/head_l515.srdf.xacro
new file mode 100644
index 0000000000..fdc9de6879
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/robots/head_l515.srdf.xacro
@@ -0,0 +1,6 @@
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/robots/head_l515.urdf.xacro b/jsk_fetch_robot/jsk_fetch_startup/robots/head_l515.urdf.xacro
new file mode 100644
index 0000000000..24885a8324
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/robots/head_l515.urdf.xacro
@@ -0,0 +1,43 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/robots/insta360_stand.urdf.xacro b/jsk_fetch_robot/jsk_fetch_startup/robots/insta360_stand.urdf.xacro
new file mode 100644
index 0000000000..690742cfa6
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/robots/insta360_stand.urdf.xacro
@@ -0,0 +1,15 @@
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/robots/jsk_fetch.urdf.xacro b/jsk_fetch_robot/jsk_fetch_startup/robots/jsk_fetch.urdf.xacro
index a3caf26abf..ede62cbb2c 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/robots/jsk_fetch.urdf.xacro
+++ b/jsk_fetch_robot/jsk_fetch_startup/robots/jsk_fetch.urdf.xacro
@@ -1,9 +1,11 @@
-
-
+
+
+
+
@@ -17,4 +19,10 @@
+
+
+
+
+
+
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/certbot_renewhook.sh b/jsk_fetch_robot/jsk_fetch_startup/scripts/certbot_renewhook.sh
new file mode 100755
index 0000000000..d37fe27421
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/certbot_renewhook.sh
@@ -0,0 +1,7 @@
+#!/bin/sh
+
+SITE=dialogflow.$(hostname).jsk.imi.i.u-tokyo.ac.jp
+
+cd /etc/letsencrypt/live/$SITE
+cat fullchain.pem privkey.pem > /etc/haproxy/certs/$SITE.pem
+systemctl restart haproxy
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/correct_position.py b/jsk_fetch_robot/jsk_fetch_startup/scripts/correct_position.py
index db9c04f6c7..006ee85c3c 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/scripts/correct_position.py
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/correct_position.py
@@ -21,7 +21,8 @@ def __init__(self):
if robot_name == 'fetch15':
dock_name = '/eng2/7f/room73B2-fetch-dock-front'
elif robot_name == 'fetch1075':
- dock_name = '/eng2/7f/room73B2-fetch-dock-entrance'
+ dock_name = '/eng2/7f/room73B2-fetch-dock2-front'
+ # dock_name = '/eng2/7f/room73B2-fetch-dock-entrance'
for spot in spots.markers:
if spot.text == dock_name:
self.dock_pose = spot.pose
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/dstat.bash b/jsk_fetch_robot/jsk_fetch_startup/scripts/dstat.bash
new file mode 100755
index 0000000000..440237145e
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/dstat.bash
@@ -0,0 +1,14 @@
+#!/usr/bin/env bash
+
+# Keep only the last 1000 lines of csv log before executing dstat
+CSV_FILE=/home/fetch/Documents/jsk_dstat.csv
+
+if [ -e $CSV_FILE ]; then
+ cp -f $CSV_FILE $CSV_FILE.bk
+ tail -n 1000 $CSV_FILE.bk > $CSV_FILE
+ rm -f $CSV_FILE.bk
+else
+ touch $CSV_FILE
+fi
+
+dstat -tl --cpufreq -c -C all --top-cpu-adv -dgimnprsTy --output $CSV_FILE
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/fetchctl.sh b/jsk_fetch_robot/jsk_fetch_startup/scripts/fetchctl.sh
new file mode 100755
index 0000000000..ec5d8f0b84
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/fetchctl.sh
@@ -0,0 +1,63 @@
+#!/bin/bash
+
+function usage() {
+ echo "Usage: $0 "
+ exit 1
+}
+
+function start_jobs() {
+ sudo supervisorctl start roscore
+ sudo supervisorctl start robot
+ sudo supervisorctl start jsk-shutdown
+ sudo supervisorctl start jsk-rfcomm-bind
+ sudo supervisorctl start jsk-object-detector
+ sudo supervisorctl start jsk-network-monitor
+ sudo supervisorctl start jsk-log-wifi
+ sudo supervisorctl start jsk-human-pose-estimator
+ sudo supervisorctl start jsk-gdrive
+ sudo supervisorctl start jsk-fetch-startup
+ sudo supervisorctl start jsk-dstat
+ sudo supervisorctl start jsk-dialog
+ sudo supervisorctl start jsk-app-scheduler
+}
+
+function restart_jobs() {
+ sudo supervisorctl restart roscore
+ sudo supervisorctl restart robot
+ sudo supervisorctl restart jsk-shutdown
+ sudo supervisorctl restart jsk-rfcomm-bind
+ sudo supervisorctl restart jsk-object-detector
+ sudo supervisorctl restart jsk-network-monitor
+ sudo supervisorctl restart jsk-log-wifi
+ sudo supervisorctl restart jsk-human-pose-estimator
+ sudo supervisorctl restart jsk-gdrive
+ sudo supervisorctl restart jsk-fetch-startup
+ sudo supervisorctl restart jsk-dstat
+ sudo supervisorctl restart jsk-dialog
+ sudo supervisorctl restart jsk-app-scheduler
+}
+
+function stop_jobs() {
+ sudo supervisorctl stop roscore
+ sudo supervisorctl stop robot
+ sudo supervisorctl stop jsk-shutdown
+ sudo supervisorctl stop jsk-rfcomm-bind
+ sudo supervisorctl stop jsk-object-detector
+ sudo supervisorctl stop jsk-network-monitor
+ sudo supervisorctl stop jsk-log-wifi
+ sudo supervisorctl stop jsk-human-pose-estimator
+ sudo supervisorctl stop jsk-gdrive
+ sudo supervisorctl stop jsk-fetch-startup
+ sudo supervisorctl stop jsk-dstat
+ sudo supervisorctl stop jsk-dialog
+ sudo supervisorctl stop jsk-app-scheduler
+}
+
+command=$1
+
+case "$command" in
+ start) start_jobs;;
+ restart) restart_jobs;;
+ stop) stop_jobs;;
+ *) usage;;
+esac
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/log-wifi-link.sh b/jsk_fetch_robot/jsk_fetch_startup/scripts/log-wifi-link.sh
index 9de64ee353..3afeeb5c6d 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/scripts/log-wifi-link.sh
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/log-wifi-link.sh
@@ -1,25 +1,51 @@
#!/bin/bash
IFACE=wlan0
-OUTPATH=/var/log/wifi.log
INTERVAL=10
+PING_COUNT=3
+PING_TIMEOUT=1
+PING_TARGET="www.google.com"
wifi_status(){
local ssid=$(iw $IFACE link | grep SSID | cut -d: -f2)
local freq=$(iw $IFACE link | grep freq | cut -d':' -f2)
- local signal=$(cat /proc/net/wireless | grep wlan0 | awk '{print $3}')
- local level=$(cat /proc/net/wireless | grep wlan0 | awk '{print $4}')
- local noise=$(cat /proc/net/wireless | grep wlan0 | awk '{print $5}')
+ local signal=$(cat /proc/net/wireless | grep $IFACE | awk '{print $3}')
+ local level=$(cat /proc/net/wireless | grep $IFACE | awk '{print $4}')
+ local noise=$(cat /proc/net/wireless | grep $IFACE | awk '{print $5}')
local bitrate=$(iw $IFACE link | grep bitrate | cut -d':' -f2)
- echo "$(date -Iseconds),$ssid,$freq,$signal,$level,$noise,$bitrate"
+ local ping_result_raw=$(ping -I $IFACE $PING_TARGET -c $PING_COUNT -W $PING_TIMEOUT 2> /dev/null | tail -n 1)
+ if [ -z "$ping_result_raw" ]; then
+ # minus ping indicates ping fails
+ local ping_result_duration=-1000
+ else
+ local ping_result_duration=$(echo $ping_result_raw | cut -d " " -f 4 | cut -d "/" -f 2)
+ fi
+ echo "$(date -Iseconds),$ssid,$freq,$signal,$level,$noise,$bitrate, $ping_result_duration"
}
-OUTDIR=$(dirname $OUTPATH)
-if [ ! -d $OUTDIR ]; then
- mkdir -p $OUTDIR
-fi
+print_help(){
+ echo "Usage: log-wifi-link.sh [-h]"
+ echo " [-i INTERVAL (default: 10)]"
+ echo " [-I IFACE (default: wlan0)]"
+ echo " [-c PING_COUNT (default: 3)]"
+ echo " [-W PING_TIMEOUT (default: 1)]"
+ echo " [-t PING_TARGET (default: \"www.google.com\")]"
+ exit 1
+}
+
+while getopts hi:I:c:W:t: o
+do
+ case "$o" in
+ i) INTERVAL=$OPTARG;;
+ I) IFACE="$OPTARG";;
+ c) PING_COUNT=$OPTARG;;
+ W) PING_TIMEOUT=$OPTARG;;
+ t) PING_TARGET="$OPTARG";;
+ h) print_help;;
+ esac
+done
while true; do
- wifi_status >> $OUTPATH
+ wifi_status
sleep $INTERVAL
done
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/network_monitor.py b/jsk_fetch_robot/jsk_fetch_startup/scripts/network_monitor.py
index 1f67266a30..782f5479a5 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/scripts/network_monitor.py
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/network_monitor.py
@@ -47,21 +47,21 @@
TOUCH_FILE = "/home/fetch/.Network_Monitor_Restarted_Robot"
-def ping(use_arping, hosts):
+def ping(use_arping, hosts, network_interface='wlan0'):
for host in hosts:
for _ in xrange(5):
print(datetime.now(),"Sending ping request")
if use_arping:
- response = os.system("arping -I wlan0 -c 1 " + host)
+ response = os.system("arping -I {} -c 1 {}".format(network_interface, host))
else:
- response = os.system("ping -c 1 -q " + host)
+ response = os.system("ping -c 1 -q {}".format(host))
if response == 0:
return True
sleep(5)
return False
-def get_sanshiro():
- return [['sanshiro', 'sanshiro']]
+def get_sanshiro(network_profile_id):
+ return [[network_profile_id, 'sanshiro']]
def get_saved_networks(filepaths=None):
# NetworkManager has its own ID for networks, often multiple for the same
@@ -90,19 +90,19 @@ def get_saved_networks(filepaths=None):
# for reconnecting to 'sanshiro'
-def reconnect_to_wlan():
- if call(["nmcli", "d", "disconnect", "iface", "wlan0"]) == 0:
+def reconnect_to_wlan(network_interface='wlan0', network_profile_id='sanshiro'):
+ if call(["nmcli", "d", "disconnect", "iface", network_interface]) == 0:
sleep(0.3)
- print("disconnect from wlan0")
+ print("Network disconnected with interface \"{}\"".format(network_interface))
else:
- print("'nmcli d disconnect iface wlan0' do not work correctly!")
+ print("'nmcli d disconnect iface {}' do not work correctly!".format(network_interface))
- if call(["nmcli", "c", "up", "id", "sanshiro"]) == 0:
+ if call(["nmcli", "c", "up", "id", network_profile_id]) == 0:
sleep(0.3)
- print("connect to sanshiro")
+ print("Network connected with profile \"{}\"".format(network_profile_id))
return True
else:
- print("'nmcli c up id sanshiro' do not work correctly!")
+ print("'nmcli c up id {}' do not work correctly!".format(network_profile_id))
return False
@@ -150,13 +150,13 @@ def connect_by_nmcli_id(nid, ssid="SSID not given"):
return False
-def attempt_reconnect_to_network():
+def attempt_reconnect_to_network(network_interface, network_profile_id):
# First, try to connect to sanshiro
- if connect_by_nmcli_id('sanshiro', 'sanshiro'):
+ if connect_by_nmcli_id(network_profile_id, 'sanshiro'):
return True
# Get list of stored 'wireless' connections; [id, ssid]
- known = get_saved_networks() # alternative : known = get_sanshiro()
+ known = get_saved_networks() # alternative : known = get_sanshiro(network_profile_id)
# Get list of unique available wifi network ssids and their strongest signal
avail = get_avail_networks_by_strength()
@@ -170,7 +170,7 @@ def attempt_reconnect_to_network():
for ntwk in to_try:
if connect_by_nmcli_id(*ntwk):
return True
- if reconnect_to_wlan():
+ if reconnect_to_wlan(network_interface, network_profile_id):
return True
return False
@@ -249,12 +249,12 @@ def main(args):
while True:
try:
# Check if we can ping the access point
- if not ping(args.arping, hostnames):
+ if not ping(args.arping, hostnames, args.interface):
print(datetime.now(), "Cannot connect to", ', '.join(hostnames))
sound_goal.goal.sound_request.arg = "ピングが通りません"
pub.publish(sound_goal)
# Try to connect to any known network
- if not attempt_reconnect_to_network():
+ if not attempt_reconnect_to_network(args.interface, args.profile):
# TODO use check_output instead of Popen and handle truly
# bad issues with computer restarts or notification.
print(datetime.now(), "Restarting network-manager")
@@ -302,6 +302,10 @@ def main(args):
help="One or more comma delimited hostname(s) to ping. Hostnames will "
"be tried in order supplied until one is successfully contacted. Default: "
"Looks for NETWORK_MONITOR_HOSTNAMES in environment.")
+ parser.add_argument('-I', '--interface', default='wlan0',
+ help='Network interface to be used.')
+ parser.add_argument('--profile', default='sanshiro',
+ help='Network profile to be used.')
parser.add_argument("-a", "--arping", action='store_true',
help="Use arping instead of ping.")
parser.add_argument("--enable-reboot-option", action='store_true',
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/odometry_transformer.py b/jsk_fetch_robot/jsk_fetch_startup/scripts/odometry_transformer.py
index 4871e08891..e85db4d5eb 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/scripts/odometry_transformer.py
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/odometry_transformer.py
@@ -67,7 +67,8 @@ def cb_odometry(self, msg):
math.isnan(msg.twist.twist.angular.x) or
math.isnan(msg.twist.twist.angular.y) or
math.isnan(msg.twist.twist.angular.z)):
- rospy.logwarn('Recieved an odom message with nan values')
+ rospy.logwarn_throttle(
+ 10, 'Received an odom message with nan values')
return
pos_t265_odombased = np.array([
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/storage_warning.sh b/jsk_fetch_robot/jsk_fetch_startup/scripts/storage_warning.sh
new file mode 100755
index 0000000000..10f059f0b4
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/storage_warning.sh
@@ -0,0 +1,40 @@
+#!/usr/bin/env bash
+
+# Check storage percentage
+STORAGE_PERCENTAGE=`df -h / | awk 'NR==2 {print $5}' | sed -e 's/\%//g'`
+
+# If storage_percentage greater than 80%
+if [ $STORAGE_PERCENTAGE -gt 80 ]; then
+ SEND_EMAIL=1
+ # In root directory
+ ROOTDIR_STORAGE=`du -h -d 0 /* 2>/dev/null | sort -hr | awk '{print $2 " " $1}'`
+ # In home directory
+ HOMEDIR_STORAGE=`du -h -d 0 /home/* 2>/dev/null | sort -hr | awk '{print $2 " " $1}'`
+else
+ echo -e "No problem with the storace capacity. Storage percentage: $STORAGE_PERCENTAGE"
+fi
+
+if [ -n "$SEND_EMAIL" ]; then
+ rostopic pub -1 /email jsk_robot_startup/Email "header:
+ seq: 0
+ stamp: {secs: 0, nsecs: 0}
+ frame_id: ''
+subject: 'Storage warning'
+body: 'Storage usage is ${STORAGE_PERCENTAGE}%. Please remove unnecessary files.
+
+
+In root directory:
+
+${ROOTDIR_STORAGE}
+
+
+In home directory:
+
+${HOMEDIR_STORAGE}'
+sender_address: '$(hostname)@jsk.imi.i.u-tokyo.ac.jp'
+receiver_address: 'fetch@jsk.imi.i.u-tokyo.ac.jp'
+smtp_server: ''
+smtp_port: ''
+attached_files: []"
+ echo -e "Storage percentage: $STORAGE_PERCENTAGE\n Root directory: $ROOTDIR_STORAGE\n Home directory: $HOMEDIR_STORAGE\n"
+fi
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/update_workspace.sh b/jsk_fetch_robot/jsk_fetch_startup/scripts/update_workspace.sh
index f27e401366..5646eb2875 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/scripts/update_workspace.sh
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/update_workspace.sh
@@ -1,43 +1,5 @@
#!/usr/bin/env bash
-. $HOME/ros/melodic/devel/setup.bash
-# Filename should end with .txt to preview the contents in a web browser
-LOGFILE=$HOME/ros/melodic/update_workspace.txt
-
-{
-set -x
-# Update workspace
-cd $HOME/ros/melodic/src
-ln -sf $(rospack find jsk_fetch_startup)/../jsk_fetch.rosinstall.$ROS_DISTRO $HOME/ros/melodic/src/.rosinstall
-wstool foreach --git 'git stash'
-wstool update --delete-changed-uris
-WSTOOL_UPDATE_RESULT=$?
-cd $HOME/ros/melodic
-catkin clean aques_talk collada_urdf_jsk_patch -y
-catkin init
-catkin config -DCMAKE_BUILD_TYPE=Release
-catkin build
-CATKIN_BUILD_RESULT=$?
-# Send mail
-MAIL_BODY=""
-if [ $WSTOOL_UPDATE_RESULT -ne 0 ]; then
- MAIL_BODY=$MAIL_BODY"Please wstool update workspace manually. "
-fi
-if [ $CATKIN_BUILD_RESULT -ne 0 ]; then
- MAIL_BODY=$MAIL_BODY"Please catkin build workspace manually."
-fi
-set +x
-} > $LOGFILE 2>&1
-if [ -n "$MAIL_BODY" ]; then
- rostopic pub -1 /email jsk_robot_startup/Email "header:
- seq: 0
- stamp: {secs: 0, nsecs: 0}
- frame_id: ''
-subject: 'Daily workspace update fails'
-body: '$MAIL_BODY'
-sender_address: '$(hostname)@jsk.imi.i.u-tokyo.ac.jp'
-receiver_address: 'fetch@jsk.imi.i.u-tokyo.ac.jp'
-smtp_server: ''
-smtp_port: ''
-attached_files: ['$LOGFILE']"
-fi
+cp $(rospack find jsk_fetch_startup)/scripts/update_workspace_main.sh /tmp/update_workspace.sh
+/tmp/update_workspace.sh $@
+rm /tmp/update_workspace.sh
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/update_workspace_main.sh b/jsk_fetch_robot/jsk_fetch_startup/scripts/update_workspace_main.sh
new file mode 100755
index 0000000000..74eb47693b
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/update_workspace_main.sh
@@ -0,0 +1,113 @@
+#!/usr/bin/env bash
+
+function usage()
+{
+ echo "Usage: $0 [-w workspace_directory] [-h] [-l]
+
+optional arguments:
+ -h show this help
+ -w WORKSPACE_PATH specify target workspace
+ -l do not send a mail
+"
+}
+
+function get_full_path()
+{
+ echo "$(cd $(dirname $1) && pwd)/$(basename $1)"
+}
+
+SEND_MAIL=true
+WORKSPACE=$(get_full_path $HOME/ros/melodic)
+
+while getopts hlw: OPT
+do
+ case $OPT in
+ w)
+ WORKSPACE=$(get_full_path $OPTARG)
+ ;;
+ l)
+ SEND_MAIL=false
+ ;;
+ h)
+ usage
+ exit 1
+ ;;
+ *)
+ usage
+ exit 1
+ ;;
+ esac
+done
+
+if [ ! -e $WORKSPACE ]; then
+ echo "No workspace $WORKSPACE"
+ exit 1
+fi
+
+. $WORKSPACE/devel/setup.bash
+# Filename should end with .txt to preview the contents in a web browser
+LOGFILE=$WORKSPACE/update_workspace.txt
+
+{
+set -x
+# Update workspace
+
+wstool foreach -t $WORKSPACE/src --git 'git stash'
+wstool foreach -t $WORKSPACE/src --git 'git fetch origin --prune'
+wstool update -t $WORKSPACE/src jsk-ros-pkg/jsk_robot
+ln -sf $(rospack find jsk_fetch_startup)/../jsk_fetch.rosinstall.$ROS_DISTRO $WORKSPACE/src/.rosinstall
+wstool update -t $WORKSPACE/src --delete-changed-uris
+# Forcefully checkout specified branch
+wstool foreach -t $WORKSPACE/src --git --shell 'branchname=$(git rev-parse --abbrev-ref HEAD); if [ $branchname != "HEAD" ]; then git reset --hard HEAD; git checkout origin/$branchname; git branch -D $branchname; git checkout -b $branchname --track origin/$branchname; fi'
+wstool update -t $WORKSPACE/src
+WSTOOL_UPDATE_RESULT=$?
+# Rosdep Install
+sudo apt-get update -y
+rosdep update
+# jsk_footstep_planner is not released for melodic
+# jsk_footstep_controller is not released for melodic
+# librealsense2 should not be installed from ROS repository
+# realsense-ros should not be installed from ROS repository
+rosdep install --from-paths $WORKSPACE/src --ignore-src -y -r --skip-keys \
+"\
+jsk_footstep_controller \
+jsk_footstep_planner \
+librealsense2 \
+realsense2_camera \
+realsense2_description \
+"
+ROSDEP_INSTALL_RESULT=$?
+# Build workspace
+cd $WORKSPACE
+catkin clean aques_talk collada_urdf_jsk_patch libcmt -y
+catkin init
+catkin config -DCMAKE_BUILD_TYPE=Release
+catkin build --continue-on-failure
+CATKIN_BUILD_RESULT=$?
+# Send mail
+MAIL_BODY=""
+if [ $WSTOOL_UPDATE_RESULT -ne 0 ]; then
+ MAIL_BODY=$MAIL_BODY"Please wstool update workspace manually. "
+fi
+if [ $ROSDEP_INSTALL_RESULT -ne 0 ]; then
+ MAIL_BODY=$MAIL_BODY"Please install dependencies manually. "
+fi
+if [ $CATKIN_BUILD_RESULT -ne 0 ]; then
+ MAIL_BODY=$MAIL_BODY"Please catkin build workspace manually."
+fi
+set +x
+} 2>&1 | tee $LOGFILE
+if [ -n "$MAIL_BODY" ] && [ "${SEND_MAIL}" == "true" ]; then
+ echo "Sent a mail"
+ rostopic pub -1 /email jsk_robot_startup/Email "header:
+ seq: 0
+ stamp: {secs: 0, nsecs: 0}
+ frame_id: ''
+subject: 'Daily workspace update fails'
+body: '$MAIL_BODY'
+sender_address: '$(hostname)@jsk.imi.i.u-tokyo.ac.jp'
+receiver_address: 'fetch@jsk.imi.i.u-tokyo.ac.jp'
+smtp_server: ''
+smtp_port: ''
+attached_files: ['$LOGFILE']"
+fi
diff --git a/jsk_fetch_robot/jsk_fetch_startup/scripts/warning.py b/jsk_fetch_robot/jsk_fetch_startup/scripts/warning.py
index 6591399caa..029e8b281c 100755
--- a/jsk_fetch_robot/jsk_fetch_startup/scripts/warning.py
+++ b/jsk_fetch_robot/jsk_fetch_startup/scripts/warning.py
@@ -9,7 +9,6 @@
from sound_play.libsoundplay import SoundClient
from actionlib_msgs.msg import GoalStatus, GoalStatusArray
-from diagnostic_msgs.msg import DiagnosticArray, DiagnosticStatus
from fetch_driver_msgs.msg import RobotState
from geometry_msgs.msg import Twist
from power_msgs.msg import BatteryState, BreakerState
@@ -36,40 +35,6 @@ def terminate_thread(thread):
ctypes.pythonapi.PyThreadState_SetAsyncExc(thread.ident, None)
raise SystemError("PyThreadState_SetAsyncExc failed")
-class DiagnosticsSpeakThread(threading.Thread):
- def __init__(self, error_status):
- threading.Thread.__init__(self)
- self.volume = rospy.get_param("~volume", 1.0)
- self.error_status = error_status
- self.start()
-
- def run(self):
- global sound
- for status in self.error_status:
- # ignore error status if the error already occured in the latest 10 minites
- if status.message in error_status_in_10_min:
- if rospy.Time.now().secs - error_status_in_10_min[status.message] < 600:
- continue
- else:
- error_status_in_10_min[status.message] = rospy.Time.now().secs
- else:
- error_status_in_10_min[status.message] = rospy.Time.now().secs
- # we can ignore "Joystick not open."
- if status.message == "Joystick not open." :
- continue
- if status.name == "supply_breaker" and status.message == "Disabled." :
- continue
- #
- text = "Error on {}, {}".format(status.name, status.message)
- rospy.loginfo(text)
- text = text.replace('_', ', ')
- sound.say(text, 'voice_kal_diphone', volume=self.volume)
- time.sleep(5)
-
- def stop(self):
- terminate_thread(self)
- self.join()
-
class Warning:
def __init__(self):
time.sleep(1)
@@ -77,22 +42,13 @@ def __init__(self):
self.battery_state_msgs = BatteryState()
self.twist_msgs = Twist()
#
- self.diagnostics_speak_thread = {}
self.auto_undocking = False
- self.diagnostics_list = []
- if rospy.get_param("~speak_warn", True):
- self.diagnostics_list.append(DiagnosticStatus.WARN)
- if rospy.get_param("~speak_error", True):
- self.diagnostics_list.append(DiagnosticStatus.ERROR)
- if rospy.get_param("~speak_stale", True):
- self.diagnostics_list.append(DiagnosticStatus.STALE)
#
self.base_breaker = rospy.ServiceProxy('base_breaker', BreakerCommand)
#
self.battery_sub = rospy.Subscriber("battery_state", BatteryState, self.battery_callback, queue_size = 1)
self.cmd_vel_sub = rospy.Subscriber("base_controller/command_unchecked", Twist, self.cmd_vel_callback, queue_size = 1)
self.robot_state_sub = rospy.Subscriber("robot_state", RobotState, self.robot_state_callback, queue_size = 1)
- self.diagnostics_status_sub = rospy.Subscriber("diagnostics", DiagnosticArray, self.diagnostics_status_callback, queue_size = 1)
self.undock_sub = rospy.Subscriber("/undock/status", GoalStatusArray, self.undock_status_callback)
#
self.cmd_vel_pub = rospy.Publisher("base_controller/command", Twist, queue_size=1)
@@ -118,8 +74,8 @@ def battery_callback(self, msg):
time.sleep(5)
def cmd_vel_callback(self, msg):
- ## warn when cmd_vel is issued while the robot is charning
- rospy.logdebug("cmd_vel : x:{} y:{} z:{}, battery.is_charning {}".format(msg.linear.x,msg.linear.y,msg.angular.z,self.battery_state_msgs.is_charging))
+ ## warn when cmd_vel is issued while the robot is charging
+ rospy.logdebug("cmd_vel : x:{} y:{} z:{}, battery.is_charging {}".format(msg.linear.x,msg.linear.y,msg.angular.z,self.battery_state_msgs.is_charging))
breaker_enabled = True
try:
breaker_status = filter(lambda n: n.name=='base_breaker', self.robot_state_msgs.breakers)[0]
@@ -140,29 +96,6 @@ def cmd_vel_callback(self, msg):
##
self.twist_msgs = msg
- def diagnostics_status_callback(self, msg):
- ##
- self.diagnostic_status_msgs = msg.status
- ##
- ## check if this comes from /robot_driver
- callerid = msg._connection_header['callerid']
- if callerid not in self.diagnostics_speak_thread:
- self.diagnostics_speak_thread[callerid] = None
- error_status = filter(lambda n: n.level in self.diagnostics_list, msg.status)
- # when RunStopped, ignore message from *_mcb and *_breaker
- if self.robot_state_msgs.runstopped:
- error_status = filter(lambda n: not (re.match("\w*_(mcb|breaker)",n.name) or (n.name == "Mainboard" and n.message == "Runstop pressed")), error_status)
- if not error_status : # error_status is not []
- if self.diagnostics_speak_thread[callerid] and self.diagnostics_speak_thread[callerid].is_alive():
- self.diagnostics_speak_thread[callerid].stop()
- return
- # make sure that diagnostics_speak_thread is None, when the thread is terminated
- if self.diagnostics_speak_thread[callerid] and not self.diagnostics_speak_thread[callerid].is_alive():
- self.diagnostics_speak_thread[callerid] = None
- # run new thread
- if self.diagnostics_speak_thread[callerid] is None:
- self.diagnostics_speak_thread[callerid] = DiagnosticsSpeakThread(error_status)
-
if __name__ == "__main__":
global sound
# store error status and time of the error in the latest 10 minites
diff --git a/jsk_fetch_robot/jsk_fetch_startup/src/trashbins_pose_estimator.cpp b/jsk_fetch_robot/jsk_fetch_startup/src/trashbins_pose_estimator.cpp
new file mode 100644
index 0000000000..0adecd9ffb
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/src/trashbins_pose_estimator.cpp
@@ -0,0 +1,82 @@
+#include
+
+#include
+#include
+#include
+#include
+#include
+#include
+#include
+
+class TrashbinsPoseEstimator{
+private:
+ double _trashbinL, _trashbinW, _trashbinH; // trashbin size param
+ ros::Subscriber _trashbinHandleSub;
+ ros::Subscriber _pointCloud;
+ ros::Publisher _virtualTrashbinContainerPub;
+ jsk_recognition_msgs::BoundingBoxArray _trashbins; // trashbin object
+ geometry_msgs::Quaternion defaultOrientation;
+
+public:
+ // constructor
+ TrashbinsPoseEstimator(ros::NodeHandle &_nh, ros::NodeHandle &_pnh){
+ _pnh.getParam("trashbin_l", this->_trashbinL);
+ _pnh.getParam("trashbin_w", this->_trashbinW);
+ _pnh.getParam("trashbin_h", this->_trashbinH);
+ this->_trashbinHandleSub = _nh.subscribe("input", 10, &TrashbinsPoseEstimator::DrawVirtualTrashbinContainerCB, this);
+ this->_virtualTrashbinContainerPub = _nh.advertise("output", 10);
+ // init default orientation const
+ this->defaultOrientation.w = 1.0;
+ this->defaultOrientation.x = 0.0;
+ this->defaultOrientation.y = 0.0;
+ this->defaultOrientation.z = 0.0;
+ };
+ // destructor
+ ~TrashbinsPoseEstimator(){
+ ROS_INFO("Killing node...");
+ };
+
+ // draw virtual trashbin container and publish for debugging
+ void DrawVirtualTrashbinContainerCB(const jsk_recognition_msgs::BoundingBoxArray& msg){
+ boost::shared_ptr handles(new jsk_recognition_msgs::BoundingBoxArray), trashbins(new jsk_recognition_msgs::BoundingBoxArray);
+ boost::shared_ptr handle(new jsk_recognition_msgs::BoundingBox), trashbin(new jsk_recognition_msgs::BoundingBox);
+ boost::shared_ptr handleCenterPosition(new geometry_msgs::Point), trashbinCenterPosition(new geometry_msgs::Point);
+
+ if (msg.boxes.empty()) {
+ ROS_DEBUG("No handle candidates");
+ } else {
+ ROS_DEBUG_STREAM(msg.boxes.size() << " handle candidates" << std::endl);
+ *handles = msg;
+ for (int i = 0; i < handles->boxes.size(); i++) {
+ *handle = handles->boxes.at(i);
+ // decide trashbin center position
+ *handleCenterPosition = handle->pose.position;
+ trashbinCenterPosition->x =
+ handleCenterPosition->x + this->_trashbinL / 2.0;
+ trashbinCenterPosition->y = handleCenterPosition->y;
+ trashbinCenterPosition->z =
+ handleCenterPosition->z - this->_trashbinH / 2.0;
+ // make BoundingBox trashbin
+ trashbin->header = handle->header;
+ trashbin->pose.position = *trashbinCenterPosition;
+ trashbin->pose.orientation = this->defaultOrientation;
+ trashbin->dimensions.x = this->_trashbinL;
+ trashbin->dimensions.y = this->_trashbinW;
+ trashbin->dimensions.z = this->_trashbinH;
+ trashbin->label = i;
+ trashbins->boxes.push_back(*trashbin);
+ }
+ trashbins->header = handles->header;
+ this->_virtualTrashbinContainerPub.publish(*trashbins);
+ this->_trashbins = *trashbins;
+ }
+ }
+};
+
+int main(int argc, char **argv){
+ ros::init(argc, argv, "trashbin_pose_estimator");
+ ros::NodeHandle nh;
+ ros::NodeHandle pnh("~");
+ TrashbinsPoseEstimator node = TrashbinsPoseEstimator(nh, pnh);
+ ros::spin();
+}
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-app-scheduler.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-app-scheduler.conf
index 57944374f6..23dcfcb3be 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-app-scheduler.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-app-scheduler.conf
@@ -1,8 +1,9 @@
[program:jsk-app-scheduler]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup wait_app_manager.bash && roslaunch app_scheduler app_scheduler.launch yaml_path:=/var/lib/robot/app_schedule.yaml --wait --screen"
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup wait_app_manager.bash && roslaunch app_scheduler app_scheduler.launch yaml_path:=/var/lib/robot/app_schedule.yaml --wait --screen"
stopsignal=TERM
directory=/home/fetch/ros/melodic
-autostart=true
+; autostart=true
+autostart=false
autorestart=false
stdout_logfile=/var/log/ros/jsk-app-scheduler.log
stderr_logfile=/var/log/ros/jsk-app-scheduler.log
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-coral.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-coral.conf
deleted file mode 100644
index d7cf1778cf..0000000000
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-coral.conf
+++ /dev/null
@@ -1,11 +0,0 @@
-[program:jsk-coral]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/coral_ws/devel/setup.bash && roslaunch coral_usb edgetpu_object_detector.launch INPUT_IMAGE:=/head_camera/rgb/image_rect_color model_file:=/home/fetch/.ros/data/coral_usb/mobilenet_v2_ssd_73b2_kitchen_finetuning_20200528.tflite label_file:=/home/fetch/.ros/data/coral_usb/73b2_kitchen_labels.txt --screen --wait"
-stopsignal=TERM
-directory=/home/fetch/ros/coral_ws
-autostart=true
-autorestart=false
-stdout_logfile=/var/log/ros/jsk-coral.log
-stderr_logfile=/var/log/ros/jsk-coral.log
-user=fetch
-environment=ROSCONSOLE_FORMAT="[${severity}] [${time}] [${node}:${logger}]: ${message}",PYTHONUNBUFFERED=1
-priority=200
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-dialog.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-dialog.conf
index 581d4d8f87..50db8b9ed5 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-dialog.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-dialog.conf
@@ -1,11 +1,12 @@
[program:jsk-dialog]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup wait_app_manager.bash && roslaunch dialogflow_task_executive dialogflow_task_executive.launch run_app_manager:=false --wait --screen"
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup wait_app_manager.bash && roslaunch jsk_fetch_startup fetch_dialogflow_task_executive.launch run_app_manager:=false webhook_server:=true hostname:=$(hostname) --wait --screen "
stopsignal=TERM
directory=/home/fetch/ros/melodic
-autostart=true
+; autostart=true
+autostart=false
autorestart=false
stdout_logfile=/var/log/ros/jsk-dialog.log
stderr_logfile=/var/log/ros/jsk-dialog.log
user=fetch
-environment=ROSCONSOLE_FORMAT="[${severity}] [${time}] [${node}:${logger}]: ${message}",GOOGLE_APPLICATION_CREDENTIALS=/var/lib/robot/dialogflow/JSK-Fetch-a384d3498680.json,DIALOGFLOW_PROJECT_ID=fetch-kiedno,PYTHONUNBUFFERED=1
+environment=ROSCONSOLE_FORMAT="[${severity}] [${time}] [${node}:${logger}]: ${message}",GOOGLE_APPLICATION_CREDENTIALS=/var/lib/robot/dialogflow/fetch-credential.json,DIALOGFLOW_PROJECT_ID=fetch-kiedno,PYTHONUNBUFFERED=1
priority=200
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-dstat.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-dstat.conf
new file mode 100644
index 0000000000..4fcf56a3e3
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-dstat.conf
@@ -0,0 +1,11 @@
+; Install dstat
+; sudo apt install dstat
+[program:jsk-dstat]
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup dstat.bash"
+stopsignal=TERM
+autostart=true
+autorestart=false
+stdout_logfile=/var/log/ros/jsk-dstat.log
+stderr_logfile=/var/log/ros/jsk-dstat.log
+user=root
+priority=1
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-fetch-startup-outside.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-fetch-startup-outside.conf
new file mode 100644
index 0000000000..463ddf138e
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-fetch-startup-outside.conf
@@ -0,0 +1,11 @@
+[program:jsk-fetch-startup-outside]
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config_outside.bash ];then . /var/lib/robot/config_outside.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup setup_audio.bash && roslaunch jsk_fetch_startup fetch_bringup.launch hostname:=$(hostname) boot_sound:=true default_speaker:=$DEFAULT_SPEAKER default_english_speaker:=$DEFAULT_ENGLISH_SPEAKER default_warning_speaker:=$DEFAULT_WARNING_SPEAKER network_interface:=$NETWORK_DEFAULT_WIFI_INTERFACE --wait"
+stopsignal=TERM
+directory=/home/fetch/ros/melodic
+autostart=false
+autorestart=false
+stdout_logfile=/var/log/ros/jsk-fetch-startup.log
+stderr_logfile=/var/log/ros/jsk-fetch-startup.log
+user=fetch
+environment=ROSCONSOLE_FORMAT="[${severity}] [${time}] [${node}:${logger}]: ${message}",AUDIO_DEVICE="alsa_output.usb-1130_USB_AUDIO-00.analog-stereo",PYTHONUNBUFFERED=1
+priority=100
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-fetch-startup.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-fetch-startup.conf
index 5292717154..69eb29af34 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-fetch-startup.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-fetch-startup.conf
@@ -1,5 +1,5 @@
[program:jsk-fetch-startup]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup setup_audio.bash && roslaunch jsk_fetch_startup fetch_bringup.launch hostname:=$(hostname) boot_sound:=true use_voice_text:=false --wait"
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup setup_audio.bash && roslaunch jsk_fetch_startup fetch_bringup.launch hostname:=$(hostname) --wait"
stopsignal=TERM
directory=/home/fetch/ros/melodic
autostart=true
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-gdrive.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-gdrive.conf
index a79cc1deb3..dd8e281cda 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-gdrive.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-gdrive.conf
@@ -1,8 +1,9 @@
[program:jsk-gdrive]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && roslaunch gdrive_ros gdrive_server.launch --wait --screen"
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && roslaunch gdrive_ros gdrive_server.launch --wait --screen respawn:=true"
stopsignal=TERM
directory=/home/fetch/ros/melodic
-autostart=true
+; autostart=true
+autostart=false
autorestart=false
stdout_logfile=/var/log/ros/jsk-gdrive.log
stderr_logfile=/var/log/ros/jsk-gdrive.log
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-log-wifi.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-log-wifi.conf
index cdb54b8638..a9723545b8 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-log-wifi.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-log-wifi.conf
@@ -1,5 +1,5 @@
[program:jsk-log-wifi]
-command=/bin/bash -c ". /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup log-wifi-link.sh"
+command=/bin/bash -c ". /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && rosrun jsk_fetch_startup log-wifi-link.sh -I $NETWORK_DEFAULT_WIFI_INTERFACE"
stopsignal=TERM
autostart=true
autorestart=false
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-network-monitor-outside.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-network-monitor-outside.conf
new file mode 100644
index 0000000000..2dbd597ae2
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-network-monitor-outside.conf
@@ -0,0 +1,10 @@
+[program:jsk-network-monitor-outside]
+command=/bin/bash -c ". /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config_outside.bash ];then . /var/lib/robot/config_outside.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup network_monitor.py --interface $NETWORK_DEFAULT_WIFI_INTERFACE --profile $NETWORK_DEFAULT_PROFILE_ID"
+stopsignal=TERM
+autostart=false
+autorestart=false
+stdout_logfile=/var/log/ros/jsk-network-monitor.log
+stderr_logfile=/var/log/ros/jsk-network-monitor.log
+user=root
+environment=ROSCONSOLE_FORMAT="[${severity}] [${time}] [${node}:${logger}]: ${message}",NETWORK_MONITOR_HOSTNAMES="google.com",PYTHONUNBUFFERED=1
+priority=200
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-network-monitor.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-network-monitor.conf
index bf05c87a6b..30e1578f91 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-network-monitor.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-network-monitor.conf
@@ -1,5 +1,5 @@
[program:jsk-network-monitor]
-command=/bin/bash -c ". /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup network_monitor.py"
+command=/bin/bash -c ". /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup network_monitor.py --interface $NETWORK_DEFAULT_WIFI_INTERFACE --profile $NETWORK_DEFAULT_PROFILE_ID"
stopsignal=TERM
autostart=true
autorestart=false
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-rfcomm-bind.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-rfcomm-bind.conf
index 16f10c4710..b80954f2ab 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-rfcomm-bind.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-rfcomm-bind.conf
@@ -1,5 +1,5 @@
[program:jsk-rfcomm-bind]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && roslaunch jsk_robot_startup rfcomm_bind.launch --wait"
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && roslaunch jsk_robot_startup rfcomm_bind.launch --wait"
stopsignal=TERM
directory=/home/fetch/ros/melodic
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-shutdown.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-shutdown.conf
index bdc0bd78eb..37feca3d06 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-shutdown.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/jsk-shutdown.conf
@@ -1,5 +1,5 @@
[program:jsk-shutdown]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_robot_startup shutdown.py --wait"
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_robot_startup shutdown.py ~input:=/battery_state _input_condition:='m.is_charging is False' --wait"
stopsignal=TERM
directory=/home/fetch/ros/melodic
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/robot-outside.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/robot-outside.conf
new file mode 100644
index 0000000000..90f32f5811
--- /dev/null
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/robot-outside.conf
@@ -0,0 +1,11 @@
+[program:robot-outside]
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config_outside.bash ];then . /var/lib/robot/config_outside.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup link_calibration_files.bash && roslaunch jsk_fetch_startup fetch.launch launch_teleop:=false --wait --screen"
+stopsignal=TERM
+directory=/home/fetch/ros/melodic
+autostart=false
+autorestart=unexpected
+stdout_logfile=/var/log/ros/robot.log
+stderr_logfile=/var/log/ros/robot.log
+user=ros
+environment=ROS_LOG_DIR=/var/log/ros,ROSCONSOLE_FORMAT="[${severity}] [${time}] [${node}:${logger}]: ${message}",FETCH_CALIBRATED_URDF=/etc/ros/melodic/calibrated_2020_12_04_02_08_34.urdf,FETCH_CALIBRATED_RGB=/etc/ros/melodic/rgb_2020_12_04_02_08_34.yaml,FETCH_CALIBRATED_DEPTH=/etc/ros/melodic/depth_2020_12_04_02_08_34.yaml,PYTHONUNBUFFERED=1
+priority=10
diff --git a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/robot.conf b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/robot.conf
index d265c59b0d..0b33af1409 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/robot.conf
+++ b/jsk_fetch_robot/jsk_fetch_startup/supervisor_scripts/robot.conf
@@ -1,5 +1,5 @@
[program:robot]
-command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && rosrun jsk_fetch_startup link_calibration_files.bash && roslaunch jsk_fetch_startup fetch.launch launch_teleop:=false --wait --screen"
+command=/bin/bash -c ". /opt/ros/roscore_poststart.bash && . /home/fetch/ros/melodic/devel/setup.bash && if [ -e /var/lib/robot/config.bash ];then . /var/lib/robot/config.bash ;fi && export ROS_MASTER_URI=http://localhost:11311 && rossetclient $NETWORK_DEFAULT_ROS_INTERFACE && rosrun jsk_fetch_startup link_calibration_files.bash && roslaunch jsk_fetch_startup fetch.launch launch_teleop:=false --wait --screen"
stopsignal=TERM
directory=/home/fetch/ros/melodic
autostart=true
diff --git a/jsk_fetch_robot/jsk_fetch_startup/tmuxinator_yaml/log.yml b/jsk_fetch_robot/jsk_fetch_startup/tmuxinator_yaml/log.yml
index 5f2e1d51bf..85062f575d 100644
--- a/jsk_fetch_robot/jsk_fetch_startup/tmuxinator_yaml/log.yml
+++ b/jsk_fetch_robot/jsk_fetch_startup/tmuxinator_yaml/log.yml
@@ -36,9 +36,14 @@ windows:
- jsk-fetch-startup: tail -f -n 1000 /var/log/ros/jsk-fetch-startup.log
- jsk-network-monitor: tail -f -n 1000 /var/log/ros/jsk-network-monitor.log
- jsk-log-wifi: tail -f -n 1000 /var/log/ros/jsk-log-wifi.log
- - jsk-app-scheduler: tail -f -n 1000 /var/log/ros/jsk-app-scheduler.log
- - jsk-coral: tail -f -n 1000 /var/log/ros/jsk-coral.log
- - jsk-dialog: tail -f -n 1000 /var/log/ros/jsk-dialog.log
- - jsk-gdrive: tail -f -n 1000 /var/log/ros/jsk-gdrive.log
- - jsk-shutdown: tail -f -n 1000 /var/log/ros/jsk-shutdown.log
- - jsk-rfcomm-bind: tail -f -n 1000 /var/log/ros/jsk-rfcomm-bind.log
+ - jsk-dstat: tail -f -n 1000 /var/log/ros/jsk-dstat.log
+ # - jsk-app-scheduler: tail -f -n 1000 /var/log/ros/jsk-app-scheduler.log
+ # - jsk-coral: tail -f -n 1000 /var/log/ros/jsk-coral.log
+ # - jsk-object-detector: tail -f -n 1000 /var/log/ros/jsk-object-detector.log
+ # - jsk-panorama-object-detector: tail -f -n 1000 /var/log/ros/jsk-panorama-object-detector.log
+ # - jsk-human-pose-estimator: tail -f -n 1000 /var/log/ros/jsk-human-pose-estimator.log
+ # - jsk-panorama-human-pose-estimator: tail -f -n 1000 /var/log/ros/jsk-panorama-human-pose-estimator.log
+ # - jsk-dialog: tail -f -n 1000 /var/log/ros/jsk-dialog.log
+ # - jsk-gdrive: tail -f -n 1000 /var/log/ros/jsk-gdrive.log
+ # - jsk-shutdown: tail -f -n 1000 /var/log/ros/jsk-shutdown.log
+ # - jsk-rfcomm-bind: tail -f -n 1000 /var/log/ros/jsk-rfcomm-bind.log
diff --git a/jsk_robot_common/jsk_robot_startup/apps/robot_apps.installed b/jsk_robot_common/jsk_robot_startup/apps/robot_apps.installed
index e142d9282c..378c08693f 100644
--- a/jsk_robot_common/jsk_robot_startup/apps/robot_apps.installed
+++ b/jsk_robot_common/jsk_robot_startup/apps/robot_apps.installed
@@ -3,3 +3,7 @@ apps:
display: Personal use
- app: jsk_robot_startup/check_use_sim_time
display: Check /use_sim_time param
+ - app: jsk_robot_startup/roseus_resume_interrupt
+ display: Interrupt
+ - app: jsk_robot_startup/roseus_resume_resume
+ display: Resume
diff --git a/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.app b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.app
new file mode 100644
index 0000000000..d6ea2dad0f
--- /dev/null
+++ b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.app
@@ -0,0 +1,6 @@
+display: Interrupt
+platform: all
+run: rostopic/rostopic
+run_args: "pub -1 /roseus_resume/interrupt std_msgs/Empty"
+interface: jsk_robot_startup/roseus_resume_interrupt.interface
+icon: jsk_robot_startup/roseus_resume_interrupt.png
diff --git a/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.interface b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.interface
new file mode 100644
index 0000000000..044105d644
--- /dev/null
+++ b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.interface
@@ -0,0 +1,2 @@
+published_topics: {}
+subscribed_topics: {}
diff --git a/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.png b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.png
new file mode 100644
index 0000000000..352d08ee47
Binary files /dev/null and b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_interrupt/roseus_resume_interrupt.png differ
diff --git a/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.app b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.app
new file mode 100644
index 0000000000..c21b0d3a49
--- /dev/null
+++ b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.app
@@ -0,0 +1,6 @@
+display: Resume
+platform: all
+run: rostopic/rostopic
+run_args: "pub -1 /roseus_resume/resume std_msgs/Empty"
+interface: jsk_robot_startup/roseus_resume_resume.interface
+icon: jsk_robot_startup/roseus_resume_resume.png
diff --git a/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.interface b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.interface
new file mode 100644
index 0000000000..044105d644
--- /dev/null
+++ b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.interface
@@ -0,0 +1,2 @@
+published_topics: {}
+subscribed_topics: {}
diff --git a/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.png b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.png
new file mode 100644
index 0000000000..235869778e
Binary files /dev/null and b/jsk_robot_common/jsk_robot_startup/apps/roseus_resume_resume/roseus_resume_resume.png differ
diff --git a/jsk_robot_common/jsk_robot_startup/lifelog/app_manager.launch b/jsk_robot_common/jsk_robot_startup/lifelog/app_manager.launch
index f1f90dc1e3..1e7a5be105 100644
--- a/jsk_robot_common/jsk_robot_startup/lifelog/app_manager.launch
+++ b/jsk_robot_common/jsk_robot_startup/lifelog/app_manager.launch
@@ -2,6 +2,8 @@
+
+
@@ -44,6 +46,7 @@
delay_between_messages: 0
max_message_size: None
fragment_timeout: 600
+ unregister_timeout: 100000000
+
+
diff --git a/jsk_robot_common/jsk_robot_startup/lifelog/mongodb.launch b/jsk_robot_common/jsk_robot_startup/lifelog/mongodb.launch
index 13c62d42f4..e7b6b684d8 100644
--- a/jsk_robot_common/jsk_robot_startup/lifelog/mongodb.launch
+++ b/jsk_robot_common/jsk_robot_startup/lifelog/mongodb.launch
@@ -34,6 +34,7 @@
+
diff --git a/jsk_robot_common/jsk_robot_startup/lifelog/tweet.launch b/jsk_robot_common/jsk_robot_startup/lifelog/tweet.launch
index b22b0d066c..827bef6832 100644
--- a/jsk_robot_common/jsk_robot_startup/lifelog/tweet.launch
+++ b/jsk_robot_common/jsk_robot_startup/lifelog/tweet.launch
@@ -27,12 +27,21 @@
output="$(arg output)" respawn="true" >
+
+
+ account_info: $(arg account_info)
+
+
+
+
+