forked from youngyangyang04/leetcode-master
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
5d7e028
commit 029cf01
Showing
18 changed files
with
643 additions
and
544 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,193 @@ | ||
|
||
|
||
# 84.柱状图中最大的矩形 | ||
|
||
链接:https://leetcode-cn.com/problems/largest-rectangle-in-histogram/ | ||
|
||
给定 n 个非负整数,用来表示柱状图中各个柱子的高度。每个柱子彼此相邻,且宽度为 1 。 | ||
|
||
求在该柱状图中,能够勾勒出来的矩形的最大面积。 | ||
|
||
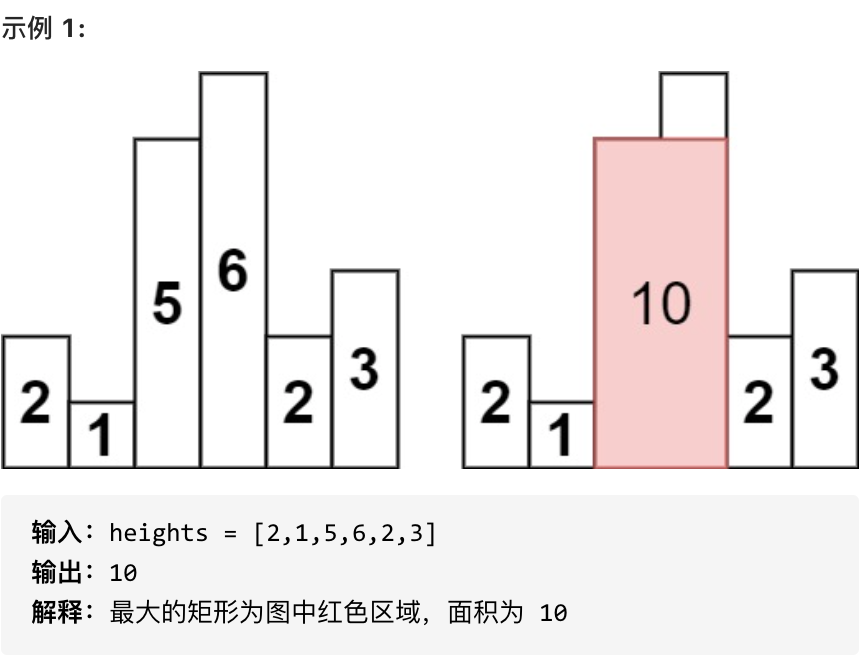 | ||
|
||
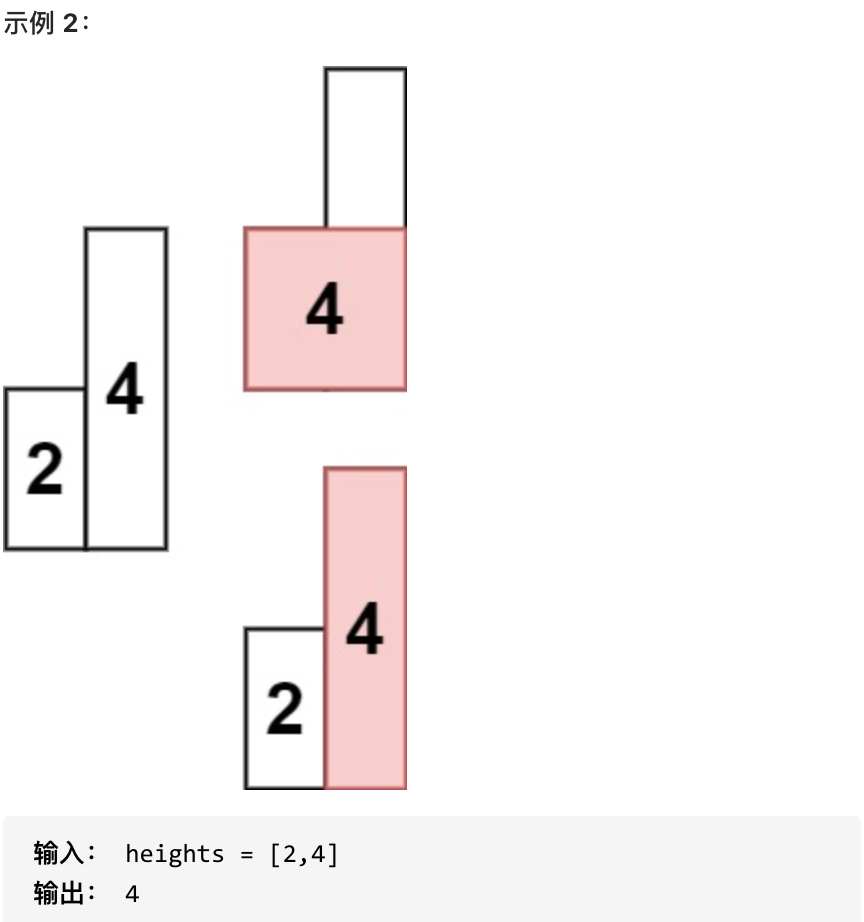 | ||
|
||
|
||
# 思路 | ||
|
||
本题和[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw),是遥相呼应的两道题目,建议都要仔细做一做,原理上有很多相同的地方,但细节上又有差异,更可以加深对单调栈的理解! | ||
|
||
其实这两道题目先做那一道都可以,但我先写的42.接雨水的题解,所以如果没做过接雨水的话,建议先做一做接雨水,可以参考我的题解:[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw) | ||
|
||
我们先来看一下双指针的解法: | ||
|
||
## 双指针解法 | ||
|
||
```C++ | ||
class Solution { | ||
public: | ||
int largestRectangleArea(vector<int>& heights) { | ||
int sum = 0; | ||
for (int i = 0; i < heights.size(); i++) { | ||
int left = i; | ||
int right = i; | ||
for (; left >= 0; left--) { | ||
if (heights[left] < heights[i]) break; | ||
} | ||
for (; right < heights.size(); right++) { | ||
if (heights[right] < heights[i]) break; | ||
} | ||
int w = right - left - 1; | ||
int h = heights[i]; | ||
sum = max(sum, w * h); | ||
} | ||
return sum; | ||
} | ||
}; | ||
``` | ||
如上代码并不能通过leetcode,超时了,因为时间复杂度是O(n^2)。 | ||
## 动态规划 | ||
本题动态规划的写法整体思路和[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw)是一致的,但要比[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw)难一些。 | ||
难就难在本题要记录记录每个柱子 左边第一个小于该柱子的下标,而不是左边第一个小于该柱子的高度。 | ||
所以需要循环查找,也就是下面在寻找的过程中使用了while,详细请看下面注释,整理思路在题解:[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw)中已经介绍了。 | ||
```C++ | ||
class Solution { | ||
public: | ||
int largestRectangleArea(vector<int>& heights) { | ||
vector<int> minLeftIndex(heights.size()); | ||
vector<int> minRightIndex(heights.size()); | ||
int size = heights.size(); | ||
// 记录每个柱子 左边第一个小于该柱子的下标 | ||
minLeftIndex[0] = -1; // 注意这里初始化,防止下面while死循环 | ||
for (int i = 1; i < size; i++) { | ||
int t = i - 1; | ||
// 这里不是用if,而是不断向左寻找的过程 | ||
while (t >= 0 && heights[t] >= heights[i]) t = minLeftIndex[t]; | ||
minLeftIndex[i] = t; | ||
} | ||
// 记录每个柱子 右边第一个小于该柱子的下标 | ||
minRightIndex[size - 1] = size; // 注意这里初始化,防止下面while死循环 | ||
for (int i = size - 2; i >= 0; i--) { | ||
int t = i + 1; | ||
// 这里不是用if,而是不断向右寻找的过程 | ||
while (t < size && heights[t] >= heights[i]) t = minRightIndex[t]; | ||
minRightIndex[i] = t; | ||
} | ||
// 求和 | ||
int result = 0; | ||
for (int i = 0; i < size; i++) { | ||
int sum = heights[i] * (minRightIndex[i] - minLeftIndex[i] - 1); | ||
result = max(sum, result); | ||
} | ||
return result; | ||
} | ||
}; | ||
``` | ||
|
||
## 单调栈 | ||
|
||
本地单调栈的解法和接雨水的题目是遥相呼应的。 | ||
|
||
为什么这么说呢,[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw)是找每个柱子左右两边第一个大于该柱子高度的柱子,而本题是找每个柱子左右两边第一个小于该柱子的柱子。 | ||
|
||
**这里就涉及到了单调栈很重要的性质,就是单调栈里的顺序,是从小到大还是从大到小**。 | ||
|
||
在题解[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw)中我讲解了接雨水的单调栈从栈头(元素从栈头弹出)到栈底的顺序应该是从小到大的顺序。 | ||
|
||
那么因为本题是要找每个柱子左右两边第一个小于该柱子的柱子,所以从栈头(元素从栈头弹出)到栈底的顺序应该是从大到小的顺序! | ||
|
||
我来举一个例子,如图: | ||
|
||
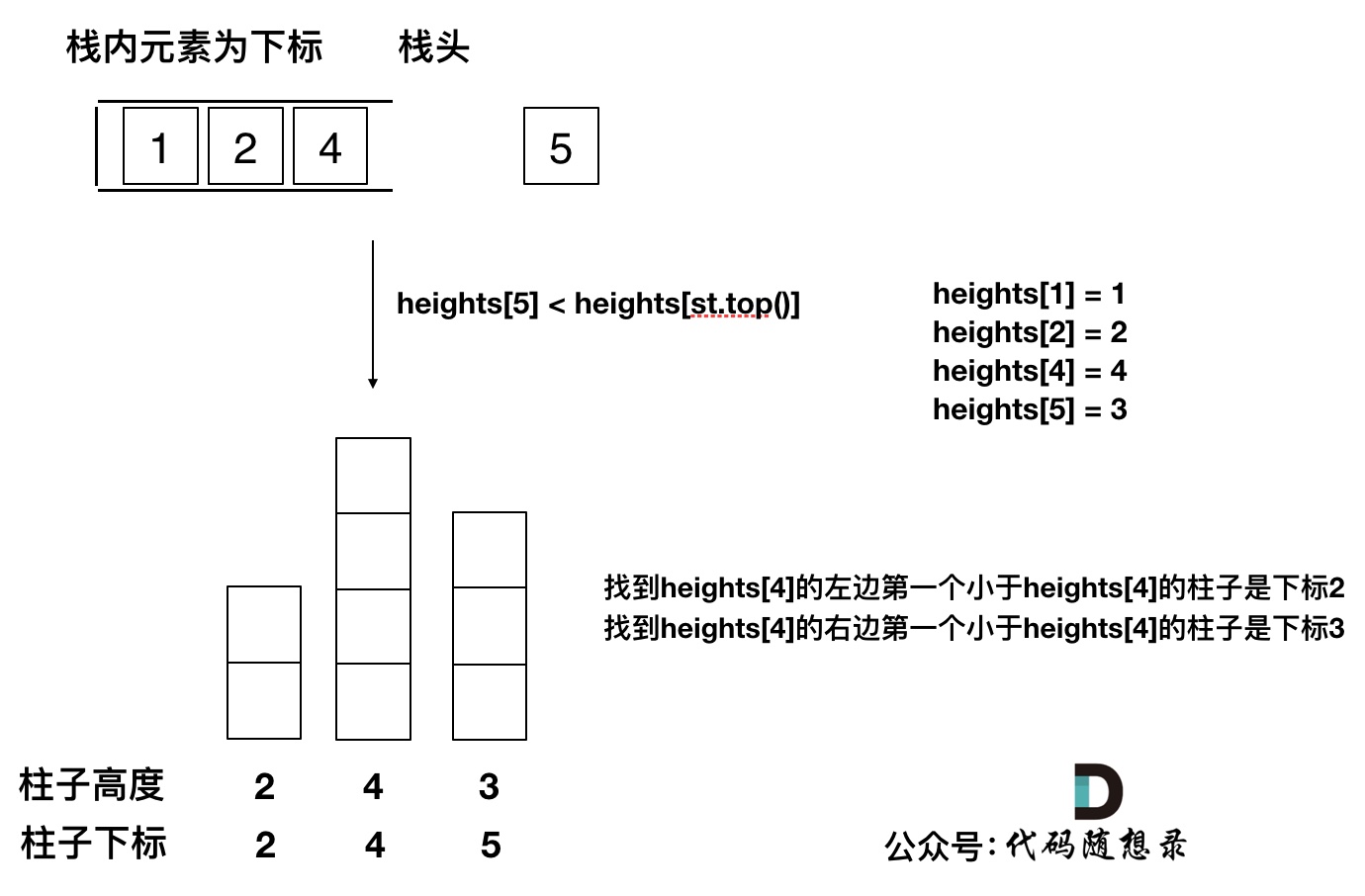 | ||
|
||
只有栈里从大到小的顺序,才能保证栈顶元素找到左右两边第一个小于栈顶元素的柱子。 | ||
|
||
所以本题单调栈的顺序正好与接雨水反过来。 | ||
|
||
此时大家应该可以发现其实就是**栈顶和栈顶的下一个元素以及要入栈的三个元素组成了我们要求最大面积的高度和宽度** | ||
|
||
理解这一点,对单调栈就掌握的比较到位了。 | ||
|
||
除了栈内元素顺序和接雨水不同,剩下的逻辑就都差不多了,在题解[42. 接雨水](https://mp.weixin.qq.com/s/QogENxhotboct9wn7GgYUw)我已经对单调栈的各个方面做了详细讲解,这里就不赘述了。 | ||
|
||
剩下就是分析清楚如下三种情况: | ||
|
||
* 情况一:当前遍历的元素heights[i]小于栈顶元素heights[st.top()]的情况 | ||
* 情况二:当前遍历的元素heights[i]等于栈顶元素heights[st.top()]的情况 | ||
* 情况三:当前遍历的元素heights[i]大于栈顶元素heights[st.top()]的情况 | ||
|
||
C++代码如下: | ||
|
||
```C++ | ||
// 版本一 | ||
class Solution { | ||
public: | ||
int largestRectangleArea(vector<int>& heights) { | ||
stack<int> st; | ||
heights.insert(heights.begin(), 0); // 数组头部加入元素0 | ||
heights.push_back(0); // 数组尾部加入元素0 | ||
st.push(0); | ||
int result = 0; | ||
// 第一个元素已经入栈,从下表1开始 | ||
for (int i = 1; i < heights.size(); i++) { | ||
// 注意heights[i] 是和heights[st.top()] 比较 ,st.top()是下表 | ||
if (heights[i] > heights[st.top()]) { | ||
st.push(i); | ||
} else if (heights[i] == heights[st.top()]) { | ||
st.pop(); // 这个可以加,可以不加,效果一样,思路不同 | ||
st.push(i); | ||
} else { | ||
while (heights[i] < heights[st.top()]) { // 注意是while | ||
int mid = st.top(); | ||
st.pop(); | ||
int left = st.top(); | ||
int right = i; | ||
int w = right - left - 1; | ||
int h = heights[mid]; | ||
result = max(result, w * h); | ||
} | ||
st.push(i); | ||
} | ||
} | ||
return result; | ||
} | ||
}; | ||
|
||
``` | ||
代码精简之后: | ||
```C++ | ||
// 版本二 | ||
class Solution { | ||
public: | ||
int largestRectangleArea(vector<int>& heights) { | ||
stack<int> st; | ||
heights.insert(heights.begin(), 0); // 数组头部加入元素0 | ||
heights.push_back(0); // 数组尾部加入元素0 | ||
st.push(0); | ||
int result = 0; | ||
for (int i = 1; i < heights.size(); i++) { | ||
while (heights[i] < heights[st.top()]) { | ||
int mid = st.top(); | ||
st.pop(); | ||
int w = i - st.top() - 1; | ||
int h = heights[mid]; | ||
result = max(result, w * h); | ||
} | ||
st.push(i); | ||
} | ||
return result; | ||
} | ||
}; | ||
``` | ||
|
||
这里我依然建议大家按部就班把版本一写出来,把情况一二三分析清楚,然后在精简代码到版本二。 直接看版本二容易忽略细节! | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
|
@@ -251,6 +251,8 @@ public: | |
# 相关题目推荐 | ||
这两道题目基本和本题是一样的,只要稍加修改就可以AC。 | ||
* 100.相同的树 | ||
* 572.另一个树的子树 | ||
|
Oops, something went wrong.