diff --git a/.github/workflows/coolshapes.js.yml b/.github/workflows/coolshapes.js.yml
index 8c106e8..912190f 100644
--- a/.github/workflows/coolshapes.js.yml
+++ b/.github/workflows/coolshapes.js.yml
@@ -12,7 +12,7 @@ jobs:
steps:
- uses: actions/checkout@v3
- - name: Builds and t
+ - name: Builds and test
uses: actions/setup-node@v3
with:
node-version: 16.x
diff --git a/.github/workflows/release.js.yml b/.github/workflows/release.js.yml
new file mode 100644
index 0000000..3ddc44e
--- /dev/null
+++ b/.github/workflows/release.js.yml
@@ -0,0 +1,19 @@
+name: Publish Package
+on:
+ release:
+ types: [published]
+jobs:
+ build:
+ runs-on: ubuntu-latest
+ steps:
+ - uses: actions/checkout@v4
+ - uses: actions/setup-node@v4
+ with:
+ node-version: '20.x'
+ registry-url: 'https://registry.npmjs.org'
+ - run: npm ci
+ - run: npm run test
+ - run: npm run build
+ - run: npm publish
+ env:
+ NODE_AUTH_TOKEN: ${{ secrets.NPM_TOKEN }}
\ No newline at end of file
diff --git a/.gitignore b/.gitignore
index fd10abd..4691766 100644
--- a/.gitignore
+++ b/.gitignore
@@ -1,3 +1,5 @@
.DS_Store
node_modules
dist/
+.idea
+*.env
\ No newline at end of file
diff --git a/README.md b/README.md
index 96f15c2..85c0248 100644
--- a/README.md
+++ b/README.md
@@ -1,35 +1,37 @@
-[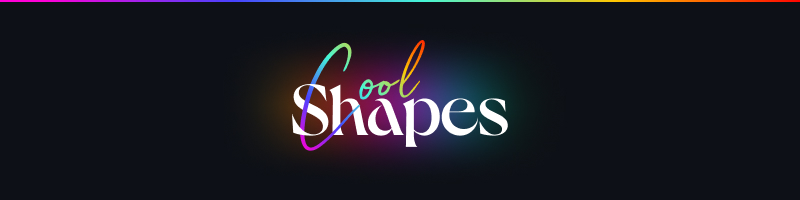](https://coolshap.es)
+
#
Coolshapes
-
-A simple, fun project for the sake of creating some cool-looking abstract shapes with little grainy gradients crafted by [@realvjy](https://x.com/realvjy). Coolshapes is a completely open-source set of 100+ abstract shapes crafted for any design and development projects. Free for both commercial and personal use. Licensed under MIT.
+A simple, fun project for the sake of creating some cool-looking abstract shapes with little grainy gradients crafted
+by [@realvjy](https://x.com/realvjy). Coolshapes is a completely open-source set of 100+ abstract shapes crafted for any
+design and development projects. Free for both commercial and personal use. Licensed under MIT.
### Table of Contents
- [Coolshapes](#coolshapes)
- - [Table of Contents](#table-of-contents)
- - [Installation](#installation)
- - [How to use](#how-to-use)
- - [Global component example](#global-component-example)
- - [Component with shape category example](#component-with-shape-category-example)
- - [Generating random shapes](#generating-random-shapes)
- - [Using random shape function](#using-random-shape-function)
- - [Other Methods](#other-methods)
- - [Renders the shapes from all catagories](#renders-the-shapes-from-all-catagories)
- - [syntax](#syntax)
- - [Props](#props)
- - [Categories](#categories)
- - [Others](#others)
- - [cjs](#cjs)
- - [Figma File](#figma-file)
- - [Contributing](#contributing)
- - [Support \& Donation](#support--donation)
+ - [Table of Contents](#table-of-contents)
+ - [Installation](#installation)
+ - [How to use](#how-to-use)
+ - [Global component example](#global-component-example)
+ - [Shape category components example](#component-with-shape-category-example)
+ - [Generating random shapes](#generating-random-shapes)
+ - [Random shape function](#using-random-shape-function)
+ - [Other Methods](#other-methods)
+ - [Accessing the shapes object](#renders-the-shapes-from-all-catagories)
+ - [Props](#props)
+ - [Categories](#categories)
+ - [Others](#others)
+ - [Classname and css](#classnames-and-css)
+ - [Builds](#builds)
+ - [Figma File](#figma-file)
+ - [Credits](#credits)
+ - [Contributing](#contributing)
+ - [Support \& Donation](#support--donation)
## Installation
@@ -45,7 +47,8 @@ yarn add coolshapes-react
## How to use
-There are two types of components available in **Coolshapes**, which you can use: **Global component** and **Component with a category of shapes**. Then, you can pass additional [props](#props) to adjust the shapes and their properties available on Coolshapes SVG elements.
+There are two types of components available in **Coolshapes**, which you can use: **Global component** and **Component
+for each category of shapes**. and, you can pass additional [props](#props) to adjust the shapes.
#### Global component example
@@ -55,7 +58,7 @@ Just import the Global component `Coolshape` and it will work.
import { Coolshape } from "coolshapes-react";
const App = () => {
- return ;
+ return ;
};
export default App;
@@ -69,30 +72,29 @@ You can import the component for specific category - `Star`,`Ellipse`... etc - a
import { Star } from "coolshapes-react";
const App = () => {
- return ;
+ return ;
};
export default App;
```
-
#### Generating random shapes
-setting the `random` [prop](#props) to true or leaving the `index` or `type` prop empty would replace the shape with a random shape every time it renders.
+setting the `random` [prop](#props) to true or leaving the `index` or `type` prop empty would replace the shape with a
+random shape every time it renders.
```js
// renders a random shape from any category
const Component = () => {
- return ;
+ return ;
};
// renders a shape from the category star
const Component2 = () => {
- return ;
+ return ;
};
```
#### Using random shape function
-
```js
import { getRandomShape } from "coolshapes-react";
```
@@ -127,7 +129,7 @@ const shapes = {
}
```
-#### Renders the shapes from all catagories
+#### Renders the shapes from all categories
```jsx
import { shapes } from "coolshapes-react";
@@ -135,9 +137,10 @@ import { shapes } from "coolshapes-react";
const ShapeGrid = () => {
return (
<>
- {Object.keys(shapes).map((shapeType, _) => {
- return shapes[shapeType].map((Shape, index) => {
- return ;
+ {
+ Object.keys(shapes).map((shapeType, _) => {
+ return shapes[shapeType].map((Shape, index) => {
+ return ;
});
})}
>
@@ -158,44 +161,61 @@ const StarComponent1 = starComponents[0];
### Props
-| name | data type | default | description |
-| --------------------- | --------- | ------- | ------------------------------------------------------------------------------------------------------------------------------------------------ |
-| `size` | _Number_ | 200 | The dimension of shape |
-| [`type`](#categories) | _String_ | random | The category of shapes, if left empty it would randomly select a category. |
-| `noise` | _Boolean_ | true | Whether to add noise to the shape or not. |
-| `index` | _Number_ | random | The index of shape within the shape [category](#categories), it will randomly select a shape from the category if type prop given. Start from 0. |
-| `random` | _Boolean_ | false | If set true it would select a random component |
+| name | data type | default | description |
+|-----------------------|-----------|---------|----------------------------------------------------------------------------------------------------------------------------------------------------------|
+| `size` | _Number_ | 200 | The dimension of shape |
+| [`type`](#categories) | _String_ | random | The category of shapes, if left empty it will randomly select a category. |
+| `noise` | _Boolean_ | true | Whether to add noise to the shape or not. |
+| `index` | _Number_ | random | The index of shape within the shape [category](#categories), it would randomly select a shape from the category if type prop given. index starts from 0. |
+| `random` | _Boolean_ | false | If set true it will select a random component |
-**Notes:**
-Index starts from number 0, so if you want to retrive the first shape of any category, you would use the index number 0.
+#### notes
+> Index starts from number 0, so if you want to retrieve the first shape of any category, you would use the index number 0.
+> The shapes are SVG components behind the hood, so it's also supports all the props that can be passed in a svg element.
### Categories
There are a total of **115** shapes available in Coolshapes under the following categories.
-| name | count |
-| ------------- | -------- |
-| `star` | 13 |
-| `triangle` | 14 |
-| `moon` | 15 |
-| `polygon` | 8 |
-| `flower` | 16 |
-| `rectangle` | 9 |
-| `ellipse` | 12 |
-| `wheel` | 7 |
-| `misc` | 11 |
-| `number` | 10 |
-
-**Note:** When importing the components the name would be capitalized, but when passing as prop, the name would be lower case.
+| name | count |
+|-------------|-------|
+| `star` | 13 |
+| `triangle` | 14 |
+| `moon` | 15 |
+| `polygon` | 8 |
+| `flower` | 16 |
+| `rectangle` | 9 |
+| `ellipse` | 12 |
+| `wheel` | 7 |
+| `misc` | 11 |
+| `number` | 10 |
+
+**Note:** While passing the category name in the component, the name will be in lower case.
### Others
-#### Name & Class
-All components have inbuilt name starting 1. But, index starts from 0.
-*Example: `` when rendered it's component name and class name is `Star1`*
+#### ClassNames and css
-#### umd & es
-There are `umd` and `es` included in bundled version of the module,
+all the components have classname `coolshapes` assigned as default, but you can also pass custom classname of yours.
+```jsx
+import { Star } from 'coolshapes-react';
+
+export const Shape = () => {
+ return(
+
+ )
+}
+```
+```scss
+// make the shapes size responsive similar to img elements.
+.coolshapes{
+ width: 100%;
+ max-height: 48px;
+}
+```
+#### Builds
+
+We have given `umd`, `es` and `cjs` versions of the build.
#### cjs
@@ -203,40 +223,44 @@ There are `umd` and `es` included in bundled version of the module,
const Coolshapes = require("coolshapes-react");
```
-using with react in the browser
+Using with react in the browser
```html
-
-
-
-
-
-
-
-
-
-
-
+
+
+
+
+
+
+
+
+
+
+
```
+##### unpkg
+```
+https://unpkg.com/coolshapes-react/dist/umd/coolshapes.js
+```
## Figma File
Figma file coming soon on community
-## Contributing
-
-Created by realvjy. You are always welcome to share your feedback on twitter or any social media platform.
+## Credits
+Crafted by realvjy. You are always welcome to share your feedback on twitter or any other social media platform.
-If you want to contribute. Just create a [pull request](https://github.com/realvjy/coolshapes-react/pulls).
+## Contributing
+If you want to contribute, create a [pull request](https://github.com/realvjy/coolshapes-react/pulls).
## Support & Donation
diff --git a/package.json b/package.json
index fa16f81..8526178 100644
--- a/package.json
+++ b/package.json
@@ -40,7 +40,6 @@
{
"name": "0xgreenapple",
"url": "https://greenapple.one",
- "twitter": "https://x.com/0xgreenapple",
"email": "0xgreenapple@gmail.com"
}
],
diff --git a/tests/shape.test.tsx b/tests/shape.test.tsx
index 974cefc..590c96f 100644
--- a/tests/shape.test.tsx
+++ b/tests/shape.test.tsx
@@ -4,9 +4,9 @@ import { render } from "@testing-library/react";
import React, { ForwardRefExoticComponent } from "react";
import shapes, { componentId, getRandomShape, shapeTypes } from "../src/shapes";
import { ShapeType } from "../src/lib";
-import { Coolshape, Star } from "../src/lib/shapes";
+import { Coolshape, Star } from "../src";
-describe("using every icons from the defined component list", async () => {
+describe("using every icons from the defined component list", () => {
const shapeTypes = Object.keys(shapes) as Array;
shapeTypes.forEach((type) => {
shapes[type].forEach((Shape, i) => {