- Add transfomer class for MLKR (Metric Learning for Kernel Regression) that performs transformation/projection along to the target variables. The following are examples of transforming toy example data using PCA and MLKR.
require 'rumale'
def make_regression(n_samples: 500, n_features: 10, n_informative: 4, n_targets: 1)
n_informative = [n_features, n_informative].min
rng = Random.new(42)
x = Rumale::Utils.rand_normal([n_samples, n_features], rng)
ground_truth = Numo::DFloat.zeros(n_features, n_targets)
ground_truth[0...n_informative, true] = 100 * Rumale::Utils.rand_uniform([n_informative, n_targets], rng)
y = x.dot(ground_truth)
y = y.flatten
rand_ids = Array(0...n_samples).shuffle(random: rng)
x = x[rand_ids, true].dup
y = y[rand_ids].dup
[x, y]
end
x, y = make_regression
pca = Rumale::Decomposition::PCA.new(n_components: 10)
z_pca = pca.fit_transform(x, y)
mlkr = Rumale::MetricLearning::MLKR.new(n_components: nil, init: 'pca')
z_mlkr = mlkr.fit_transform(x, y)
# After that, these results are visualized by multidimensional scaling.
PCA:
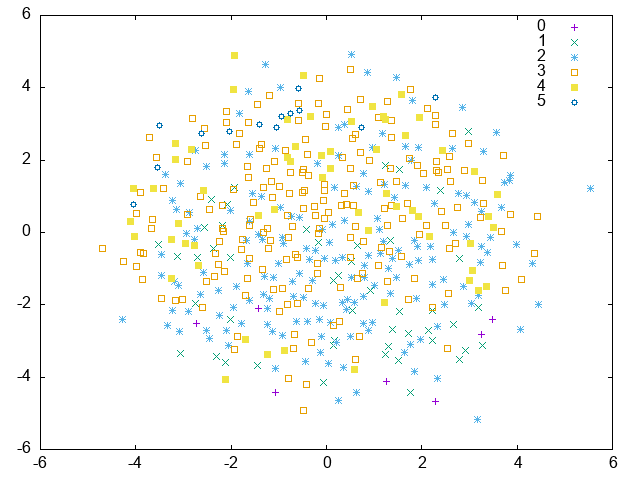
MLKR:
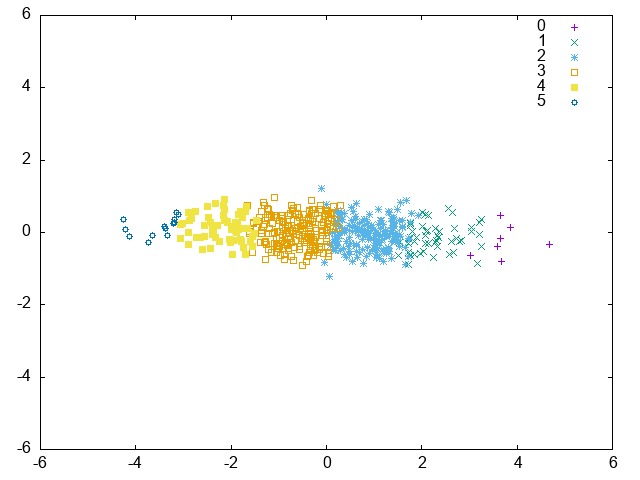