-
Notifications
You must be signed in to change notification settings - Fork 11
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add region handling of boundary points from arc (#61)
Inspired by @jekoie issue [#222](curtacircuitos/pcb-tools#222) from [pcb-tools](https://github.com/curtacircuitos/pcb-tools) repository > Below gerber file, why pcb-tools draw it in a wrong way? > > ``` > G04 This file illustrates how to use levels to create holes* > %FSLAX25Y25*% > %MOMM*% > G01* > G04 First level: big square - dark polarity* > %LPD*% > G36* > X250000Y250000D02* > X1750000D01* > Y1750000D01* > X250000D01* > Y250000D01* > G37* > G04 Second level: big circle - clear polarity* > %LPC*% > G36* > G75* > X500000Y1000000D02* > G03* > X500000Y1000000I500000J0D01* > G37* > G04 Third level: small square - dark polarity* > %LPD*% > G36* > X750000Y750000D02* > X1250000D01* > Y1250000D01* > X750000D01* > Y750000D01* > G37* > G04 Fourth level: small circle - clear polarity* > %LPC*% > G36* > G75* > X1150000Y1000000D02* > G03* > X1150000Y1000000I250000J0D01* > G37* > M02* > ``` > > It should be this: > 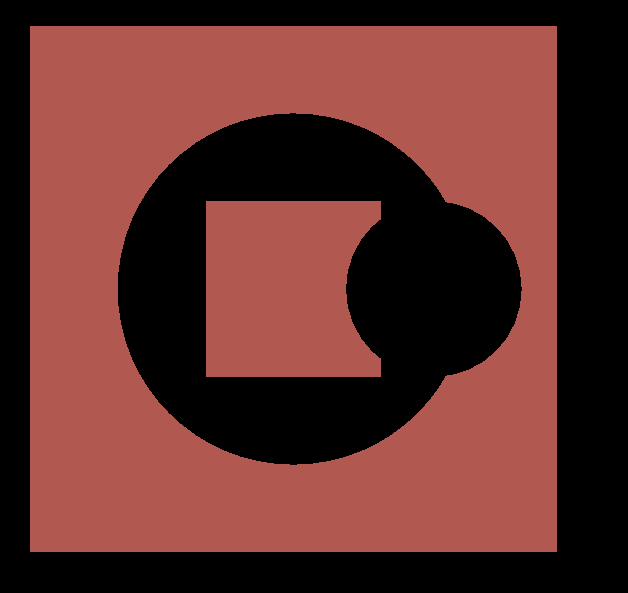 > > pcb-tools draw this: > 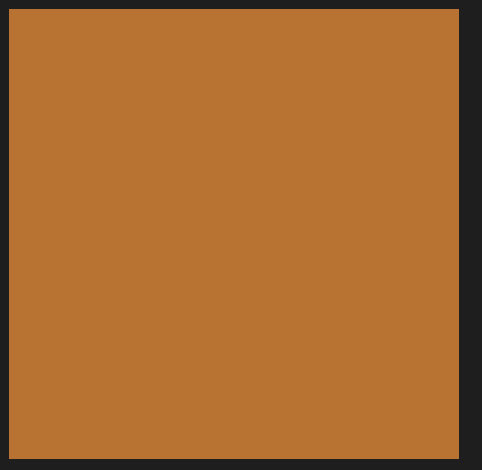 Above code mostly works, but required additional `G01` in before rectangle region creation: ``` G04 Third level: small square - dark polarity* G01* ``` Additionally, during development of this feature I might have revealed hidden issue with centering images with debug features enabled, further investigation is needed.
- Loading branch information
Showing
7 changed files
with
186 additions
and
67 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,10 +1,11 @@ | ||
"""Wrapper for plot operation token.""" | ||
from __future__ import annotations | ||
|
||
from typing import TYPE_CHECKING, Any, Iterable, Tuple | ||
from typing import TYPE_CHECKING, Any, Generator, Iterable, Tuple | ||
|
||
from pygerber.gerberx3.math.offset import Offset | ||
from pygerber.gerberx3.math.vector_2d import Vector2D | ||
from pygerber.gerberx3.state_enums import DrawMode | ||
from pygerber.gerberx3.state_enums import DrawMode, Polarity | ||
from pygerber.gerberx3.tokenizer.tokens.coordinate import Coordinate, CoordinateType | ||
from pygerber.gerberx3.tokenizer.tokens.token import Token | ||
|
||
|
@@ -55,39 +56,108 @@ def update_drawing_state( | |
start_position = state.current_position | ||
|
||
draw_commands: list[DrawCommand] = [] | ||
current_aperture = backend.get_private_aperture_handle( | ||
state.get_current_aperture(), | ||
) | ||
|
||
if state.is_region: | ||
polarity = state.polarity.to_region_variant() | ||
else: | ||
polarity = state.polarity | ||
|
||
draw_commands.append( | ||
backend.get_draw_paste_cls()( | ||
backend=backend, | ||
polarity=polarity, | ||
center_position=start_position, | ||
other=current_aperture.drawing_target, | ||
if not state.is_region or backend.options.draw_region_outlines: | ||
draw_commands.extend( | ||
self._create_draw_commands( | ||
state, | ||
backend, | ||
end_position, | ||
start_position, | ||
polarity, | ||
), | ||
) | ||
|
||
if state.is_region: | ||
self._create_region_points( | ||
state, | ||
backend, | ||
end_position, | ||
start_position, | ||
polarity, | ||
) | ||
|
||
return ( | ||
state.model_copy( | ||
update={ | ||
"current_position": end_position, | ||
}, | ||
), | ||
draw_commands, | ||
) | ||
|
||
def _create_region_points( # noqa: PLR0913 | ||
self, | ||
state: State, | ||
backend: Backend, | ||
end_position: Vector2D, | ||
start_position: Vector2D, | ||
polarity: Polarity, | ||
) -> None: | ||
if state.draw_mode == DrawMode.Linear: | ||
if state.is_region: | ||
state.region_boundary_points.append(start_position) | ||
state.region_boundary_points.append(end_position) | ||
state.region_boundary_points.append(start_position) | ||
state.region_boundary_points.append(end_position) | ||
|
||
draw_commands.append( | ||
backend.get_draw_vector_line_cls()( | ||
elif state.draw_mode in ( | ||
DrawMode.ClockwiseCircular, | ||
DrawMode.CounterclockwiseCircular, | ||
): | ||
i = state.parse_coordinate(self.i) | ||
j = state.parse_coordinate(self.j) | ||
|
||
center_offset = Vector2D(x=i, y=j) | ||
|
||
state.region_boundary_points.extend( | ||
backend.get_draw_arc_cls()( | ||
backend=backend, | ||
polarity=polarity, | ||
start_position=start_position, | ||
dx_dy_center=center_offset, | ||
end_position=end_position, | ||
width=current_aperture.get_line_width(), | ||
), | ||
width=Offset.NULL, | ||
is_clockwise=(state.draw_mode == DrawMode.ClockwiseCircular), | ||
# Will require tweaking if support for single quadrant mode | ||
# will be desired. | ||
is_multi_quadrant=True, | ||
).calculate_arc_points(), | ||
) | ||
|
||
else: | ||
raise NotImplementedError(state.draw_mode) | ||
|
||
def _create_draw_commands( # noqa: PLR0913 | ||
self, | ||
state: State, | ||
backend: Backend, | ||
end_position: Vector2D, | ||
start_position: Vector2D, | ||
polarity: Polarity, | ||
) -> Generator[DrawCommand, None, None]: | ||
current_aperture = backend.get_private_aperture_handle( | ||
state.get_current_aperture(), | ||
) | ||
yield backend.get_draw_paste_cls()( | ||
backend=backend, | ||
polarity=polarity, | ||
center_position=start_position, | ||
other=current_aperture.drawing_target, | ||
) | ||
|
||
if state.draw_mode == DrawMode.Linear: | ||
if not state.is_region or backend.options.draw_region_outlines: | ||
yield backend.get_draw_vector_line_cls()( | ||
backend=backend, | ||
polarity=polarity, | ||
start_position=start_position, | ||
end_position=end_position, | ||
width=current_aperture.get_line_width(), | ||
) | ||
|
||
elif state.draw_mode in ( | ||
DrawMode.ClockwiseCircular, | ||
DrawMode.CounterclockwiseCircular, | ||
|
@@ -96,15 +166,8 @@ def update_drawing_state( | |
j = state.parse_coordinate(self.j) | ||
|
||
center_offset = Vector2D(x=i, y=j) | ||
|
||
if state.is_region: | ||
state.region_boundary_points.append(start_position) | ||
# TODO([email protected]): Add region boundary points for region | ||
# https://github.com/Argmaster/pygerber/issues/29 | ||
state.region_boundary_points.append(end_position) | ||
|
||
draw_commands.append( | ||
backend.get_draw_arc_cls()( | ||
if not state.is_region or backend.options.draw_region_outlines: | ||
yield backend.get_draw_arc_cls()( | ||
backend=backend, | ||
polarity=polarity, | ||
start_position=start_position, | ||
|
@@ -115,38 +178,16 @@ def update_drawing_state( | |
# Will require tweaking if support for single quadrant mode | ||
# will be desired. | ||
is_multi_quadrant=True, | ||
), | ||
) | ||
) | ||
|
||
else: | ||
raise NotImplementedError(state.draw_mode) | ||
|
||
draw_commands.append( | ||
backend.get_draw_paste_cls()( | ||
backend=backend, | ||
polarity=polarity, | ||
center_position=end_position, | ||
other=current_aperture.drawing_target, | ||
), | ||
) | ||
|
||
if not state.is_region or backend.options.draw_region_outlines: | ||
return ( | ||
state.model_copy( | ||
update={ | ||
"current_position": end_position, | ||
}, | ||
), | ||
draw_commands, | ||
) | ||
|
||
return ( | ||
state.model_copy( | ||
update={ | ||
"current_position": end_position, | ||
}, | ||
), | ||
(), | ||
yield backend.get_draw_paste_cls()( | ||
backend=backend, | ||
polarity=polarity, | ||
center_position=end_position, | ||
other=current_aperture.drawing_target, | ||
) | ||
|
||
def __str__(self) -> str: | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
G04 This file illustrates how to use levels to create holes* | ||
%FSLAX25Y25*% | ||
%MOMM*% | ||
G01* | ||
G04 First level: big square - dark polarity* | ||
%LPD*% | ||
G36* | ||
X250000Y250000D02* | ||
X1750000D01* | ||
Y1750000D01* | ||
X250000D01* | ||
Y250000D01* | ||
G37* | ||
G04 Second level: big circle - clear polarity* | ||
%LPC*% | ||
G36* | ||
G75* | ||
X500000Y1000000D02* | ||
G03* | ||
X500000Y1000000I500000J0D01* | ||
G37* | ||
G04 Third level: small square - dark polarity* | ||
G01* | ||
%LPD*% | ||
G36* | ||
X750000Y750000D02* | ||
X1250000D01* | ||
Y1250000D01* | ||
X750000D01* | ||
Y750000D01* | ||
G37* | ||
G04 Fourth level: small circle - clear polarity* | ||
%LPC*% | ||
G36* | ||
G75* | ||
X1150000Y1000000D02* | ||
G03* | ||
X1150000Y1000000I250000J0D01* | ||
G37* | ||
M02* |
Binary file modified
BIN
+1.06 KB
(100%)
test/gerberx3/test_rasterized_2d/reference/basic/sample-2/source/image.png
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Binary file modified
BIN
+1.06 KB
(100%)
test/gerberx3/test_rasterized_2d/reference/basic/sample-2/source_b/image.png
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
"""Rasterized2D tests based on KicadGerberX2 board.""" | ||
|
||
from __future__ import annotations | ||
|
||
from test.gerberx3.test_rasterized_2d.common import make_rasterized_2d_test | ||
|
||
test_sample = make_rasterized_2d_test( | ||
__file__, | ||
"test/assets/gerberx3/pcb_tools_issues", | ||
dpi=1000, | ||
) |