-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
1 changed file
with
116 additions
and
3 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,9 +1,122 @@ | ||
# GoHF | ||
# GoHF ✨ | ||
|
||
A new Go HTTP framework | ||
<img align="right" width="100px" src="https://raw.githubusercontent.com/gohf-http/assets/refs/heads/main/logo.png"> | ||
|
||
# Installation | ||
[](https://github.com/gohf-http/gohf/actions/workflows/test.yml) | ||
[](https://pkg.go.dev/github.com/gohf-http/gohf) | ||
[](https://github.com/gohf-http/gohf/releases) | ||
[](https://github.com/gohf-http/gohf#readme) | ||
[](https://github.com/gohf-http/gohf/graphs/commit-activity) | ||
[](https://github.com/gohf-http/gohf/blob/main/LICENSE) | ||
|
||
**GO** **H**ttp **F**ramework | ||
|
||
# ❓ WHY GoHF | ||
|
||
- [Easier error handling](#feature-easier-error-handing) | ||
- [Support middleware](#feature-middleware) | ||
- [Support sub-routers (route grouping)](#feature-sub-router) | ||
- [Customizable response](https://github.com/gohf-http/gohf/blob/main/gohf_responses/json_response.go) | ||
- Lightweight | ||
- Based on [net/http](https://pkg.go.dev/net/http) | ||
|
||
# 📍 Getting started | ||
|
||
```sh | ||
go get github.com/gohf-http/gohf | ||
``` | ||
|
||
[Hello GoHF Example](#-hello-gohf-example) | ||
|
||
# 🪄 Features | ||
|
||
### Feature: Easier Error Handing | ||
|
||
```go | ||
router.Handle("/greeting", func(c *gohf.Context) gohf.Response { | ||
name := c.Req.GetQuery("name") | ||
if name == "" { | ||
return gohf_responses.NewErrorResponse( | ||
http.StatusBadRequest, | ||
errors.New("Name is required"), | ||
) | ||
} | ||
|
||
greeting := fmt.Sprintf("Hello, %s!", name) | ||
return gohf_responses.NewTextResponse(http.StatusOK, greeting) | ||
}) | ||
``` | ||
|
||
### Feature: Middleware | ||
|
||
```go | ||
router.Use(func(c *gohf.Context) gohf.Response { | ||
token := c.Req.GetHeader("Authorization") | ||
if !isValidToken(token) { | ||
return gohf_responses.NewErrorResponse( | ||
http.StatusUnauthorized, | ||
errors.New("Invalid token"), | ||
) | ||
} | ||
|
||
return c.Next() | ||
}) | ||
``` | ||
|
||
This is how middleware works in GoHF. | ||
|
||
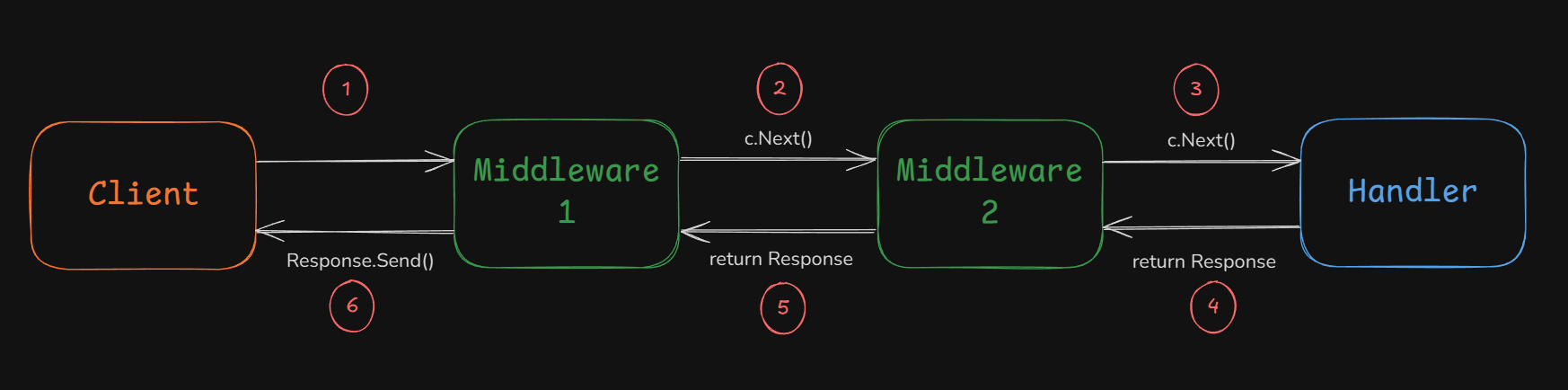 | ||
|
||
### Feature: Sub-Router | ||
|
||
```go | ||
authRouter := router.SubRouter("/auth") | ||
authRouter.Use(AuthMiddleware) | ||
authRouter.Handle("GET /users", func(c *gohf.Context) gohf.Response { | ||
// ... | ||
}) | ||
``` | ||
|
||
# 📘 Hello GoHF Example | ||
|
||
```go | ||
package main | ||
|
||
import ( | ||
"errors" | ||
"fmt" | ||
"log" | ||
"net/http" | ||
|
||
"github.com/gohf-http/gohf" | ||
"github.com/gohf-http/gohf/gohf_responses" | ||
) | ||
|
||
func main() { | ||
router := gohf.New() | ||
|
||
router.Handle("/greeting", func(c *gohf.Context) gohf.Response { | ||
name := c.Req.GetQuery("name") | ||
if name == "" { | ||
return gohf_responses.NewErrorResponse( | ||
http.StatusBadRequest, | ||
errors.New("Name is required"), | ||
) | ||
} | ||
|
||
greeting := fmt.Sprintf("Hello, %s!", name) | ||
return gohf_responses.NewTextResponse(http.StatusOK, greeting) | ||
}) | ||
|
||
router.Use(func(c *gohf.Context) gohf.Response { | ||
return gohf_responses.NewErrorResponse( | ||
http.StatusNotFound, | ||
errors.New("Page not found"), | ||
) | ||
}) | ||
|
||
mux := router.CreateServeMux() | ||
log.Fatal(http.ListenAndServe(":8080", mux)) | ||
} | ||
``` |