-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
7 changed files
with
191 additions
and
14 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,37 @@ | ||
@[toc] | ||
|
||
# 题目描述 | ||
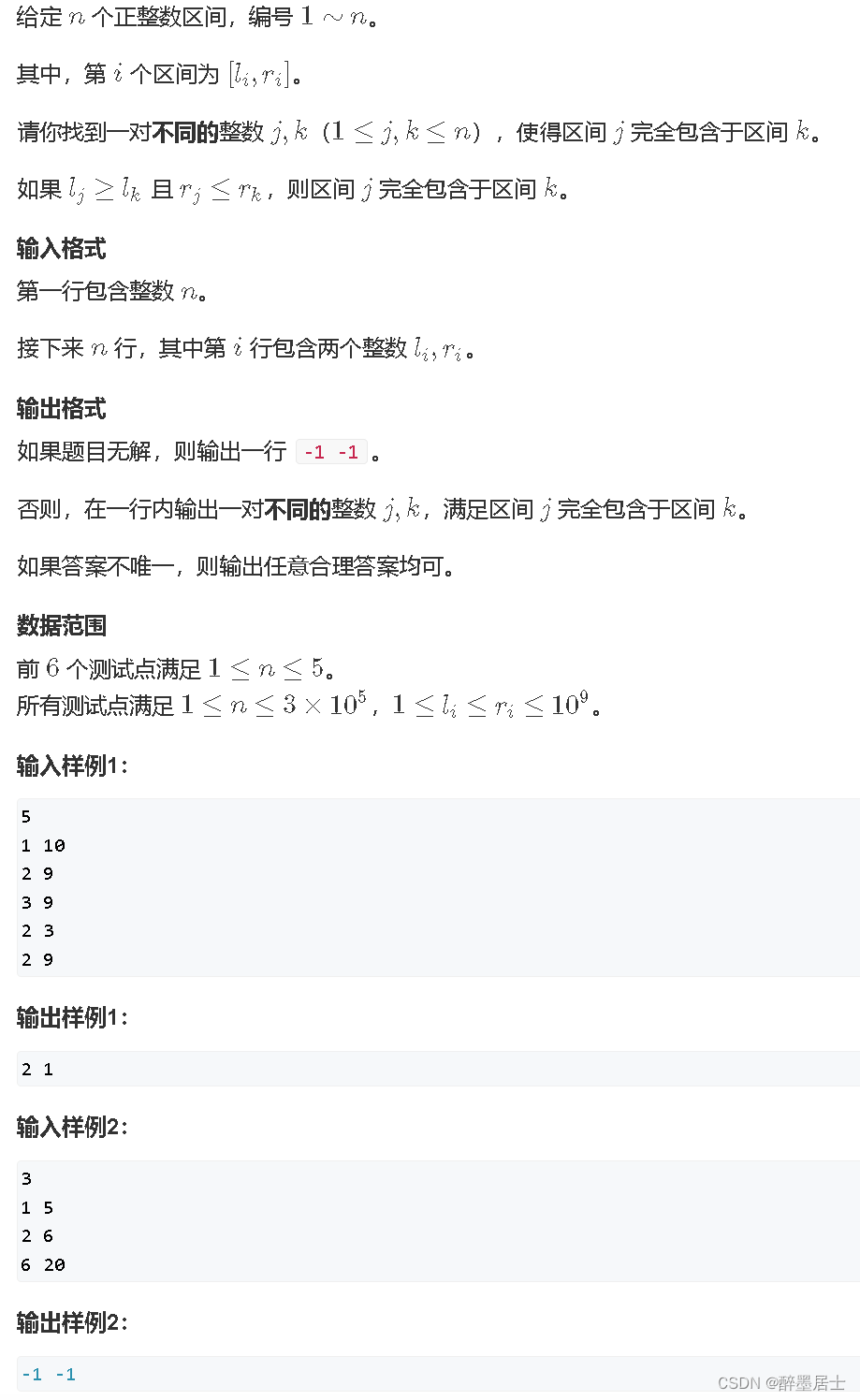 | ||
|
||
# 题解思路 | ||
记录所有区间和区间对应的索引 | ||
|
||
按照区间左端点进行排序 | ||
|
||
然后遍历排序后的区间 | ||
|
||
如果当前区间的右端点相比于前一个区间的右端点 有所上升或者不变则输出当前区间的索引和前一个区间的索引,然后结束循环 | ||
|
||
如果当前区间的左端点等于前一个区间的左端点,则输出前一个区间的索引和当前区间的索引,然后结束循环 | ||
|
||
如果区间遍历完毕还没找到满足条件的区间,则输出-1, -1 | ||
|
||
# 题解代码 | ||
```python | ||
n = int(input()) | ||
parts = [] | ||
for i in range(n): | ||
a, b = map(int, input().split()) | ||
parts.append([a,b,i]) | ||
|
||
parts.sort() | ||
for i in range(1, n): | ||
if parts[i][1] <= parts[i-1][1]: | ||
print(parts[i][2] + 1, parts[i-1][2] + 1) | ||
break | ||
if parts[i][0] == parts[i-1][0]: | ||
print(parts[i-1][2] + 1, parts[i][2] + 1) | ||
break | ||
else: | ||
print('-1 -1') | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,40 @@ | ||
@[toc] | ||
|
||
# 题目描述 | ||
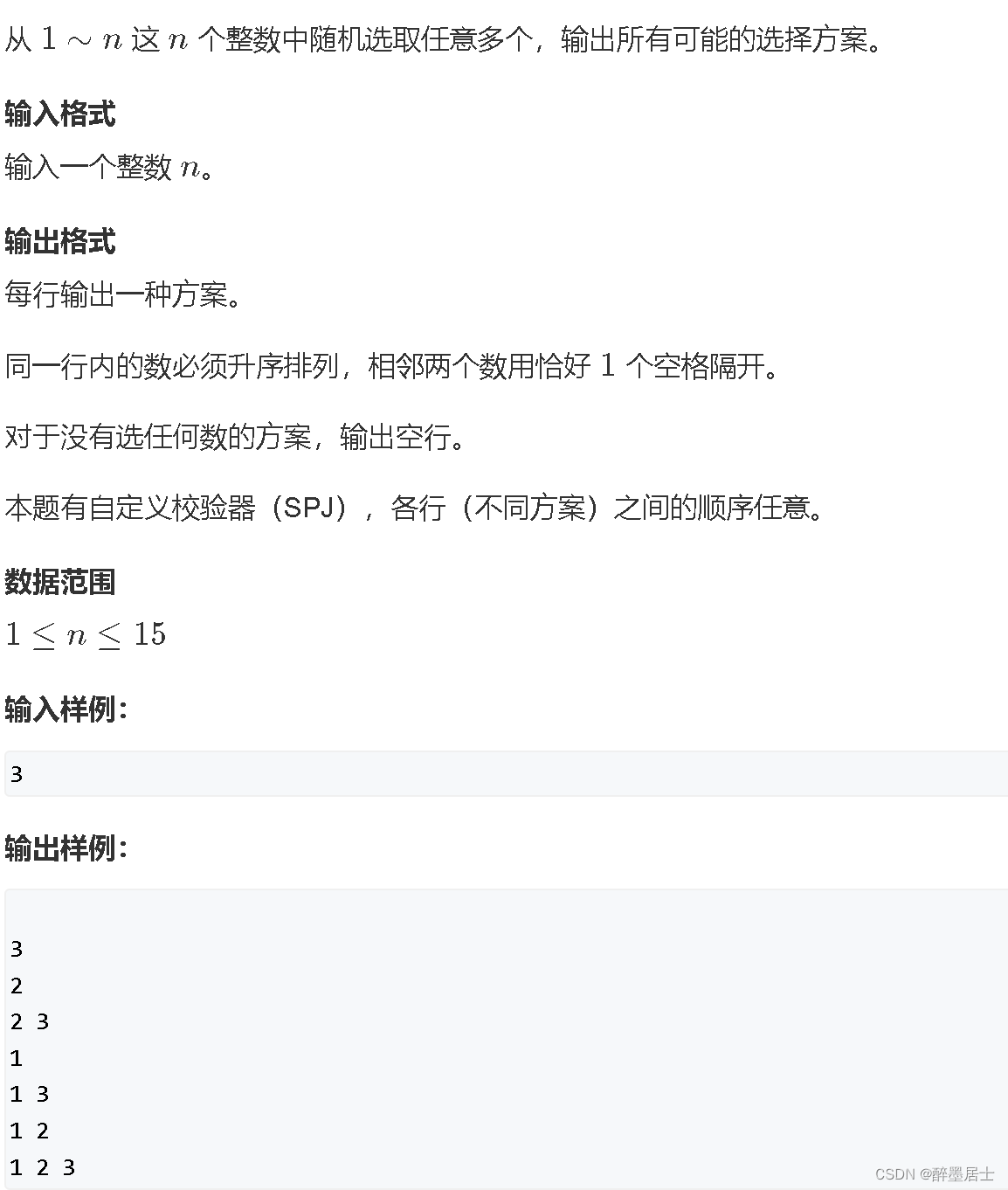 | ||
|
||
# 题解思路 | ||
本题相当于二叉树的深度优先遍历,树的第i层是第i个数选或不选 | ||
我们记录当前递归的深度deep | ||
然后用state进行状态压缩,state第i位是1表示选第i个数,第i位是0表示不选第i个数 | ||
进行dfs | ||
如果当前深度为n,则说明当前已经递归完前n层,此时将state对应要选择的数打印出来,然后返回 | ||
深度加一 | ||
state不变动,表示不选当前层对应的数,然后进行递归 | ||
state当前位 置为1,表示选择当前层对应的数,然后进行递归 | ||
深度减一 | ||
state当前位 置为0 | ||
|
||
直到递归结束,程序退出 | ||
# 题解代码 | ||
```python | ||
n = int(input()) | ||
deep = 0 | ||
state = 0 | ||
def dfs(): | ||
global deep | ||
global state | ||
if deep == n: | ||
for i in range(n): | ||
if state >> i & 1 == 1: | ||
print(i + 1, end=' ') | ||
print() | ||
return | ||
deep += 1 | ||
dfs() | ||
state = state | 1 << (deep - 1) | ||
dfs() | ||
deep -= 1 | ||
state -= 1 << deep | ||
dfs() | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,57 @@ | ||
@[toc] | ||
|
||
# 题目描述 | ||
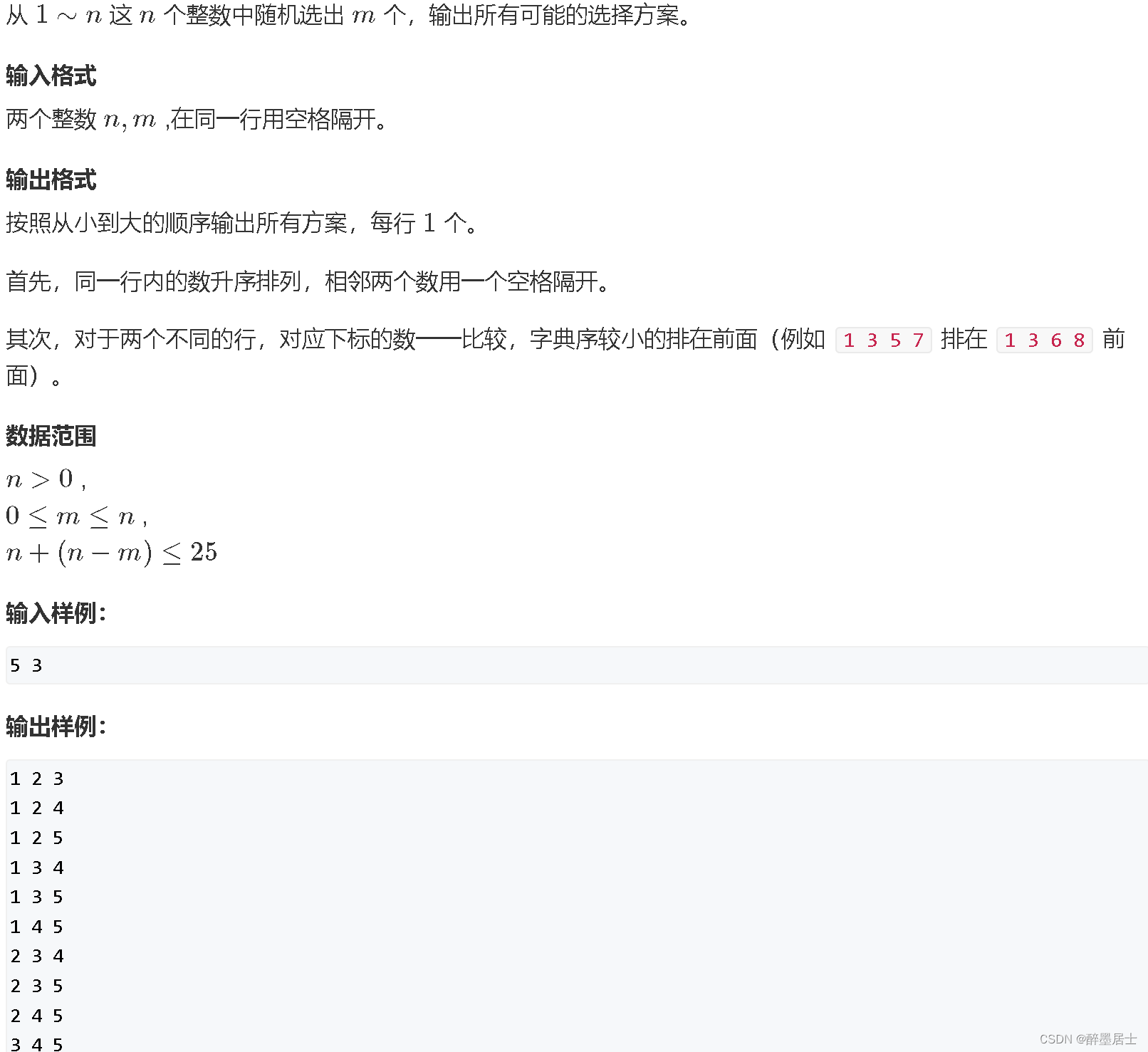 | ||
|
||
# 题解思路 | ||
本题相当于二叉树的深度优先遍历,树的第i层表示第i个数选或不选,当选择了m次左节点后退出 | ||
我们记录当前递归的深度deep | ||
然后用state进行状态压缩,state第i位是1表示选第i个数,第i位是0表示不选第i个数 | ||
count表示我们选择数的个数 | ||
|
||
进行dfs | ||
|
||
当前还能选择的数的个数即n - deep,当前还应选择的数的个数即m - count | ||
如果当前还能选择的数的个数小于当前还应选择的数的个数,则退出搜索 | ||
|
||
如果当前选择的数的个数为m,则说明当前数已经选完,此时将state对应要选择的数打印出来,然后返回 | ||
|
||
state当前位 置为1,深度加一,当前选择的数的个数加一,表示选择当前层对应的数,然后进行递归 | ||
|
||
恢复state和count当前层初始的state和count | ||
进行递归 | ||
深度减一 | ||
|
||
直到递归结束,程序退出 | ||
|
||
# 题解代码 | ||
```python | ||
n, m = map(int, input().split()) | ||
|
||
deep = 0 | ||
state = 0 | ||
count = 0 | ||
def dfs(): | ||
global deep | ||
global state | ||
global count | ||
if n - deep < m - count: | ||
return | ||
if count == m: | ||
for i in range(n): | ||
if state >> i & 1 == 1: | ||
print(i + 1, end = ' ') | ||
print() | ||
return | ||
|
||
state |= 1 << deep | ||
deep += 1 | ||
count += 1 | ||
dfs() | ||
state -= 1 << (deep - 1) | ||
count -= 1 | ||
|
||
dfs() | ||
deep -= 1 | ||
dfs() | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,42 @@ | ||
@[toc] | ||
|
||
# 题目描述 | ||
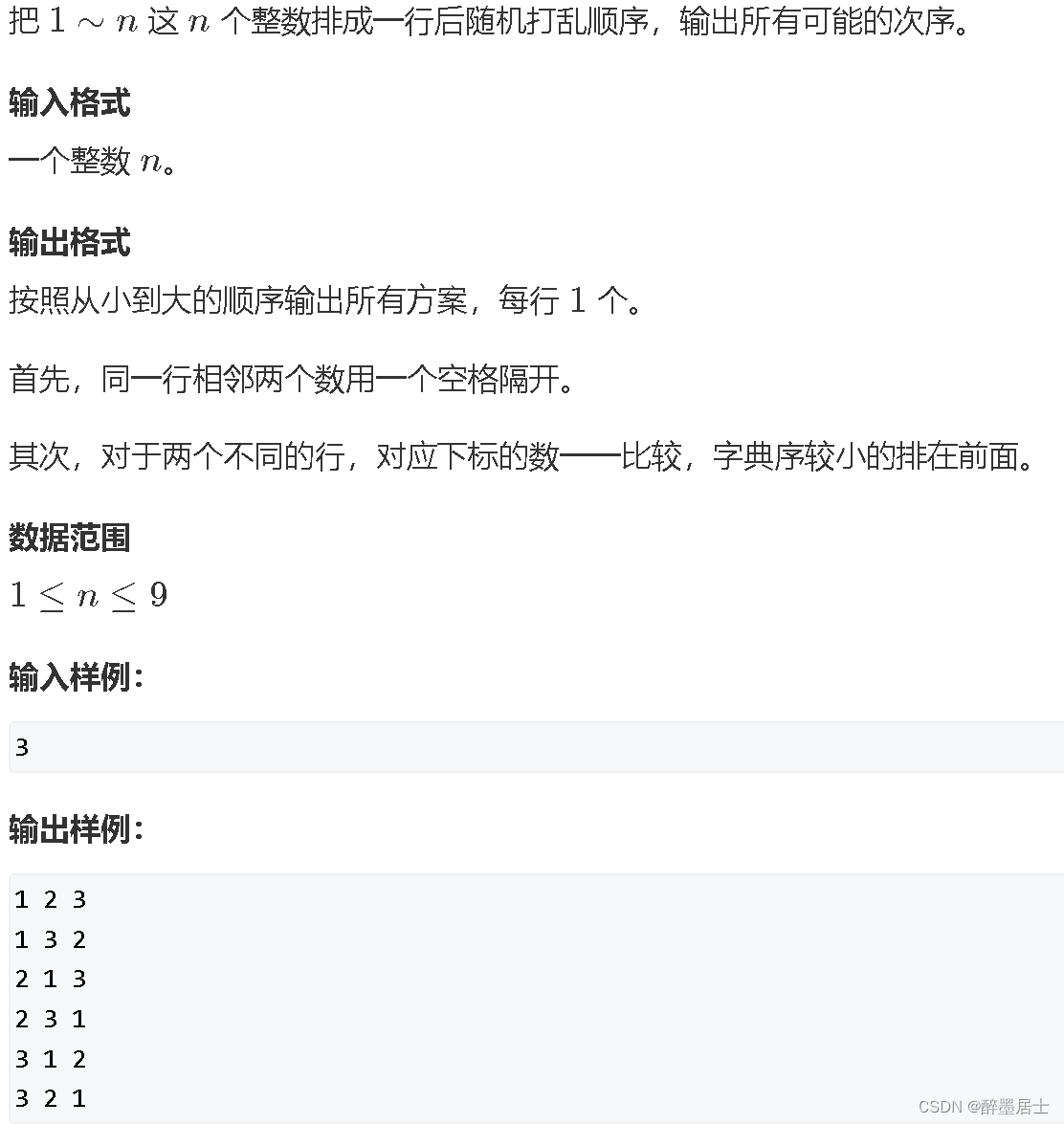 | ||
|
||
# 题解思路 | ||
定义递归深度deep,数字使用情况used,选择的数字顺序path | ||
|
||
进行递归 | ||
|
||
终止条件为递归深度达到n层时,打印path,然后返回 | ||
|
||
深度加一 | ||
遍历未使用的数字,选择数字,然后进行递归,递归结束,恢复used | ||
恢复深度 | ||
|
||
直到整个递归结束,程序结束 | ||
# 题解代码 | ||
```python | ||
n = int(input()) | ||
|
||
used = 0 | ||
deep = 0 | ||
path = [0 for _ in range(n)] | ||
def dfs(): | ||
global used | ||
global deep | ||
if deep == n: | ||
for i in range(n): | ||
print(path[i], end=' ') | ||
print() | ||
return | ||
deep += 1 | ||
for i in range(n): | ||
if used >> i & 1 == 0: | ||
path[deep - 1] = i + 1 | ||
used |= 1 << i | ||
dfs() | ||
used -= 1 << i | ||
deep -= 1 | ||
dfs() | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters